Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Metadata / MetadataArtifactLoaderXmlReaderWrapper.cs / 1 / MetadataArtifactLoaderXmlReaderWrapper.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections; using System.Diagnostics; using System.Text; using System.Xml; using System.Security.Permissions; namespace System.Data.Metadata.Edm { ////// This class represents a wrapper around an XmlReader to be used to load metadata. /// Note that the XmlReader object isn't created here -- the wrapper simply stores /// a reference to it -- therefore we do not Close() the reader when we Dispose() /// the wrapper, i.e., Dispose() is a no-op. /// internal class MetadataArtifactLoaderXmlReaderWrapper : MetadataArtifactLoader, IComparable { private readonly XmlReader _reader = null; private readonly string _resourceUri = null; ////// Constructor - saves off the XmlReader in a private data field /// /// The path to the resource to load public MetadataArtifactLoaderXmlReaderWrapper(XmlReader xmlReader) { _reader = xmlReader; _resourceUri = xmlReader.BaseURI; } public override string Path { get { if (string.IsNullOrEmpty(this._resourceUri)) { return string.Empty; } else { return this._resourceUri; } } } ////// Implementation of IComparable.CompareTo() /// /// The object to compare to ///0 if the loaders are "equal" (i.e., have the same _path value) public int CompareTo(object obj) { MetadataArtifactLoaderXmlReaderWrapper loader = obj as MetadataArtifactLoaderXmlReaderWrapper; if (loader != null) { if (Object.ReferenceEquals(this._reader, loader._reader)) { return 0; } else { return -1; } } Debug.Assert(false, "object is not a MetadataArtifactLoaderXmlReaderWrapper"); return -1; } ////// Equals() returns true if the objects have the same _path value /// /// The object to compare to ///true if the objects have the same _path value public override bool Equals(object obj) { return this.CompareTo(obj) == 0; } ////// GetHashCode override that defers the result to the _path member variable. /// ///public override int GetHashCode() { return _reader.GetHashCode(); } public override void CollectFilePermissionPaths(List paths, DataSpace spaceToGet) { // no op } /// /// Get paths to artifacts for a specific DataSpace. /// /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetPaths(DataSpace spaceToGet) { List list = new List (); if (MetadataArtifactLoader.IsArtifactOfDataSpace(Path, spaceToGet)) { list.Add(Path); } return list; } /// /// Get paths to all artifacts /// ///A List of strings identifying paths to all resources public override ListGetPaths() { return new List (new string[] { Path }); } /// /// Get XmlReaders for all resources /// ///A List of XmlReaders for all resources public override ListGetReaders(Dictionary sourceDictionary) { List list = new List (); list.Add(this._reader); if (sourceDictionary != null) { sourceDictionary.Add(this, _reader); } return list; } /// /// Create and return an XmlReader around the resource represented by this instance /// if it is of the requested DataSpace type. /// /// The DataSpace corresponding to the requested artifacts ///A List of XmlReader objects public override ListCreateReaders(DataSpace spaceToGet) { List list = new List (); if (MetadataArtifactLoader.IsArtifactOfDataSpace(Path, spaceToGet)) { list.Add(_reader); } return list; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Collections; using System.Diagnostics; using System.Text; using System.Xml; using System.Security.Permissions; namespace System.Data.Metadata.Edm { ////// This class represents a wrapper around an XmlReader to be used to load metadata. /// Note that the XmlReader object isn't created here -- the wrapper simply stores /// a reference to it -- therefore we do not Close() the reader when we Dispose() /// the wrapper, i.e., Dispose() is a no-op. /// internal class MetadataArtifactLoaderXmlReaderWrapper : MetadataArtifactLoader, IComparable { private readonly XmlReader _reader = null; private readonly string _resourceUri = null; ////// Constructor - saves off the XmlReader in a private data field /// /// The path to the resource to load public MetadataArtifactLoaderXmlReaderWrapper(XmlReader xmlReader) { _reader = xmlReader; _resourceUri = xmlReader.BaseURI; } public override string Path { get { if (string.IsNullOrEmpty(this._resourceUri)) { return string.Empty; } else { return this._resourceUri; } } } ////// Implementation of IComparable.CompareTo() /// /// The object to compare to ///0 if the loaders are "equal" (i.e., have the same _path value) public int CompareTo(object obj) { MetadataArtifactLoaderXmlReaderWrapper loader = obj as MetadataArtifactLoaderXmlReaderWrapper; if (loader != null) { if (Object.ReferenceEquals(this._reader, loader._reader)) { return 0; } else { return -1; } } Debug.Assert(false, "object is not a MetadataArtifactLoaderXmlReaderWrapper"); return -1; } ////// Equals() returns true if the objects have the same _path value /// /// The object to compare to ///true if the objects have the same _path value public override bool Equals(object obj) { return this.CompareTo(obj) == 0; } ////// GetHashCode override that defers the result to the _path member variable. /// ///public override int GetHashCode() { return _reader.GetHashCode(); } public override void CollectFilePermissionPaths(List paths, DataSpace spaceToGet) { // no op } /// /// Get paths to artifacts for a specific DataSpace. /// /// The DataSpace for the artifacts of interest ///A List of strings identifying paths to all artifacts for a specific DataSpace public override ListGetPaths(DataSpace spaceToGet) { List list = new List (); if (MetadataArtifactLoader.IsArtifactOfDataSpace(Path, spaceToGet)) { list.Add(Path); } return list; } /// /// Get paths to all artifacts /// ///A List of strings identifying paths to all resources public override ListGetPaths() { return new List (new string[] { Path }); } /// /// Get XmlReaders for all resources /// ///A List of XmlReaders for all resources public override ListGetReaders(Dictionary sourceDictionary) { List list = new List (); list.Add(this._reader); if (sourceDictionary != null) { sourceDictionary.Add(this, _reader); } return list; } /// /// Create and return an XmlReader around the resource represented by this instance /// if it is of the requested DataSpace type. /// /// The DataSpace corresponding to the requested artifacts ///A List of XmlReader objects public override ListCreateReaders(DataSpace spaceToGet) { List list = new List (); if (MetadataArtifactLoader.IsArtifactOfDataSpace(Path, spaceToGet)) { list.Add(_reader); } return list; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
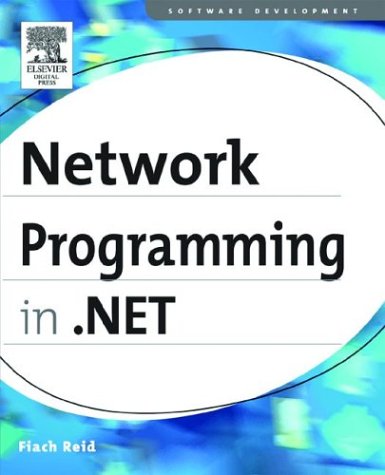
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AppDomainFactory.cs
- XmlAnyAttributeAttribute.cs
- WindowsToolbarItemAsMenuItem.cs
- BaseConfigurationRecord.cs
- TiffBitmapDecoder.cs
- __TransparentProxy.cs
- XmlSchemaRedefine.cs
- RectKeyFrameCollection.cs
- MethodBody.cs
- HandlerBase.cs
- TemplateInstanceAttribute.cs
- DictionaryBase.cs
- FreeFormDesigner.cs
- DynamicRenderer.cs
- Image.cs
- ConfigurationSection.cs
- DomNameTable.cs
- ObjectDataSourceStatusEventArgs.cs
- OdbcErrorCollection.cs
- ContainerUtilities.cs
- TdsParserStateObject.cs
- ApplicationTrust.cs
- BroadcastEventHelper.cs
- HttpResponseInternalWrapper.cs
- XmlnsPrefixAttribute.cs
- DataTrigger.cs
- LinkClickEvent.cs
- DoubleIndependentAnimationStorage.cs
- DataObjectAttribute.cs
- DynamicFilter.cs
- DateTimeUtil.cs
- TimeBoundedCache.cs
- FormViewInsertedEventArgs.cs
- InfoCardTrace.cs
- FileClassifier.cs
- SmtpDigestAuthenticationModule.cs
- BasicHttpSecurityMode.cs
- EntityConnectionStringBuilderItem.cs
- ManagementNamedValueCollection.cs
- FixedPosition.cs
- TypeFieldSchema.cs
- XmlConvert.cs
- AuthorizationRuleCollection.cs
- TextEditorDragDrop.cs
- LinkConverter.cs
- ADMembershipUser.cs
- StyleHelper.cs
- RepeaterItemEventArgs.cs
- AnnotationObservableCollection.cs
- QilPatternVisitor.cs
- TypeNameConverter.cs
- DataGridViewBindingCompleteEventArgs.cs
- WindowsListView.cs
- HttpCapabilitiesSectionHandler.cs
- TextChangedEventArgs.cs
- FastPropertyAccessor.cs
- SectionInformation.cs
- WaitHandle.cs
- RegexGroupCollection.cs
- TrackingWorkflowEventArgs.cs
- CorePropertiesFilter.cs
- ConnectionManagementElementCollection.cs
- ExtendedPropertyDescriptor.cs
- TextEditorLists.cs
- AnchoredBlock.cs
- _ListenerRequestStream.cs
- EntityDataSourceMemberPath.cs
- ServiceParser.cs
- XmlValidatingReader.cs
- WmlLabelAdapter.cs
- RSAPKCS1KeyExchangeFormatter.cs
- WhitespaceRuleReader.cs
- HtmlButton.cs
- ExpressionConverter.cs
- TemplateBindingExpressionConverter.cs
- ColumnCollection.cs
- SupportsEventValidationAttribute.cs
- Assembly.cs
- SocketElement.cs
- PathGeometry.cs
- DetailsView.cs
- HostProtectionException.cs
- ColorIndependentAnimationStorage.cs
- DataColumnCollection.cs
- DynamicPropertyReader.cs
- DataSetViewSchema.cs
- GroupDescription.cs
- QualifierSet.cs
- RowUpdatedEventArgs.cs
- ReadOnlyAttribute.cs
- SchemaHelper.cs
- AttributeQuery.cs
- XPathDocument.cs
- DispatcherHooks.cs
- CompModSwitches.cs
- MenuEventArgs.cs
- AssociationEndMember.cs
- StatusBarPanelClickEvent.cs
- TableRowGroupCollection.cs
- FacetValueContainer.cs