Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / Security / Cryptography / TripleDES.cs / 1 / TripleDES.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // TripleDES.cs // namespace System.Security.Cryptography { [System.Runtime.InteropServices.ComVisible(true)] public abstract class TripleDES : SymmetricAlgorithm { private static KeySizes[] s_legalBlockSizes = { new KeySizes(64, 64, 0) }; private static KeySizes[] s_legalKeySizes = { new KeySizes(2*64, 3*64, 64) }; // // protected constructors // protected TripleDES() { KeySizeValue = 3*64; BlockSizeValue = 64; FeedbackSizeValue = BlockSizeValue; LegalBlockSizesValue = s_legalBlockSizes; LegalKeySizesValue = s_legalKeySizes; } // // public properties // public override byte[] Key { get { if (KeyValue == null) { // Never hand back a weak key do { GenerateKey(); } while (IsWeakKey(KeyValue)); } return (byte[]) KeyValue.Clone(); } set { if (value == null) throw new ArgumentNullException("value"); if (!ValidKeySize(value.Length * 8)) { // must convert bytes to bits throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidKeySize")); } if (IsWeakKey(value)) { throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidKey_Weak"),"TripleDES"); } KeyValue = (byte[]) value.Clone(); KeySizeValue = value.Length * 8; } } // // public methods // new static public TripleDES Create() { return Create("System.Security.Cryptography.TripleDES"); } new static public TripleDES Create(String str) { return (TripleDES) CryptoConfig.CreateFromName(str); } public static bool IsWeakKey(byte[] rgbKey) { // All we have to check for here is (a) we're in 3-key mode (192 bits), and // (b) either K1 == K2 or K2 == K3 if (!IsLegalKeySize(rgbKey)) { throw new CryptographicException(Environment.GetResourceString("Cryptography_InvalidKeySize")); } byte[] rgbOddParityKey = Utils.FixupKeyParity(rgbKey); if (EqualBytes(rgbOddParityKey,0,8,8)) return(true); if ((rgbOddParityKey.Length == 24) && EqualBytes(rgbOddParityKey,8,16,8)) return(true); return(false); } // // private methods // private static bool EqualBytes(byte[] rgbKey, int start1, int start2, int count) { if (start1 < 0) throw new ArgumentOutOfRangeException("start1", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (start2 < 0) throw new ArgumentOutOfRangeException("start2", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if ((start1+count) > rgbKey.Length) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidValue")); if ((start2+count) > rgbKey.Length) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidValue")); for (int i = 0; i < count; i++) { if (rgbKey[start1+i] != rgbKey[start2+i]) return(false); } return(true); } private static bool IsLegalKeySize(byte[] rgbKey) { if (rgbKey != null && ((rgbKey.Length == 16) || (rgbKey.Length == 24))) return(true); return(false); } } }
Link Menu
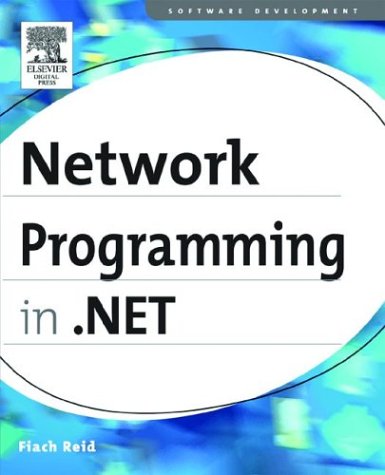
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ActivityBindForm.cs
- Effect.cs
- SiteMapHierarchicalDataSourceView.cs
- SqlCommandBuilder.cs
- BindingExpression.cs
- ProfileSection.cs
- SiteIdentityPermission.cs
- Convert.cs
- SignedXmlDebugLog.cs
- ElementMarkupObject.cs
- GridViewCancelEditEventArgs.cs
- OdbcCommandBuilder.cs
- DocumentXPathNavigator.cs
- TemplateField.cs
- DocumentSequenceHighlightLayer.cs
- VerbConverter.cs
- CaretElement.cs
- EntityDataSourceConfigureObjectContextPanel.cs
- EventLogConfiguration.cs
- AccessibilityHelperForVista.cs
- ZipIOExtraFieldElement.cs
- OracleDateTime.cs
- BigInt.cs
- Atom10FeedFormatter.cs
- VideoDrawing.cs
- DataGridSortCommandEventArgs.cs
- ColumnMapProcessor.cs
- TemplateBindingExpressionConverter.cs
- ModelUIElement3D.cs
- SqlReferenceCollection.cs
- ToolStripActionList.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- DeferredSelectedIndexReference.cs
- NavigationCommands.cs
- TransformPattern.cs
- ToolStripSystemRenderer.cs
- RuntimeConfig.cs
- MutexSecurity.cs
- ImpersonateTokenRef.cs
- SafeMarshalContext.cs
- CacheMemory.cs
- SystemSounds.cs
- DBDataPermissionAttribute.cs
- BaseParaClient.cs
- ReachBasicContext.cs
- FontFamily.cs
- ContentPlaceHolder.cs
- DockPatternIdentifiers.cs
- TransactionFlowBindingElementImporter.cs
- DataTableReader.cs
- AsyncStreamReader.cs
- HScrollProperties.cs
- AvTraceFormat.cs
- XmlHierarchicalDataSourceView.cs
- Icon.cs
- HistoryEventArgs.cs
- AttachedAnnotationChangedEventArgs.cs
- Image.cs
- SchemaElementDecl.cs
- ExtensionSurface.cs
- Compiler.cs
- ListBox.cs
- VirtualPathUtility.cs
- IconHelper.cs
- FunctionNode.cs
- SafeEventLogReadHandle.cs
- FtpRequestCacheValidator.cs
- Stacktrace.cs
- TypeTypeConverter.cs
- _DisconnectOverlappedAsyncResult.cs
- SessionStateSection.cs
- GridViewUpdatedEventArgs.cs
- DocumentViewerHelper.cs
- DesignTimeXamlWriter.cs
- HttpConfigurationContext.cs
- NavigationWindow.cs
- MemberPathMap.cs
- smtpconnection.cs
- UntypedNullExpression.cs
- DefaultValueConverter.cs
- QilStrConcatenator.cs
- TextMarkerSource.cs
- securitymgrsite.cs
- SmiMetaData.cs
- AdPostCacheSubstitution.cs
- RegexCapture.cs
- SchemaTypeEmitter.cs
- MemoryMappedViewStream.cs
- DefaultValueAttribute.cs
- HttpCacheVaryByContentEncodings.cs
- OleAutBinder.cs
- XmlTextEncoder.cs
- Camera.cs
- RightsManagementPermission.cs
- WebBrowserUriTypeConverter.cs
- DbParameterCollection.cs
- SettingsPropertyCollection.cs
- Brush.cs
- WorkflowWebService.cs
- ApplicationSettingsBase.cs