Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CompMod / System / ComponentModel / Design / Serialization / DesignerSerializerAttribute.cs / 1 / DesignerSerializerAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design.Serialization { using System.Security.Permissions; ////// This attribute can be placed on a class to indicate what serialization /// object should be used to serialize the class at design time. /// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Interface, AllowMultiple = true, Inherited = true)] public sealed class DesignerSerializerAttribute : Attribute { private string serializerTypeName; private string serializerBaseTypeName; private string typeId; ////// Creates a new designer serialization attribute. /// public DesignerSerializerAttribute(Type serializerType, Type baseSerializerType) { this.serializerTypeName = serializerType.AssemblyQualifiedName; this.serializerBaseTypeName = baseSerializerType.AssemblyQualifiedName; } ////// Creates a new designer serialization attribute. /// public DesignerSerializerAttribute(string serializerTypeName, Type baseSerializerType) { this.serializerTypeName = serializerTypeName; this.serializerBaseTypeName = baseSerializerType.AssemblyQualifiedName; } ////// Creates a new designer serialization attribute. /// public DesignerSerializerAttribute(string serializerTypeName, string baseSerializerTypeName) { this.serializerTypeName = serializerTypeName; this.serializerBaseTypeName = baseSerializerTypeName; } ////// Retrieves the fully qualified type name of the serializer. /// public string SerializerTypeName { get { return serializerTypeName; } } ////// Retrieves the fully qualified type name of the serializer base type. /// public string SerializerBaseTypeName { get { return serializerBaseTypeName; } } ////// /// public override object TypeId { get { if (typeId == null) { string baseType = serializerBaseTypeName; int comma = baseType.IndexOf(','); if (comma != -1) { baseType = baseType.Substring(0, comma); } typeId = GetType().FullName + baseType; } return typeId; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// This defines a unique ID for this attribute type. It is used /// by filtering algorithms to identify two attributes that are /// the same type. For most attributes, this just returns the /// Type instance for the attribute. EditorAttribute overrides /// this to include the type of the editor base type. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design.Serialization { using System.Security.Permissions; ////// This attribute can be placed on a class to indicate what serialization /// object should be used to serialize the class at design time. /// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Interface, AllowMultiple = true, Inherited = true)] public sealed class DesignerSerializerAttribute : Attribute { private string serializerTypeName; private string serializerBaseTypeName; private string typeId; ////// Creates a new designer serialization attribute. /// public DesignerSerializerAttribute(Type serializerType, Type baseSerializerType) { this.serializerTypeName = serializerType.AssemblyQualifiedName; this.serializerBaseTypeName = baseSerializerType.AssemblyQualifiedName; } ////// Creates a new designer serialization attribute. /// public DesignerSerializerAttribute(string serializerTypeName, Type baseSerializerType) { this.serializerTypeName = serializerTypeName; this.serializerBaseTypeName = baseSerializerType.AssemblyQualifiedName; } ////// Creates a new designer serialization attribute. /// public DesignerSerializerAttribute(string serializerTypeName, string baseSerializerTypeName) { this.serializerTypeName = serializerTypeName; this.serializerBaseTypeName = baseSerializerTypeName; } ////// Retrieves the fully qualified type name of the serializer. /// public string SerializerTypeName { get { return serializerTypeName; } } ////// Retrieves the fully qualified type name of the serializer base type. /// public string SerializerBaseTypeName { get { return serializerBaseTypeName; } } ////// /// public override object TypeId { get { if (typeId == null) { string baseType = serializerBaseTypeName; int comma = baseType.IndexOf(','); if (comma != -1) { baseType = baseType.Substring(0, comma); } typeId = GetType().FullName + baseType; } return typeId; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// This defines a unique ID for this attribute type. It is used /// by filtering algorithms to identify two attributes that are /// the same type. For most attributes, this just returns the /// Type instance for the attribute. EditorAttribute overrides /// this to include the type of the editor base type. /// ///
Link Menu
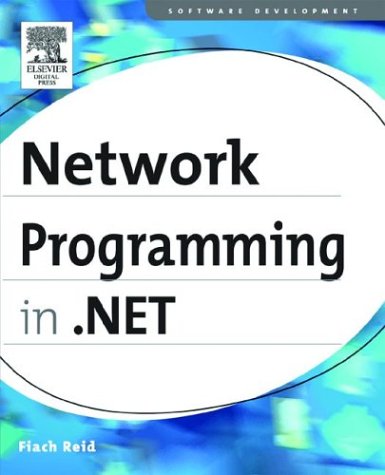
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ZipIOLocalFileHeader.cs
- ChangeBlockUndoRecord.cs
- DirectionalLight.cs
- DbConnectionFactory.cs
- DataFormats.cs
- MD5CryptoServiceProvider.cs
- ListManagerBindingsCollection.cs
- HttpCookiesSection.cs
- WinFormsComponentEditor.cs
- ClientFormsIdentity.cs
- LocalFileSettingsProvider.cs
- RedistVersionInfo.cs
- GeneralTransform3DTo2D.cs
- ClientSettingsSection.cs
- ResourcePermissionBaseEntry.cs
- CalloutQueueItem.cs
- ResXDataNode.cs
- SqlUtil.cs
- ServiceDefaults.cs
- SchemaComplexType.cs
- DbException.cs
- MimeObjectFactory.cs
- CodeDOMUtility.cs
- MailMessageEventArgs.cs
- DES.cs
- InvalidEnumArgumentException.cs
- ProgressiveCrcCalculatingStream.cs
- SQLSingleStorage.cs
- PartialClassGenerationTaskInternal.cs
- Cursor.cs
- RemotingServices.cs
- AssociationEndMember.cs
- PeerCollaboration.cs
- WebHttpElement.cs
- Tile.cs
- AttachedPropertyMethodSelector.cs
- ButtonField.cs
- RedirectionProxy.cs
- PrivateFontCollection.cs
- WriterOutput.cs
- DataBoundControlHelper.cs
- LogicalMethodInfo.cs
- HighlightOverlayGlyph.cs
- Stroke2.cs
- MarkedHighlightComponent.cs
- ReachFixedDocumentSerializerAsync.cs
- AtomServiceDocumentSerializer.cs
- ExtentCqlBlock.cs
- OperatorExpressions.cs
- ProcessThread.cs
- CodeAccessPermission.cs
- FormatSettings.cs
- JsonServiceDocumentSerializer.cs
- GradientStop.cs
- InputScopeConverter.cs
- ImageField.cs
- FontStyles.cs
- LocalizationComments.cs
- ReliableDuplexSessionChannel.cs
- NativeMethods.cs
- VectorAnimation.cs
- ClassHandlersStore.cs
- XmlDictionaryString.cs
- StatusBar.cs
- CodeThrowExceptionStatement.cs
- IndexedString.cs
- Propagator.JoinPropagator.cs
- TextLine.cs
- MergablePropertyAttribute.cs
- StrongNameKeyPair.cs
- SymLanguageType.cs
- SolidColorBrush.cs
- MsmqUri.cs
- CommandLineParser.cs
- StructuredTypeInfo.cs
- Brush.cs
- DataGridItemCollection.cs
- ValidationSummary.cs
- XmlHierarchyData.cs
- DateTimeOffsetStorage.cs
- PageAdapter.cs
- SqlNodeTypeOperators.cs
- DataRecordInternal.cs
- CollectionView.cs
- TextServicesCompartmentEventSink.cs
- Int16Storage.cs
- IndependentlyAnimatedPropertyMetadata.cs
- MDIWindowDialog.cs
- AnonymousIdentificationModule.cs
- MatrixKeyFrameCollection.cs
- x509store.cs
- DbSetClause.cs
- RegistryExceptionHelper.cs
- CollectionChangeEventArgs.cs
- FormViewDeletedEventArgs.cs
- ListViewInsertEventArgs.cs
- NullableConverter.cs
- XmlUtilWriter.cs
- IntSecurity.cs
- FlagsAttribute.cs