Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / DurationConverter.cs / 1305600 / DurationConverter.cs
//------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2004 // // File: DurationConverter.cs //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security; namespace System.Windows { ////// Provides a type converter to convert Duration to and from other representations. /// public class DurationConverter : TypeConverter { ////// CanConvertFrom - Returns whether or not this class can convert from a given type /// ///public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } /// /// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible ///public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if ( destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } /// /// ConvertFrom /// ///public override object ConvertFrom( ITypeDescriptorContext td, CultureInfo cultureInfo, object value) { string stringValue = value as string; // Override the converter for sentinel values if (stringValue != null) { stringValue = stringValue.Trim(); if (stringValue == "Automatic") { return Duration.Automatic; } else if (stringValue == "Forever") { return Duration.Forever; } } TimeSpan duration = TimeSpan.Zero; if(_timeSpanConverter == null) { _timeSpanConverter = new TimeSpanConverter(); } duration = (TimeSpan)_timeSpanConverter.ConvertFrom(td, cultureInfo, value); return new Duration(duration); } /// /// TypeConverter method implementation. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// /// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for Duration, not an arbitrary class /// [SecurityCritical] public override object ConvertTo( ITypeDescriptorContext context, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType != null && value is Duration) { Duration durationValue = (Duration)value; if (destinationType == typeof(InstanceDescriptor)) { MemberInfo mi; if (durationValue.HasTimeSpan) { mi = typeof(Duration).GetConstructor(new Type[] { typeof(TimeSpan) }); return new InstanceDescriptor(mi, new object[] { durationValue.TimeSpan }); } else if (durationValue == Duration.Forever) { mi = typeof(Duration).GetProperty("Forever"); return new InstanceDescriptor(mi, null); } else { Debug.Assert(durationValue == Duration.Automatic); // Only other legal duration type mi = typeof(Duration).GetProperty("Automatic"); return new InstanceDescriptor(mi, null); } } else if (destinationType == typeof(string)) { return durationValue.ToString(); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, cultureInfo, value, destinationType); } private static TimeSpanConverter _timeSpanConverter; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2004 // // File: DurationConverter.cs //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security; namespace System.Windows { ////// Provides a type converter to convert Duration to and from other representations. /// public class DurationConverter : TypeConverter { ////// CanConvertFrom - Returns whether or not this class can convert from a given type /// ///public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } /// /// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible ///public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if ( destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } /// /// ConvertFrom /// ///public override object ConvertFrom( ITypeDescriptorContext td, CultureInfo cultureInfo, object value) { string stringValue = value as string; // Override the converter for sentinel values if (stringValue != null) { stringValue = stringValue.Trim(); if (stringValue == "Automatic") { return Duration.Automatic; } else if (stringValue == "Forever") { return Duration.Forever; } } TimeSpan duration = TimeSpan.Zero; if(_timeSpanConverter == null) { _timeSpanConverter = new TimeSpanConverter(); } duration = (TimeSpan)_timeSpanConverter.ConvertFrom(td, cultureInfo, value); return new Duration(duration); } /// /// TypeConverter method implementation. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// /// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for Duration, not an arbitrary class /// [SecurityCritical] public override object ConvertTo( ITypeDescriptorContext context, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType != null && value is Duration) { Duration durationValue = (Duration)value; if (destinationType == typeof(InstanceDescriptor)) { MemberInfo mi; if (durationValue.HasTimeSpan) { mi = typeof(Duration).GetConstructor(new Type[] { typeof(TimeSpan) }); return new InstanceDescriptor(mi, new object[] { durationValue.TimeSpan }); } else if (durationValue == Duration.Forever) { mi = typeof(Duration).GetProperty("Forever"); return new InstanceDescriptor(mi, null); } else { Debug.Assert(durationValue == Duration.Automatic); // Only other legal duration type mi = typeof(Duration).GetProperty("Automatic"); return new InstanceDescriptor(mi, null); } } else if (destinationType == typeof(string)) { return durationValue.ToString(); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, cultureInfo, value, destinationType); } private static TimeSpanConverter _timeSpanConverter; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
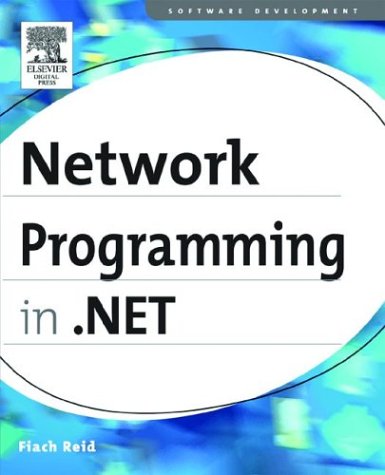
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PermissionSet.cs
- StatusBarDesigner.cs
- Attributes.cs
- EntityDataSourceUtil.cs
- NetStream.cs
- Convert.cs
- UrlPath.cs
- WindowsImpersonationContext.cs
- XPathConvert.cs
- WriteableOnDemandStream.cs
- AbstractSvcMapFileLoader.cs
- ReferenceConverter.cs
- BypassElement.cs
- WeakReference.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- SspiHelper.cs
- Delegate.cs
- SpellerHighlightLayer.cs
- CommonObjectSecurity.cs
- TypeToken.cs
- LeftCellWrapper.cs
- _ListenerRequestStream.cs
- TextRunCache.cs
- FlagsAttribute.cs
- EventLogInternal.cs
- CompilerCollection.cs
- TemplatePropertyEntry.cs
- BuildProviderCollection.cs
- ClientProtocol.cs
- ImageCollectionEditor.cs
- RtfNavigator.cs
- SmiRequestExecutor.cs
- HtmlShimManager.cs
- SEHException.cs
- LogSwitch.cs
- RuntimeConfig.cs
- WriteableBitmap.cs
- ObjectDataSource.cs
- EventEntry.cs
- JpegBitmapEncoder.cs
- RequestBringIntoViewEventArgs.cs
- HostedBindingBehavior.cs
- OutputCacheProviderCollection.cs
- PasswordValidationException.cs
- Propagator.JoinPropagator.cs
- SingleConverter.cs
- EntityDataSourceEntityTypeFilterConverter.cs
- BitmapEffectvisualstate.cs
- GetReadStreamResult.cs
- AsyncCompletedEventArgs.cs
- XmlSchemas.cs
- IndentTextWriter.cs
- DataObjectAttribute.cs
- SecurityTokenProvider.cs
- ASCIIEncoding.cs
- TagNameToTypeMapper.cs
- LayoutEngine.cs
- TextRangeAdaptor.cs
- ProfileSection.cs
- ClientApiGenerator.cs
- ServiceRouteHandler.cs
- HttpRequest.cs
- XPathAxisIterator.cs
- thaishape.cs
- FreezableDefaultValueFactory.cs
- CollectionViewGroupRoot.cs
- ZoneLinkButton.cs
- CapabilitiesUse.cs
- TrustLevelCollection.cs
- ListenerElementsCollection.cs
- PlacementWorkspace.cs
- XmlDownloadManager.cs
- Rect3DConverter.cs
- DesignTimeValidationFeature.cs
- ManifestSignedXml.cs
- SQLInt64Storage.cs
- ChangesetResponse.cs
- LinearKeyFrames.cs
- PlanCompiler.cs
- Point4D.cs
- LayoutDump.cs
- ClientRuntimeConfig.cs
- HwndMouseInputProvider.cs
- ManipulationVelocities.cs
- Types.cs
- AnnouncementEventArgs.cs
- ClientUIRequest.cs
- COM2TypeInfoProcessor.cs
- PngBitmapDecoder.cs
- CatalogPartDesigner.cs
- ForEachAction.cs
- DispatcherSynchronizationContext.cs
- MetadataWorkspace.cs
- SqlRewriteScalarSubqueries.cs
- ReflectEventDescriptor.cs
- WebServiceResponse.cs
- WpfPayload.cs
- X500Name.cs
- SliderAutomationPeer.cs
- ScriptManager.cs