Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / MS / Internal / FontCache / CanonicalFontFamilyReference.cs / 1 / CanonicalFontFamilyReference.cs
//------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: The CanonicalFontFamilyReference class is the internal representation of a font // family reference. "Canonical" in this case means it has a normalized form and // the location part (if any) has been resolved to an absolute URI. // // See spec at http://team/sites/Avalon/Specs/Font%20Family%20References.doc // // History: // 1-30-2006 : niklasb - Created // //----------------------------------------------------------------------- using System; using MS.Internal; namespace MS.Internal.FontCache { internal sealed class CanonicalFontFamilyReference { ////// Create a CanonicalFontFamilyReference given a base URI and string. /// /// Base URI used to resolve the location part, if it is relative. /// Font family reference string, in the normalized form returned /// by Util.GetNormalizedFontFamilyReference. ///Returns a new CanonicalFontFamilyReference or CanonicalFontFamilyReference.Unresolved. public static CanonicalFontFamilyReference Create(Uri baseUri, string normalizedString) { string locationString; string escapedFamilyName; if (SplitFontFamilyReference(normalizedString, out locationString, out escapedFamilyName)) { Uri absoluteUri = null; string fileName = null; bool resolved = false; if (locationString == null || Util.IsReferenceToWindowsFonts(locationString)) { // No location (e.g., "#Arial") or file-name-only location (e.g., "arial.ttf#Arial") fileName = locationString; resolved = true; } else { if (Uri.TryCreate(locationString, UriKind.Absolute, out absoluteUri)) { // Location is an absolute URI. Make sure it's a supported scheme. resolved = Util.IsSupportedSchemeForAbsoluteFontFamilyUri(absoluteUri); } else if (baseUri != null && Util.IsEnumerableFontUriScheme(baseUri)) { // Location is relative to the base URI. resolved = Uri.TryCreate(baseUri, locationString, out absoluteUri); } } if (resolved) { return new CanonicalFontFamilyReference(absoluteUri, fileName, Uri.UnescapeDataString(escapedFamilyName)); } } return _unresolved; } ////// Represents a font family reference that could not be resolved, e.g., because of an /// invalid location or unsupported scheme. /// public static CanonicalFontFamilyReference Unresolved { get { return _unresolved; } } ////// Font family name. This string is not URI encoded (escaped). /// public string FamilyName { get { return _familyName; } } ////// If a font family reference's location part comprises a file name only (e.g., "arial.ttf#Arial") /// this property is the URI-encoded file name. In this case, the implied location of the file is /// the default Windows Fonts folder and the LocationUri property is null. In all other cases, /// this property is null. /// public string EscapedFileName { get { return _escapedFileName; } } ////// Gets the font location if a specific location is given; otherwise returns null indicating /// the default Windows Fonts folder. /// public Uri LocationUri { get { return _absoluteLocationUri; } } public bool Equals(CanonicalFontFamilyReference other) { return other != null && other._absoluteLocationUri == _absoluteLocationUri && other._escapedFileName == _escapedFileName && other._familyName == _familyName; } public override bool Equals(object obj) { return Equals(obj as CanonicalFontFamilyReference); } public override int GetHashCode() { if (_absoluteLocationUri == null && _escapedFileName == null) { // Typical case where no location is specified return _familyName.GetHashCode(); } else { // Either we have a URI or a file name, never both int hash = (_absoluteLocationUri != null) ? _absoluteLocationUri.GetHashCode() : _escapedFileName.GetHashCode(); // Combine the location hash with the family name hash hash = HashFn.HashMultiply(hash) + _familyName.GetHashCode(); return HashFn.HashScramble(hash); } } private CanonicalFontFamilyReference(Uri absoluteLocationUri, string escapedFileName, string familyName) { _absoluteLocationUri = absoluteLocationUri; _escapedFileName = escapedFileName; _familyName = familyName; } private static bool SplitFontFamilyReference(string normalizedString, out string locationString, out string escapedFamilyName) { int familyNameIndex; if (normalizedString[0] == '#') { locationString = null; familyNameIndex = 1; } else { int i = normalizedString.IndexOf('#'); locationString = normalizedString.Substring(0, i); familyNameIndex = i + 1; } if (familyNameIndex < normalizedString.Length) { escapedFamilyName = normalizedString.Substring(familyNameIndex); return true; } else { escapedFamilyName = null; return false; } } private Uri _absoluteLocationUri; private string _escapedFileName; private string _familyName; private static readonly CanonicalFontFamilyReference _unresolved = new CanonicalFontFamilyReference(null, null, string.Empty); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: The CanonicalFontFamilyReference class is the internal representation of a font // family reference. "Canonical" in this case means it has a normalized form and // the location part (if any) has been resolved to an absolute URI. // // See spec at http://team/sites/Avalon/Specs/Font%20Family%20References.doc // // History: // 1-30-2006 : niklasb - Created // //----------------------------------------------------------------------- using System; using MS.Internal; namespace MS.Internal.FontCache { internal sealed class CanonicalFontFamilyReference { ////// Create a CanonicalFontFamilyReference given a base URI and string. /// /// Base URI used to resolve the location part, if it is relative. /// Font family reference string, in the normalized form returned /// by Util.GetNormalizedFontFamilyReference. ///Returns a new CanonicalFontFamilyReference or CanonicalFontFamilyReference.Unresolved. public static CanonicalFontFamilyReference Create(Uri baseUri, string normalizedString) { string locationString; string escapedFamilyName; if (SplitFontFamilyReference(normalizedString, out locationString, out escapedFamilyName)) { Uri absoluteUri = null; string fileName = null; bool resolved = false; if (locationString == null || Util.IsReferenceToWindowsFonts(locationString)) { // No location (e.g., "#Arial") or file-name-only location (e.g., "arial.ttf#Arial") fileName = locationString; resolved = true; } else { if (Uri.TryCreate(locationString, UriKind.Absolute, out absoluteUri)) { // Location is an absolute URI. Make sure it's a supported scheme. resolved = Util.IsSupportedSchemeForAbsoluteFontFamilyUri(absoluteUri); } else if (baseUri != null && Util.IsEnumerableFontUriScheme(baseUri)) { // Location is relative to the base URI. resolved = Uri.TryCreate(baseUri, locationString, out absoluteUri); } } if (resolved) { return new CanonicalFontFamilyReference(absoluteUri, fileName, Uri.UnescapeDataString(escapedFamilyName)); } } return _unresolved; } ////// Represents a font family reference that could not be resolved, e.g., because of an /// invalid location or unsupported scheme. /// public static CanonicalFontFamilyReference Unresolved { get { return _unresolved; } } ////// Font family name. This string is not URI encoded (escaped). /// public string FamilyName { get { return _familyName; } } ////// If a font family reference's location part comprises a file name only (e.g., "arial.ttf#Arial") /// this property is the URI-encoded file name. In this case, the implied location of the file is /// the default Windows Fonts folder and the LocationUri property is null. In all other cases, /// this property is null. /// public string EscapedFileName { get { return _escapedFileName; } } ////// Gets the font location if a specific location is given; otherwise returns null indicating /// the default Windows Fonts folder. /// public Uri LocationUri { get { return _absoluteLocationUri; } } public bool Equals(CanonicalFontFamilyReference other) { return other != null && other._absoluteLocationUri == _absoluteLocationUri && other._escapedFileName == _escapedFileName && other._familyName == _familyName; } public override bool Equals(object obj) { return Equals(obj as CanonicalFontFamilyReference); } public override int GetHashCode() { if (_absoluteLocationUri == null && _escapedFileName == null) { // Typical case where no location is specified return _familyName.GetHashCode(); } else { // Either we have a URI or a file name, never both int hash = (_absoluteLocationUri != null) ? _absoluteLocationUri.GetHashCode() : _escapedFileName.GetHashCode(); // Combine the location hash with the family name hash hash = HashFn.HashMultiply(hash) + _familyName.GetHashCode(); return HashFn.HashScramble(hash); } } private CanonicalFontFamilyReference(Uri absoluteLocationUri, string escapedFileName, string familyName) { _absoluteLocationUri = absoluteLocationUri; _escapedFileName = escapedFileName; _familyName = familyName; } private static bool SplitFontFamilyReference(string normalizedString, out string locationString, out string escapedFamilyName) { int familyNameIndex; if (normalizedString[0] == '#') { locationString = null; familyNameIndex = 1; } else { int i = normalizedString.IndexOf('#'); locationString = normalizedString.Substring(0, i); familyNameIndex = i + 1; } if (familyNameIndex < normalizedString.Length) { escapedFamilyName = normalizedString.Substring(familyNameIndex); return true; } else { escapedFamilyName = null; return false; } } private Uri _absoluteLocationUri; private string _escapedFileName; private string _familyName; private static readonly CanonicalFontFamilyReference _unresolved = new CanonicalFontFamilyReference(null, null, string.Empty); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
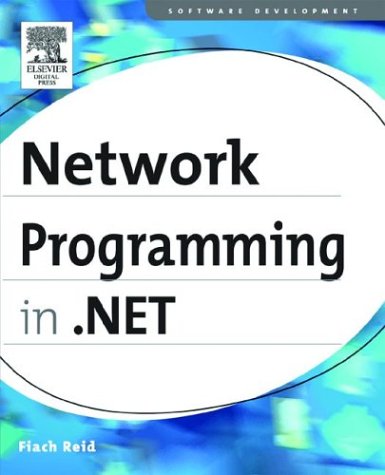
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StyleBamlRecordReader.cs
- ProxyWebPartManager.cs
- WarningException.cs
- ScriptRegistrationManager.cs
- RSAOAEPKeyExchangeDeformatter.cs
- SamlSecurityToken.cs
- StringConcat.cs
- LocatorPartList.cs
- SingleAnimationBase.cs
- StrongNameMembershipCondition.cs
- CheckBoxBaseAdapter.cs
- PriorityBinding.cs
- UIElementCollection.cs
- LicenseManager.cs
- DataBinding.cs
- ParseChildrenAsPropertiesAttribute.cs
- SecUtil.cs
- SystemNetworkInterface.cs
- selecteditemcollection.cs
- ScrollBarAutomationPeer.cs
- TextChangedEventArgs.cs
- ConfigurationConverterBase.cs
- InternalResources.cs
- EditorPartChrome.cs
- IndicCharClassifier.cs
- IChannel.cs
- XmlUtf8RawTextWriter.cs
- SqlDataSourceStatusEventArgs.cs
- ArcSegment.cs
- XmlDocument.cs
- ScrollProviderWrapper.cs
- DocumentAutomationPeer.cs
- TimeSpanStorage.cs
- PopupRoot.cs
- FileFormatException.cs
- TdsParserStateObject.cs
- ContextConfiguration.cs
- PathData.cs
- ExtractorMetadata.cs
- ListControl.cs
- GeometryConverter.cs
- ActivitySurrogate.cs
- smtpconnection.cs
- ObjectListShowCommandsEventArgs.cs
- XmlElementAttribute.cs
- ClientSettingsStore.cs
- SafeCertificateStore.cs
- ErrorFormatter.cs
- WindowClosedEventArgs.cs
- RectangleF.cs
- BlurBitmapEffect.cs
- Decimal.cs
- RectangleHotSpot.cs
- InputManager.cs
- SafeCertificateContext.cs
- BindingObserver.cs
- TransformValueSerializer.cs
- PassportIdentity.cs
- TagNameToTypeMapper.cs
- TextContainerHelper.cs
- ScalarRestriction.cs
- SqlCacheDependency.cs
- LocationUpdates.cs
- CodePageUtils.cs
- ViewDesigner.cs
- AsymmetricSignatureFormatter.cs
- EndPoint.cs
- FixedSOMPageElement.cs
- DesignBindingConverter.cs
- XmlWriter.cs
- ManagementObjectSearcher.cs
- sitestring.cs
- ComponentResourceKey.cs
- TreeSet.cs
- ScriptingAuthenticationServiceSection.cs
- MetaTableHelper.cs
- ResizeBehavior.cs
- TextEditorCharacters.cs
- BrowserCapabilitiesFactoryBase.cs
- MetadataUtil.cs
- MultiSelectRootGridEntry.cs
- GenericTextProperties.cs
- DataListCommandEventArgs.cs
- SqlConnectionPoolGroupProviderInfo.cs
- ExceptionUtility.cs
- CodeAttributeArgumentCollection.cs
- BufferedReceiveManager.cs
- PeerNameRecord.cs
- Error.cs
- EnvironmentPermission.cs
- DataStreams.cs
- ResizeGrip.cs
- ErrorsHelper.cs
- webproxy.cs
- ColorMap.cs
- ParamArrayAttribute.cs
- SocketInformation.cs
- AuthenticateEventArgs.cs
- WebPartTransformerCollection.cs
- DecimalConverter.cs