Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Shapes / Polyline.cs / 1 / Polyline.cs
//---------------------------------------------------------------------------- // File: Polyline.cs // // Description: // Implementation of Polyline shape element. // // History: // 05/30/02 - AdSmith - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using MS.Internal; using System; namespace System.Windows.Shapes { ////// The Polyline shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// public sealed class Polyline : Shape { #region Constructors ////// Instantiates a new instance of a Polyline. /// public Polyline() { } #endregion Constructors #region Dynamic Properties ////// Points property /// public static readonly DependencyProperty PointsProperty = DependencyProperty.Register( "Points", typeof(PointCollection), typeof(Polyline), new FrameworkPropertyMetadata(new FreezableDefaultValueFactory(PointCollection.Empty), FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender)); ////// Points property /// public PointCollection Points { get { return (PointCollection)GetValue(PointsProperty); } set { SetValue(PointsProperty, value); } } ////// FillRule property /// public static readonly DependencyProperty FillRuleProperty = DependencyProperty.Register( "FillRule", typeof(FillRule), typeof(Polyline), new FrameworkPropertyMetadata( FillRule.EvenOdd, FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsFillRuleValid) ); ////// FillRule property /// public FillRule FillRule { get { return (FillRule)GetValue(FillRuleProperty); } set { SetValue(FillRuleProperty, value); } } #endregion Dynamic Properties #region Protected Methods and Properties ////// Get the polyline that defines this shape /// protected override Geometry DefiningGeometry { get { return _polylineGeometry; } } #endregion #region Internal methods internal override void CacheDefiningGeometry() { PointCollection pointCollection = Points; PathFigure pathFigure = new PathFigure(); // Are we degenerate? // Yes, if we don't have data if (pointCollection == null) { _polylineGeometry = Geometry.Empty; return; } // Create the Polyline PathGeometry // ISSUE-[....]-07/11/2003 - Bug 859068 // The constructor for PathFigure that takes a PointCollection is internal in the Core // so the below causes an A/V. Consider making it public. if (pointCollection.Count > 0) { pathFigure.StartPoint = pointCollection[0]; if (pointCollection.Count > 1) { Point[] array = new Point[pointCollection.Count - 1]; for (int i = 1; i < pointCollection.Count; i++) { array[i - 1] = pointCollection[i]; } pathFigure.Segments.Add(new PolyLineSegment(array, true)); } } PathGeometry polylineGeometry = new PathGeometry(); polylineGeometry.Figures.Add(pathFigure); // Set FillRule polylineGeometry.FillRule = FillRule; if (polylineGeometry.Bounds == Rect.Empty) { _polylineGeometry = Geometry.Empty; } else { _polylineGeometry = polylineGeometry; } } #endregion Internal methods #region Private Methods and Members private Geometry _polylineGeometry; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // File: Polyline.cs // // Description: // Implementation of Polyline shape element. // // History: // 05/30/02 - AdSmith - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using MS.Internal; using System; namespace System.Windows.Shapes { ////// The Polyline shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// public sealed class Polyline : Shape { #region Constructors ////// Instantiates a new instance of a Polyline. /// public Polyline() { } #endregion Constructors #region Dynamic Properties ////// Points property /// public static readonly DependencyProperty PointsProperty = DependencyProperty.Register( "Points", typeof(PointCollection), typeof(Polyline), new FrameworkPropertyMetadata(new FreezableDefaultValueFactory(PointCollection.Empty), FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender)); ////// Points property /// public PointCollection Points { get { return (PointCollection)GetValue(PointsProperty); } set { SetValue(PointsProperty, value); } } ////// FillRule property /// public static readonly DependencyProperty FillRuleProperty = DependencyProperty.Register( "FillRule", typeof(FillRule), typeof(Polyline), new FrameworkPropertyMetadata( FillRule.EvenOdd, FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(System.Windows.Media.ValidateEnums.IsFillRuleValid) ); ////// FillRule property /// public FillRule FillRule { get { return (FillRule)GetValue(FillRuleProperty); } set { SetValue(FillRuleProperty, value); } } #endregion Dynamic Properties #region Protected Methods and Properties ////// Get the polyline that defines this shape /// protected override Geometry DefiningGeometry { get { return _polylineGeometry; } } #endregion #region Internal methods internal override void CacheDefiningGeometry() { PointCollection pointCollection = Points; PathFigure pathFigure = new PathFigure(); // Are we degenerate? // Yes, if we don't have data if (pointCollection == null) { _polylineGeometry = Geometry.Empty; return; } // Create the Polyline PathGeometry // ISSUE-[....]-07/11/2003 - Bug 859068 // The constructor for PathFigure that takes a PointCollection is internal in the Core // so the below causes an A/V. Consider making it public. if (pointCollection.Count > 0) { pathFigure.StartPoint = pointCollection[0]; if (pointCollection.Count > 1) { Point[] array = new Point[pointCollection.Count - 1]; for (int i = 1; i < pointCollection.Count; i++) { array[i - 1] = pointCollection[i]; } pathFigure.Segments.Add(new PolyLineSegment(array, true)); } } PathGeometry polylineGeometry = new PathGeometry(); polylineGeometry.Figures.Add(pathFigure); // Set FillRule polylineGeometry.FillRule = FillRule; if (polylineGeometry.Bounds == Rect.Empty) { _polylineGeometry = Geometry.Empty; } else { _polylineGeometry = polylineGeometry; } } #endregion Internal methods #region Private Methods and Members private Geometry _polylineGeometry; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
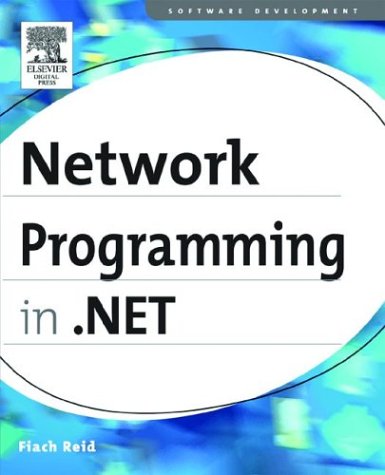
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Errors.cs
- UnsafeNativeMethods.cs
- HwndSource.cs
- RuleCache.cs
- DataGridViewElement.cs
- TextEndOfSegment.cs
- AuthorizationContext.cs
- SafeLocalAllocation.cs
- ApplicationServiceHelper.cs
- CacheForPrimitiveTypes.cs
- TextElement.cs
- ServiceHttpHandlerFactory.cs
- Imaging.cs
- CngAlgorithmGroup.cs
- InvalidCardException.cs
- OdbcError.cs
- DirectoryGroupQuery.cs
- PropertyInfoSet.cs
- XmlSchemaDatatype.cs
- SpellCheck.cs
- RadioButtonStandardAdapter.cs
- BasicHttpSecurityMode.cs
- ParagraphResult.cs
- FlagsAttribute.cs
- PasswordBoxAutomationPeer.cs
- MsmqChannelFactory.cs
- DrawingGroup.cs
- RoleGroupCollection.cs
- DataGridViewRowPrePaintEventArgs.cs
- SqlUtil.cs
- ExpressionBindingCollection.cs
- EnumType.cs
- StringUtil.cs
- PolyLineSegmentFigureLogic.cs
- Token.cs
- SoapTypeAttribute.cs
- CacheChildrenQuery.cs
- CachedPathData.cs
- UdpTransportSettings.cs
- RowVisual.cs
- DefaultValueTypeConverter.cs
- SqlConnectionFactory.cs
- xmlglyphRunInfo.cs
- EventLogPermissionHolder.cs
- BindingFormattingDialog.cs
- XmlException.cs
- WebPartsPersonalizationAuthorization.cs
- WebScriptMetadataMessage.cs
- ParenthesizePropertyNameAttribute.cs
- IndentedWriter.cs
- RangeBaseAutomationPeer.cs
- VarInfo.cs
- ZipIOCentralDirectoryFileHeader.cs
- VisualCollection.cs
- rsa.cs
- ObjectResult.cs
- PseudoWebRequest.cs
- COM2IPerPropertyBrowsingHandler.cs
- basenumberconverter.cs
- XmlDesigner.cs
- ContentType.cs
- Decoder.cs
- StringSorter.cs
- CheckedListBox.cs
- ListViewUpdateEventArgs.cs
- DesignTimeHTMLTextWriter.cs
- Trace.cs
- SurrogateSelector.cs
- ErrorWebPart.cs
- NegatedConstant.cs
- TextUtf8RawTextWriter.cs
- DataGridViewAccessibleObject.cs
- EmptyReadOnlyDictionaryInternal.cs
- RegisteredScript.cs
- DataServiceException.cs
- TextParaLineResult.cs
- ObjectItemCachedAssemblyLoader.cs
- InvalidAsynchronousStateException.cs
- Events.cs
- LambdaValue.cs
- WeakReferenceEnumerator.cs
- TimeIntervalCollection.cs
- SiteMapNodeItem.cs
- MultiPageTextView.cs
- OrderedEnumerableRowCollection.cs
- HandlerWithFactory.cs
- CodeAttributeDeclaration.cs
- updatecommandorderer.cs
- SettingsAttributeDictionary.cs
- SigningCredentials.cs
- LocalsItemDescription.cs
- ChainedAsyncResult.cs
- Stack.cs
- DecodeHelper.cs
- CreateRefExpr.cs
- ZipIOExtraFieldPaddingElement.cs
- DataServiceQueryOfT.cs
- HttpConfigurationSystem.cs
- RadioButton.cs
- InfoCardSymmetricCrypto.cs