Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / HtmlControls / HtmlImage.cs / 1305376 / HtmlImage.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlImage.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System; using System.Globalization; using System.Collections; using System.ComponentModel; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ ControlBuilderAttribute(typeof(HtmlEmptyTagControlBuilder)) ] public class HtmlImage : HtmlControl { /* * Creates an intrinsic Html IMG control. */ ////// The ////// class defines the methods, properties, and events /// for the HtmlImage server control. /// This class provides programmatic access on the server to /// the HTML <img> element. /// /// public HtmlImage() : base("img") { } /* * Alt property */ ///Initializes a new instance of the ///class. /// [ WebCategory("Appearance"), Localizable(true), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Alt { get { string s = Attributes["alt"]; return((s != null) ? s : String.Empty); } set { Attributes["alt"] = MapStringAttributeToString(value); } } /* * Align property */ ////// Gets or sets the alternative caption that the /// browser displays if image is either unavailable or has not been downloaded yet. /// ////// [ WebCategory("Appearance"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Align { get { string s = Attributes["align"]; return((s != null) ? s : String.Empty); } set { Attributes["align"] = MapStringAttributeToString(value); } } /* * Border property, size of border in pixels. */ ///Gets or sets the alignment of the image with /// surrounding text. ////// [ WebCategory("Appearance"), DefaultValue(0), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int Border { get { string s = Attributes["border"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["border"] = MapIntegerAttributeToString(value); } } /* * Height property */ ////// Gets or sets the width of image border, in pixels. /// ////// [ WebCategory("Layout"), DefaultValue(100), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int Height { get { string s = Attributes["height"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["height"] = MapIntegerAttributeToString(value); } } /* * Src property. */ ////// Gets or sets /// the height of the image. By default, this is expressed in /// pixels, /// but can be a expressed as a percentage. /// ////// [ WebCategory("Behavior"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), UrlProperty() ] public string Src { get { string s = Attributes["src"]; return((s != null) ? s : String.Empty); } set { Attributes["src"] = MapStringAttributeToString(value); } } /* * Width property */ ////// Gets or sets the name of and path to the /// image file to be displayed. This can be an absolute or /// relative path. /// ////// [ WebCategory("Layout"), DefaultValue(100), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int Width { get { string s = Attributes["width"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["width"] = MapIntegerAttributeToString(value); } } /* * Override to render unique name attribute. * The name attribute is owned by the framework. */ ////// Gets or sets the width of the image. By default, this is /// expressed in pixels, /// but can be a expressed as a percentage. /// ////// /// protected override void RenderAttributes(HtmlTextWriter writer) { PreProcessRelativeReferenceAttribute(writer, "src"); base.RenderAttributes(writer); writer.Write(" /"); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlImage.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System; using System.Globalization; using System.Collections; using System.ComponentModel; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ ControlBuilderAttribute(typeof(HtmlEmptyTagControlBuilder)) ] public class HtmlImage : HtmlControl { /* * Creates an intrinsic Html IMG control. */ ////// The ////// class defines the methods, properties, and events /// for the HtmlImage server control. /// This class provides programmatic access on the server to /// the HTML <img> element. /// /// public HtmlImage() : base("img") { } /* * Alt property */ ///Initializes a new instance of the ///class. /// [ WebCategory("Appearance"), Localizable(true), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Alt { get { string s = Attributes["alt"]; return((s != null) ? s : String.Empty); } set { Attributes["alt"] = MapStringAttributeToString(value); } } /* * Align property */ ////// Gets or sets the alternative caption that the /// browser displays if image is either unavailable or has not been downloaded yet. /// ////// [ WebCategory("Appearance"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Align { get { string s = Attributes["align"]; return((s != null) ? s : String.Empty); } set { Attributes["align"] = MapStringAttributeToString(value); } } /* * Border property, size of border in pixels. */ ///Gets or sets the alignment of the image with /// surrounding text. ////// [ WebCategory("Appearance"), DefaultValue(0), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int Border { get { string s = Attributes["border"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["border"] = MapIntegerAttributeToString(value); } } /* * Height property */ ////// Gets or sets the width of image border, in pixels. /// ////// [ WebCategory("Layout"), DefaultValue(100), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int Height { get { string s = Attributes["height"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["height"] = MapIntegerAttributeToString(value); } } /* * Src property. */ ////// Gets or sets /// the height of the image. By default, this is expressed in /// pixels, /// but can be a expressed as a percentage. /// ////// [ WebCategory("Behavior"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), UrlProperty() ] public string Src { get { string s = Attributes["src"]; return((s != null) ? s : String.Empty); } set { Attributes["src"] = MapStringAttributeToString(value); } } /* * Width property */ ////// Gets or sets the name of and path to the /// image file to be displayed. This can be an absolute or /// relative path. /// ////// [ WebCategory("Layout"), DefaultValue(100), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int Width { get { string s = Attributes["width"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["width"] = MapIntegerAttributeToString(value); } } /* * Override to render unique name attribute. * The name attribute is owned by the framework. */ ////// Gets or sets the width of the image. By default, this is /// expressed in pixels, /// but can be a expressed as a percentage. /// ////// /// protected override void RenderAttributes(HtmlTextWriter writer) { PreProcessRelativeReferenceAttribute(writer, "src"); base.RenderAttributes(writer); writer.Write(" /"); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
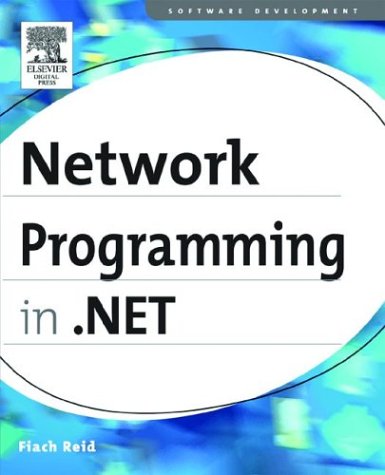
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PrinterUnitConvert.cs
- Metafile.cs
- XmlSchemaAttribute.cs
- IisTraceWebEventProvider.cs
- SnapshotChangeTrackingStrategy.cs
- Timeline.cs
- _AutoWebProxyScriptHelper.cs
- SerializationException.cs
- OleDbParameter.cs
- URLIdentityPermission.cs
- ContentPlaceHolder.cs
- CheckBoxField.cs
- DataGridItemEventArgs.cs
- PageStatePersister.cs
- DocumentGrid.cs
- RelationshipConverter.cs
- ResetableIterator.cs
- DynamicPropertyHolder.cs
- RunInstallerAttribute.cs
- SerializationException.cs
- GetImportFileNameRequest.cs
- BooleanToVisibilityConverter.cs
- HostExecutionContextManager.cs
- Part.cs
- ButtonField.cs
- AuthorizationRuleCollection.cs
- TabletCollection.cs
- ComponentEditorForm.cs
- GPRECT.cs
- SmiSettersStream.cs
- HScrollBar.cs
- GeneratedView.cs
- ContentTypeSettingClientMessageFormatter.cs
- ReliableDuplexSessionChannel.cs
- DashStyle.cs
- Visual3DCollection.cs
- WeakReference.cs
- RedirectionProxy.cs
- Mapping.cs
- WebSysDescriptionAttribute.cs
- LinqDataSourceStatusEventArgs.cs
- ProcessActivityTreeOptions.cs
- HttpPostClientProtocol.cs
- Literal.cs
- DiscreteKeyFrames.cs
- DataGridViewColumnEventArgs.cs
- NavigationProperty.cs
- ColorConverter.cs
- WindowProviderWrapper.cs
- EnvelopedPkcs7.cs
- BaseResourcesBuildProvider.cs
- ComboBoxAutomationPeer.cs
- LinkTarget.cs
- ChainOfDependencies.cs
- WpfSharedXamlSchemaContext.cs
- SqlPersistenceProviderFactory.cs
- ObjectStateFormatter.cs
- Stylesheet.cs
- IPGlobalProperties.cs
- PointHitTestParameters.cs
- WebScriptServiceHost.cs
- SqlDataSourceView.cs
- FontUnit.cs
- OutputCacheProviderCollection.cs
- AssociativeAggregationOperator.cs
- NativeObjectSecurity.cs
- SkinBuilder.cs
- AttributeExtensions.cs
- DocumentAutomationPeer.cs
- BamlResourceSerializer.cs
- KeyValueSerializer.cs
- ConstructorBuilder.cs
- BindingList.cs
- ScriptingProfileServiceSection.cs
- ValueChangedEventManager.cs
- ContextMenu.cs
- PageSettings.cs
- LogicalExpr.cs
- DateTimeValueSerializerContext.cs
- DataGridViewSelectedRowCollection.cs
- GridItemProviderWrapper.cs
- BehaviorEditorPart.cs
- XmlAutoDetectWriter.cs
- InlineCollection.cs
- ObjectDataSourceSelectingEventArgs.cs
- BinaryParser.cs
- WinFormsUtils.cs
- TraceProvider.cs
- DataBoundControlAdapter.cs
- RemoteWebConfigurationHostServer.cs
- ArrayWithOffset.cs
- WbmpConverter.cs
- PackageStore.cs
- MapPathBasedVirtualPathProvider.cs
- RowToParametersTransformer.cs
- LazyInitializer.cs
- DiscoveryEndpointValidator.cs
- TableLayoutStyle.cs
- LinearGradientBrush.cs
- Menu.cs