Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / DbModificationCommandTree.cs / 1305376 / DbModificationCommandTree.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; using System.Data.Common.Utils; using System.Linq; namespace System.Data.Common.CommandTrees { ////// Represents a DML operation expressed as a canonical command tree /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbModificationCommandTree : DbCommandTree { private readonly DbExpressionBinding _target; private System.Collections.ObjectModel.ReadOnlyCollection_parameters; internal DbModificationCommandTree(MetadataWorkspace metadata, DataSpace dataSpace, DbExpressionBinding target) : base(metadata, dataSpace) { EntityUtil.CheckArgumentNull(target, "target"); this._target = target; } /// /// Gets the public DbExpressionBinding Target { get { return _target; } } ///that specifies the target table for the DML operation. /// /// Returns true if this modification command returns a reader (for instance, to return server generated values) /// internal abstract bool HasReader { get; } internal override IEnumerable> GetParameters() { if (this._parameters == null) { this._parameters = ParameterRetriever.GetParameters(this); } return this._parameters.Select(p => new KeyValuePair (p.ParameterName, p.ResultType)); } internal override void DumpStructure(ExpressionDumper dumper) { if (this.Target != null) { dumper.Dump(this.Target, "Target"); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; using System.Data.Common.Utils; using System.Linq; namespace System.Data.Common.CommandTrees { ////// Represents a DML operation expressed as a canonical command tree /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbModificationCommandTree : DbCommandTree { private readonly DbExpressionBinding _target; private System.Collections.ObjectModel.ReadOnlyCollection_parameters; internal DbModificationCommandTree(MetadataWorkspace metadata, DataSpace dataSpace, DbExpressionBinding target) : base(metadata, dataSpace) { EntityUtil.CheckArgumentNull(target, "target"); this._target = target; } /// /// Gets the public DbExpressionBinding Target { get { return _target; } } ///that specifies the target table for the DML operation. /// /// Returns true if this modification command returns a reader (for instance, to return server generated values) /// internal abstract bool HasReader { get; } internal override IEnumerable> GetParameters() { if (this._parameters == null) { this._parameters = ParameterRetriever.GetParameters(this); } return this._parameters.Select(p => new KeyValuePair (p.ParameterName, p.ResultType)); } internal override void DumpStructure(ExpressionDumper dumper) { if (this.Target != null) { dumper.Dump(this.Target, "Target"); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
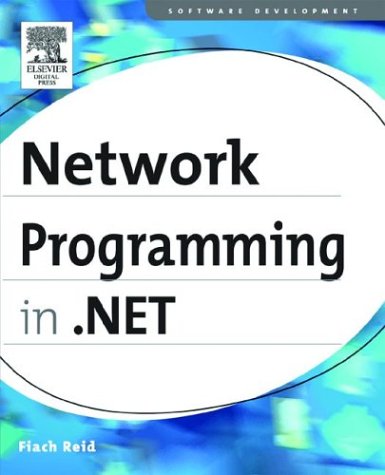
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FreezableDefaultValueFactory.cs
- TypeInfo.cs
- OutgoingWebRequestContext.cs
- ContainerControl.cs
- XmlTextWriter.cs
- WebConvert.cs
- QilSortKey.cs
- ListComponentEditor.cs
- DesignDataSource.cs
- DocumentScope.cs
- RunInstallerAttribute.cs
- KnownTypesProvider.cs
- LocalizableResourceBuilder.cs
- EntityDataSourceColumn.cs
- ObjectSecurity.cs
- Typeface.cs
- SrgsElement.cs
- SerTrace.cs
- AnnotationHelper.cs
- TemplatedWizardStep.cs
- AuthenticationServiceManager.cs
- XslException.cs
- RadioButtonList.cs
- DataServiceExpressionVisitor.cs
- ProviderCommandInfoUtils.cs
- HttpWebResponse.cs
- CurrentChangedEventManager.cs
- CellRelation.cs
- ToolboxItemAttribute.cs
- SignatureToken.cs
- _NegoState.cs
- ZipIOCentralDirectoryFileHeader.cs
- DoubleCollectionValueSerializer.cs
- QilXmlReader.cs
- FileDialog_Vista.cs
- WindowsAuthenticationEventArgs.cs
- ThemeInfoAttribute.cs
- DomainUpDown.cs
- VisualBrush.cs
- KeyedCollection.cs
- Accessible.cs
- QilScopedVisitor.cs
- SoapSchemaMember.cs
- Privilege.cs
- MouseButton.cs
- ImageDrawing.cs
- BindingCollectionElement.cs
- HttpModuleActionCollection.cs
- BaseProcessor.cs
- ResourceContainer.cs
- ScrollPatternIdentifiers.cs
- PassportIdentity.cs
- LabelDesigner.cs
- Debug.cs
- XmlSchemaProviderAttribute.cs
- Root.cs
- ReliableChannelFactory.cs
- DiscoveryReferences.cs
- PrintSystemException.cs
- FormatterConverter.cs
- PageAsyncTask.cs
- HybridObjectCache.cs
- propertyentry.cs
- PrivilegeNotHeldException.cs
- VisualStates.cs
- Nullable.cs
- UseAttributeSetsAction.cs
- Literal.cs
- BinaryUtilClasses.cs
- UniqueConstraint.cs
- SystemBrushes.cs
- DiagnosticTrace.cs
- Simplifier.cs
- ScrollableControlDesigner.cs
- FlowPosition.cs
- FamilyTypefaceCollection.cs
- StructuredType.cs
- VisualState.cs
- WebProxyScriptElement.cs
- ImmComposition.cs
- ColorMap.cs
- XmlFileEditor.cs
- SettingsSection.cs
- PrintPreviewControl.cs
- EntityTypeBase.cs
- StretchValidation.cs
- GeometryHitTestParameters.cs
- DataBindingHandlerAttribute.cs
- FieldNameLookup.cs
- MethodImplAttribute.cs
- Html32TextWriter.cs
- ResetableIterator.cs
- ProjectionQueryOptionExpression.cs
- TTSEvent.cs
- AllMembershipCondition.cs
- DataBinding.cs
- SolidBrush.cs
- JoinGraph.cs
- WorkflowRuntimeServiceElement.cs
- SystemTcpConnection.cs