Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / ToolStripStatusLabel.cs / 1 / ToolStripStatusLabel.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.ComponentModel; using System.Drawing; using System.Drawing.Design; using System.Diagnostics; using System.Windows.Forms.ButtonInternal; using System.Security.Permissions; using System.Security; using System.Windows.Forms.Layout; using System.Windows.Forms.Design; ////// /// A non selectable winbar item /// [ToolStripItemDesignerAvailability(ToolStripItemDesignerAvailability.StatusStrip)] public class ToolStripStatusLabel : ToolStripLabel { private Border3DStyle borderStyle = Border3DStyle.Flat; private ToolStripStatusLabelBorderSides borderSides = ToolStripStatusLabelBorderSides.None; private bool spring = false; ////// /// A non selectable winbar item /// public ToolStripStatusLabel() { } public ToolStripStatusLabel(string text):base(text,null,false,null) { } public ToolStripStatusLabel(Image image):base(null,image,false,null) { } public ToolStripStatusLabel(string text, Image image):base(text,image,false,null) { } public ToolStripStatusLabel(string text, Image image, EventHandler onClick):base(text,image,/*isLink=*/false,onClick,null) { } public ToolStripStatusLabel(string text, Image image, EventHandler onClick, string name) :base(text,image,/*isLink=*/false,onClick, name) { } ////// Creates an instance of the object that defines how image and text /// gets laid out in the ToolStripItem /// internal override ToolStripItemInternalLayout CreateInternalLayout() { return new ToolStripStatusLabelLayout(this); } [Browsable(false)] [EditorBrowsable(EditorBrowsableState.Advanced)] public new ToolStripItemAlignment Alignment { get { return base.Alignment; } set { base.Alignment = value; } } ///[ DefaultValue(Border3DStyle.Flat), SRDescription(SR.ToolStripStatusLabelBorderStyleDescr), SRCategory(SR.CatAppearance) ] public Border3DStyle BorderStyle { get { return borderStyle; } set { if (!ClientUtils.IsEnumValid_NotSequential(value, (int)value, (int)Border3DStyle.Adjust, (int)Border3DStyle.Bump, (int)Border3DStyle.Etched, (int)Border3DStyle.Flat, (int)Border3DStyle.Raised, (int)Border3DStyle.RaisedInner, (int)Border3DStyle.RaisedOuter, (int)Border3DStyle.Sunken, (int)Border3DStyle.SunkenInner, (int)Border3DStyle.SunkenOuter )) { throw new InvalidEnumArgumentException("value", (int)value, typeof(Border3DStyle)); } if (borderStyle != value) { borderStyle = value; Invalidate(); } } } /// [ DefaultValue(ToolStripStatusLabelBorderSides.None), SRDescription(SR.ToolStripStatusLabelBorderSidesDescr), SRCategory(SR.CatAppearance) ] public ToolStripStatusLabelBorderSides BorderSides { get { return borderSides; } set { // no Enum.IsDefined as this is a flags enum. if (borderSides != value) { borderSides = value; LayoutTransaction.DoLayout(Owner,this, PropertyNames.BorderStyle); Invalidate(); } } } protected internal override Padding DefaultMargin { get { return new Padding(0, 3, 0, 2); } } [ DefaultValue(false), SRDescription(SR.ToolStripStatusLabelSpringDescr), SRCategory(SR.CatAppearance) ] public bool Spring { get { return spring; } set { if (spring != value) { spring = value; if (ParentInternal != null) { LayoutTransaction.DoLayout(ParentInternal, this, PropertyNames.Spring); } } } } public override System.Drawing.Size GetPreferredSize(System.Drawing.Size constrainingSize) { if (BorderSides != ToolStripStatusLabelBorderSides.None) { return base.GetPreferredSize(constrainingSize) + new Size(4, 4); } else { return base.GetPreferredSize(constrainingSize); } } /// /// /// Inheriting classes should override this method to handle this event. /// protected override void OnPaint(System.Windows.Forms.PaintEventArgs e) { if (this.Owner != null) { ToolStripRenderer renderer = this.Renderer; renderer.DrawToolStripStatusLabelBackground(new ToolStripItemRenderEventArgs(e.Graphics, this)); if ((DisplayStyle & ToolStripItemDisplayStyle.Image) == ToolStripItemDisplayStyle.Image) { renderer.DrawItemImage(new ToolStripItemImageRenderEventArgs(e.Graphics, this, InternalLayout.ImageRectangle)); } PaintText(e.Graphics); } } ////// This class performs internal layout for the "split button button" portion of a split button. /// Its main job is to make sure the inner button has the same parent as the split button, so /// that layout can be performed using the correct graphics context. /// private class ToolStripStatusLabelLayout : ToolStripItemInternalLayout { ToolStripStatusLabel owner; public ToolStripStatusLabelLayout(ToolStripStatusLabel owner) : base(owner) { this.owner = owner; } protected override ToolStripItemLayoutOptions CommonLayoutOptions() { ToolStripItemLayoutOptions layoutOptions = base.CommonLayoutOptions(); layoutOptions.borderSize = 0; return layoutOptions; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.ComponentModel; using System.Drawing; using System.Drawing.Design; using System.Diagnostics; using System.Windows.Forms.ButtonInternal; using System.Security.Permissions; using System.Security; using System.Windows.Forms.Layout; using System.Windows.Forms.Design; ////// /// A non selectable winbar item /// [ToolStripItemDesignerAvailability(ToolStripItemDesignerAvailability.StatusStrip)] public class ToolStripStatusLabel : ToolStripLabel { private Border3DStyle borderStyle = Border3DStyle.Flat; private ToolStripStatusLabelBorderSides borderSides = ToolStripStatusLabelBorderSides.None; private bool spring = false; ////// /// A non selectable winbar item /// public ToolStripStatusLabel() { } public ToolStripStatusLabel(string text):base(text,null,false,null) { } public ToolStripStatusLabel(Image image):base(null,image,false,null) { } public ToolStripStatusLabel(string text, Image image):base(text,image,false,null) { } public ToolStripStatusLabel(string text, Image image, EventHandler onClick):base(text,image,/*isLink=*/false,onClick,null) { } public ToolStripStatusLabel(string text, Image image, EventHandler onClick, string name) :base(text,image,/*isLink=*/false,onClick, name) { } ////// Creates an instance of the object that defines how image and text /// gets laid out in the ToolStripItem /// internal override ToolStripItemInternalLayout CreateInternalLayout() { return new ToolStripStatusLabelLayout(this); } [Browsable(false)] [EditorBrowsable(EditorBrowsableState.Advanced)] public new ToolStripItemAlignment Alignment { get { return base.Alignment; } set { base.Alignment = value; } } ///[ DefaultValue(Border3DStyle.Flat), SRDescription(SR.ToolStripStatusLabelBorderStyleDescr), SRCategory(SR.CatAppearance) ] public Border3DStyle BorderStyle { get { return borderStyle; } set { if (!ClientUtils.IsEnumValid_NotSequential(value, (int)value, (int)Border3DStyle.Adjust, (int)Border3DStyle.Bump, (int)Border3DStyle.Etched, (int)Border3DStyle.Flat, (int)Border3DStyle.Raised, (int)Border3DStyle.RaisedInner, (int)Border3DStyle.RaisedOuter, (int)Border3DStyle.Sunken, (int)Border3DStyle.SunkenInner, (int)Border3DStyle.SunkenOuter )) { throw new InvalidEnumArgumentException("value", (int)value, typeof(Border3DStyle)); } if (borderStyle != value) { borderStyle = value; Invalidate(); } } } /// [ DefaultValue(ToolStripStatusLabelBorderSides.None), SRDescription(SR.ToolStripStatusLabelBorderSidesDescr), SRCategory(SR.CatAppearance) ] public ToolStripStatusLabelBorderSides BorderSides { get { return borderSides; } set { // no Enum.IsDefined as this is a flags enum. if (borderSides != value) { borderSides = value; LayoutTransaction.DoLayout(Owner,this, PropertyNames.BorderStyle); Invalidate(); } } } protected internal override Padding DefaultMargin { get { return new Padding(0, 3, 0, 2); } } [ DefaultValue(false), SRDescription(SR.ToolStripStatusLabelSpringDescr), SRCategory(SR.CatAppearance) ] public bool Spring { get { return spring; } set { if (spring != value) { spring = value; if (ParentInternal != null) { LayoutTransaction.DoLayout(ParentInternal, this, PropertyNames.Spring); } } } } public override System.Drawing.Size GetPreferredSize(System.Drawing.Size constrainingSize) { if (BorderSides != ToolStripStatusLabelBorderSides.None) { return base.GetPreferredSize(constrainingSize) + new Size(4, 4); } else { return base.GetPreferredSize(constrainingSize); } } /// /// /// Inheriting classes should override this method to handle this event. /// protected override void OnPaint(System.Windows.Forms.PaintEventArgs e) { if (this.Owner != null) { ToolStripRenderer renderer = this.Renderer; renderer.DrawToolStripStatusLabelBackground(new ToolStripItemRenderEventArgs(e.Graphics, this)); if ((DisplayStyle & ToolStripItemDisplayStyle.Image) == ToolStripItemDisplayStyle.Image) { renderer.DrawItemImage(new ToolStripItemImageRenderEventArgs(e.Graphics, this, InternalLayout.ImageRectangle)); } PaintText(e.Graphics); } } ////// This class performs internal layout for the "split button button" portion of a split button. /// Its main job is to make sure the inner button has the same parent as the split button, so /// that layout can be performed using the correct graphics context. /// private class ToolStripStatusLabelLayout : ToolStripItemInternalLayout { ToolStripStatusLabel owner; public ToolStripStatusLabelLayout(ToolStripStatusLabel owner) : base(owner) { this.owner = owner; } protected override ToolStripItemLayoutOptions CommonLayoutOptions() { ToolStripItemLayoutOptions layoutOptions = base.CommonLayoutOptions(); layoutOptions.borderSize = 0; return layoutOptions; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
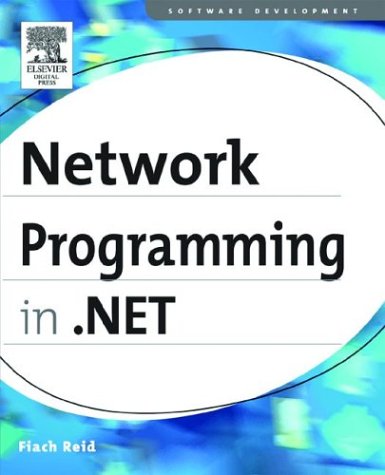
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EmptyTextWriter.cs
- BasePropertyDescriptor.cs
- UrlPropertyAttribute.cs
- RemoteCryptoTokenProvider.cs
- XhtmlBasicLabelAdapter.cs
- PatternMatcher.cs
- XPathNodeHelper.cs
- ToolStripSystemRenderer.cs
- ClientConfigurationSystem.cs
- HelloOperation11AsyncResult.cs
- AttachedPropertyBrowsableAttribute.cs
- MediaTimeline.cs
- URLString.cs
- StringResourceManager.cs
- DoubleAnimationBase.cs
- WebScriptServiceHostFactory.cs
- HtmlImage.cs
- RegionIterator.cs
- FrameworkElement.cs
- ReadingWritingEntityEventArgs.cs
- OracleBFile.cs
- ComponentCommands.cs
- GACMembershipCondition.cs
- CodeGenerator.cs
- BitmapImage.cs
- TypedOperationInfo.cs
- GACIdentityPermission.cs
- TraceListener.cs
- ManagementBaseObject.cs
- CollectionConverter.cs
- SqlServices.cs
- FrugalList.cs
- DataSourceConverter.cs
- IndexedString.cs
- ConfigurationValidatorBase.cs
- ClientRuntimeConfig.cs
- processwaithandle.cs
- ConditionalAttribute.cs
- CodePrimitiveExpression.cs
- ImageListImageEditor.cs
- HitTestDrawingContextWalker.cs
- BaseCAMarshaler.cs
- TypeUtil.cs
- DrawToolTipEventArgs.cs
- DeferredTextReference.cs
- ToolboxItemFilterAttribute.cs
- Menu.cs
- MsmqOutputSessionChannel.cs
- ContentTextAutomationPeer.cs
- SparseMemoryStream.cs
- BindingSourceDesigner.cs
- controlskin.cs
- ReliableReplySessionChannel.cs
- BCLDebug.cs
- SQLInt16.cs
- DetailsViewInsertEventArgs.cs
- XslException.cs
- ActivityPreviewDesigner.cs
- EntityStoreSchemaFilterEntry.cs
- SmiGettersStream.cs
- Clause.cs
- IdnMapping.cs
- CharAnimationUsingKeyFrames.cs
- SoapIgnoreAttribute.cs
- NotifyIcon.cs
- FirstMatchCodeGroup.cs
- TaiwanCalendar.cs
- DataGridViewTextBoxColumn.cs
- FileNotFoundException.cs
- InvokeProviderWrapper.cs
- KeySplineConverter.cs
- XamlFilter.cs
- DBCSCodePageEncoding.cs
- Constraint.cs
- SchemaCollectionCompiler.cs
- Page.cs
- SuppressMessageAttribute.cs
- ChannelSinkStacks.cs
- Metadata.cs
- CompiledQueryCacheEntry.cs
- _ListenerResponseStream.cs
- Polyline.cs
- FilterElement.cs
- ManagedFilter.cs
- TextCompositionEventArgs.cs
- WebPartConnectionCollection.cs
- LineVisual.cs
- Wow64ConfigurationLoader.cs
- CollectionViewGroup.cs
- PrintPreviewControl.cs
- Buffer.cs
- KeyInfo.cs
- XPathConvert.cs
- TextContainerChangedEventArgs.cs
- PeerObject.cs
- QuaternionRotation3D.cs
- PrtTicket_Base.cs
- HitTestParameters3D.cs
- LineUtil.cs
- EdmItemCollection.cs