Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / ConnectionPoolRegistry.cs / 1 / ConnectionPoolRegistry.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Diagnostics; using System.ServiceModel; using System.Collections.Generic; using System.ServiceModel.Diagnostics; abstract class ConnectionPoolRegistry { Dictionary> registry; protected ConnectionPoolRegistry() { registry = new Dictionary >(); } object ThisLock { get { return this.registry; } } // NOTE: performs the open on the pool for you public ConnectionPool Lookup(IConnectionOrientedTransportChannelFactorySettings settings) { ConnectionPool result = null; string key = settings.ConnectionPoolGroupName; lock (ThisLock) { List registryEntry = null; if (registry.TryGetValue(key, out registryEntry)) { for (int i = 0; i < registryEntry.Count; i++) { if (registryEntry[i].IsCompatible(settings) && registryEntry[i].TryOpen()) { result = registryEntry[i]; break; } } } else { registryEntry = new List (); registry.Add(key, registryEntry); } if (result == null) { result = CreatePool(settings); registryEntry.Add(result); } } return result; } protected abstract ConnectionPool CreatePool(IConnectionOrientedTransportChannelFactorySettings settings); public void Release(ConnectionPool pool, TimeSpan timeout) { lock (ThisLock) { if (pool.Close(timeout)) { List registryEntry = registry[pool.Name]; for (int i = 0; i < registryEntry.Count; i++) { if (object.ReferenceEquals(registryEntry[i], pool)) { registryEntry.RemoveAt(i); break; } } if (registryEntry.Count == 0) { registry.Remove(pool.Name); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
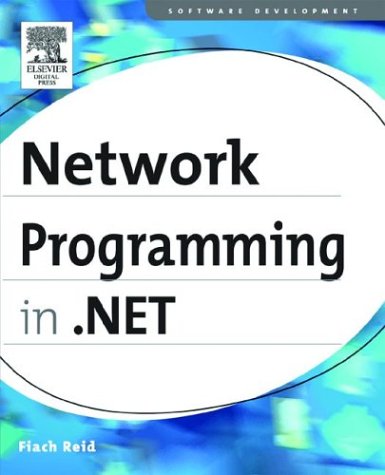
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Msmq.cs
- SqlInternalConnection.cs
- WebPartTracker.cs
- ThicknessAnimationUsingKeyFrames.cs
- SmiMetaData.cs
- SubMenuStyleCollection.cs
- TableItemStyle.cs
- SystemColorTracker.cs
- ControllableStoryboardAction.cs
- DaylightTime.cs
- DataKeyArray.cs
- UnsafeNativeMethods.cs
- ItemContainerGenerator.cs
- Rfc4050KeyFormatter.cs
- XamlFigureLengthSerializer.cs
- Sql8ConformanceChecker.cs
- SessionStateSection.cs
- TableFieldsEditor.cs
- TrackingServices.cs
- DescendantQuery.cs
- FlowNode.cs
- WebPartZoneDesigner.cs
- UriParserTemplates.cs
- ServiceReference.cs
- SHA256.cs
- ProfilePropertySettingsCollection.cs
- XmlSchemaExternal.cs
- DataGridItemCollection.cs
- PropertyCollection.cs
- WindowVisualStateTracker.cs
- SafeBitVector32.cs
- DeferredElementTreeState.cs
- TableStyle.cs
- CodeDefaultValueExpression.cs
- Emitter.cs
- BackStopAuthenticationModule.cs
- SystemIPGlobalProperties.cs
- ScrollProperties.cs
- PenThread.cs
- MenuItem.cs
- CacheEntry.cs
- ServiceHttpModule.cs
- ApplicationProxyInternal.cs
- _ListenerRequestStream.cs
- XmlAttributeProperties.cs
- Configuration.cs
- TextDecorationLocationValidation.cs
- TextOnlyOutput.cs
- TextTreeNode.cs
- TrustManagerPromptUI.cs
- DoubleLinkList.cs
- TimelineClockCollection.cs
- PointConverter.cs
- DllNotFoundException.cs
- SoapIgnoreAttribute.cs
- NamedPipeProcessProtocolHandler.cs
- Logging.cs
- DataGridPageChangedEventArgs.cs
- SkipQueryOptionExpression.cs
- XsdSchemaFileEditor.cs
- InvalidCastException.cs
- LocationUpdates.cs
- DataGridViewCellFormattingEventArgs.cs
- ServiceOperation.cs
- TextParagraph.cs
- XsdBuilder.cs
- LayoutTableCell.cs
- BufferBuilder.cs
- XmlILTrace.cs
- CellTreeNodeVisitors.cs
- Brush.cs
- PropertyChangedEventManager.cs
- ComplexObject.cs
- InternalTypeHelper.cs
- Msec.cs
- ApplicationProxyInternal.cs
- XmlSchemaParticle.cs
- HttpStreamXmlDictionaryReader.cs
- HtmlFormWrapper.cs
- CompilerState.cs
- InternalsVisibleToAttribute.cs
- DashStyles.cs
- CheckoutException.cs
- TextDecorations.cs
- TablePattern.cs
- DispatchWrapper.cs
- CodeObject.cs
- TagNameToTypeMapper.cs
- CatalogPartCollection.cs
- hresults.cs
- UnauthorizedWebPart.cs
- util.cs
- AutoGeneratedFieldProperties.cs
- DetailsViewPageEventArgs.cs
- NetNamedPipeBinding.cs
- ContainerParaClient.cs
- TimeSpanValidator.cs
- TextParagraphView.cs
- SaveFileDialog.cs
- XmlDataSource.cs