Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CommonUI / System / Drawing / SolidBrush.cs / 1 / SolidBrush.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Runtime.InteropServices; using System.Diagnostics; using System; using Microsoft.Win32; using System.ComponentModel; using System.Drawing.Internal; ////// /// public sealed class SolidBrush : Brush, ISystemColorTracker { // GDI+ doesn't understand system colors, so we need to cache the value here private Color color = Color.Empty; private bool immutable; /** * Create a new solid fill brush object */ ////// Defines a brush made up of a single color. Brushes are /// used to fill graphics shapes such as rectangles, ellipses, pies, polygons, and paths. /// ////// /// public SolidBrush(Color color) { this.color = color; IntPtr brush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateSolidFill(this.color.ToArgb(), out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrush(brush); if (color.IsSystemColor) SystemColorTracker.Add(this); } internal SolidBrush(Color color, bool immutable) : this(color) { this.immutable = immutable; } ////// Initializes a new instance of the ///class of the specified /// color. /// /// Constructor to initialized this object from a GDI+ Brush native pointer. /// internal SolidBrush( IntPtr nativeBrush ) { Debug.Assert( nativeBrush != IntPtr.Zero, "Initializing native brush with null." ); SetNativeBrush( nativeBrush ); } ////// /// Creates an exact copy of this public override object Clone() { IntPtr cloneBrush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCloneBrush(new HandleRef(this, this.NativeBrush), out cloneBrush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); // We intentionally lose the "immutable" bit. return new SolidBrush(cloneBrush); } ///. /// protected override void Dispose(bool disposing) { if (!disposing) { immutable = false; } else if (immutable) { throw new ArgumentException(SR.GetString(SR.CantChangeImmutableObjects, "Brush")); } base.Dispose(disposing); } /// /// /// public Color Color { get { if (this.color == Color.Empty) { int colorARGB = 0; int status = SafeNativeMethods.Gdip.GdipGetSolidFillColor(new HandleRef(this, this.NativeBrush), out colorARGB); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); this.color = Color.FromArgb(colorARGB); } // GDI+ doesn't understand system colors, so we can't use GdipGetSolidFillColor in the general case return this.color; } set { if (immutable) { throw new ArgumentException(SR.GetString(SR.CantChangeImmutableObjects, "Brush")); } if( this.color != value ) { Color oldColor = this.color; InternalSetColor(value); // if (value.IsSystemColor && !oldColor.IsSystemColor) { SystemColorTracker.Add(this); } } } } // Sets the color even if the brush is considered immutable private void InternalSetColor(Color value) { int status = SafeNativeMethods.Gdip.GdipSetSolidFillColor(new HandleRef(this, this.NativeBrush), value.ToArgb()); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); this.color = value; } ////// The color of this ///. /// /// void ISystemColorTracker.OnSystemColorChanged() { if( this.NativeBrush != IntPtr.Zero ){ InternalSetColor(color); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Runtime.InteropServices; using System.Diagnostics; using System; using Microsoft.Win32; using System.ComponentModel; using System.Drawing.Internal; ////// /// public sealed class SolidBrush : Brush, ISystemColorTracker { // GDI+ doesn't understand system colors, so we need to cache the value here private Color color = Color.Empty; private bool immutable; /** * Create a new solid fill brush object */ ////// Defines a brush made up of a single color. Brushes are /// used to fill graphics shapes such as rectangles, ellipses, pies, polygons, and paths. /// ////// /// public SolidBrush(Color color) { this.color = color; IntPtr brush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateSolidFill(this.color.ToArgb(), out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrush(brush); if (color.IsSystemColor) SystemColorTracker.Add(this); } internal SolidBrush(Color color, bool immutable) : this(color) { this.immutable = immutable; } ////// Initializes a new instance of the ///class of the specified /// color. /// /// Constructor to initialized this object from a GDI+ Brush native pointer. /// internal SolidBrush( IntPtr nativeBrush ) { Debug.Assert( nativeBrush != IntPtr.Zero, "Initializing native brush with null." ); SetNativeBrush( nativeBrush ); } ////// /// Creates an exact copy of this public override object Clone() { IntPtr cloneBrush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCloneBrush(new HandleRef(this, this.NativeBrush), out cloneBrush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); // We intentionally lose the "immutable" bit. return new SolidBrush(cloneBrush); } ///. /// protected override void Dispose(bool disposing) { if (!disposing) { immutable = false; } else if (immutable) { throw new ArgumentException(SR.GetString(SR.CantChangeImmutableObjects, "Brush")); } base.Dispose(disposing); } /// /// /// public Color Color { get { if (this.color == Color.Empty) { int colorARGB = 0; int status = SafeNativeMethods.Gdip.GdipGetSolidFillColor(new HandleRef(this, this.NativeBrush), out colorARGB); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); this.color = Color.FromArgb(colorARGB); } // GDI+ doesn't understand system colors, so we can't use GdipGetSolidFillColor in the general case return this.color; } set { if (immutable) { throw new ArgumentException(SR.GetString(SR.CantChangeImmutableObjects, "Brush")); } if( this.color != value ) { Color oldColor = this.color; InternalSetColor(value); // if (value.IsSystemColor && !oldColor.IsSystemColor) { SystemColorTracker.Add(this); } } } } // Sets the color even if the brush is considered immutable private void InternalSetColor(Color value) { int status = SafeNativeMethods.Gdip.GdipSetSolidFillColor(new HandleRef(this, this.NativeBrush), value.ToArgb()); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); this.color = value; } ////// The color of this ///. /// /// void ISystemColorTracker.OnSystemColorChanged() { if( this.NativeBrush != IntPtr.Zero ){ InternalSetColor(color); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
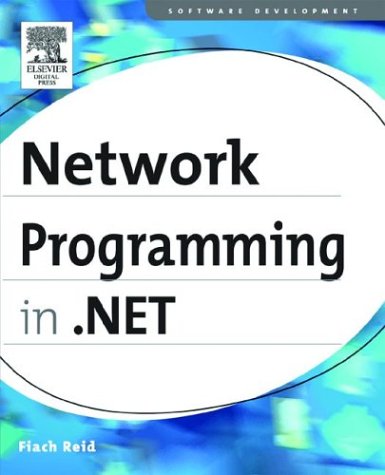
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeHelper.cs
- CorrelationValidator.cs
- TableRow.cs
- TableHeaderCell.cs
- _Events.cs
- Thumb.cs
- PersonalizationAdministration.cs
- VirtualDirectoryMappingCollection.cs
- Brush.cs
- LoginAutoFormat.cs
- PopupRoot.cs
- DecoderReplacementFallback.cs
- DateTime.cs
- HttpClientChannel.cs
- SatelliteContractVersionAttribute.cs
- ColorAnimation.cs
- SqlHelper.cs
- LoadMessageLogger.cs
- AddressHeader.cs
- Margins.cs
- TabletCollection.cs
- SimpleHandlerFactory.cs
- FactoryGenerator.cs
- OutgoingWebResponseContext.cs
- TextControlDesigner.cs
- ProcessModuleDesigner.cs
- SocketAddress.cs
- UserControlBuildProvider.cs
- OrderPreservingMergeHelper.cs
- InfoCardClaim.cs
- AnonymousIdentificationSection.cs
- TypeCodeDomSerializer.cs
- SelectingProviderEventArgs.cs
- SyntaxCheck.cs
- GroupBoxAutomationPeer.cs
- graph.cs
- InkCollectionBehavior.cs
- RuntimeEnvironment.cs
- InkCanvas.cs
- TitleStyle.cs
- SupportingTokenBindingElement.cs
- SamlAuthorizationDecisionStatement.cs
- TaskDesigner.cs
- ItemsPanelTemplate.cs
- _SingleItemRequestCache.cs
- XmlValidatingReaderImpl.cs
- PropertyGeneratedEventArgs.cs
- SingleConverter.cs
- CryptographicAttribute.cs
- arc.cs
- SendingRequestEventArgs.cs
- XmlHierarchicalEnumerable.cs
- __TransparentProxy.cs
- ExpressionPrefixAttribute.cs
- ApplicationContext.cs
- PassportAuthentication.cs
- EntityDataSourceMemberPath.cs
- UserControlAutomationPeer.cs
- GatewayIPAddressInformationCollection.cs
- DataGridViewCellConverter.cs
- RelatedImageListAttribute.cs
- CompiledRegexRunnerFactory.cs
- ValidatorCompatibilityHelper.cs
- StylusTip.cs
- TypeConverterHelper.cs
- SqlUDTStorage.cs
- srgsitem.cs
- MasterPageBuildProvider.cs
- URLIdentityPermission.cs
- LabelExpression.cs
- TransformerInfo.cs
- ToolStripScrollButton.cs
- xdrvalidator.cs
- ThumbAutomationPeer.cs
- WeakKeyDictionary.cs
- DataChangedEventManager.cs
- NestedContainer.cs
- ProfileSettingsCollection.cs
- DesignRelation.cs
- QilStrConcatenator.cs
- DeadCharTextComposition.cs
- MinimizableAttributeTypeConverter.cs
- DrawingState.cs
- SimpleBitVector32.cs
- VolatileEnlistmentMultiplexing.cs
- ApplicationHost.cs
- WindowsHyperlink.cs
- ProfileEventArgs.cs
- XhtmlBasicLinkAdapter.cs
- HostedNamedPipeTransportManager.cs
- WebConfigurationFileMap.cs
- ArraySet.cs
- GridViewSelectEventArgs.cs
- WebPartConnectionsCancelEventArgs.cs
- XmlObjectSerializerReadContextComplexJson.cs
- XmlSerializationWriter.cs
- BaseWebProxyFinder.cs
- HttpCapabilitiesSectionHandler.cs
- SessionIDManager.cs
- ExecutionScope.cs