Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Channels / HttpStreamXmlDictionaryReader.cs / 1 / HttpStreamXmlDictionaryReader.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Collections.Generic; using System.Collections.ObjectModel; using System.IO; using System.Net; using System.Xml; using System.Text; using System.ServiceModel.Dispatcher; using System.ServiceModel.Syndication; using System.ServiceModel.Web; class HttpStreamXmlDictionaryReader : XmlDictionaryReader { const int InitialBufferSize = 1024; string base64StringValue; bool isStreamClosed; NameTable nameTable; StreamPosition position; XmlDictionaryReaderQuotas quotas; bool readBase64AsString; Stream stream; public HttpStreamXmlDictionaryReader(Stream stream, XmlDictionaryReaderQuotas quotas) : base() { if (stream == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("stream"); } this.stream = stream; this.position = StreamPosition.None; if (quotas == null) { quotas = XmlDictionaryReaderQuotas.Max; } this.quotas = quotas; } enum StreamPosition { None, StartElement, Stream, EndElement, EOF } public override int AttributeCount { get { return 0; } } public override string BaseURI { get { return string.Empty; } } public override bool CanCanonicalize { get { return false; } } public override bool CanReadBinaryContent { get { return true; } } public override bool CanReadValueChunk { get { return false; } } public override bool CanResolveEntity { get { return false; } } public override int Depth { get { return (this.position == StreamPosition.Stream) ? 1 : 0; } } public override bool EOF { get { return (this.position == StreamPosition.EOF); } } public override bool HasAttributes { get { return false; } } public override bool HasValue { get { return (this.position == StreamPosition.Stream); } } public override bool IsDefault { get { return false; } } public override bool IsEmptyElement { get { return false; } } public override string LocalName { get { return (this.position == StreamPosition.StartElement) ? HttpStreamMessage.StreamElementName : null; } } public override string NamespaceURI { get { return string.Empty; } } public override XmlNameTable NameTable { get { if (this.nameTable == null) { this.nameTable = new NameTable(); this.nameTable.Add(HttpStreamMessage.StreamElementName); } return this.nameTable; } } public override XmlNodeType NodeType { get { switch (position) { case StreamPosition.StartElement: return XmlNodeType.Element; case StreamPosition.Stream: return XmlNodeType.Text; case StreamPosition.EndElement: return XmlNodeType.EndElement; case StreamPosition.EOF: return XmlNodeType.None; default: return XmlNodeType.None; } } } public override string Prefix { get { return string.Empty; } } public override XmlDictionaryReaderQuotas Quotas { get { return this.quotas; } } public override ReadState ReadState { get { switch (this.position) { case StreamPosition.None: return ReadState.Initial; case StreamPosition.StartElement: case StreamPosition.Stream: case StreamPosition.EndElement: return ReadState.Interactive; case StreamPosition.EOF: return ReadState.Closed; default: Fx.Assert("This should never get hit"); return ReadState.Error; } } } public override string Value { get { switch (this.position) { case StreamPosition.Stream: return GetStreamAsBase64String(); default: return string.Empty; } } } public override void Close() { if (!this.isStreamClosed) { try { this.stream.Close(); } finally { this.position = StreamPosition.EOF; this.isStreamClosed = true; } } } public override string GetAttribute(int i) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("i")); } public override string GetAttribute(string name, string namespaceURI) { return null; } public override string GetAttribute(string name) { return null; } public override string LookupNamespace(string prefix) { if (prefix == string.Empty) { return string.Empty; } else if (prefix == "xml") { return Atom10FeedFormatter.XmlNs; } else if (prefix == "xmlns") { return Atom10FeedFormatter.XmlNsNs; } else { return null; } } public override bool MoveToAttribute(string name, string ns) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(SR2.GetString(SR2.CannotMoveToAttribute2, name, ns))); } public override bool MoveToAttribute(string name) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(SR2.GetString(SR2.CannotMoveToAttribute1, name))); } public override bool MoveToElement() { if (this.position == StreamPosition.None) { this.position = StreamPosition.StartElement; return true; } return false; } public override bool MoveToFirstAttribute() { return false; } public override bool MoveToNextAttribute() { return false; } public override bool Read() { switch (this.position) { case StreamPosition.None: position = StreamPosition.StartElement; return true; case StreamPosition.StartElement: position = StreamPosition.Stream; return true; case StreamPosition.Stream: position = StreamPosition.EndElement; return true; case StreamPosition.EndElement: position = StreamPosition.EOF; return false; case StreamPosition.EOF: return false; default: Fx.Assert("This should never get hit"); return false; } } public override bool ReadAttributeValue() { return false; } public override int ReadContentAsBase64(byte[] buffer, int index, int count) { if (buffer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("buffer"); } if (index < 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("index")); } if (count < 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("count")); } EnsureInStream(); int numBytesRead = stream.Read(buffer, index, count); if (numBytesRead == 0) { this.position = StreamPosition.EndElement; } return numBytesRead; } public override int ReadContentAsBinHex(byte[] buffer, int index, int count) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } public override void ResolveEntity() { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } void EnsureInStream() { if (this.position != StreamPosition.Stream) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new XmlException(SR2.GetString(SR2.ReaderNotPositionedAtByteStream))); } } string GetStreamAsBase64String() { if (!this.readBase64AsString) { this.base64StringValue = Convert.ToBase64String(ReadContentAsBase64()); this.readBase64AsString = true; } return this.base64StringValue; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
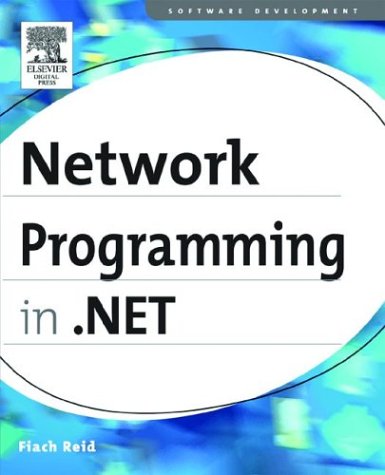
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XsdDateTime.cs
- ObjectSet.cs
- XmlNavigatorFilter.cs
- WriteableBitmap.cs
- PagerSettings.cs
- Pkcs9Attribute.cs
- DesignerEventService.cs
- PersonalizationEntry.cs
- TextSimpleMarkerProperties.cs
- ThreadWorkerController.cs
- httpserverutility.cs
- SingleKeyFrameCollection.cs
- WindowsAuthenticationEventArgs.cs
- SurrogateChar.cs
- SoapSchemaExporter.cs
- ElementHost.cs
- DataServiceHostFactory.cs
- ISAPIApplicationHost.cs
- SequenceNumber.cs
- ContentFileHelper.cs
- TableLayoutPanelCodeDomSerializer.cs
- SmtpNtlmAuthenticationModule.cs
- ViewService.cs
- CommandBinding.cs
- SecurityHelper.cs
- SystemIPInterfaceStatistics.cs
- KeyValueConfigurationElement.cs
- _PooledStream.cs
- XmlSchemaAll.cs
- CapabilitiesAssignment.cs
- ProtocolInformationWriter.cs
- DataServiceKeyAttribute.cs
- FileDialog_Vista.cs
- Parser.cs
- AlternateViewCollection.cs
- ValidationHelper.cs
- ChangeTracker.cs
- PreservationFileWriter.cs
- XmlSerializationReader.cs
- SerialPinChanges.cs
- HtmlTableRowCollection.cs
- RemotingServices.cs
- PermissionRequestEvidence.cs
- HtmlInputCheckBox.cs
- SiteMembershipCondition.cs
- ClientScriptManager.cs
- WorkflowViewElement.cs
- AlignmentXValidation.cs
- DocumentViewerHelper.cs
- XmlCDATASection.cs
- ServicePointManagerElement.cs
- FaultBookmark.cs
- CommandDevice.cs
- CodeLabeledStatement.cs
- IRCollection.cs
- Cloud.cs
- BitmapFrame.cs
- SqlDataSourceSelectingEventArgs.cs
- XmlSchemaAppInfo.cs
- BitmapData.cs
- _RegBlobWebProxyDataBuilder.cs
- PageParserFilter.cs
- ResourceKey.cs
- XmlSchemaImporter.cs
- QuaternionAnimationUsingKeyFrames.cs
- HttpListenerPrefixCollection.cs
- CharacterMetricsDictionary.cs
- Vector3DAnimationBase.cs
- TrustLevelCollection.cs
- DeviceContext2.cs
- ContractInferenceHelper.cs
- CellIdBoolean.cs
- CssTextWriter.cs
- ClientCultureInfo.cs
- ImageClickEventArgs.cs
- SendSecurityHeaderElementContainer.cs
- MetadataExchangeBindings.cs
- VectorAnimationBase.cs
- SQLString.cs
- PropertyGeneratedEventArgs.cs
- ActivityWithResultWrapper.cs
- MessageContractAttribute.cs
- CodeSpit.cs
- ResponseStream.cs
- CodeMemberProperty.cs
- SafeBitVector32.cs
- WindowsSlider.cs
- KeyNotFoundException.cs
- ModelPropertyImpl.cs
- ResolveNameEventArgs.cs
- FilterEventArgs.cs
- HttpResponseHeader.cs
- StorageAssociationSetMapping.cs
- GridViewCellAutomationPeer.cs
- WebResponse.cs
- PickBranchDesigner.xaml.cs
- NotEqual.cs
- SrgsElement.cs
- ColorMatrix.cs
- XmlUtf8RawTextWriter.cs