Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / FamilyTypefaceCollection.cs / 1 / FamilyTypefaceCollection.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2002 // // File: FamilyTypefaceCollection.cs // // Contents: FamilyTypefaceCollection // // Created: 2-5-05 Niklas Borson (niklasb) // //----------------------------------------------------------------------- using System; using System.Globalization; using SC=System.Collections; using System.Collections.Generic; using MS.Internal.FontFace; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// List of FamilyTypeface objects in a FontFamily, in lookup order. /// public sealed class FamilyTypefaceCollection : IList, SC.IList { private const int InitialCapacity = 2; private ICollection _innerList; private FamilyTypeface[] _items; private int _count; /// /// Constructs a read-write list of FamilyTypeface objects. /// internal FamilyTypefaceCollection() { _innerList = null; _items = null; _count = 0; } ////// Constructes a read-only list that wraps an ICollection. /// internal FamilyTypefaceCollection(ICollectioninnerList) { _innerList = innerList; _items = null; _count = innerList.Count; } #region IEnumerable members /// /// Returns an enumerator for iterating through the list. /// public IEnumeratorGetEnumerator() { return new Enumerator(this); } SC.IEnumerator SC.IEnumerable.GetEnumerator() { return new Enumerator(this); } #endregion #region ICollection methods /// /// Adds a FamilyTypeface to the font family. /// public void Add(FamilyTypeface item) { InsertItem(_count, item); } ////// Removes all FamilyTypeface objects from the FontFamily. /// public void Clear() { ClearItems(); } ////// Determines whether the FontFamily contains the specified FamilyTypeface. /// public bool Contains(FamilyTypeface item) { return FindItem(item) >= 0; } ////// Copies the contents of the list to the specified array. /// public void CopyTo(FamilyTypeface[] array, int index) { CopyItems(array, index); } void SC.ICollection.CopyTo(Array array, int index) { CopyItems(array, index); } bool SC.ICollection.IsSynchronized { get { return false; } } object SC.ICollection.SyncRoot { get { return this; } } ////// Removes the specified FamilyTypeface. /// public bool Remove(FamilyTypeface item) { VerifyChangeable(); int i = FindItem(item); if (i >= 0) { RemoveAt(i); return true; } return false; } ////// Gets the number of items in the list. /// public int Count { get { return _count; } } ////// Gets a value indicating whether the FamilyTypeface list can be changed. /// public bool IsReadOnly { get { return _innerList != null; } } #endregion #region IList members ////// Gets the index of the specified FamilyTypeface. /// public int IndexOf(FamilyTypeface item) { return FindItem(item); } ////// Inserts a FamilyTypeface into the list. /// public void Insert(int index, FamilyTypeface item) { InsertItem(index, item); } ////// Removes the FamilyTypeface at the specified index. /// public void RemoveAt(int index) { RemoveItem(index); } ////// Gets or sets the FamilyTypeface at the specified index. /// public FamilyTypeface this[int index] { get { return GetItem(index); } set { SetItem(index, value); } } int SC.IList.Add(object value) { return InsertItem(_count, ConvertValue(value)); } bool SC.IList.Contains(object value) { return FindItem(value as FamilyTypeface) >= 0; } int SC.IList.IndexOf(object value) { return FindItem(value as FamilyTypeface); } void SC.IList.Insert(int index, object item) { InsertItem(index, ConvertValue(item)); } void SC.IList.Remove(object value) { VerifyChangeable(); int i = FindItem(value as FamilyTypeface); if (i >= 0) RemoveItem(i); } bool SC.IList.IsFixedSize { get { return IsReadOnly; } } object SC.IList.this[int index] { get { return GetItem(index); } set { SetItem(index, ConvertValue(value)); } } #endregion #region Internal implementation private int InsertItem(int index, FamilyTypeface item) { if (item == null) throw new ArgumentNullException("item"); VerifyChangeable(); // Validate the index. if (index < 0 || index > Count) throw new ArgumentOutOfRangeException("index"); // We can't have two items with same style, weight, stretch. if (FindItem(item) >= 0) throw new ArgumentException(SR.Get(SRID.CompositeFont_DuplicateTypeface)); // Make room for the new item. if (_items == null) { _items = new FamilyTypeface[InitialCapacity]; } else if (_count == _items.Length) { FamilyTypeface[] items = new FamilyTypeface[_count * 2]; for (int i = 0; i < index; ++i) items[i] = _items[i]; for (int i = index; i < _count; ++i) items[i + 1] = _items[i]; _items = items; } else if (index < _count) { for (int i = _count - 1; i >= index; --i) _items[i + 1] = _items[i]; } // Add the item. _items[index] = item; _count++; return index; } private void InitializeItemsFromInnerList() { if (_innerList != null && _items == null) { // Create the array. FamilyTypeface[] items = new FamilyTypeface[_count]; // Create a FamilyTypeface for each Typeface in the inner list. int i = 0; foreach (Typeface face in _innerList) { items[i++] = new FamilyTypeface(face); } // For thread-safety, set _items to the fully-initialized array at the end. _items = items; } } private FamilyTypeface GetItem(int index) { RangeCheck(index); InitializeItemsFromInnerList(); return _items[index]; } private void SetItem(int index, FamilyTypeface item) { if (item == null) throw new ArgumentNullException("item"); VerifyChangeable(); RangeCheck(index); _items[index] = item; } private void ClearItems() { VerifyChangeable(); _count = 0; _items = null; } private void RemoveItem(int index) { VerifyChangeable(); RangeCheck(index); _count--; for (int i = index; i < _count; ++i) { _items[i] = _items[i + 1]; } _items[_count] = null; } private int FindItem(FamilyTypeface item) { InitializeItemsFromInnerList(); if (_count != 0 && item != null) { for (int i = 0; i < _count; ++i) { if (GetItem(i).Equals(item)) return i; } } return -1; } private void RangeCheck(int index) { if (index < 0 || index >= _count) throw new ArgumentOutOfRangeException("index"); } private void VerifyChangeable() { if (_innerList != null) throw new NotSupportedException(SR.Get(SRID.General_ObjectIsReadOnly)); } private FamilyTypeface ConvertValue(object obj) { if (obj == null) throw new ArgumentNullException(); FamilyTypeface familyTypeface = obj as FamilyTypeface; if (familyTypeface == null) throw new ArgumentException(SR.Get(SRID.CannotConvertType, obj.GetType(), typeof(FamilyTypeface))); return familyTypeface; } private void CopyItems(Array array, int index) { if (array == null) throw new ArgumentNullException("array"); if (array.Rank != 1) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_ArrayCannotBeMultidimensional)); Type elementType = array.GetType().GetElementType(); if (!elementType.IsAssignableFrom(typeof(FamilyTypeface))) throw new ArgumentException(SR.Get(SRID.CannotConvertType, typeof(FamilyTypeface[]), elementType)); if (index >= array.Length) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_IndexGreaterThanOrEqualToArrayLength, "index", "array")); if (_count > array.Length - index) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, index, "array")); if (_count != 0) { InitializeItemsFromInnerList(); Array.Copy(_items, 0, array, index, _count); } } private class Enumerator : IEnumerator, SC.IEnumerator { FamilyTypefaceCollection _list; int _index; FamilyTypeface _current; internal Enumerator(FamilyTypefaceCollection list) { _list = list; _index = -1; _current = null; } public bool MoveNext() { int count = _list.Count; if (_index < count) { _index++; if (_index < count) { _current = _list[_index]; return true; } } _current = null; return false; } void SC.IEnumerator.Reset() { _index = -1; } public FamilyTypeface Current { get { return _current; } } object SC.IEnumerator.Current { get { // If there is no current item a non-generic IEnumerator should throw an exception, // but a generic IEnumerator is not required to. if (_current == null) throw new InvalidOperationException(SR.Get(SRID.Enumerator_VerifyContext)); return _current; } } public void Dispose() { } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2002 // // File: FamilyTypefaceCollection.cs // // Contents: FamilyTypefaceCollection // // Created: 2-5-05 Niklas Borson (niklasb) // //----------------------------------------------------------------------- using System; using System.Globalization; using SC=System.Collections; using System.Collections.Generic; using MS.Internal.FontFace; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { /// /// List of FamilyTypeface objects in a FontFamily, in lookup order. /// public sealed class FamilyTypefaceCollection : IList, SC.IList { private const int InitialCapacity = 2; private ICollection _innerList; private FamilyTypeface[] _items; private int _count; /// /// Constructs a read-write list of FamilyTypeface objects. /// internal FamilyTypefaceCollection() { _innerList = null; _items = null; _count = 0; } ////// Constructes a read-only list that wraps an ICollection. /// internal FamilyTypefaceCollection(ICollectioninnerList) { _innerList = innerList; _items = null; _count = innerList.Count; } #region IEnumerable members /// /// Returns an enumerator for iterating through the list. /// public IEnumeratorGetEnumerator() { return new Enumerator(this); } SC.IEnumerator SC.IEnumerable.GetEnumerator() { return new Enumerator(this); } #endregion #region ICollection methods /// /// Adds a FamilyTypeface to the font family. /// public void Add(FamilyTypeface item) { InsertItem(_count, item); } ////// Removes all FamilyTypeface objects from the FontFamily. /// public void Clear() { ClearItems(); } ////// Determines whether the FontFamily contains the specified FamilyTypeface. /// public bool Contains(FamilyTypeface item) { return FindItem(item) >= 0; } ////// Copies the contents of the list to the specified array. /// public void CopyTo(FamilyTypeface[] array, int index) { CopyItems(array, index); } void SC.ICollection.CopyTo(Array array, int index) { CopyItems(array, index); } bool SC.ICollection.IsSynchronized { get { return false; } } object SC.ICollection.SyncRoot { get { return this; } } ////// Removes the specified FamilyTypeface. /// public bool Remove(FamilyTypeface item) { VerifyChangeable(); int i = FindItem(item); if (i >= 0) { RemoveAt(i); return true; } return false; } ////// Gets the number of items in the list. /// public int Count { get { return _count; } } ////// Gets a value indicating whether the FamilyTypeface list can be changed. /// public bool IsReadOnly { get { return _innerList != null; } } #endregion #region IList members ////// Gets the index of the specified FamilyTypeface. /// public int IndexOf(FamilyTypeface item) { return FindItem(item); } ////// Inserts a FamilyTypeface into the list. /// public void Insert(int index, FamilyTypeface item) { InsertItem(index, item); } ////// Removes the FamilyTypeface at the specified index. /// public void RemoveAt(int index) { RemoveItem(index); } ////// Gets or sets the FamilyTypeface at the specified index. /// public FamilyTypeface this[int index] { get { return GetItem(index); } set { SetItem(index, value); } } int SC.IList.Add(object value) { return InsertItem(_count, ConvertValue(value)); } bool SC.IList.Contains(object value) { return FindItem(value as FamilyTypeface) >= 0; } int SC.IList.IndexOf(object value) { return FindItem(value as FamilyTypeface); } void SC.IList.Insert(int index, object item) { InsertItem(index, ConvertValue(item)); } void SC.IList.Remove(object value) { VerifyChangeable(); int i = FindItem(value as FamilyTypeface); if (i >= 0) RemoveItem(i); } bool SC.IList.IsFixedSize { get { return IsReadOnly; } } object SC.IList.this[int index] { get { return GetItem(index); } set { SetItem(index, ConvertValue(value)); } } #endregion #region Internal implementation private int InsertItem(int index, FamilyTypeface item) { if (item == null) throw new ArgumentNullException("item"); VerifyChangeable(); // Validate the index. if (index < 0 || index > Count) throw new ArgumentOutOfRangeException("index"); // We can't have two items with same style, weight, stretch. if (FindItem(item) >= 0) throw new ArgumentException(SR.Get(SRID.CompositeFont_DuplicateTypeface)); // Make room for the new item. if (_items == null) { _items = new FamilyTypeface[InitialCapacity]; } else if (_count == _items.Length) { FamilyTypeface[] items = new FamilyTypeface[_count * 2]; for (int i = 0; i < index; ++i) items[i] = _items[i]; for (int i = index; i < _count; ++i) items[i + 1] = _items[i]; _items = items; } else if (index < _count) { for (int i = _count - 1; i >= index; --i) _items[i + 1] = _items[i]; } // Add the item. _items[index] = item; _count++; return index; } private void InitializeItemsFromInnerList() { if (_innerList != null && _items == null) { // Create the array. FamilyTypeface[] items = new FamilyTypeface[_count]; // Create a FamilyTypeface for each Typeface in the inner list. int i = 0; foreach (Typeface face in _innerList) { items[i++] = new FamilyTypeface(face); } // For thread-safety, set _items to the fully-initialized array at the end. _items = items; } } private FamilyTypeface GetItem(int index) { RangeCheck(index); InitializeItemsFromInnerList(); return _items[index]; } private void SetItem(int index, FamilyTypeface item) { if (item == null) throw new ArgumentNullException("item"); VerifyChangeable(); RangeCheck(index); _items[index] = item; } private void ClearItems() { VerifyChangeable(); _count = 0; _items = null; } private void RemoveItem(int index) { VerifyChangeable(); RangeCheck(index); _count--; for (int i = index; i < _count; ++i) { _items[i] = _items[i + 1]; } _items[_count] = null; } private int FindItem(FamilyTypeface item) { InitializeItemsFromInnerList(); if (_count != 0 && item != null) { for (int i = 0; i < _count; ++i) { if (GetItem(i).Equals(item)) return i; } } return -1; } private void RangeCheck(int index) { if (index < 0 || index >= _count) throw new ArgumentOutOfRangeException("index"); } private void VerifyChangeable() { if (_innerList != null) throw new NotSupportedException(SR.Get(SRID.General_ObjectIsReadOnly)); } private FamilyTypeface ConvertValue(object obj) { if (obj == null) throw new ArgumentNullException(); FamilyTypeface familyTypeface = obj as FamilyTypeface; if (familyTypeface == null) throw new ArgumentException(SR.Get(SRID.CannotConvertType, obj.GetType(), typeof(FamilyTypeface))); return familyTypeface; } private void CopyItems(Array array, int index) { if (array == null) throw new ArgumentNullException("array"); if (array.Rank != 1) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_ArrayCannotBeMultidimensional)); Type elementType = array.GetType().GetElementType(); if (!elementType.IsAssignableFrom(typeof(FamilyTypeface))) throw new ArgumentException(SR.Get(SRID.CannotConvertType, typeof(FamilyTypeface[]), elementType)); if (index >= array.Length) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_IndexGreaterThanOrEqualToArrayLength, "index", "array")); if (_count > array.Length - index) throw new ArgumentException(SR.Get(SRID.Collection_CopyTo_NumberOfElementsExceedsArrayLength, index, "array")); if (_count != 0) { InitializeItemsFromInnerList(); Array.Copy(_items, 0, array, index, _count); } } private class Enumerator : IEnumerator, SC.IEnumerator { FamilyTypefaceCollection _list; int _index; FamilyTypeface _current; internal Enumerator(FamilyTypefaceCollection list) { _list = list; _index = -1; _current = null; } public bool MoveNext() { int count = _list.Count; if (_index < count) { _index++; if (_index < count) { _current = _list[_index]; return true; } } _current = null; return false; } void SC.IEnumerator.Reset() { _index = -1; } public FamilyTypeface Current { get { return _current; } } object SC.IEnumerator.Current { get { // If there is no current item a non-generic IEnumerator should throw an exception, // but a generic IEnumerator is not required to. if (_current == null) throw new InvalidOperationException(SR.Get(SRID.Enumerator_VerifyContext)); return _current; } } public void Dispose() { } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
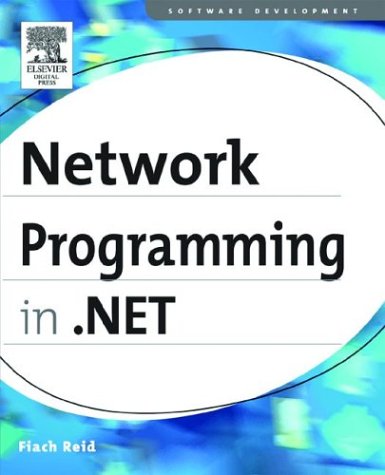
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EndpointIdentity.cs
- DataContext.cs
- ParallelTimeline.cs
- PathFigureCollection.cs
- NotImplementedException.cs
- ObfuscationAttribute.cs
- OracleConnectionFactory.cs
- RewritingValidator.cs
- RecognizedPhrase.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- PageEventArgs.cs
- QueryResponse.cs
- BufferBuilder.cs
- CreateParams.cs
- ResXResourceReader.cs
- StoreAnnotationsMap.cs
- DrawingImage.cs
- SpecularMaterial.cs
- WinEventHandler.cs
- PartManifestEntry.cs
- ChangePassword.cs
- BitmapEffectGroup.cs
- WebResponse.cs
- MaskInputRejectedEventArgs.cs
- CodeArrayIndexerExpression.cs
- TraceInternal.cs
- UIElementPropertyUndoUnit.cs
- TreeNodeCollection.cs
- RoleGroupCollection.cs
- StrokeNodeEnumerator.cs
- Int32.cs
- IdentityManager.cs
- StatusCommandUI.cs
- CompleteWizardStep.cs
- Bind.cs
- WorkflowRuntimeEndpoint.cs
- BaseTypeViewSchema.cs
- RoutedPropertyChangedEventArgs.cs
- FirstQueryOperator.cs
- RtfToken.cs
- _ListenerRequestStream.cs
- TraceUtility.cs
- DoubleConverter.cs
- RegexCode.cs
- MulticastDelegate.cs
- DataSourceXmlAttributeAttribute.cs
- Message.cs
- Keyboard.cs
- SudsCommon.cs
- DataGridViewColumn.cs
- SerializationFieldInfo.cs
- PerformanceCounterPermission.cs
- TypefaceMap.cs
- MenuItem.cs
- HostingPreferredMapPath.cs
- SelectionWordBreaker.cs
- TypeResolver.cs
- XmlSchemaAppInfo.cs
- TransformDescriptor.cs
- QilValidationVisitor.cs
- DataGridRowDetailsEventArgs.cs
- SelectionWordBreaker.cs
- EntityWithKeyStrategy.cs
- XhtmlBasicPageAdapter.cs
- RoleGroupCollection.cs
- EditorServiceContext.cs
- ByteStack.cs
- AppliedDeviceFiltersEditor.cs
- unitconverter.cs
- ConfigurationSettings.cs
- PowerModeChangedEventArgs.cs
- HotSpot.cs
- MetadataItemEmitter.cs
- EntityUtil.cs
- XmlNodeWriter.cs
- DatatypeImplementation.cs
- WindowsListBox.cs
- ExpressionBindingCollection.cs
- CustomAttribute.cs
- MobileResource.cs
- RuntimeCompatibilityAttribute.cs
- ActiveXSerializer.cs
- CommandHelper.cs
- WebPartChrome.cs
- ConfigurationConverterBase.cs
- AQNBuilder.cs
- RunWorkerCompletedEventArgs.cs
- Single.cs
- Screen.cs
- RangeValuePattern.cs
- _HeaderInfo.cs
- DelegateTypeInfo.cs
- XsdDateTime.cs
- DataSetUtil.cs
- WhitespaceRuleReader.cs
- StyleSelector.cs
- DataContractSerializerMessageContractImporter.cs
- SqlDataSourceRefreshSchemaForm.cs
- DataGridViewColumnDividerDoubleClickEventArgs.cs
- SimpleWebHandlerParser.cs