Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Serializers / AtomServiceDocumentSerializer.cs / 1305376 / AtomServiceDocumentSerializer.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a serializer for the Atom Service Document format. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Serializers { using System; using System.Data.Services.Providers; using System.Diagnostics; using System.IO; using System.Text; using System.Xml; ////// Provides support for serializing service models as /// a Service Document. /// ////// For more information, see http://tools.ietf.org/html/rfc5023#section-8. /// [DebuggerDisplay("AtomServiceDocumentSerializer={baseUri}")] internal sealed class AtomServiceDocumentSerializer : XmlDocumentSerializer { ///XML prefix for the Atom Publishing Protocol namespace. private const string AppNamespacePrefix = "app"; ///XML prefix for the Atom namespace. private const string AtomNamespacePrefix = "atom"; ////// Initializes a new AtomServiceDocumentSerializer, ready to write /// out the Service Document for a data provider. /// /// Stream to which output should be sent. /// Base URI from which resources should be resolved. /// Data provider from which metadata should be gathered. /// Text encoding for the response. internal AtomServiceDocumentSerializer( Stream output, Uri baseUri, DataServiceProviderWrapper provider, Encoding encoding) : base(output, baseUri, provider, encoding) { } ///Writes the Service Document to the output stream. /// Data service instance. internal override void WriteRequest(IDataService service) { try { this.Writer.WriteStartElement(XmlConstants.AtomPublishingServiceElementName, XmlConstants.AppNamespace); this.IncludeCommonNamespaces(); this.Writer.WriteStartElement("", XmlConstants.AtomPublishingWorkspaceElementName, XmlConstants.AppNamespace); this.Writer.WriteStartElement(XmlConstants.AtomTitleElementName, XmlConstants.AtomNamespace); this.Writer.WriteString(XmlConstants.AtomPublishingWorkspaceDefaultValue); this.Writer.WriteEndElement(); foreach (ResourceSetWrapper container in this.Provider.ResourceSets) { this.Writer.WriteStartElement("", XmlConstants.AtomPublishingCollectionElementName, XmlConstants.AppNamespace); this.Writer.WriteAttributeString(XmlConstants.AtomHRefAttributeName, container.Name); this.Writer.WriteStartElement(XmlConstants.AtomTitleElementName, XmlConstants.AtomNamespace); this.Writer.WriteString(container.Name); this.Writer.WriteEndElement(); // Close 'title' element. this.Writer.WriteEndElement(); // Close 'collection' element. } this.Writer.WriteEndElement(); // Close 'workspace' element. this.Writer.WriteEndElement(); // Close 'service' element. } finally { this.Writer.Close(); } } ///Ensures that common namespaces are included in the topmost tag. ////// This method should be called by any method that may write a /// topmost element tag. /// private void IncludeCommonNamespaces() { Debug.Assert( this.Writer.WriteState == WriteState.Element, "this.writer.WriteState == WriteState.Element - otherwise, not called at beginning - " + this.Writer.WriteState); this.Writer.WriteAttributeString(XmlConstants.XmlNamespacePrefix, XmlConstants.XmlBaseAttributeName, null, this.BaseUri.AbsoluteUri); this.Writer.WriteAttributeString(XmlConstants.XmlnsNamespacePrefix, AtomNamespacePrefix, null, XmlConstants.AtomNamespace); this.Writer.WriteAttributeString(XmlConstants.XmlnsNamespacePrefix, AppNamespacePrefix, null, XmlConstants.AppNamespace); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a serializer for the Atom Service Document format. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Serializers { using System; using System.Data.Services.Providers; using System.Diagnostics; using System.IO; using System.Text; using System.Xml; ////// Provides support for serializing service models as /// a Service Document. /// ////// For more information, see http://tools.ietf.org/html/rfc5023#section-8. /// [DebuggerDisplay("AtomServiceDocumentSerializer={baseUri}")] internal sealed class AtomServiceDocumentSerializer : XmlDocumentSerializer { ///XML prefix for the Atom Publishing Protocol namespace. private const string AppNamespacePrefix = "app"; ///XML prefix for the Atom namespace. private const string AtomNamespacePrefix = "atom"; ////// Initializes a new AtomServiceDocumentSerializer, ready to write /// out the Service Document for a data provider. /// /// Stream to which output should be sent. /// Base URI from which resources should be resolved. /// Data provider from which metadata should be gathered. /// Text encoding for the response. internal AtomServiceDocumentSerializer( Stream output, Uri baseUri, DataServiceProviderWrapper provider, Encoding encoding) : base(output, baseUri, provider, encoding) { } ///Writes the Service Document to the output stream. /// Data service instance. internal override void WriteRequest(IDataService service) { try { this.Writer.WriteStartElement(XmlConstants.AtomPublishingServiceElementName, XmlConstants.AppNamespace); this.IncludeCommonNamespaces(); this.Writer.WriteStartElement("", XmlConstants.AtomPublishingWorkspaceElementName, XmlConstants.AppNamespace); this.Writer.WriteStartElement(XmlConstants.AtomTitleElementName, XmlConstants.AtomNamespace); this.Writer.WriteString(XmlConstants.AtomPublishingWorkspaceDefaultValue); this.Writer.WriteEndElement(); foreach (ResourceSetWrapper container in this.Provider.ResourceSets) { this.Writer.WriteStartElement("", XmlConstants.AtomPublishingCollectionElementName, XmlConstants.AppNamespace); this.Writer.WriteAttributeString(XmlConstants.AtomHRefAttributeName, container.Name); this.Writer.WriteStartElement(XmlConstants.AtomTitleElementName, XmlConstants.AtomNamespace); this.Writer.WriteString(container.Name); this.Writer.WriteEndElement(); // Close 'title' element. this.Writer.WriteEndElement(); // Close 'collection' element. } this.Writer.WriteEndElement(); // Close 'workspace' element. this.Writer.WriteEndElement(); // Close 'service' element. } finally { this.Writer.Close(); } } ///Ensures that common namespaces are included in the topmost tag. ////// This method should be called by any method that may write a /// topmost element tag. /// private void IncludeCommonNamespaces() { Debug.Assert( this.Writer.WriteState == WriteState.Element, "this.writer.WriteState == WriteState.Element - otherwise, not called at beginning - " + this.Writer.WriteState); this.Writer.WriteAttributeString(XmlConstants.XmlNamespacePrefix, XmlConstants.XmlBaseAttributeName, null, this.BaseUri.AbsoluteUri); this.Writer.WriteAttributeString(XmlConstants.XmlnsNamespacePrefix, AtomNamespacePrefix, null, XmlConstants.AtomNamespace); this.Writer.WriteAttributeString(XmlConstants.XmlnsNamespacePrefix, AppNamespacePrefix, null, XmlConstants.AppNamespace); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
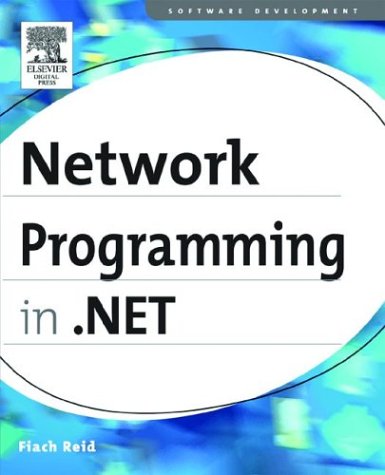
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BrowserCapabilitiesCompiler.cs
- EnumerableCollectionView.cs
- EncodingDataItem.cs
- DataGridViewTextBoxCell.cs
- EntityExpressionVisitor.cs
- ValidatorCollection.cs
- BCLDebug.cs
- CachedPathData.cs
- FunctionNode.cs
- SqlNamer.cs
- Task.cs
- ClipboardData.cs
- MarkupWriter.cs
- PixelFormatConverter.cs
- PrintPreviewDialog.cs
- XPathBinder.cs
- Zone.cs
- HttpRawResponse.cs
- unsafeIndexingFilterStream.cs
- TcpConnectionPool.cs
- CodeNamespace.cs
- BCryptHashAlgorithm.cs
- MethodRental.cs
- XPathNodeInfoAtom.cs
- ImpersonationOption.cs
- ResourcesBuildProvider.cs
- Crc32.cs
- DependencyProperty.cs
- DataKey.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- ValueTypeFixupInfo.cs
- WorkflowElementDialog.cs
- EntitySqlQueryState.cs
- MarkupCompiler.cs
- ReachIDocumentPaginatorSerializerAsync.cs
- CharUnicodeInfo.cs
- DataMemberFieldEditor.cs
- BooleanAnimationUsingKeyFrames.cs
- ObjectView.cs
- UrlPropertyAttribute.cs
- SystemException.cs
- ProcessRequestArgs.cs
- CustomBindingElement.cs
- XmlDomTextWriter.cs
- CanonicalFontFamilyReference.cs
- ScriptingWebServicesSectionGroup.cs
- XmlNodeChangedEventArgs.cs
- GenerateHelper.cs
- UdpDuplexChannel.cs
- DataGridHelper.cs
- XmlMembersMapping.cs
- SecurityTokenTypes.cs
- unsafeIndexingFilterStream.cs
- StateItem.cs
- X509RecipientCertificateServiceElement.cs
- ErasingStroke.cs
- FormClosedEvent.cs
- ImageCodecInfo.cs
- UInt32Storage.cs
- Logging.cs
- ResourceType.cs
- GlobalProxySelection.cs
- WebDisplayNameAttribute.cs
- ComAwareEventInfo.cs
- SettingsPropertyWrongTypeException.cs
- SignatureConfirmations.cs
- ActivationArguments.cs
- TcpWorkerProcess.cs
- OutputCacheProfile.cs
- Char.cs
- XmlNamespaceMapping.cs
- TextHintingModeValidation.cs
- XsltSettings.cs
- SiteOfOriginPart.cs
- TraversalRequest.cs
- HMACSHA256.cs
- EncodingInfo.cs
- DataGridPageChangedEventArgs.cs
- URLBuilder.cs
- EntityViewContainer.cs
- GridViewColumnCollection.cs
- TreeViewCancelEvent.cs
- PrintDocument.cs
- EventSourceCreationData.cs
- TextTreeUndo.cs
- mda.cs
- UIntPtr.cs
- Activity.cs
- ApplicationFileCodeDomTreeGenerator.cs
- StateWorkerRequest.cs
- ExtentCqlBlock.cs
- MouseDevice.cs
- ThrowHelper.cs
- DesignerOptionService.cs
- LineServices.cs
- ApplicationId.cs
- ContextMenuStrip.cs
- RecognizedAudio.cs
- JapaneseCalendar.cs
- FormViewCommandEventArgs.cs