Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / WinFormsIntegration / System / Windows / Integration / PropertyMap.cs / 1 / PropertyMap.cs
using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Data; using System.Runtime.InteropServices; using System.Runtime.Serialization; using System.Security.Permissions; using System.Windows.Interop; using MS.Win32; using SD = System.Drawing; using SWF = System.Windows.Forms; using SW = System.Windows; using SWC = System.Windows.Controls; using SWM = System.Windows.Media; using SWMI = System.Windows.Media.Imaging; using SWS = System.Windows.Markup; using SWI = System.Windows.Input; using System.Reflection; namespace System.Windows.Forms.Integration { ////// Provides a translation function for a mapped property of the host control. /// /// The host control (either WindowsFormsHost or ElementHost) whose property is being mapped. /// The name of the property being translated. /// The new value of the property public delegate void PropertyTranslator(object host, String propertyName, object value); ////// Defines how property changes in the host control are mapped to the hosted control or element. /// [PermissionSet(SecurityAction.LinkDemand, Name = "FullTrust")] [PermissionSet(SecurityAction.InheritanceDemand, Name = "FullTrust")] public class PropertyMap { private object _sourceObject; private Dictionary_defaultTranslators; private Dictionary _wrappedDictionary; /// /// Initializes a new instance of the PropertyMap class. /// public PropertyMap() { _wrappedDictionary = new Dictionary(); } /// /// Initializes a new instance of a System.Windows.Forms.Integration.PropertyMap. /// public PropertyMap(object sourceObject) : this() { _sourceObject = sourceObject; } ////// Identifies the source of the properties in the property map. /// protected object SourceObject { get { return _sourceObject; } } ////// Gets or sets the PropertyTranslator for the specified property name. If no /// PropertyTranslator exists, it will be added to the property map. If a /// PropertyTranslator already exists, it will be replaced. /// /// The name of the host control property whose PropertyTranslator you want to get /// or set. ///public PropertyTranslator this[String propertyName] { get { ThrowIfPropertyDoesntExistOnSource(propertyName); PropertyTranslator value; if (_wrappedDictionary.TryGetValue(propertyName, out value)) { return value; } return null; } set { //Run through our regular invalid property checks, then //apply the translator if (string.IsNullOrEmpty(propertyName)) { throw new ArgumentNullException(string.Format(CultureInfo.CurrentCulture, SR.Get(SRID.WFI_NullArgument), "propertyName")); } if (value == null) { throw new ArgumentNullException(string.Format(CultureInfo.CurrentCulture, SR.Get(SRID.WFI_NullArgument), "translator")); } ThrowIfPropertyDoesntExistOnSource(propertyName); _wrappedDictionary[propertyName] = value; //This will replace an existing mapping, unlike Add. Apply(propertyName); } } /// /// Returns an ICollection of strings identifying all of the host control /// properties that have been mapped. /// public ICollection Keys { get { return _wrappedDictionary.Keys; } } ////// Returns an ICollection of all PropertyTranslator delegates currently being used to /// map the host control properties. /// public ICollection Values { get { return _wrappedDictionary.Values; } } ////// Adds a PropertyTranslator delegate to the property map that runs when the specified property /// of the host control changes. /// /// A string containing the name of the host control property to translate. /// A PropertyTranslator delegate which will be called when the specificied property changes. public void Add(String propertyName, PropertyTranslator translator) { if (Contains(propertyName)) { throw new InvalidOperationException(string.Format(CultureInfo.CurrentCulture, SR.Get(SRID.WFI_PropertyMappingExists), propertyName)); } this[propertyName] = translator; } private void ThrowIfPropertyDoesntExistOnSource(string propertyName) { if (SourceObject != null) { if (GetProperty(propertyName) == null) { // Property 'Foreground' doesn't exist on type 'Window' throw new ArgumentException(string.Format(CultureInfo.CurrentCulture, SR.Get(SRID.WFI_PropertyDoesntExist), propertyName, SourceObject.GetType().FullName)); } } } ////// Runs the PropertyTranslator for the given property, based on the /// SourceObject's current value. /// /// public void Apply(string propertyName) { if (Contains(propertyName)) { ThrowIfPropertyDoesntExistOnSource(propertyName); PropertyInfo property = GetProperty(propertyName); if (property != null) { RunTranslator(this[propertyName], SourceObject, propertyName, property.GetValue(SourceObject, null)); } } } ////// Runs the PropertyTranslator for all properties, based on the /// SourceObject's current values. /// public void ApplyAll() { foreach (KeyValuePairentry in DefaultTranslators) { Apply(entry.Key); } } private PropertyInfo GetProperty(string propertyName) { if (SourceObject == null) { return null; } return SourceObject.GetType().GetProperty(propertyName, Type.EmptyTypes); } /// /// Removes all property mappings from the property map so that the properties /// are no longer translated. /// public void Clear() { _wrappedDictionary.Clear(); } ////// Determines whether the specified property is being mapped. /// /// A string containing the name of the host control property. ///public bool Contains(String propertyName) { return _wrappedDictionary.ContainsKey(propertyName); } /// /// Removes the specified property from the property map so that the property is /// no longer translated. /// /// A string containing the name of the host control property. public void Remove(String propertyName) { _wrappedDictionary.Remove(propertyName); } ////// Gets the list of properties that are translated by default. /// protected DictionaryDefaultTranslators { get { if (_defaultTranslators == null) { _defaultTranslators = new Dictionary (); } return _defaultTranslators; } } internal bool PropertyMappedToEmptyTranslator(string propertyName) { return (this[propertyName] == EmptyPropertyTranslator); } /// /// Translator for individual property /// internal void EmptyPropertyTranslator(object host, string propertyName, object value) { } ////// Restores the default property mapping for the specified property. /// /// A string containing the name of the host control property. public void Reset(String propertyName) { if (string.IsNullOrEmpty(propertyName)) { throw new ArgumentNullException(string.Format(CultureInfo.CurrentCulture, SR.Get(SRID.WFI_ArgumentNullOrEmpty), "propertyName")); } //Resetting means get the value from the list of default values, //if it's there, otherwise removing the item you've added. PropertyTranslator value; if (DefaultTranslators.TryGetValue(propertyName, out value)) { this[propertyName] = value; } else { Remove(propertyName); } } ////// Restores the default property mapping for all of the host control's properties. /// public void ResetAll() { Clear(); AddDefaultPropertyTranslators(); } private void AddDefaultPropertyTranslators() { foreach (KeyValuePairentry in DefaultTranslators) { Add(entry.Key, entry.Value); } } /// /// Use this to look up and call the proper property translation delegate. This finds the /// delegate in this mapping, and calls it with the parameters provided. /// /// The host control (either WindowsFormsHost or ElementHost) whose property is being mapped. /// The name of the property being translated. /// The new value of the property. internal void OnPropertyChanged(object host, string propertyName, object value) { PropertyTranslator translator; if (!_wrappedDictionary.TryGetValue(propertyName, out translator) || translator == null) { return; } RunTranslator(translator, host, propertyName, value); } //Disable the PreSharp error 56500 Avoid `swallowing errors by catching non-specific exceptions. //In this specific case, we are catching the exception and firing an event which can be handled in user code //The user handling the event can make the determination on whether or not the exception should be rethrown //(Wrapped in an InvalidOperationException). #pragma warning disable 1634, 1691 #pragma warning disable 56500 internal void RunTranslator(PropertyTranslator translator, object host, string propertyName, object value) { try { translator(host, propertyName, value); } catch (Exception ex) { PropertyMappingExceptionEventArgs args = new PropertyMappingExceptionEventArgs(ex, propertyName, value); if (_propertyMappingError != null) { _propertyMappingError(SourceObject, args); } if (args.ThrowException) { throw new InvalidOperationException(SR.Get(SRID.WFI_PropertyMapError), ex); } } } #pragma warning restore 56500 #pragma warning restore 1634, 1691 private event EventHandler_propertyMappingError; /// /// Occurs when an exception is raised by a PropertyMap delegate. /// public event EventHandlerPropertyMappingError { add { _propertyMappingError += value; } remove { _propertyMappingError -= value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Data; using System.Runtime.InteropServices; using System.Runtime.Serialization; using System.Security.Permissions; using System.Windows.Interop; using MS.Win32; using SD = System.Drawing; using SWF = System.Windows.Forms; using SW = System.Windows; using SWC = System.Windows.Controls; using SWM = System.Windows.Media; using SWMI = System.Windows.Media.Imaging; using SWS = System.Windows.Markup; using SWI = System.Windows.Input; using System.Reflection; namespace System.Windows.Forms.Integration { /// /// Provides a translation function for a mapped property of the host control. /// /// The host control (either WindowsFormsHost or ElementHost) whose property is being mapped. /// The name of the property being translated. /// The new value of the property public delegate void PropertyTranslator(object host, String propertyName, object value); ////// Defines how property changes in the host control are mapped to the hosted control or element. /// [PermissionSet(SecurityAction.LinkDemand, Name = "FullTrust")] [PermissionSet(SecurityAction.InheritanceDemand, Name = "FullTrust")] public class PropertyMap { private object _sourceObject; private Dictionary_defaultTranslators; private Dictionary _wrappedDictionary; /// /// Initializes a new instance of the PropertyMap class. /// public PropertyMap() { _wrappedDictionary = new Dictionary(); } /// /// Initializes a new instance of a System.Windows.Forms.Integration.PropertyMap. /// public PropertyMap(object sourceObject) : this() { _sourceObject = sourceObject; } ////// Identifies the source of the properties in the property map. /// protected object SourceObject { get { return _sourceObject; } } ////// Gets or sets the PropertyTranslator for the specified property name. If no /// PropertyTranslator exists, it will be added to the property map. If a /// PropertyTranslator already exists, it will be replaced. /// /// The name of the host control property whose PropertyTranslator you want to get /// or set. ///public PropertyTranslator this[String propertyName] { get { ThrowIfPropertyDoesntExistOnSource(propertyName); PropertyTranslator value; if (_wrappedDictionary.TryGetValue(propertyName, out value)) { return value; } return null; } set { //Run through our regular invalid property checks, then //apply the translator if (string.IsNullOrEmpty(propertyName)) { throw new ArgumentNullException(string.Format(CultureInfo.CurrentCulture, SR.Get(SRID.WFI_NullArgument), "propertyName")); } if (value == null) { throw new ArgumentNullException(string.Format(CultureInfo.CurrentCulture, SR.Get(SRID.WFI_NullArgument), "translator")); } ThrowIfPropertyDoesntExistOnSource(propertyName); _wrappedDictionary[propertyName] = value; //This will replace an existing mapping, unlike Add. Apply(propertyName); } } /// /// Returns an ICollection of strings identifying all of the host control /// properties that have been mapped. /// public ICollection Keys { get { return _wrappedDictionary.Keys; } } ////// Returns an ICollection of all PropertyTranslator delegates currently being used to /// map the host control properties. /// public ICollection Values { get { return _wrappedDictionary.Values; } } ////// Adds a PropertyTranslator delegate to the property map that runs when the specified property /// of the host control changes. /// /// A string containing the name of the host control property to translate. /// A PropertyTranslator delegate which will be called when the specificied property changes. public void Add(String propertyName, PropertyTranslator translator) { if (Contains(propertyName)) { throw new InvalidOperationException(string.Format(CultureInfo.CurrentCulture, SR.Get(SRID.WFI_PropertyMappingExists), propertyName)); } this[propertyName] = translator; } private void ThrowIfPropertyDoesntExistOnSource(string propertyName) { if (SourceObject != null) { if (GetProperty(propertyName) == null) { // Property 'Foreground' doesn't exist on type 'Window' throw new ArgumentException(string.Format(CultureInfo.CurrentCulture, SR.Get(SRID.WFI_PropertyDoesntExist), propertyName, SourceObject.GetType().FullName)); } } } ////// Runs the PropertyTranslator for the given property, based on the /// SourceObject's current value. /// /// public void Apply(string propertyName) { if (Contains(propertyName)) { ThrowIfPropertyDoesntExistOnSource(propertyName); PropertyInfo property = GetProperty(propertyName); if (property != null) { RunTranslator(this[propertyName], SourceObject, propertyName, property.GetValue(SourceObject, null)); } } } ////// Runs the PropertyTranslator for all properties, based on the /// SourceObject's current values. /// public void ApplyAll() { foreach (KeyValuePairentry in DefaultTranslators) { Apply(entry.Key); } } private PropertyInfo GetProperty(string propertyName) { if (SourceObject == null) { return null; } return SourceObject.GetType().GetProperty(propertyName, Type.EmptyTypes); } /// /// Removes all property mappings from the property map so that the properties /// are no longer translated. /// public void Clear() { _wrappedDictionary.Clear(); } ////// Determines whether the specified property is being mapped. /// /// A string containing the name of the host control property. ///public bool Contains(String propertyName) { return _wrappedDictionary.ContainsKey(propertyName); } /// /// Removes the specified property from the property map so that the property is /// no longer translated. /// /// A string containing the name of the host control property. public void Remove(String propertyName) { _wrappedDictionary.Remove(propertyName); } ////// Gets the list of properties that are translated by default. /// protected DictionaryDefaultTranslators { get { if (_defaultTranslators == null) { _defaultTranslators = new Dictionary (); } return _defaultTranslators; } } internal bool PropertyMappedToEmptyTranslator(string propertyName) { return (this[propertyName] == EmptyPropertyTranslator); } /// /// Translator for individual property /// internal void EmptyPropertyTranslator(object host, string propertyName, object value) { } ////// Restores the default property mapping for the specified property. /// /// A string containing the name of the host control property. public void Reset(String propertyName) { if (string.IsNullOrEmpty(propertyName)) { throw new ArgumentNullException(string.Format(CultureInfo.CurrentCulture, SR.Get(SRID.WFI_ArgumentNullOrEmpty), "propertyName")); } //Resetting means get the value from the list of default values, //if it's there, otherwise removing the item you've added. PropertyTranslator value; if (DefaultTranslators.TryGetValue(propertyName, out value)) { this[propertyName] = value; } else { Remove(propertyName); } } ////// Restores the default property mapping for all of the host control's properties. /// public void ResetAll() { Clear(); AddDefaultPropertyTranslators(); } private void AddDefaultPropertyTranslators() { foreach (KeyValuePairentry in DefaultTranslators) { Add(entry.Key, entry.Value); } } /// /// Use this to look up and call the proper property translation delegate. This finds the /// delegate in this mapping, and calls it with the parameters provided. /// /// The host control (either WindowsFormsHost or ElementHost) whose property is being mapped. /// The name of the property being translated. /// The new value of the property. internal void OnPropertyChanged(object host, string propertyName, object value) { PropertyTranslator translator; if (!_wrappedDictionary.TryGetValue(propertyName, out translator) || translator == null) { return; } RunTranslator(translator, host, propertyName, value); } //Disable the PreSharp error 56500 Avoid `swallowing errors by catching non-specific exceptions. //In this specific case, we are catching the exception and firing an event which can be handled in user code //The user handling the event can make the determination on whether or not the exception should be rethrown //(Wrapped in an InvalidOperationException). #pragma warning disable 1634, 1691 #pragma warning disable 56500 internal void RunTranslator(PropertyTranslator translator, object host, string propertyName, object value) { try { translator(host, propertyName, value); } catch (Exception ex) { PropertyMappingExceptionEventArgs args = new PropertyMappingExceptionEventArgs(ex, propertyName, value); if (_propertyMappingError != null) { _propertyMappingError(SourceObject, args); } if (args.ThrowException) { throw new InvalidOperationException(SR.Get(SRID.WFI_PropertyMapError), ex); } } } #pragma warning restore 56500 #pragma warning restore 1634, 1691 private event EventHandler_propertyMappingError; /// /// Occurs when an exception is raised by a PropertyMap delegate. /// public event EventHandlerPropertyMappingError { add { _propertyMappingError += value; } remove { _propertyMappingError -= value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
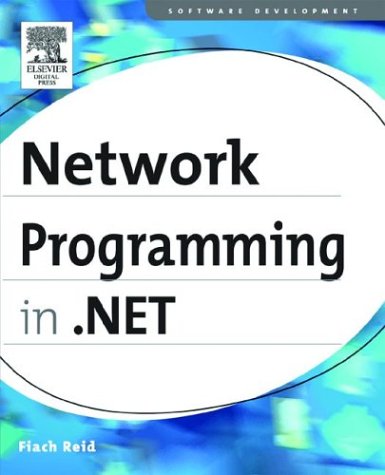
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WmlCalendarAdapter.cs
- Application.cs
- GenericUriParser.cs
- TextEffect.cs
- PersonalizationProvider.cs
- ListItemCollection.cs
- LayoutEngine.cs
- Exception.cs
- DoubleAnimationBase.cs
- BindingExpressionUncommonField.cs
- Stylesheet.cs
- XmlSchemaSimpleTypeRestriction.cs
- MethodCallConverter.cs
- SymLanguageVendor.cs
- ValueUnavailableException.cs
- PropertyIDSet.cs
- ChannelEndpointElementCollection.cs
- DataPagerFieldCollection.cs
- UnsafeNativeMethods.cs
- ControlParameter.cs
- NamespaceTable.cs
- OdbcPermission.cs
- InvalidPrinterException.cs
- BufferedOutputStream.cs
- AssertSection.cs
- OdbcEnvironmentHandle.cs
- UndoManager.cs
- MessageQueue.cs
- DataKey.cs
- HMACRIPEMD160.cs
- PrintDialog.cs
- Number.cs
- HashCodeCombiner.cs
- ReadOnlyHierarchicalDataSource.cs
- DataTransferEventArgs.cs
- CopyNamespacesAction.cs
- UnionCodeGroup.cs
- MaxValueConverter.cs
- BitmapEncoder.cs
- ProvideValueServiceProvider.cs
- ZipIORawDataFileBlock.cs
- OptimisticConcurrencyException.cs
- MissingManifestResourceException.cs
- MD5.cs
- ScriptMethodAttribute.cs
- SiteOfOriginPart.cs
- DataGridViewAutoSizeModeEventArgs.cs
- DbConnectionHelper.cs
- ScriptHandlerFactory.cs
- EdmValidator.cs
- CqlGenerator.cs
- Figure.cs
- DataPagerFieldCommandEventArgs.cs
- Int32CAMarshaler.cs
- Dump.cs
- X509ServiceCertificateAuthenticationElement.cs
- ToolStripStatusLabel.cs
- ProcessInfo.cs
- SqlExpressionNullability.cs
- Helper.cs
- DBPropSet.cs
- ProfileGroupSettingsCollection.cs
- SqlExpressionNullability.cs
- Boolean.cs
- NamespaceCollection.cs
- DashStyle.cs
- XPathQilFactory.cs
- ThreadStaticAttribute.cs
- ThrowHelper.cs
- AppDomain.cs
- TraceHelpers.cs
- PrintPreviewControl.cs
- CopyNamespacesAction.cs
- ScriptingSectionGroup.cs
- UiaCoreTypesApi.cs
- BindToObject.cs
- PolicyLevel.cs
- BufferAllocator.cs
- InvokeProviderWrapper.cs
- Vertex.cs
- AspNetSynchronizationContext.cs
- StrokeFIndices.cs
- FontStyle.cs
- EventlogProvider.cs
- DaylightTime.cs
- FacetValues.cs
- SortDescription.cs
- PrimitiveDataContract.cs
- ListViewItemSelectionChangedEvent.cs
- MouseBinding.cs
- StateChangeEvent.cs
- KeyValueConfigurationCollection.cs
- HttpCapabilitiesBase.cs
- RenderDataDrawingContext.cs
- DataListAutoFormat.cs
- BinaryParser.cs
- TrackingProvider.cs
- VarRemapper.cs
- shaper.cs
- ResourceAttributes.cs