Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Automation / Peers / DocumentAutomationPeer.cs / 1 / DocumentAutomationPeer.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: DocumentAutomationPeer.cs // // Description: AutomationPeer associated with flow and fixed documents. // //--------------------------------------------------------------------------- using System.Collections.Generic; // Listusing System.Security; // SecurityCritical using System.Windows.Documents; // ITextContainer using System.Windows.Interop; // HwndSource using System.Windows.Media; // Visual using MS.Internal; // PointUtil using MS.Internal.Automation; // TextAdaptor using MS.Internal.Documents; // TextContainerHelper namespace System.Windows.Automation.Peers { /// /// AutomationPeer associated with flow and fixed documents. /// public class DocumentAutomationPeer : ContentTextAutomationPeer { ////// Constructor. /// /// Owner of the AutomationPeer. public DocumentAutomationPeer(FrameworkContentElement owner) : base(owner) { } ////// Notify the peer that it has been disconnected. /// internal void OnDisconnected() { if (_textPattern != null) { _textPattern.Dispose(); _textPattern = null; } } ////// ////// /// Since DocumentAutomationPeer gives access to its content through /// TextPattern, this method always returns null. /// protected override ListGetChildrenCore() { if (_childrenStart != null && _childrenEnd != null) { ITextContainer textContainer = ((IServiceProvider)Owner).GetService(typeof(ITextContainer)) as ITextContainer; return TextContainerHelper.GetAutomationPeersFromRange(_childrenStart, _childrenEnd, textContainer.Start); } return null; } /// /// public override object GetPattern(PatternInterface patternInterface) { object returnValue = null; if (patternInterface == PatternInterface.Text) { if (_textPattern == null) { if (Owner is IServiceProvider) { ITextContainer textContainer = ((IServiceProvider)Owner).GetService(typeof(ITextContainer)) as ITextContainer; if (textContainer != null) { _textPattern = new TextAdaptor(this, textContainer); } } } returnValue = _textPattern; } return returnValue; } ////// /// protected override AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Document; } ////// /// ////// protected override string GetClassNameCore() { return "Document"; } /// /// protected override bool IsControlElementCore() { return true; } ////// /// ////// /// Critical - Calls PresentationSource.CriticalFromVisual to get the source for this visual /// TreatAsSafe - The returned PresenationSource object is not exposed and is only used for converting /// co-ordinates to screen space. /// [SecurityCritical, SecurityTreatAsSafe] protected override Rect GetBoundingRectangleCore() { UIElement uiScope; Rect boundingRect = CalculateBoundingRect(false, out uiScope); if (boundingRect != Rect.Empty && uiScope != null) { HwndSource hwndSource = PresentationSource.CriticalFromVisual(uiScope) as HwndSource; if (hwndSource != null) { boundingRect = PointUtil.ElementToRoot(boundingRect, uiScope, hwndSource); boundingRect = PointUtil.RootToClient(boundingRect, hwndSource); boundingRect = PointUtil.ClientToScreen(boundingRect, hwndSource); } } return boundingRect; } ////// ////// /// Critical - Calls PresentationSource.CriticalFromVisual to get the source for this visual /// TreatAsSafe - The returned PresenationSource object is not exposed and is only used for converting /// co-ordinates to screen space. /// [SecurityCritical, SecurityTreatAsSafe] protected override Point GetClickablePointCore() { Point point = new Point(); UIElement uiScope; Rect boundingRect = CalculateBoundingRect(true, out uiScope); if (boundingRect != Rect.Empty && uiScope != null) { HwndSource hwndSource = PresentationSource.CriticalFromVisual(uiScope) as HwndSource; if (hwndSource != null) { boundingRect = PointUtil.ElementToRoot(boundingRect, uiScope, hwndSource); boundingRect = PointUtil.RootToClient(boundingRect, hwndSource); boundingRect = PointUtil.ClientToScreen(boundingRect, hwndSource); point = new Point(boundingRect.Left + boundingRect.Width * 0.1, boundingRect.Top + boundingRect.Height * 0.1); } } return point; } ////// protected override bool IsOffscreenCore() { UIElement uiScope; Rect boundingRect = CalculateBoundingRect(true, out uiScope); return (boundingRect == Rect.Empty || uiScope == null); } ////// /// Gets collection of AutomationPeers for given text range. /// internal override ListGetAutomationPeersFromRange(ITextPointer start, ITextPointer end) { _childrenStart = start.CreatePointer(); _childrenEnd = end.CreatePointer(); ResetChildrenCache(); return GetChildren(); } /// /// Calculate visible rectangle. /// private Rect CalculateBoundingRect(bool clipToVisible, out UIElement uiScope) { uiScope = null; Rect boundingRect = Rect.Empty; if (Owner is IServiceProvider) { ITextContainer textContainer = ((IServiceProvider)Owner).GetService(typeof(ITextContainer)) as ITextContainer; ITextView textView = (textContainer != null) ? textContainer.TextView : null; if (textView != null) { // Make sure TextView is updated if (!textView.IsValid) { if (!textView.Validate()) { textView = null; } if (textView != null && !textView.IsValid) { textView = null; } } // Get bounding rect from TextView. if (textView != null) { boundingRect = new Rect(textView.RenderScope.RenderSize); uiScope = textView.RenderScope; // Compute visible portion of the rectangle. if (clipToVisible) { Visual visual = textView.RenderScope; while (visual != null && boundingRect != Rect.Empty) { if (VisualTreeHelper.GetClip(visual) != null) { GeneralTransform transform = textView.RenderScope.TransformToAncestor(visual).Inverse; // Safer version of transform to descendent (doing the inverse ourself), // we want the rect inside of our space. (Which is always rectangular and much nicer to work with) if (transform != null) { Rect clipBounds = VisualTreeHelper.GetClip(visual).Bounds; clipBounds = transform.TransformBounds(clipBounds); boundingRect.Intersect(clipBounds); } else { // No visibility if non-invertable transform exists. boundingRect = Rect.Empty; } } visual = VisualTreeHelper.GetParent(visual) as Visual; } } } } } return boundingRect; } private ITextPointer _childrenStart; private ITextPointer _childrenEnd; private TextAdaptor _textPattern; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: DocumentAutomationPeer.cs // // Description: AutomationPeer associated with flow and fixed documents. // //--------------------------------------------------------------------------- using System.Collections.Generic; // Listusing System.Security; // SecurityCritical using System.Windows.Documents; // ITextContainer using System.Windows.Interop; // HwndSource using System.Windows.Media; // Visual using MS.Internal; // PointUtil using MS.Internal.Automation; // TextAdaptor using MS.Internal.Documents; // TextContainerHelper namespace System.Windows.Automation.Peers { /// /// AutomationPeer associated with flow and fixed documents. /// public class DocumentAutomationPeer : ContentTextAutomationPeer { ////// Constructor. /// /// Owner of the AutomationPeer. public DocumentAutomationPeer(FrameworkContentElement owner) : base(owner) { } ////// Notify the peer that it has been disconnected. /// internal void OnDisconnected() { if (_textPattern != null) { _textPattern.Dispose(); _textPattern = null; } } ////// ////// /// Since DocumentAutomationPeer gives access to its content through /// TextPattern, this method always returns null. /// protected override ListGetChildrenCore() { if (_childrenStart != null && _childrenEnd != null) { ITextContainer textContainer = ((IServiceProvider)Owner).GetService(typeof(ITextContainer)) as ITextContainer; return TextContainerHelper.GetAutomationPeersFromRange(_childrenStart, _childrenEnd, textContainer.Start); } return null; } /// /// public override object GetPattern(PatternInterface patternInterface) { object returnValue = null; if (patternInterface == PatternInterface.Text) { if (_textPattern == null) { if (Owner is IServiceProvider) { ITextContainer textContainer = ((IServiceProvider)Owner).GetService(typeof(ITextContainer)) as ITextContainer; if (textContainer != null) { _textPattern = new TextAdaptor(this, textContainer); } } } returnValue = _textPattern; } return returnValue; } ////// /// protected override AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Document; } ////// /// ////// protected override string GetClassNameCore() { return "Document"; } /// /// protected override bool IsControlElementCore() { return true; } ////// /// ////// /// Critical - Calls PresentationSource.CriticalFromVisual to get the source for this visual /// TreatAsSafe - The returned PresenationSource object is not exposed and is only used for converting /// co-ordinates to screen space. /// [SecurityCritical, SecurityTreatAsSafe] protected override Rect GetBoundingRectangleCore() { UIElement uiScope; Rect boundingRect = CalculateBoundingRect(false, out uiScope); if (boundingRect != Rect.Empty && uiScope != null) { HwndSource hwndSource = PresentationSource.CriticalFromVisual(uiScope) as HwndSource; if (hwndSource != null) { boundingRect = PointUtil.ElementToRoot(boundingRect, uiScope, hwndSource); boundingRect = PointUtil.RootToClient(boundingRect, hwndSource); boundingRect = PointUtil.ClientToScreen(boundingRect, hwndSource); } } return boundingRect; } ////// ////// /// Critical - Calls PresentationSource.CriticalFromVisual to get the source for this visual /// TreatAsSafe - The returned PresenationSource object is not exposed and is only used for converting /// co-ordinates to screen space. /// [SecurityCritical, SecurityTreatAsSafe] protected override Point GetClickablePointCore() { Point point = new Point(); UIElement uiScope; Rect boundingRect = CalculateBoundingRect(true, out uiScope); if (boundingRect != Rect.Empty && uiScope != null) { HwndSource hwndSource = PresentationSource.CriticalFromVisual(uiScope) as HwndSource; if (hwndSource != null) { boundingRect = PointUtil.ElementToRoot(boundingRect, uiScope, hwndSource); boundingRect = PointUtil.RootToClient(boundingRect, hwndSource); boundingRect = PointUtil.ClientToScreen(boundingRect, hwndSource); point = new Point(boundingRect.Left + boundingRect.Width * 0.1, boundingRect.Top + boundingRect.Height * 0.1); } } return point; } ////// protected override bool IsOffscreenCore() { UIElement uiScope; Rect boundingRect = CalculateBoundingRect(true, out uiScope); return (boundingRect == Rect.Empty || uiScope == null); } ////// /// Gets collection of AutomationPeers for given text range. /// internal override ListGetAutomationPeersFromRange(ITextPointer start, ITextPointer end) { _childrenStart = start.CreatePointer(); _childrenEnd = end.CreatePointer(); ResetChildrenCache(); return GetChildren(); } /// /// Calculate visible rectangle. /// private Rect CalculateBoundingRect(bool clipToVisible, out UIElement uiScope) { uiScope = null; Rect boundingRect = Rect.Empty; if (Owner is IServiceProvider) { ITextContainer textContainer = ((IServiceProvider)Owner).GetService(typeof(ITextContainer)) as ITextContainer; ITextView textView = (textContainer != null) ? textContainer.TextView : null; if (textView != null) { // Make sure TextView is updated if (!textView.IsValid) { if (!textView.Validate()) { textView = null; } if (textView != null && !textView.IsValid) { textView = null; } } // Get bounding rect from TextView. if (textView != null) { boundingRect = new Rect(textView.RenderScope.RenderSize); uiScope = textView.RenderScope; // Compute visible portion of the rectangle. if (clipToVisible) { Visual visual = textView.RenderScope; while (visual != null && boundingRect != Rect.Empty) { if (VisualTreeHelper.GetClip(visual) != null) { GeneralTransform transform = textView.RenderScope.TransformToAncestor(visual).Inverse; // Safer version of transform to descendent (doing the inverse ourself), // we want the rect inside of our space. (Which is always rectangular and much nicer to work with) if (transform != null) { Rect clipBounds = VisualTreeHelper.GetClip(visual).Bounds; clipBounds = transform.TransformBounds(clipBounds); boundingRect.Intersect(clipBounds); } else { // No visibility if non-invertable transform exists. boundingRect = Rect.Empty; } } visual = VisualTreeHelper.GetParent(visual) as Visual; } } } } } return boundingRect; } private ITextPointer _childrenStart; private ITextPointer _childrenEnd; private TextAdaptor _textPattern; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
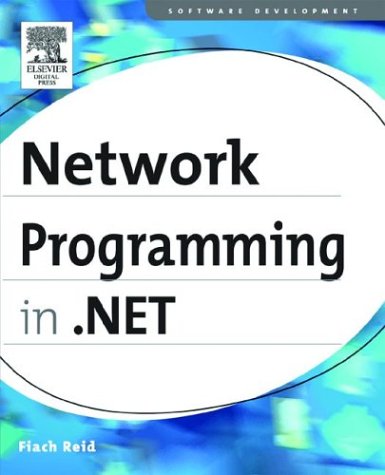
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RequestBringIntoViewEventArgs.cs
- Options.cs
- DependencyPropertyAttribute.cs
- FileVersionInfo.cs
- SHA384Managed.cs
- PhonemeConverter.cs
- TreeViewEvent.cs
- OdbcConnectionStringbuilder.cs
- BitmapImage.cs
- ConfigurationManagerHelper.cs
- IxmlLineInfo.cs
- HMACSHA384.cs
- BasicKeyConstraint.cs
- MenuStrip.cs
- MenuItemBindingCollection.cs
- CompilerGeneratedAttribute.cs
- ReliableChannelBinder.cs
- SqlInfoMessageEvent.cs
- AssociationSetMetadata.cs
- UnitySerializationHolder.cs
- DeviceContext2.cs
- DataViewSettingCollection.cs
- SingleTagSectionHandler.cs
- SecurityManager.cs
- DeleteStoreRequest.cs
- ToolStripLocationCancelEventArgs.cs
- MenuItemBindingCollection.cs
- documentation.cs
- GlyphInfoList.cs
- AdRotator.cs
- Executor.cs
- DataColumnChangeEvent.cs
- BaseCodeDomTreeGenerator.cs
- SortQueryOperator.cs
- PropertyGridView.cs
- Crc32.cs
- safex509handles.cs
- HttpSysSettings.cs
- UIElement3D.cs
- SmtpClient.cs
- MediaSystem.cs
- StorageMappingItemLoader.cs
- RenderCapability.cs
- DeploymentSection.cs
- ParameterReplacerVisitor.cs
- DockPanel.cs
- SafeBitVector32.cs
- StrokeCollectionConverter.cs
- XamlStream.cs
- NavigatorOutput.cs
- JournalEntryListConverter.cs
- HtmlElement.cs
- DefaultExpressionVisitor.cs
- MexTcpBindingElement.cs
- LinkDescriptor.cs
- MailSettingsSection.cs
- DataGridViewButtonCell.cs
- Parser.cs
- WindowsGraphics2.cs
- XmlSchemaGroup.cs
- ParseHttpDate.cs
- oledbmetadatacolumnnames.cs
- MemberInfoSerializationHolder.cs
- MsmqIntegrationMessagePool.cs
- CompositeFontFamily.cs
- SessionStateSection.cs
- PlatformNotSupportedException.cs
- DataGridTemplateColumn.cs
- ReturnType.cs
- dataobject.cs
- DynamicDiscoSearcher.cs
- Point3DKeyFrameCollection.cs
- ProfileProvider.cs
- InvokePatternIdentifiers.cs
- TracePayload.cs
- UnescapedXmlDiagnosticData.cs
- SqlTransaction.cs
- FormsAuthenticationModule.cs
- NumericUpDownAcceleration.cs
- AutomationElementIdentifiers.cs
- ButtonBase.cs
- MetafileHeader.cs
- ResponseStream.cs
- ConfigurationSchemaErrors.cs
- ServiceObjectContainer.cs
- EmbeddedMailObjectsCollection.cs
- XmlCharCheckingReader.cs
- XsdDuration.cs
- WeakReferenceKey.cs
- TextRenderingModeValidation.cs
- SimpleLine.cs
- AnnotationStore.cs
- XmlnsCompatibleWithAttribute.cs
- SynchronousSendBindingElement.cs
- SocketPermission.cs
- MarkupExtensionReturnTypeAttribute.cs
- HtmlTableCell.cs
- PersistChildrenAttribute.cs
- DispatcherTimer.cs
- FixedSOMTextRun.cs