Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Controls / Primitives / PopupRoot.cs / 1 / PopupRoot.cs
using System; using System.Collections; using System.Windows.Threading; using System.Windows.Data; using System.Windows.Documents; using System.Windows.Input; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Markup; using System.Runtime.InteropServices; using MS.Utility; using MS.Win32; using MS.Internal; using MS.Internal.Data; using MS.Internal.KnownBoxes; namespace System.Windows.Controls.Primitives { ////// The root element inside a popup. /// internal sealed class PopupRoot : FrameworkElement { #region Constructors static PopupRoot() { SnapsToDevicePixelsProperty.OverrideMetadata(typeof(PopupRoot), new FrameworkPropertyMetadata(BooleanBoxes.TrueBox)); } ////// Default constructor /// internal PopupRoot() : base() { Initialize(); } private void Initialize() { // Popup root has a decorator used for // applying the transforms _transformDecorator = new Decorator(); AddVisualChild(_transformDecorator); // Clip so animations do not extend beyond its bounds _transformDecorator.ClipToBounds = true; // Under the transfrom decorator is an Adorner // decorator that handles rendering adorners // and the animated popup translations _adornerDecorator = new NonLogicalAdornerDecorator(); _transformDecorator.Child = _adornerDecorator; } #endregion #region Visual Children ////// Returns the Visual children count. /// protected override int VisualChildrenCount { get { return 1; } } ////// Returns the child at the specified index. /// protected override Visual GetVisualChild(int index) { if (index != 0) { throw new ArgumentOutOfRangeException("index", index, SR.Get(SRID.Visual_ArgumentOutOfRange)); } return _transformDecorator; } #endregion #region Automation ////// Creates AutomationPeer ( protected override System.Windows.Automation.Peers.AutomationPeer OnCreateAutomationPeer() { return new System.Windows.Automation.Peers.PopupRootAutomationPeer(this); } #endregion Automation #region Properties internal UIElement Child { get { return _adornerDecorator.Child; } set { _adornerDecorator.Child = value; } } internal Vector AnimationOffset { get { TranslateTransform transform = _adornerDecorator.RenderTransform as TranslateTransform; if (transform != null) { return new Vector(transform.X, transform.Y); } return new Vector(); } } ///) /// /// This is the transform matrix that the popup content "inherits" from the placement target. /// internal Transform Transform { set { _transformDecorator.LayoutTransform = value; } } #endregion #region Layout ////// Invoked when remeasuring the control is required. /// /// The control cannot return a size larger than the constraint. ///The size of the child restricted to 75% of screen protected override Size MeasureOverride(Size constraint) { _transformDecorator.Measure(new Size(Double.PositiveInfinity, Double.PositiveInfinity)); Size desiredSize = _transformDecorator.DesiredSize; // Ask popup to restrict our size Popup popup = Parent as Popup; if (popup != null) { Size restrictedSize = popup.RestrictSize(desiredSize); if (Math.Abs(restrictedSize.Width - desiredSize.Width) > Popup.Tolerance || Math.Abs(restrictedSize.Height - desiredSize.Height) > Popup.Tolerance) { desiredSize = restrictedSize; _transformDecorator.Measure(desiredSize); } } return desiredSize; } ////// ArrangeOverride allows for the customization of the positioning of children. /// /// The final size that element should use to arrange itself and its children. protected override Size ArrangeOverride(Size arrangeSize) { _transformDecorator.Arrange(new Rect(arrangeSize)); return arrangeSize; } ////// Sets up bindings between (Min/Max)Width/Height properties on Popup and PopupRoot. /// /// The parent Popup. internal void SetupLayoutBindings(Popup popup) { Binding binding = new Binding("Width"); binding.Mode = BindingMode.OneWay; binding.Source = popup; _adornerDecorator.SetBinding(WidthProperty, binding); binding = new Binding("Height"); binding.Mode = BindingMode.OneWay; binding.Source = popup; _adornerDecorator.SetBinding(HeightProperty, binding); binding = new Binding("MinWidth"); binding.Mode = BindingMode.OneWay; binding.Source = popup; _adornerDecorator.SetBinding(MinWidthProperty, binding); binding = new Binding("MinHeight"); binding.Mode = BindingMode.OneWay; binding.Source = popup; _adornerDecorator.SetBinding(MinHeightProperty, binding); binding = new Binding("MaxWidth"); binding.Mode = BindingMode.OneWay; binding.Source = popup; _adornerDecorator.SetBinding(MaxWidthProperty, binding); binding = new Binding("MaxHeight"); binding.Mode = BindingMode.OneWay; binding.Source = popup; _adornerDecorator.SetBinding(MaxHeightProperty, binding); } // Popup is transparent, change opacity of root internal void SetupFadeAnimation(Duration duration, bool visible) { DoubleAnimation anim = new DoubleAnimation(visible ? 0.0 : 1.0, visible ? 1.0 : 0.0, duration, FillBehavior.HoldEnd); BeginAnimation(PopupRoot.OpacityProperty, anim); } // Popup is transparent, we can leave popup size alone // and animate the translation of the popup internal void SetupTranslateAnimations(PopupAnimation animationType, Duration duration, bool animateFromRight, bool animateFromBottom) { UIElement child = Child; if (child == null) return; TranslateTransform transform = _adornerDecorator.RenderTransform as TranslateTransform; if (transform == null) { transform = new TranslateTransform(); _adornerDecorator.RenderTransform = transform; } if (animationType == PopupAnimation.Scroll) { // If the flow direction of the child is different than ours, animate in opposite direction FlowDirection childFlowDirection = (FlowDirection)child.GetValue(FlowDirectionProperty); FlowDirection thisFlowDirection = FlowDirection; if (childFlowDirection != thisFlowDirection) { animateFromRight = !animateFromRight; } double width = _adornerDecorator.RenderSize.Width; DoubleAnimation xAnim = new DoubleAnimation(animateFromRight ? width : -width, 0.0, duration, FillBehavior.Stop); transform.BeginAnimation(TranslateTransform.XProperty, xAnim); } double height = _adornerDecorator.RenderSize.Height; DoubleAnimation yAnim = new DoubleAnimation(animateFromBottom ? height : -height, 0.0, duration, FillBehavior.Stop); transform.BeginAnimation(TranslateTransform.YProperty, yAnim); } // Clear animations on this and _adorner internal void StopAnimations() { BeginAnimation(PopupRoot.OpacityProperty, null); TranslateTransform transform = _adornerDecorator.RenderTransform as TranslateTransform; if (transform != null) { transform.BeginAnimation(TranslateTransform.XProperty, null); transform.BeginAnimation(TranslateTransform.YProperty, null); } } #endregion #region Tree Overrides internal override bool IgnoreModelParentBuildRoute(RoutedEventArgs e) { // We do not want QueryCursor event to bubble up past this node if(e is QueryCursorEventArgs) { return true; } // Defer to the child to determine if we should route events up the logical tree. FrameworkElement child = Child as FrameworkElement; if(child != null) { return child.IgnoreModelParentBuildRoute(e); } else { return base.IgnoreModelParentBuildRoute(e); } } #endregion #region Data private Decorator _transformDecorator; // The decorator used to apply animations private AdornerDecorator _adornerDecorator; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
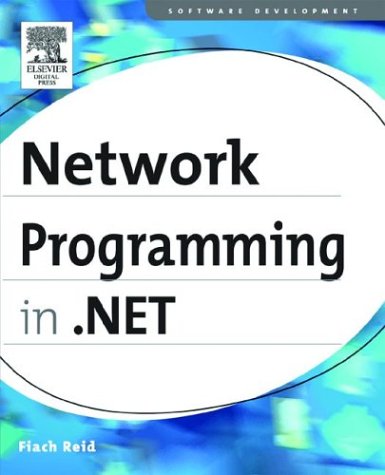
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridLength.cs
- WebPartDeleteVerb.cs
- VersionPair.cs
- sqlstateclientmanager.cs
- CollectionView.cs
- ToolStripDropDown.cs
- GenerateHelper.cs
- TextParagraph.cs
- SystemWebCachingSectionGroup.cs
- SurrogateEncoder.cs
- MenuItemBindingCollection.cs
- XmlSchemaSimpleTypeRestriction.cs
- HttpHandlerActionCollection.cs
- Msec.cs
- Button.cs
- GridPattern.cs
- VisualCollection.cs
- DesignSurfaceManager.cs
- ExceptionAggregator.cs
- TTSEngineProxy.cs
- DataGridViewRowCancelEventArgs.cs
- SecurityManager.cs
- SynchronizationLockException.cs
- XPathNode.cs
- ConnectionManagementElementCollection.cs
- Adorner.cs
- ToolboxService.cs
- SqlMethodAttribute.cs
- cookiecontainer.cs
- SqlNotificationRequest.cs
- SmiMetaDataProperty.cs
- XmlCharCheckingWriter.cs
- SerialPinChanges.cs
- ScrollProperties.cs
- Opcode.cs
- SiteMapPath.cs
- HtmlInputFile.cs
- _SslStream.cs
- BamlBinaryWriter.cs
- UInt16Storage.cs
- LineGeometry.cs
- ISFClipboardData.cs
- InProcStateClientManager.cs
- TextTreeRootNode.cs
- altserialization.cs
- TextServicesHost.cs
- DataControlFieldCollection.cs
- TreeIterators.cs
- OleDbErrorCollection.cs
- Light.cs
- DataTable.cs
- DrawTreeNodeEventArgs.cs
- HeaderedItemsControl.cs
- ScriptIgnoreAttribute.cs
- TabPage.cs
- XPathBuilder.cs
- MappingItemCollection.cs
- PermissionSetEnumerator.cs
- FileLogRecordStream.cs
- PolyLineSegment.cs
- StylusLogic.cs
- CookieHandler.cs
- SQLConvert.cs
- AssemblyContextControlItem.cs
- ProfileEventArgs.cs
- HttpRuntimeSection.cs
- WebPartDisplayModeCollection.cs
- BufferedStream2.cs
- SafeRightsManagementQueryHandle.cs
- ColumnMapTranslator.cs
- FileDialogCustomPlacesCollection.cs
- ComboBoxRenderer.cs
- RequestQueryParser.cs
- FixedSOMLineCollection.cs
- InstanceCollisionException.cs
- ConcurrentStack.cs
- SymbolDocumentGenerator.cs
- SystemWebExtensionsSectionGroup.cs
- XmlDocument.cs
- _BufferOffsetSize.cs
- ReaderContextStackData.cs
- COM2PropertyDescriptor.cs
- CultureTableRecord.cs
- Attribute.cs
- SystemColorTracker.cs
- XomlCompilerResults.cs
- BooleanExpr.cs
- CacheDependency.cs
- SingleObjectCollection.cs
- SmiTypedGetterSetter.cs
- Page.cs
- String.cs
- CodeRemoveEventStatement.cs
- RightsManagementResourceHelper.cs
- VideoDrawing.cs
- ExpressionWriter.cs
- CoreSwitches.cs
- NameValueConfigurationCollection.cs
- webbrowsersite.cs
- DocumentScope.cs