Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / ApplicationContext.cs / 1 / ApplicationContext.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Diagnostics; using System.ComponentModel; ////// /// ApplicationContext provides contextual information about an application /// thread. Specifically this allows an application author to redifine what /// circurmstances cause a message loop to exit. By default the application /// context listens to the close event on the mainForm, then exits the /// thread's message loop. /// public class ApplicationContext : IDisposable { Form mainForm; object userData; ////// /// Creates a new ApplicationContext with no mainForm. /// public ApplicationContext() : this(null) { } ////// /// Creates a new ApplicationContext with the specified mainForm. /// If OnMainFormClosed is not overriden, the thread's message /// loop will be terminated when mainForm is closed. /// public ApplicationContext(Form mainForm) { this.MainForm = mainForm; } ///~ApplicationContext() { Dispose(false); } /// /// /// Determines the mainForm for this context. This may be changed /// at anytime. /// If OnMainFormClosed is not overriden, the thread's message /// loop will be terminated when mainForm is closed. /// public Form MainForm { get { return mainForm; } set { EventHandler onClose = new EventHandler(OnMainFormDestroy); if (mainForm != null) { mainForm.HandleDestroyed -= onClose; } mainForm = value; if (mainForm != null) { mainForm.HandleDestroyed += onClose; } } } ///[ SRCategory(SR.CatData), Localizable(false), Bindable(true), SRDescription(SR.ControlTagDescr), DefaultValue(null), TypeConverter(typeof(StringConverter)), ] public object Tag { get { return userData; } set { userData = value; } } /// /// /// Is raised when the thread's message loop should be terminated. /// This is raised by calling ExitThread. /// public event EventHandler ThreadExit; ////// /// Disposes the context. This should dispose the mainForm. This is /// called immediately after the thread's message loop is terminated. /// Application will dispose all forms on this thread by default. /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ///protected virtual void Dispose(bool disposing) { if (disposing) { if (mainForm != null) { if (!mainForm.IsDisposed) { mainForm.Dispose(); } mainForm = null; } } } /// /// /// Causes the thread's message loop to be terminated. This /// will call ExitThreadCore. /// public void ExitThread() { ExitThreadCore(); } ////// /// Causes the thread's message loop to be terminated. /// protected virtual void ExitThreadCore() { if (ThreadExit != null) { ThreadExit(this, EventArgs.Empty); } } ////// /// Called when the mainForm is closed. The default implementation /// of this will call ExitThreadCore. /// protected virtual void OnMainFormClosed(object sender, EventArgs e) { ExitThreadCore(); } ////// Called when the mainForm is closed. The default implementation /// of this will call ExitThreadCore. /// private void OnMainFormDestroy(object sender, EventArgs e) { Form form = (Form)sender; if (!form.RecreatingHandle) { form.HandleDestroyed -= new EventHandler(OnMainFormDestroy); OnMainFormClosed(sender, e); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Diagnostics; using System.ComponentModel; ////// /// ApplicationContext provides contextual information about an application /// thread. Specifically this allows an application author to redifine what /// circurmstances cause a message loop to exit. By default the application /// context listens to the close event on the mainForm, then exits the /// thread's message loop. /// public class ApplicationContext : IDisposable { Form mainForm; object userData; ////// /// Creates a new ApplicationContext with no mainForm. /// public ApplicationContext() : this(null) { } ////// /// Creates a new ApplicationContext with the specified mainForm. /// If OnMainFormClosed is not overriden, the thread's message /// loop will be terminated when mainForm is closed. /// public ApplicationContext(Form mainForm) { this.MainForm = mainForm; } ///~ApplicationContext() { Dispose(false); } /// /// /// Determines the mainForm for this context. This may be changed /// at anytime. /// If OnMainFormClosed is not overriden, the thread's message /// loop will be terminated when mainForm is closed. /// public Form MainForm { get { return mainForm; } set { EventHandler onClose = new EventHandler(OnMainFormDestroy); if (mainForm != null) { mainForm.HandleDestroyed -= onClose; } mainForm = value; if (mainForm != null) { mainForm.HandleDestroyed += onClose; } } } ///[ SRCategory(SR.CatData), Localizable(false), Bindable(true), SRDescription(SR.ControlTagDescr), DefaultValue(null), TypeConverter(typeof(StringConverter)), ] public object Tag { get { return userData; } set { userData = value; } } /// /// /// Is raised when the thread's message loop should be terminated. /// This is raised by calling ExitThread. /// public event EventHandler ThreadExit; ////// /// Disposes the context. This should dispose the mainForm. This is /// called immediately after the thread's message loop is terminated. /// Application will dispose all forms on this thread by default. /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ///protected virtual void Dispose(bool disposing) { if (disposing) { if (mainForm != null) { if (!mainForm.IsDisposed) { mainForm.Dispose(); } mainForm = null; } } } /// /// /// Causes the thread's message loop to be terminated. This /// will call ExitThreadCore. /// public void ExitThread() { ExitThreadCore(); } ////// /// Causes the thread's message loop to be terminated. /// protected virtual void ExitThreadCore() { if (ThreadExit != null) { ThreadExit(this, EventArgs.Empty); } } ////// /// Called when the mainForm is closed. The default implementation /// of this will call ExitThreadCore. /// protected virtual void OnMainFormClosed(object sender, EventArgs e) { ExitThreadCore(); } ////// Called when the mainForm is closed. The default implementation /// of this will call ExitThreadCore. /// private void OnMainFormDestroy(object sender, EventArgs e) { Form form = (Form)sender; if (!form.RecreatingHandle) { form.HandleDestroyed -= new EventHandler(OnMainFormDestroy); OnMainFormClosed(sender, e); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
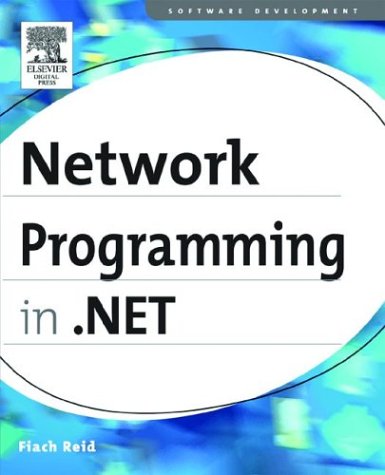
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StateItem.cs
- input.cs
- Int64.cs
- OrderedDictionary.cs
- HtmlProps.cs
- HttpListenerPrefixCollection.cs
- ListBoxChrome.cs
- CollectionChangeEventArgs.cs
- LinqExpressionNormalizer.cs
- HostingEnvironmentSection.cs
- QuaternionAnimationUsingKeyFrames.cs
- MD5.cs
- IIS7WorkerRequest.cs
- GridViewColumnCollectionChangedEventArgs.cs
- TabletDeviceInfo.cs
- FontStretch.cs
- ObjectStateManager.cs
- SByteConverter.cs
- AccessibilityHelperForVista.cs
- HttpResponse.cs
- XmlBaseReader.cs
- FormView.cs
- SafeProcessHandle.cs
- SqlNotificationEventArgs.cs
- FeatureSupport.cs
- IsolatedStorageFileStream.cs
- TdsParserSessionPool.cs
- InputScopeManager.cs
- MimeTypePropertyAttribute.cs
- SkewTransform.cs
- BasicViewGenerator.cs
- OleDbStruct.cs
- Polyline.cs
- ReferentialConstraint.cs
- Aggregates.cs
- ListBindingConverter.cs
- VolatileResourceManager.cs
- TreeNode.cs
- UnmanagedMemoryStreamWrapper.cs
- AccessDataSourceView.cs
- DBCSCodePageEncoding.cs
- Int32Converter.cs
- EmptyEnumerable.cs
- WebBrowserDocumentCompletedEventHandler.cs
- CodeDomSerializerBase.cs
- PreviousTrackingServiceAttribute.cs
- MemberInfoSerializationHolder.cs
- ContainerAction.cs
- FlowDocumentScrollViewer.cs
- HttpRuntimeSection.cs
- TextTreeUndo.cs
- indexingfiltermarshaler.cs
- XsltArgumentList.cs
- ActiveXSite.cs
- PointLight.cs
- MailFileEditor.cs
- InputProcessorProfiles.cs
- IsolatedStoragePermission.cs
- SettingsProviderCollection.cs
- ADRole.cs
- ScalarType.cs
- Graphics.cs
- ItemChangedEventArgs.cs
- CodeAccessPermission.cs
- System.Data.OracleClient_BID.cs
- DBBindings.cs
- ClientSettings.cs
- PackWebResponse.cs
- XamlUtilities.cs
- AdornedElementPlaceholder.cs
- WorkflowTraceTransfer.cs
- Trustee.cs
- Stacktrace.cs
- XPathConvert.cs
- TransformerTypeCollection.cs
- StyleCollectionEditor.cs
- DbExpressionBuilder.cs
- DBAsyncResult.cs
- WindowsSpinner.cs
- Adorner.cs
- WebPartDescriptionCollection.cs
- BitStack.cs
- AsyncStreamReader.cs
- TabPageDesigner.cs
- QilGeneratorEnv.cs
- XmlSchemaSimpleTypeList.cs
- EntityType.cs
- SQLDateTime.cs
- MemberProjectedSlot.cs
- XmlSchemaIdentityConstraint.cs
- HandoffBehavior.cs
- SelectingProviderEventArgs.cs
- TextBoxView.cs
- SqlAliaser.cs
- SessionStateUtil.cs
- ValidatedControlConverter.cs
- IgnorePropertiesAttribute.cs
- HtmlControl.cs
- SpoolingTask.cs
- DataServiceQuery.cs