Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / ParseChildrenAsPropertiesAttribute.cs / 1 / ParseChildrenAsPropertiesAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections; using System.ComponentModel; using System.Security.Permissions; using System.Web.Util; ////// Define the metadata attribute that controls use to mark the fact /// that their children are in fact properties. /// Furthermore, if a string is passed in the constructor, it specifies /// the name of the defaultproperty. /// [AttributeUsage(AttributeTargets.Class)] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class ParseChildrenAttribute : Attribute { public static readonly ParseChildrenAttribute ParseAsChildren = new ParseChildrenAttribute(false, false); public static readonly ParseChildrenAttribute ParseAsProperties = new ParseChildrenAttribute(true, false); public static readonly ParseChildrenAttribute Default = ParseAsChildren; private bool _childrenAsProps; private string _defaultProperty; private Type _childControlType; private bool _allowChanges = true; ////// Needed to use named parameters (ASURT 78869) /// public ParseChildrenAttribute() : this(false, null) { } ////// public ParseChildrenAttribute(bool childrenAsProperties) : this(childrenAsProperties, null) { } public ParseChildrenAttribute(Type childControlType) : this(false, null) { if (childControlType == null) { throw new ArgumentNullException("childControlType"); } _childControlType = childControlType; } ////// Needed to create immutable static readonly instances of this attribute /// private ParseChildrenAttribute(bool childrenAsProperties, bool allowChanges) : this(childrenAsProperties, null) { _allowChanges = allowChanges; } ////// public ParseChildrenAttribute(bool childrenAsProperties, string defaultProperty) { _childrenAsProps = childrenAsProperties; if (_childrenAsProps == true) { _defaultProperty = defaultProperty; } } ////// public Type ChildControlType { get { if (_childControlType == null) { return typeof(System.Web.UI.Control); } return _childControlType; } } ///Indicates the allowed child control type. /// This property is read-only. ////// public bool ChildrenAsProperties { get { return _childrenAsProps; } set { if (_allowChanges == false) { throw new NotSupportedException(); } _childrenAsProps = value; } } ////// public string DefaultProperty { get { if (_defaultProperty == null) { return String.Empty; } return _defaultProperty; } set { if (_allowChanges == false) { throw new NotSupportedException(); } _defaultProperty = value; } } ////// /// public override int GetHashCode() { if (_childrenAsProps == false) { return HashCodeCombiner.CombineHashCodes(_childrenAsProps.GetHashCode(), _childControlType.GetHashCode()); } else { return HashCodeCombiner.CombineHashCodes(_childrenAsProps.GetHashCode(), DefaultProperty.GetHashCode()); } } ////// /// public override bool Equals(object obj) { if (obj == this) { return true; } ParseChildrenAttribute pca = obj as ParseChildrenAttribute; if (pca != null) { if (_childrenAsProps == false) { return pca.ChildrenAsProperties == false && pca._childControlType == _childControlType; } else { return pca.ChildrenAsProperties && (DefaultProperty.Equals(pca.DefaultProperty)); } } return false; } ////// /// public override bool IsDefaultAttribute() { return this.Equals(Default); } } }
Link Menu
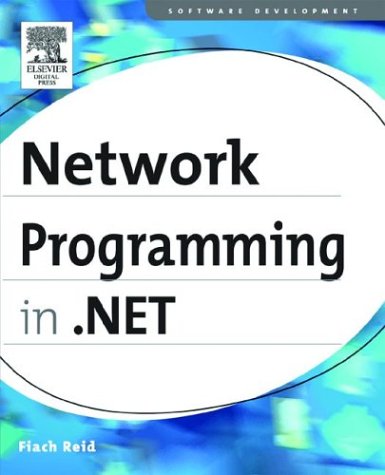
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SecurityRequiresReviewAttribute.cs
- RunWorkerCompletedEventArgs.cs
- ValidationVisibilityAttribute.cs
- RijndaelManaged.cs
- SoapReflectionImporter.cs
- Comparer.cs
- Missing.cs
- KnowledgeBase.cs
- MenuItem.cs
- Material.cs
- RecipientServiceModelSecurityTokenRequirement.cs
- OrderedDictionary.cs
- StateBag.cs
- XmlSchema.cs
- DecoderFallback.cs
- DeploymentSectionCache.cs
- Stacktrace.cs
- ToolStripGripRenderEventArgs.cs
- Helpers.cs
- CompilationSection.cs
- SecureEnvironment.cs
- RPIdentityRequirement.cs
- XmlSchemaSubstitutionGroup.cs
- ApplicationCommands.cs
- rsa.cs
- DesignerInterfaces.cs
- TraversalRequest.cs
- TimeSpan.cs
- NumberFormatter.cs
- ValueQuery.cs
- ParenthesizePropertyNameAttribute.cs
- HtmlShim.cs
- ObservableCollection.cs
- StringWriter.cs
- DataView.cs
- KernelTypeValidation.cs
- TextRange.cs
- SqlCommandSet.cs
- ISessionStateStore.cs
- StackBuilderSink.cs
- DbParameterCollection.cs
- LayoutInformation.cs
- PathHelper.cs
- TablePatternIdentifiers.cs
- DeploymentExceptionMapper.cs
- TdsEnums.cs
- RIPEMD160.cs
- CalendarDay.cs
- DataSourceUtil.cs
- Selector.cs
- CompensationToken.cs
- CaseKeyBox.xaml.cs
- SoapAttributeAttribute.cs
- ViewCellRelation.cs
- DbModificationClause.cs
- QueryBranchOp.cs
- ForEachAction.cs
- ResolveNameEventArgs.cs
- CorrelationQuery.cs
- RtfToken.cs
- SQLString.cs
- IteratorDescriptor.cs
- OutputCacheProfile.cs
- LabelLiteral.cs
- TraceLevelStore.cs
- StateDesigner.LayoutSelectionGlyph.cs
- AttributeCollection.cs
- DPCustomTypeDescriptor.cs
- DocumentViewerHelper.cs
- Thickness.cs
- CodeTypeDeclaration.cs
- DocComment.cs
- ProxyGenerationError.cs
- XmlDocument.cs
- SiteMapPath.cs
- LogEntryUtils.cs
- CodeStatement.cs
- ReferentialConstraint.cs
- SelectionItemProviderWrapper.cs
- FileSecurity.cs
- PaginationProgressEventArgs.cs
- Executor.cs
- MessageBox.cs
- DeleteHelper.cs
- ResourceKey.cs
- FrugalList.cs
- ImageConverter.cs
- PeerServiceMessageContracts.cs
- Message.cs
- WindowsFormsHostPropertyMap.cs
- BinaryFormatterWriter.cs
- Speller.cs
- Polygon.cs
- DataReaderContainer.cs
- OutputCacheModule.cs
- SmiXetterAccessMap.cs
- StyleCollection.cs
- CodeObject.cs
- WorkflowViewElement.cs
- NumberFunctions.cs