Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Regex / System / Text / RegularExpressions / RegexCapture.cs / 1305376 / RegexCapture.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- // Capture is just a location/length pair that indicates the // location of a regular expression match. A single regexp // search may return multiple Capture within each capturing // RegexGroup. namespace System.Text.RegularExpressions { ////// #if !SILVERLIGHT [ Serializable() ] #endif public class Capture { internal String _text; internal int _index; internal int _length; internal Capture(String text, int i, int l) { _text = text; _index = i; _length = l; } /* * The index of the beginning of the matched capture */ ////// Represents the results from a single subexpression capture. The object represents /// one substring for a single successful capture. ////// public int Index { get { return _index; } } /* * The length of the matched capture */ ///Returns the position in the original string where the first character of /// captured substring was found. ////// public int Length { get { return _length; } } ////// Returns the length of the captured substring. /// ////// public string Value { get { return _text.Substring(_index, _length); } } /* * The capture as a string */ ///[To be supplied.] ////// override public String ToString() { return Value; } /* * The original string */ internal String GetOriginalString() { return _text; } /* * The substring to the left of the capture */ internal String GetLeftSubstring() { return _text.Substring(0, _index); } /* * The substring to the right of the capture */ internal String GetRightSubstring() { return _text.Substring(_index + _length, _text.Length - _index - _length); } #if DBG internal virtual String Description() { StringBuilder Sb = new StringBuilder(); Sb.Append("(I = "); Sb.Append(_index); Sb.Append(", L = "); Sb.Append(_length); Sb.Append("): "); Sb.Append(_text, _index, _length); return Sb.ToString(); } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Returns /// the substring that was matched. /// ///
Link Menu
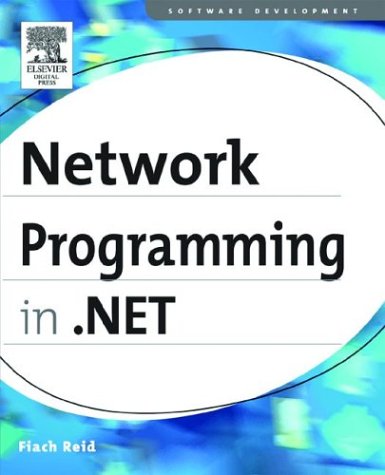
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SplitterPanelDesigner.cs
- Matrix3DConverter.cs
- WebConfigurationHost.cs
- datacache.cs
- XmlName.cs
- Stylus.cs
- BinaryObjectReader.cs
- EdmPropertyAttribute.cs
- TableItemPattern.cs
- ResourcePermissionBaseEntry.cs
- RadioButtonRenderer.cs
- VideoDrawing.cs
- GlyphsSerializer.cs
- GeometryDrawing.cs
- PhysicalOps.cs
- DurationConverter.cs
- ComAwareEventInfo.cs
- ObjectTokenCategory.cs
- CheckBoxPopupAdapter.cs
- ToolStripCustomTypeDescriptor.cs
- DataTableClearEvent.cs
- TextBlock.cs
- ToolBarOverflowPanel.cs
- DataBoundControlAdapter.cs
- QilScopedVisitor.cs
- ApplyImportsAction.cs
- LabelAutomationPeer.cs
- FlowDocumentReader.cs
- MonitorWrapper.cs
- DesignerActionUIStateChangeEventArgs.cs
- ModuleConfigurationInfo.cs
- SHA1.cs
- ImmutableCommunicationTimeouts.cs
- PrimarySelectionAdorner.cs
- LogFlushAsyncResult.cs
- X509ChainElement.cs
- MemoryMappedViewAccessor.cs
- CatalogZone.cs
- ConfigurationSectionHelper.cs
- SetIndexBinder.cs
- DropDownList.cs
- FeatureSupport.cs
- DeferredReference.cs
- CommandID.cs
- Formatter.cs
- BitmapFrameEncode.cs
- GeometryDrawing.cs
- InterleavedZipPartStream.cs
- DrawingVisualDrawingContext.cs
- TypeSystem.cs
- PrinterSettings.cs
- LocalBuilder.cs
- DirectionalLight.cs
- RegexBoyerMoore.cs
- DataSourceConverter.cs
- FontNamesConverter.cs
- ControlCachePolicy.cs
- GridViewPageEventArgs.cs
- MeasureData.cs
- MaskedTextProvider.cs
- StringOutput.cs
- SynchronousReceiveElement.cs
- DataPagerCommandEventArgs.cs
- ThreadSafeList.cs
- OdbcStatementHandle.cs
- Rotation3D.cs
- DataServiceContext.cs
- StringArrayConverter.cs
- LexicalChunk.cs
- SQLDateTime.cs
- SqlReferenceCollection.cs
- XpsS0ValidatingLoader.cs
- PersonalizableAttribute.cs
- MaterialCollection.cs
- AggregateNode.cs
- CorePropertiesFilter.cs
- DataSourceSelectArguments.cs
- Animatable.cs
- IgnoreFileBuildProvider.cs
- InstanceDescriptor.cs
- GridPattern.cs
- MessageBodyMemberAttribute.cs
- CollectionContainer.cs
- nulltextnavigator.cs
- ChannelParameterCollection.cs
- WindowsListViewItemStartMenu.cs
- DataSourceView.cs
- TransformedBitmap.cs
- HtmlInputControl.cs
- PipeStream.cs
- DataGridViewSelectedCellCollection.cs
- DataViewSettingCollection.cs
- XmlSchemaExporter.cs
- OdbcUtils.cs
- ViewgenGatekeeper.cs
- DescendantQuery.cs
- SafeProcessHandle.cs
- Color.cs
- MethodExpr.cs
- StrongNameKeyPair.cs