Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Query / InternalTrees / Metadata.cs / 1305376 / Metadata.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Data.Metadata.Edm; using System.Globalization; using System.Diagnostics; namespace System.Data.Query.InternalTrees { ////// Describes metadata about a table /// internal class TableMD { private Listm_columns; private List m_keys; private EntitySetBase m_extent; // null for transient tables private bool m_flattened; /// /// private initializer /// /// the entity set corresponding to this table (if any) private TableMD(EntitySetBase extent) { m_columns = new List(); m_keys = new List (); m_extent = extent; } /// /// Create a typed-table definition corresponding to an entityset (if specified) /// /// The table has exactly one column - the type of the column is specified by /// the "type" parameter. This table is considered to be un-"flattened" /// /// type of each element (row) of the table /// entityset corresponding to the table (if any) internal TableMD(TypeUsage type, EntitySetBase extent) : this(extent) { m_columns.Add(new ColumnMD(this, "element", type)); m_flattened = !PlanCompiler.TypeUtils.IsStructuredType(type); } ////// Creates a "flattened" table definition. /// /// The table has one column for each specified property in the "properties" parameter. /// The name and datatype of each table column are taken from the corresponding property. /// /// The keys of the table (if any) are those specified in the "keyProperties" parameter /// /// The table may correspond to an entity set (if the entityset parameter was non-null) /// /// prperties corresponding to columns of the table /// /// entityset corresponding to the table (if any) internal TableMD(IEnumerableproperties, IEnumerable keyProperties, EntitySetBase extent) : this(extent) { Dictionary columnMap = new Dictionary (); m_flattened = true; foreach (EdmProperty p in properties) { ColumnMD newColumn = new ColumnMD(this, p); m_columns.Add(newColumn); columnMap[p.Name] = newColumn; } foreach (EdmMember p in keyProperties) { ColumnMD keyColumn; if (!columnMap.TryGetValue(p.Name, out keyColumn)) { Debug.Assert(false, "keyMember not in columns?"); } else { m_keys.Add(keyColumn); } } } /// /// The extent metadata (if any) /// internal EntitySetBase Extent { get { return m_extent; } } ////// List of columns of this table /// internal ListColumns { get { return m_columns; } } /// /// Keys for this table /// internal ListKeys { get { return m_keys; } } /// /// Is this table a "flat" table? /// internal bool Flattened { get { return m_flattened; } } ////// String form - for debugging /// ///public override string ToString() { return (m_extent != null ? m_extent.Name : "Transient"); } } /// /// Describes information about each column /// internal class ColumnMD { private string m_name; private TypeUsage m_type; private EdmMember m_property; ////// Default constructor /// /// Table containing this column /// Column name /// Datatype of the column internal ColumnMD(TableMD table, string name, TypeUsage type) { m_name = name; m_type = type; } ////// More useful default constructor /// /// table containing this column /// property describing this column internal ColumnMD(TableMD table, EdmMember property) : this(table, property.Name, property.TypeUsage) { m_property = property; } ////// Column Name /// internal string Name { get { return m_name; } } ////// Datatype of the column /// internal TypeUsage Type { get { return m_type; } } ////// Is this column nullable ? /// internal bool IsNullable { get { return (m_property == null) || TypeSemantics.IsNullable(m_property); } } ////// debugging help /// ///public override string ToString() { return m_name; } } /// /// Represents one instance of a table. Contains the table metadata /// internal class Table { private TableMD m_tableMetadata; private VarList m_columns; private VarVec m_referencedColumns; private VarVec m_keys; private VarVec m_nonnullableColumns; private int m_tableId; internal Table(Command command, TableMD tableMetadata, int tableId) { m_tableMetadata = tableMetadata; m_columns = Command.CreateVarList(); m_keys = command.CreateVarVec(); m_nonnullableColumns = command.CreateVarVec(); m_tableId = tableId; DictionarycolumnVarMap = new Dictionary (); foreach (ColumnMD c in tableMetadata.Columns) { ColumnVar v = command.CreateColumnVar(this, c); columnVarMap[c.Name] = v; if (!c.IsNullable) { m_nonnullableColumns.Set(v); } } foreach (ColumnMD c in tableMetadata.Keys) { ColumnVar v = columnVarMap[c.Name]; m_keys.Set(v); } m_referencedColumns = command.CreateVarVec(m_columns); } /// /// Metadata for the table instance /// internal TableMD TableMetadata { get { return m_tableMetadata; } } ////// List of column references /// internal VarList Columns { get { return m_columns; } } ////// Get the list of all referenced columns. /// internal VarVec ReferencedColumns { get { return m_referencedColumns; } } ////// /// internal VarVec NonNullableColumns { get { return m_nonnullableColumns; } } ////// List of keys /// internal VarVec Keys { get { return m_keys; } } ////// (internal) id for this table instance /// internal int TableId { get { return m_tableId; } } ////// String form - for debugging /// ///public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "{0}::{1}", m_tableMetadata.ToString(), this.TableId); ; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Data.Metadata.Edm; using System.Globalization; using System.Diagnostics; namespace System.Data.Query.InternalTrees { ////// Describes metadata about a table /// internal class TableMD { private Listm_columns; private List m_keys; private EntitySetBase m_extent; // null for transient tables private bool m_flattened; /// /// private initializer /// /// the entity set corresponding to this table (if any) private TableMD(EntitySetBase extent) { m_columns = new List(); m_keys = new List (); m_extent = extent; } /// /// Create a typed-table definition corresponding to an entityset (if specified) /// /// The table has exactly one column - the type of the column is specified by /// the "type" parameter. This table is considered to be un-"flattened" /// /// type of each element (row) of the table /// entityset corresponding to the table (if any) internal TableMD(TypeUsage type, EntitySetBase extent) : this(extent) { m_columns.Add(new ColumnMD(this, "element", type)); m_flattened = !PlanCompiler.TypeUtils.IsStructuredType(type); } ////// Creates a "flattened" table definition. /// /// The table has one column for each specified property in the "properties" parameter. /// The name and datatype of each table column are taken from the corresponding property. /// /// The keys of the table (if any) are those specified in the "keyProperties" parameter /// /// The table may correspond to an entity set (if the entityset parameter was non-null) /// /// prperties corresponding to columns of the table /// /// entityset corresponding to the table (if any) internal TableMD(IEnumerableproperties, IEnumerable keyProperties, EntitySetBase extent) : this(extent) { Dictionary columnMap = new Dictionary (); m_flattened = true; foreach (EdmProperty p in properties) { ColumnMD newColumn = new ColumnMD(this, p); m_columns.Add(newColumn); columnMap[p.Name] = newColumn; } foreach (EdmMember p in keyProperties) { ColumnMD keyColumn; if (!columnMap.TryGetValue(p.Name, out keyColumn)) { Debug.Assert(false, "keyMember not in columns?"); } else { m_keys.Add(keyColumn); } } } /// /// The extent metadata (if any) /// internal EntitySetBase Extent { get { return m_extent; } } ////// List of columns of this table /// internal ListColumns { get { return m_columns; } } /// /// Keys for this table /// internal ListKeys { get { return m_keys; } } /// /// Is this table a "flat" table? /// internal bool Flattened { get { return m_flattened; } } ////// String form - for debugging /// ///public override string ToString() { return (m_extent != null ? m_extent.Name : "Transient"); } } /// /// Describes information about each column /// internal class ColumnMD { private string m_name; private TypeUsage m_type; private EdmMember m_property; ////// Default constructor /// /// Table containing this column /// Column name /// Datatype of the column internal ColumnMD(TableMD table, string name, TypeUsage type) { m_name = name; m_type = type; } ////// More useful default constructor /// /// table containing this column /// property describing this column internal ColumnMD(TableMD table, EdmMember property) : this(table, property.Name, property.TypeUsage) { m_property = property; } ////// Column Name /// internal string Name { get { return m_name; } } ////// Datatype of the column /// internal TypeUsage Type { get { return m_type; } } ////// Is this column nullable ? /// internal bool IsNullable { get { return (m_property == null) || TypeSemantics.IsNullable(m_property); } } ////// debugging help /// ///public override string ToString() { return m_name; } } /// /// Represents one instance of a table. Contains the table metadata /// internal class Table { private TableMD m_tableMetadata; private VarList m_columns; private VarVec m_referencedColumns; private VarVec m_keys; private VarVec m_nonnullableColumns; private int m_tableId; internal Table(Command command, TableMD tableMetadata, int tableId) { m_tableMetadata = tableMetadata; m_columns = Command.CreateVarList(); m_keys = command.CreateVarVec(); m_nonnullableColumns = command.CreateVarVec(); m_tableId = tableId; DictionarycolumnVarMap = new Dictionary (); foreach (ColumnMD c in tableMetadata.Columns) { ColumnVar v = command.CreateColumnVar(this, c); columnVarMap[c.Name] = v; if (!c.IsNullable) { m_nonnullableColumns.Set(v); } } foreach (ColumnMD c in tableMetadata.Keys) { ColumnVar v = columnVarMap[c.Name]; m_keys.Set(v); } m_referencedColumns = command.CreateVarVec(m_columns); } /// /// Metadata for the table instance /// internal TableMD TableMetadata { get { return m_tableMetadata; } } ////// List of column references /// internal VarList Columns { get { return m_columns; } } ////// Get the list of all referenced columns. /// internal VarVec ReferencedColumns { get { return m_referencedColumns; } } ////// /// internal VarVec NonNullableColumns { get { return m_nonnullableColumns; } } ////// List of keys /// internal VarVec Keys { get { return m_keys; } } ////// (internal) id for this table instance /// internal int TableId { get { return m_tableId; } } ////// String form - for debugging /// ///public override string ToString() { return String.Format(CultureInfo.InvariantCulture, "{0}::{1}", m_tableMetadata.ToString(), this.TableId); ; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
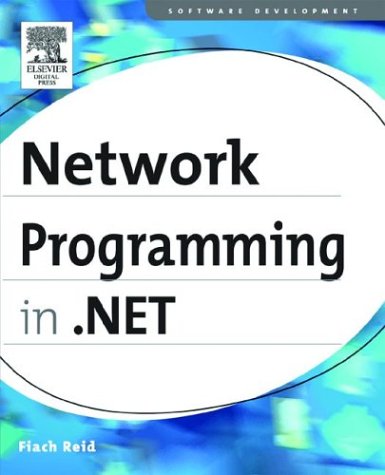
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ServiceDebugBehavior.cs
- CachedTypeface.cs
- ResourceReader.cs
- Misc.cs
- WindowsIPAddress.cs
- DataTableReader.cs
- DesignBindingPropertyDescriptor.cs
- Calendar.cs
- DBConnectionString.cs
- IpcManager.cs
- Timer.cs
- Scene3D.cs
- StreamWriter.cs
- VideoDrawing.cs
- PrintPreviewControl.cs
- CalendarKeyboardHelper.cs
- ColumnReorderedEventArgs.cs
- XmlWrappingReader.cs
- SafeLibraryHandle.cs
- UrlPropertyAttribute.cs
- SafeEventLogReadHandle.cs
- VariableQuery.cs
- SecurityUtils.cs
- HasRunnableWorkflowEvent.cs
- ControlTemplate.cs
- OrderedDictionaryStateHelper.cs
- ProcessStartInfo.cs
- MultipartContentParser.cs
- ConstraintCollection.cs
- HelpProvider.cs
- TimerEventSubscriptionCollection.cs
- DBDataPermission.cs
- SafeTokenHandle.cs
- PenContext.cs
- AutomationPropertyInfo.cs
- SizeFConverter.cs
- SetterBase.cs
- GridLengthConverter.cs
- TextDecorationUnitValidation.cs
- TaskHelper.cs
- TabControlAutomationPeer.cs
- RuleSetCollection.cs
- MailDefinition.cs
- GridItem.cs
- DecoderExceptionFallback.cs
- SqlUserDefinedTypeAttribute.cs
- CommandValueSerializer.cs
- BindingsCollection.cs
- TemplateLookupAction.cs
- SyndicationCategory.cs
- ViewBase.cs
- TokenBasedSet.cs
- OutputCacheSection.cs
- ReaderContextStackData.cs
- RegexCapture.cs
- TextControlDesigner.cs
- SByteConverter.cs
- Latin1Encoding.cs
- ImageAnimator.cs
- ArrayTypeMismatchException.cs
- TextAnchor.cs
- BidPrivateBase.cs
- MarkerProperties.cs
- SecurityCriticalDataForSet.cs
- NamedPipeTransportManager.cs
- ToolStripScrollButton.cs
- LineGeometry.cs
- System.Data.OracleClient_BID.cs
- MouseButton.cs
- Module.cs
- SByte.cs
- diagnosticsswitches.cs
- RegexCompilationInfo.cs
- DbConnectionPoolCounters.cs
- CodeMethodReturnStatement.cs
- WebPartTracker.cs
- BoundField.cs
- BuilderPropertyEntry.cs
- BridgeDataReader.cs
- BindingList.cs
- LicenseContext.cs
- List.cs
- Classification.cs
- sqlpipe.cs
- TouchesCapturedWithinProperty.cs
- SimpleWorkerRequest.cs
- AttributeCollection.cs
- XmlCodeExporter.cs
- FrameworkRichTextComposition.cs
- ScriptRegistrationManager.cs
- RtfControlWordInfo.cs
- SchemaMerger.cs
- Label.cs
- Constraint.cs
- NavigateEvent.cs
- CodeGeneratorOptions.cs
- DefaultHttpHandler.cs
- Int16Converter.cs
- OleDbStruct.cs
- Overlapped.cs