Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ALinq / SkipQueryOptionExpression.cs / 1305376 / SkipQueryOptionExpression.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Respresents a skip query option in resource bound expression tree. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Diagnostics; using System.Linq.Expressions; ////// An resource specific expression representing a skip query option. /// [DebuggerDisplay("SkipQueryOptionExpression {SkipAmount}")] internal class SkipQueryOptionExpression : QueryOptionExpression { ///amount to skip private ConstantExpression skipAmount; ////// Creates a SkipQueryOption expression /// /// the return type of the expression /// the query option value internal SkipQueryOptionExpression(Type type, ConstantExpression skipAmount) : base((ExpressionType)ResourceExpressionType.SkipQueryOption, type) { this.skipAmount = skipAmount; } ////// query option value /// internal ConstantExpression SkipAmount { get { return this.skipAmount; } } ////// Composes the ///expression with this one when it's specified multiple times. /// to compose. /// /// The expression that results from composing the internal override QueryOptionExpression ComposeMultipleSpecification(QueryOptionExpression previous) { Debug.Assert(previous != null, "other != null"); Debug.Assert(previous.GetType() == this.GetType(), "other.GetType == this.GetType() -- otherwise it's not the same specification"); Debug.Assert(this.skipAmount != null, "this.skipAmount != null"); Debug.Assert( this.skipAmount.Type == typeof(int), "this.skipAmount.Type == typeof(int) -- otherwise it wouldn't have matched the Enumerable.Skip(source, int count) signature"); int thisValue = (int)this.skipAmount.Value; int previousValue = (int)((SkipQueryOptionExpression)previous).skipAmount.Value; return new SkipQueryOptionExpression(this.Type, Expression.Constant(thisValue + previousValue, typeof(int))); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //expression with this one. /// // Copyright (c) Microsoft Corporation. All rights reserved. // //// Respresents a skip query option in resource bound expression tree. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Diagnostics; using System.Linq.Expressions; ////// An resource specific expression representing a skip query option. /// [DebuggerDisplay("SkipQueryOptionExpression {SkipAmount}")] internal class SkipQueryOptionExpression : QueryOptionExpression { ///amount to skip private ConstantExpression skipAmount; ////// Creates a SkipQueryOption expression /// /// the return type of the expression /// the query option value internal SkipQueryOptionExpression(Type type, ConstantExpression skipAmount) : base((ExpressionType)ResourceExpressionType.SkipQueryOption, type) { this.skipAmount = skipAmount; } ////// query option value /// internal ConstantExpression SkipAmount { get { return this.skipAmount; } } ////// Composes the ///expression with this one when it's specified multiple times. /// to compose. /// /// The expression that results from composing the internal override QueryOptionExpression ComposeMultipleSpecification(QueryOptionExpression previous) { Debug.Assert(previous != null, "other != null"); Debug.Assert(previous.GetType() == this.GetType(), "other.GetType == this.GetType() -- otherwise it's not the same specification"); Debug.Assert(this.skipAmount != null, "this.skipAmount != null"); Debug.Assert( this.skipAmount.Type == typeof(int), "this.skipAmount.Type == typeof(int) -- otherwise it wouldn't have matched the Enumerable.Skip(source, int count) signature"); int thisValue = (int)this.skipAmount.Value; int previousValue = (int)((SkipQueryOptionExpression)previous).skipAmount.Value; return new SkipQueryOptionExpression(this.Type, Expression.Constant(thisValue + previousValue, typeof(int))); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.expression with this one. ///
Link Menu
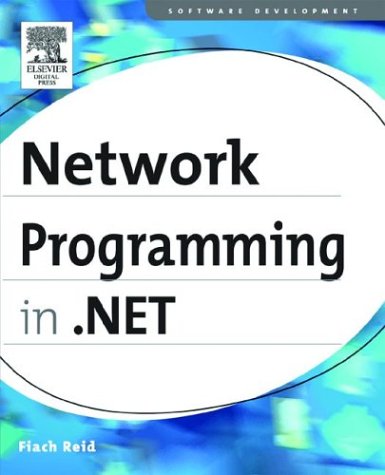
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RemotingClientProxy.cs
- PerformanceCounterManager.cs
- PasswordTextNavigator.cs
- GeneralTransform3D.cs
- PenThreadPool.cs
- FixedSOMLineRanges.cs
- PhoneCall.cs
- BinaryFormatterWriter.cs
- AttributeCollection.cs
- NavigationService.cs
- DataSourceHelper.cs
- OleDbParameterCollection.cs
- ColumnBinding.cs
- TransformerInfoCollection.cs
- LostFocusEventManager.cs
- WsrmMessageInfo.cs
- DrawItemEvent.cs
- CategoryAttribute.cs
- TraceFilter.cs
- DynamicResourceExtension.cs
- CheckBoxStandardAdapter.cs
- DefaultValidator.cs
- ModuleBuilder.cs
- ApplicationFileParser.cs
- Track.cs
- DetailsViewCommandEventArgs.cs
- TabControlAutomationPeer.cs
- SettingsProperty.cs
- SupportingTokenBindingElement.cs
- OracleConnectionString.cs
- RootNamespaceAttribute.cs
- TrackBarRenderer.cs
- FormViewInsertEventArgs.cs
- BStrWrapper.cs
- Int32CAMarshaler.cs
- XsltOutput.cs
- PropertyMap.cs
- ChannelManagerService.cs
- SQLDecimal.cs
- EnumBuilder.cs
- MasterPageCodeDomTreeGenerator.cs
- ErrorReporting.cs
- COM2PropertyBuilderUITypeEditor.cs
- BlockCollection.cs
- ICspAsymmetricAlgorithm.cs
- ComplexBindingPropertiesAttribute.cs
- BinaryNode.cs
- SqlCacheDependency.cs
- ParameterCollection.cs
- RemoteWebConfigurationHostStream.cs
- StringWriter.cs
- EmptyReadOnlyDictionaryInternal.cs
- ComPlusThreadInitializer.cs
- Margins.cs
- TransactionProxy.cs
- ClientScriptManager.cs
- PersistenceIOParticipant.cs
- MetadataUtil.cs
- BindingMemberInfo.cs
- SplineKeyFrames.cs
- StringValueSerializer.cs
- __Error.cs
- DrawingVisualDrawingContext.cs
- ProcessProtocolHandler.cs
- ItemCheckEvent.cs
- ListViewGroupConverter.cs
- SQLBinary.cs
- SqlDataSourceSelectingEventArgs.cs
- InputLanguageCollection.cs
- AnchorEditor.cs
- GreenMethods.cs
- ThreadInterruptedException.cs
- Visual.cs
- DetailsViewDeletedEventArgs.cs
- Debug.cs
- OleDbRowUpdatingEvent.cs
- TimeSpanMinutesConverter.cs
- DashStyle.cs
- IList.cs
- ToggleButton.cs
- CopyOnWriteList.cs
- processwaithandle.cs
- TextBounds.cs
- ContextTokenTypeConverter.cs
- DataGridViewCellEventArgs.cs
- SmtpTransport.cs
- DesignerOptions.cs
- EpmSourcePathSegment.cs
- ValidationResult.cs
- XmlSchemaObject.cs
- SafeViewOfFileHandle.cs
- LiteralControl.cs
- PtsContext.cs
- ScopeCompiler.cs
- RegexRunner.cs
- ActivationArguments.cs
- EmptyElement.cs
- XmlCodeExporter.cs
- WebServiceMethodData.cs
- RelatedView.cs