Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Documents / RtfToken.cs / 1 / RtfToken.cs
//---------------------------------------------------------------------------- // // File: RtfToken.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Rtf token that will specify the rtf token type, control and name. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { ////// Rtf token that include rtf token type, control, name and parameter value. /// internal class RtfToken { #region Internal Consts //----------------------------------------------------- // // Internal Consts // //----------------------------------------------------- internal const long INVALID_PARAMETER = 0x10000000; #endregion Internal Consts //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors ////// /// internal RtfToken() { } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal void Empty() { _type = RtfTokenType.TokenInvalid; _rtfControlWordInfo = null; _parameter = INVALID_PARAMETER; _text = ""; } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties internal RtfTokenType Type { get { return _type; } set { _type = value; } } internal RtfControlWordInfo RtfControlWordInfo { get { return _rtfControlWordInfo; } set { _rtfControlWordInfo = value; } } internal long Parameter { get { return HasParameter ? _parameter : 0; } set { _parameter = value; } } internal string Text { get { return _text; } set { _text = value; } } internal long ToggleValue { get { return HasParameter ? Parameter : 1; } } internal bool FlagValue { get { return (!HasParameter || (HasParameter && Parameter > 0) ? true : false); } } internal bool HasParameter { get { return _parameter != INVALID_PARAMETER; } } #endregion Internal Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private RtfTokenType _type; private RtfControlWordInfo _rtfControlWordInfo; private long _parameter; private string _text; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: RtfToken.cs // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: Rtf token that will specify the rtf token type, control and name. // //--------------------------------------------------------------------------- namespace System.Windows.Documents { ////// Rtf token that include rtf token type, control, name and parameter value. /// internal class RtfToken { #region Internal Consts //----------------------------------------------------- // // Internal Consts // //----------------------------------------------------- internal const long INVALID_PARAMETER = 0x10000000; #endregion Internal Consts //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors ////// /// internal RtfToken() { } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal void Empty() { _type = RtfTokenType.TokenInvalid; _rtfControlWordInfo = null; _parameter = INVALID_PARAMETER; _text = ""; } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties internal RtfTokenType Type { get { return _type; } set { _type = value; } } internal RtfControlWordInfo RtfControlWordInfo { get { return _rtfControlWordInfo; } set { _rtfControlWordInfo = value; } } internal long Parameter { get { return HasParameter ? _parameter : 0; } set { _parameter = value; } } internal string Text { get { return _text; } set { _text = value; } } internal long ToggleValue { get { return HasParameter ? Parameter : 1; } } internal bool FlagValue { get { return (!HasParameter || (HasParameter && Parameter > 0) ? true : false); } } internal bool HasParameter { get { return _parameter != INVALID_PARAMETER; } } #endregion Internal Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private RtfTokenType _type; private RtfControlWordInfo _rtfControlWordInfo; private long _parameter; private string _text; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
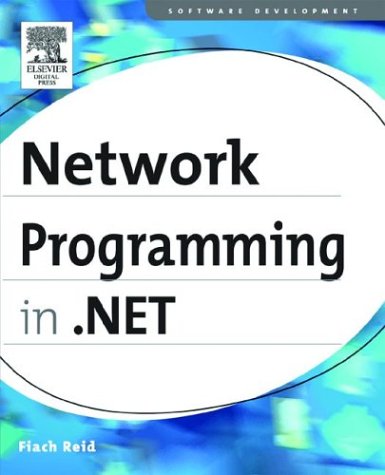
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DoubleCollectionConverter.cs
- DependencyPropertyConverter.cs
- SqlRecordBuffer.cs
- DecimalConstantAttribute.cs
- CheckPair.cs
- SignatureHelper.cs
- BaseResourcesBuildProvider.cs
- HttpGetProtocolReflector.cs
- SymDocumentType.cs
- DataGridViewAccessibleObject.cs
- OutgoingWebRequestContext.cs
- DataGridItemAttachedStorage.cs
- ListItemCollection.cs
- Rotation3DKeyFrameCollection.cs
- ControlDesignerState.cs
- Translator.cs
- IxmlLineInfo.cs
- SessionStateModule.cs
- Utils.cs
- HttpMethodConstraint.cs
- TextFormatterHost.cs
- ContentDisposition.cs
- DesignerSerializerAttribute.cs
- ScrollEventArgs.cs
- StylusSystemGestureEventArgs.cs
- EdmTypeAttribute.cs
- DeploymentSectionCache.cs
- DispatcherTimer.cs
- CodeNamespaceImportCollection.cs
- TTSEngineTypes.cs
- DataObjectCopyingEventArgs.cs
- ProfileProvider.cs
- FormatSettings.cs
- UIElementParagraph.cs
- DrawingContext.cs
- SeekStoryboard.cs
- VectorCollection.cs
- UTF8Encoding.cs
- XamlStyleSerializer.cs
- CustomErrorsSectionWrapper.cs
- PipeStream.cs
- SubMenuStyleCollectionEditor.cs
- DesignerTextViewAdapter.cs
- SplitterCancelEvent.cs
- TableLayoutSettings.cs
- ProjectionCamera.cs
- DropDownList.cs
- UnsafeNativeMethods.cs
- FormCollection.cs
- KeyEventArgs.cs
- StringConverter.cs
- Instrumentation.cs
- EntityDataSourceDesigner.cs
- SqlDataSourceView.cs
- DbConnectionPoolIdentity.cs
- MissingManifestResourceException.cs
- RenderData.cs
- ProtocolInformationWriter.cs
- LinkedDataMemberFieldEditor.cs
- RawUIStateInputReport.cs
- UnknownWrapper.cs
- XmlSchemaAnnotation.cs
- OpenTypeLayoutCache.cs
- DisplayInformation.cs
- SemanticBasicElement.cs
- GroupItemAutomationPeer.cs
- Win32KeyboardDevice.cs
- PixelFormatConverter.cs
- WebPartEditVerb.cs
- QilLiteral.cs
- DataServiceRequestException.cs
- ImageListUtils.cs
- OuterGlowBitmapEffect.cs
- TextTreeRootTextBlock.cs
- DataColumnMappingCollection.cs
- SafeLibraryHandle.cs
- QilPatternFactory.cs
- HttpHostedTransportConfiguration.cs
- SafeThreadHandle.cs
- NumberFormatInfo.cs
- MissingMemberException.cs
- XmlSchemaObject.cs
- BamlRecordReader.cs
- SafeNativeMethods.cs
- XmlSchemaSimpleTypeList.cs
- WrappingXamlSchemaContext.cs
- TypeSystemHelpers.cs
- BinaryObjectWriter.cs
- Misc.cs
- EventMappingSettingsCollection.cs
- TypeDescriptionProviderAttribute.cs
- SemanticResultValue.cs
- EdmProviderManifest.cs
- ThrowHelper.cs
- ApplicationBuildProvider.cs
- SQLMoney.cs
- XmlWriter.cs
- MaskInputRejectedEventArgs.cs
- LinearKeyFrames.cs
- SortKey.cs