Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Protocol / ProtocolInformationWriter.cs / 1 / ProtocolInformationWriter.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // Define the class that knows how to serialize WS-AT protocol information using System; using System.IO; using Microsoft.Transactions.Wsat.Recovery; namespace Microsoft.Transactions.Wsat.Protocol { enum ProtocolInformationMajorVersion : byte { v1 = 0x1 } enum ProtocolInformationMinorVersion : byte { v1 = 0x1, v2 = 0x2, } enum ProtocolInformationFlags : byte { IssuedTokensEnabled = 0x01, NetworkClientAccess = 0x02, NetworkInboundAccess = 0x04, NetworkOutboundAccess = 0x08, IsClustered = 0x10 } class ProtocolInformationWriter { ProtocolState state; public ProtocolInformationWriter(ProtocolState state) { this.state = state; } public byte[] GetProtocolInformation() { MemoryStream mem = new MemoryStream(); WriteProtocolInformation(mem); return mem.ToArray(); } void WriteProtocolInformation(MemoryStream mem) { ProtocolInformationFlags flags = GetProtocolInformationFlags(); // Version mem.WriteByte((byte)ProtocolInformationMajorVersion.v1); mem.WriteByte((byte)ProtocolInformationMinorVersion.v2); // Flags mem.WriteByte((byte)flags); // Https port SerializationUtils.WriteInt(mem, state.Config.PortConfiguration.HttpsPort); // Max timeout SerializationUtils.WriteTimeout(mem, state.Config.MaxTimeout); // Hostname used in addresses SerializationUtils.WriteString(mem, state.Config.PortConfiguration.HostName); // Base path used in addresses SerializationUtils.WriteString(mem, state.Config.PortConfiguration.BasePath); // Local node name SerializationUtils.WriteString(mem, Environment.MachineName); // End of version 1.1 // version 1.2 data // Supported protocols SerializationUtils.WriteUShort(mem, (ushort)(ProtocolVersion.Version10 | ProtocolVersion.Version11)); // End of version 1.2 data } ProtocolInformationFlags GetProtocolInformationFlags() { ProtocolInformationFlags flags = 0; // IssuedTokensEnabled if (state.Config.PortConfiguration.SupportingTokensEnabled) { flags |= ProtocolInformationFlags.IssuedTokensEnabled; } // NetworkInboundAccess if (state.TransactionManager.Settings.NetworkInboundAccess) { flags |= ProtocolInformationFlags.NetworkInboundAccess; } // NetworkOutboundAccess if (state.TransactionManager.Settings.NetworkOutboundAccess) { flags |= ProtocolInformationFlags.NetworkOutboundAccess; } // NetworkClientsAccess if (state.TransactionManager.Settings.NetworkClientAccess) { flags |= ProtocolInformationFlags.NetworkClientAccess; } // IsClustered if (state.TransactionManager.Settings.IsClustered) { flags |= ProtocolInformationFlags.IsClustered; } return flags; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
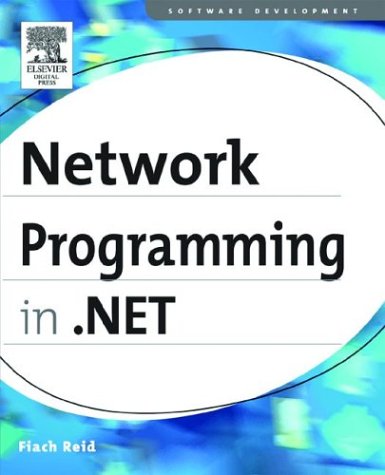
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SpAudioStreamWrapper.cs
- View.cs
- EditorZoneBase.cs
- UpdateCompiler.cs
- XXXInfos.cs
- Int64.cs
- CodeObjectCreateExpression.cs
- safelink.cs
- Timer.cs
- ExpandSegment.cs
- StyleCollection.cs
- TimeZoneNotFoundException.cs
- BindingsCollection.cs
- DataGridBoolColumn.cs
- BaseResourcesBuildProvider.cs
- InternalTransaction.cs
- ClientSession.cs
- Expressions.cs
- Group.cs
- XmlSchemaExternal.cs
- TypedReference.cs
- ObjectViewQueryResultData.cs
- MenuItemCollection.cs
- Descriptor.cs
- Empty.cs
- SqlRewriteScalarSubqueries.cs
- Setter.cs
- ControlSerializer.cs
- KeyInterop.cs
- UnsafeNativeMethods.cs
- MetadataUtil.cs
- Line.cs
- NamespaceImport.cs
- TdsRecordBufferSetter.cs
- DBCommand.cs
- RootNamespaceAttribute.cs
- ImageConverter.cs
- ProtectedConfigurationSection.cs
- PeerDuplexChannel.cs
- GenerateTemporaryTargetAssembly.cs
- TypeBuilderInstantiation.cs
- SrgsNameValueTag.cs
- DrawListViewItemEventArgs.cs
- BrowserDefinitionCollection.cs
- Brush.cs
- SetIterators.cs
- DataTemplateSelector.cs
- EmptyStringExpandableObjectConverter.cs
- XmlSigningNodeWriter.cs
- AbandonedMutexException.cs
- NavigateEvent.cs
- ChannelServices.cs
- TrackingLocationCollection.cs
- IdentityModelStringsVersion1.cs
- ResourceIDHelper.cs
- DeriveBytes.cs
- TabControl.cs
- HttpListener.cs
- ProviderConnectionPointCollection.cs
- SqlClientFactory.cs
- IntAverageAggregationOperator.cs
- Constants.cs
- SocketPermission.cs
- SemanticKeyElement.cs
- AutomationPatternInfo.cs
- RuntimeEnvironment.cs
- RegexReplacement.cs
- BulletDecorator.cs
- TextRangeProviderWrapper.cs
- AddInControllerImpl.cs
- DigestComparer.cs
- WebPartActionVerb.cs
- WebPartUserCapability.cs
- DocumentViewerBaseAutomationPeer.cs
- MdbDataFileEditor.cs
- StringExpressionSet.cs
- SelectionGlyphBase.cs
- _ListenerRequestStream.cs
- RepeaterItemEventArgs.cs
- SiteMapNodeCollection.cs
- PtsHelper.cs
- GenericParameterDataContract.cs
- NullableConverter.cs
- UIElementHelper.cs
- DSASignatureFormatter.cs
- URLMembershipCondition.cs
- TypeConverterHelper.cs
- StructuredCompositeActivityDesigner.cs
- InfoCardSymmetricAlgorithm.cs
- WebPartMenuStyle.cs
- PrintEvent.cs
- SqlClientWrapperSmiStreamChars.cs
- SinglePageViewer.cs
- RadioButtonBaseAdapter.cs
- HashRepartitionEnumerator.cs
- basemetadatamappingvisitor.cs
- SqlFlattener.cs
- ObjectView.cs
- ProvidersHelper.cs
- DivideByZeroException.cs