Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / Host / DesignSurfaceManager.cs / 1 / DesignSurfaceManager.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; ////// /// A service container that supports "fixed" services. Fixed /// services cannot be removed. /// [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] public class DesignSurfaceManager : IServiceProvider, IDisposable { private IServiceProvider _parentProvider; private ServiceContainer _serviceContainer; private ActiveDesignSurfaceChangedEventHandler _activeDesignSurfaceChanged; private DesignSurfaceEventHandler _designSurfaceCreated; private DesignSurfaceEventHandler _designSurfaceDisposed; private EventHandler _selectionChanged; ////// /// Creates a new designer application. /// public DesignSurfaceManager() : this(null) { } ////// /// Creates a new designer application and provides a /// parent service provider. /// public DesignSurfaceManager(IServiceProvider parentProvider) { _parentProvider = parentProvider; ServiceCreatorCallback callback = new ServiceCreatorCallback(OnCreateService); ServiceContainer.AddService(typeof(IDesignerEventService), callback); } ////// /// This property should be set by the designer's user interface /// whenever a designer becomes the active window. The default /// implementation of this property works with the default /// implementation of IDesignerEventService to notify interested /// parties that a new designer is now active. If you provide /// your own implementation of IDesignerEventService you should /// override this property to notify your service appropriately. /// public virtual DesignSurface ActiveDesignSurface { get { IDesignerEventService eventService = EventService; if (eventService != null) { IDesignerHost activeHost = eventService.ActiveDesigner; if (activeHost != null) { return activeHost.GetService(typeof(DesignSurface)) as DesignSurface; } } return null; } set { // If we are providing IDesignerEventService, then we are responsible for // notifying it of new designers coming into place. If we aren't // the ones providing the event service, then whoever is providing // it will be responsible for updating it when new designers // are created. // DesignerEventService eventService = EventService as DesignerEventService; if (eventService != null) { eventService.OnActivateDesigner(value); } } } ////// /// A collection of design surfaces. This is offered /// for convience, and simply maps to IDesignerEventService. /// public DesignSurfaceCollection DesignSurfaces { get { IDesignerEventService eventService = EventService; if (eventService != null) { return new DesignSurfaceCollection(eventService.Designers); } return new DesignSurfaceCollection(null); } } ////// We access this a lot. /// private IDesignerEventService EventService { get { return GetService(typeof(IDesignerEventService)) as IDesignerEventService; } } ////// /// Provides access to the designer application's /// ServiceContainer. This property allows /// inheritors to add their own services. /// protected ServiceContainer ServiceContainer { get { if (_serviceContainer == null) { _serviceContainer = new ServiceContainer(_parentProvider); } return _serviceContainer; } } ////// /// This event is raised when a new design surface gains /// activation. This is mapped through IDesignerEventService. /// public event ActiveDesignSurfaceChangedEventHandler ActiveDesignSurfaceChanged { add { if (_activeDesignSurfaceChanged == null) { IDesignerEventService eventService = EventService; if (eventService != null) { eventService.ActiveDesignerChanged += new ActiveDesignerEventHandler(OnActiveDesignerChanged); } } _activeDesignSurfaceChanged += value; } remove { _activeDesignSurfaceChanged -= value; if (_activeDesignSurfaceChanged == null) { IDesignerEventService eventService = EventService; if (eventService != null) { eventService.ActiveDesignerChanged -= new ActiveDesignerEventHandler(OnActiveDesignerChanged); } } } } ////// /// This event is raised when a new design surface is /// created. This is mapped through IDesignerEventService. /// public event DesignSurfaceEventHandler DesignSurfaceCreated { add { if (_designSurfaceCreated == null) { IDesignerEventService eventService = EventService; if (eventService != null) { eventService.DesignerCreated += new DesignerEventHandler(OnDesignerCreated); } } _designSurfaceCreated += value; } remove { _designSurfaceCreated -= value; if (_designSurfaceCreated == null) { IDesignerEventService eventService = EventService; if (eventService != null) { eventService.DesignerCreated -= new DesignerEventHandler(OnDesignerCreated); } } } } ////// /// This event is raised when a design surface is disposed. /// This is mapped through IDesignerEventService. /// public event DesignSurfaceEventHandler DesignSurfaceDisposed { add { if (_designSurfaceDisposed == null) { IDesignerEventService eventService = EventService; if (eventService != null) { eventService.DesignerDisposed += new DesignerEventHandler(OnDesignerDisposed); } } _designSurfaceDisposed += value; } remove { _designSurfaceDisposed -= value; if (_designSurfaceDisposed == null) { IDesignerEventService eventService = EventService; if (eventService != null) { eventService.DesignerDisposed -= new DesignerEventHandler(OnDesignerDisposed); } } } } ////// /// This event is raised when the active designer's /// selection of component set changes. This is mapped /// through IDesignerEventService. /// public event EventHandler SelectionChanged { add { if (_selectionChanged == null) { IDesignerEventService eventService = EventService; if (eventService != null) { eventService.SelectionChanged += new EventHandler(OnSelectionChanged); } } _selectionChanged += value; } remove { _selectionChanged -= value; if (_selectionChanged == null) { IDesignerEventService eventService = EventService; if (eventService != null) { eventService.SelectionChanged -= new EventHandler(OnSelectionChanged); } } } } ////// /// Public method to create a design surface. /// public DesignSurface CreateDesignSurface() { DesignSurface surface = CreateDesignSurfaceCore(this); // If we are providing IDesignerEventService, then we are responsible for // notifying it of new designers coming into place. If we aren't // the ones providing the event service, then whoever is providing // it will be responsible for updating it when new designers // are created. // DesignerEventService eventService = GetService(typeof(IDesignerEventService)) as DesignerEventService; if (eventService != null) { eventService.OnCreateDesigner(surface); } return surface; } ////// /// Public method to create a design surface. This method /// takes an additional service provider. This service /// provider will be combined with the service provider /// already contained within DesignSurfaceManager. Service /// requests will go to this provider first, and then bubble /// up to the service provider owned by DesignSurfaceManager. /// This allows for services to be tailored for each design /// surface. /// public DesignSurface CreateDesignSurface(IServiceProvider parentProvider) { if (parentProvider == null) { throw new ArgumentNullException("parentProvider"); } IServiceProvider mergedProvider = new MergedServiceProvider(parentProvider, this); DesignSurface surface = CreateDesignSurfaceCore(mergedProvider); // If we are providing IDesignerEventService, then we are responsible for // notifying it of new designers coming into place. If we aren't // the ones providing the event service, then whoever is providing // it will be responsible for updating it when new designers // are created. // DesignerEventService eventService = GetService(typeof(IDesignerEventService)) as DesignerEventService; if (eventService != null) { eventService.OnCreateDesigner(surface); } return surface; } ////// /// Creates an instance of a design surface. This can be /// overridden to provide a derived version of DesignSurface. /// protected virtual DesignSurface CreateDesignSurfaceCore(IServiceProvider parentProvider) { return new DesignSurface(parentProvider); } ////// /// Disposes the designer application. /// public void Dispose() { Dispose(true); } ////// /// Protected override of Dispose that allows for cleanup. /// protected virtual void Dispose(bool disposing) { if (disposing && _serviceContainer != null) { _serviceContainer.Dispose(); _serviceContainer = null; } } ////// /// Retrieves a service in this design surface's service /// container. /// public object GetService(Type serviceType) { if (_serviceContainer != null) { return _serviceContainer.GetService(serviceType); } return null; } ////// Private method that demand-creates services we offer. /// /// /// The service container requesting the service. /// /// /// The type of service being requested. /// ////// A new instance of the service. It is an error to call this with /// a service type it doesn't know how to create /// private object OnCreateService(IServiceContainer container, Type serviceType) { if (serviceType == typeof(IDesignerEventService)) { return new DesignerEventService(); } Debug.Fail("Demand created service not supported: " + serviceType.Name); return null; } ////// Handles the IDesignerEventService event and relays it to /// DesignSurfaceManager's similar event. /// private void OnActiveDesignerChanged(object sender, ActiveDesignerEventArgs e) { Debug.Assert(_activeDesignSurfaceChanged != null, "Should have detached this event handler."); if (_activeDesignSurfaceChanged != null) { DesignSurface newSurface = null; DesignSurface oldSurface = null; if (e.OldDesigner != null) { oldSurface = e.OldDesigner.GetService(typeof(DesignSurface)) as DesignSurface; } if (e.NewDesigner != null) { newSurface = e.NewDesigner.GetService(typeof(DesignSurface)) as DesignSurface; } _activeDesignSurfaceChanged(this, new ActiveDesignSurfaceChangedEventArgs(oldSurface, newSurface)); } } ////// Handles the IDesignerEventService event and relays it to /// DesignSurfaceManager's similar event. /// private void OnDesignerCreated(object sender, DesignerEventArgs e) { Debug.Assert(_designSurfaceCreated != null, "Should have detached this event handler."); if (_designSurfaceCreated != null) { DesignSurface surface = e.Designer.GetService(typeof(DesignSurface)) as DesignSurface; if (surface != null) { _designSurfaceCreated(this, new DesignSurfaceEventArgs(surface)); } } } ////// Handles the IDesignerEventService event and relays it to /// DesignSurfaceManager's similar event. /// private void OnDesignerDisposed(object sender, DesignerEventArgs e) { Debug.Assert(_designSurfaceDisposed != null, "Should have detached this event handler."); if (_designSurfaceDisposed != null) { DesignSurface surface = e.Designer.GetService(typeof(DesignSurface)) as DesignSurface; if (surface != null) { _designSurfaceDisposed(this, new DesignSurfaceEventArgs(surface)); } } } ////// Handles the IDesignerEventService event and relays it to /// DesignSurfaceManager's similar event. /// private void OnSelectionChanged(object sender, EventArgs e) { Debug.Assert(_selectionChanged != null, "Should have detached this event handler."); if (_selectionChanged != null) { _selectionChanged(this, e); } } ////// Simple service provider that merges two providers together. /// private sealed class MergedServiceProvider : IServiceProvider { private IServiceProvider _primaryProvider; private IServiceProvider _secondaryProvider; internal MergedServiceProvider(IServiceProvider primaryProvider, IServiceProvider secondaryProvider) { _primaryProvider = primaryProvider; _secondaryProvider = secondaryProvider; } object IServiceProvider.GetService(Type serviceType) { if (serviceType == null) { throw new ArgumentNullException("serviceType"); } object service = _primaryProvider.GetService(serviceType); if (service == null) { service = _secondaryProvider.GetService(serviceType); } return service; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
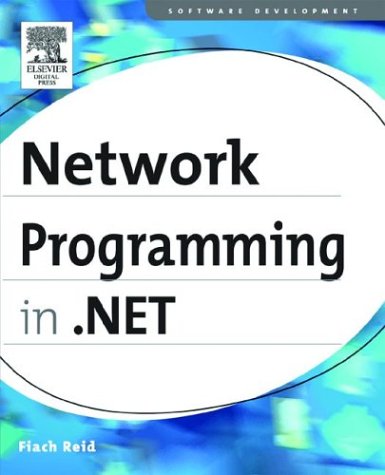
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DebuggerAttributes.cs
- TextTrailingWordEllipsis.cs
- ConfigXmlAttribute.cs
- HandleTable.cs
- WorkerRequest.cs
- XslTransform.cs
- SizeAnimation.cs
- XmlCharacterData.cs
- AttachInfo.cs
- ComponentResourceKeyConverter.cs
- AsyncPostBackTrigger.cs
- X509Certificate.cs
- CompiledIdentityConstraint.cs
- XmlDownloadManager.cs
- XPathDocumentBuilder.cs
- LineVisual.cs
- DataSvcMapFile.cs
- CriticalHandle.cs
- ButtonBaseAutomationPeer.cs
- WebPartConnectVerb.cs
- RoutedEventValueSerializer.cs
- CodeMemberProperty.cs
- ImageSource.cs
- LinearKeyFrames.cs
- LayoutEvent.cs
- SecurityAlgorithmSuite.cs
- SamlAuthorizationDecisionClaimResource.cs
- oledbconnectionstring.cs
- RtType.cs
- OleDbParameter.cs
- SettingsBase.cs
- DataMemberListEditor.cs
- CharacterMetrics.cs
- Matrix3DStack.cs
- TrustLevel.cs
- SecUtil.cs
- _Rfc2616CacheValidators.cs
- XmlText.cs
- DataBinding.cs
- HttpSessionStateWrapper.cs
- FindCriteriaCD1.cs
- MessageQueuePermission.cs
- EntityDataSourceEntityTypeFilterItem.cs
- ListViewItem.cs
- MenuItem.cs
- UserControlAutomationPeer.cs
- InputLanguageManager.cs
- BrowserCapabilitiesFactoryBase.cs
- UserPreferenceChangedEventArgs.cs
- SettingsPropertyValue.cs
- CodeDOMProvider.cs
- BitConverter.cs
- DetailsViewDeleteEventArgs.cs
- ClientProxyGenerator.cs
- FileUtil.cs
- AssertSection.cs
- RoutedEventArgs.cs
- DocumentOutline.cs
- GridView.cs
- XmlSchemaSimpleContentExtension.cs
- SchemaNamespaceManager.cs
- RecommendedAsConfigurableAttribute.cs
- SmtpDigestAuthenticationModule.cs
- ToolboxItemAttribute.cs
- ResourceSet.cs
- DataGridViewCellLinkedList.cs
- DelegateBodyWriter.cs
- Stack.cs
- ImageListUtils.cs
- XmlReaderSettings.cs
- SQLGuidStorage.cs
- Size3D.cs
- SessionEndingEventArgs.cs
- UnsafeNativeMethods.cs
- SQLBinary.cs
- ObjectDisposedException.cs
- OutputWindow.cs
- Inline.cs
- UserPreferenceChangingEventArgs.cs
- StackBuilderSink.cs
- StructureChangedEventArgs.cs
- TextSchema.cs
- RuntimeConfigurationRecord.cs
- GenerateScriptTypeAttribute.cs
- SortFieldComparer.cs
- NavigationProgressEventArgs.cs
- DataGridViewImageCell.cs
- ProxyHelper.cs
- SubMenuStyle.cs
- MultiSelector.cs
- TypeReference.cs
- Transform3D.cs
- MobileControlPersister.cs
- TextParentUndoUnit.cs
- TypedRowHandler.cs
- SqlProviderServices.cs
- OfTypeExpression.cs
- PackWebRequestFactory.cs
- LogFlushAsyncResult.cs
- WarningException.cs