Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / DeleteHelper.cs / 1305376 / DeleteHelper.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation { using System.Activities.Presentation.Model; using System.Activities.Presentation.View; using System.Collections.Generic; using System.Linq; static class DeleteHelper { public static bool CanDelete(EditingContext context) { if (context == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("context")); } bool canExecute = false; Selection selection = context.Items.GetValue(); if (null != selection && selection.SelectionCount > 0) { DesignerView designerView = context.Services.GetService (); canExecute = selection.SelectedObjects.All( p => (null != p.View && p.View is WorkflowViewElement && !p.View.Equals(designerView.RootDesigner))); } return canExecute; } public static void Delete(EditingContext context) { if (context == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("context")); } Selection selection = context.Items.GetValue (); if (null != selection) { bool selectRoot = false; DesignerView designerView = context.Services.GetService (); var toDelete = selection.SelectedObjects.Where(p => null != p.View && p.View is WorkflowViewElement && !p.View.Equals(designerView.RootDesigner)); if (toDelete.Count() > 0) { using (EditingScope es = (EditingScope)toDelete.FirstOrDefault().BeginEdit(SR.DeleteOperationEditingScopeDescription)) { Dictionary > containerToModelItemsDict = new Dictionary >(); List modelItemsPerContainer; foreach (var item in toDelete) { ICompositeView container = (ICompositeView)DragDropHelper.GetCompositeView((WorkflowViewElement)item.View); if (null != item.Parent && typeof(ActivityAction).IsAssignableFrom(item.Parent.ItemType)) { item.Parent.Properties["Handler"].ClearValue(); selectRoot = true; } else if (null != container) { if (!containerToModelItemsDict.TryGetValue(container, out modelItemsPerContainer)) { modelItemsPerContainer = new List (); containerToModelItemsDict.Add(container, modelItemsPerContainer); } modelItemsPerContainer.Add(item); } } foreach (ICompositeView container in containerToModelItemsDict.Keys) { container.OnItemsDelete(containerToModelItemsDict[container]); selectRoot = true; } if (selectRoot) { DesignerView view = context.Services.GetService (); if (null != view) { WorkflowViewElement rootView = view.RootDesigner as WorkflowViewElement; if (rootView != null) { Selection.SelectOnly(context, rootView.ModelItem); } } } es.Complete(); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
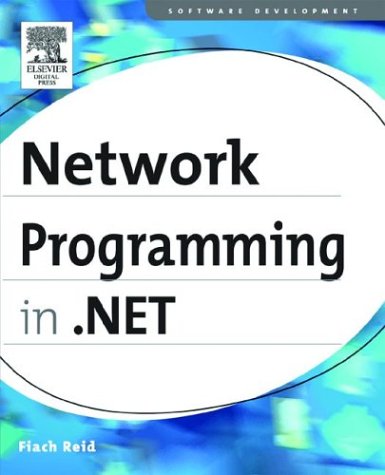
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Button.cs
- NumericUpDownAccelerationCollection.cs
- PeerCustomResolverElement.cs
- TreeNodeConverter.cs
- DbConnectionPoolOptions.cs
- ProcessHostMapPath.cs
- RsaKeyIdentifierClause.cs
- ShowExpandedMultiValueConverter.cs
- Literal.cs
- BitConverter.cs
- QuotedPrintableStream.cs
- PingReply.cs
- BitConverter.cs
- RelationshipWrapper.cs
- While.cs
- ItemAutomationPeer.cs
- PeerInvitationResponse.cs
- KoreanCalendar.cs
- Process.cs
- WbmpConverter.cs
- LockCookie.cs
- CornerRadiusConverter.cs
- QueryStringConverter.cs
- SqlVersion.cs
- ControlType.cs
- RichTextBoxContextMenu.cs
- Dictionary.cs
- EventToken.cs
- BamlStream.cs
- BuildProvidersCompiler.cs
- HostedTransportConfigurationBase.cs
- CompositeScriptReferenceEventArgs.cs
- ControlType.cs
- EntitySet.cs
- LabelLiteral.cs
- ExpressionReplacer.cs
- Resources.Designer.cs
- PostBackOptions.cs
- SymbolType.cs
- IdleTimeoutMonitor.cs
- UserControlBuildProvider.cs
- ToolStripRendererSwitcher.cs
- DataObjectMethodAttribute.cs
- SnapshotChangeTrackingStrategy.cs
- OleDbStruct.cs
- XmlSchemaAll.cs
- EventLogPermissionEntry.cs
- IERequestCache.cs
- TickBar.cs
- SmtpReplyReader.cs
- CurrentChangingEventArgs.cs
- ClassGenerator.cs
- EntityTypeEmitter.cs
- ReferentialConstraint.cs
- EventLogger.cs
- CardSpaceException.cs
- LinkedList.cs
- NullRuntimeConfig.cs
- GrammarBuilderBase.cs
- FactoryGenerator.cs
- DataServiceRequest.cs
- XmlSchemaElement.cs
- Cursor.cs
- StreamAsIStream.cs
- DeclaredTypeValidatorAttribute.cs
- Executor.cs
- EventRouteFactory.cs
- TreeNode.cs
- Ray3DHitTestResult.cs
- ListBoxItemWrapperAutomationPeer.cs
- PropertyGridView.cs
- BooleanConverter.cs
- coordinatorscratchpad.cs
- PlainXmlSerializer.cs
- SocketPermission.cs
- CqlLexer.cs
- ServerProtocol.cs
- EditingMode.cs
- ScriptServiceAttribute.cs
- KeyValueConfigurationCollection.cs
- DataGridTextBoxColumn.cs
- AsyncOperationManager.cs
- CodeRemoveEventStatement.cs
- ListViewGroupConverter.cs
- WeakReferenceList.cs
- SecondaryIndexList.cs
- XmlCharCheckingWriter.cs
- DataGridCellItemAutomationPeer.cs
- OdbcUtils.cs
- EmptyReadOnlyDictionaryInternal.cs
- XPathAncestorIterator.cs
- TagPrefixCollection.cs
- _UncName.cs
- SystemFonts.cs
- SchemaImporterExtensionElementCollection.cs
- ConnectionPoolRegistry.cs
- SurrogateEncoder.cs
- SecureEnvironment.cs
- TdsParser.cs
- ResourceContainer.cs