Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / System / Windows / Threading / DispatcherHooks.cs / 1 / DispatcherHooks.cs
using System.Security; using System.Security.Permissions; namespace System.Windows.Threading { ////// Additional information provided about a dispatcher. /// public sealed class DispatcherHooks { ////// An event indicating the the dispatcher has no more operations to process. /// ////// Note that this event will be raised by the dispatcher thread when /// there is no more pending work to do. /// /// Note also that this event could come before the last operation is /// invoked, because that is when we determine that the queue is empty. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _dispatcherInactive /// TreatAsSafe: link-demands /// public event EventHandler DispatcherInactive { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _dispatcherInactive += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _dispatcherInactive -= value; } } } ////// An event indicating that an operation was posted to the dispatcher. /// ////// Typically this is due to the BeginInvoke API, but the Invoke API can /// also cause this if any priority other than DispatcherPriority.Send is /// specified, or if the destination dispatcher is owned by a different /// thread. /// /// Note that any thread can post operations, so this event can be /// raised by any thread. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationPosted /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationPosted { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationPosted += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationPosted -= value; } } } ////// An event indicating that an operation was completed. /// ////// Note that this event will be raised by the dispatcher thread after /// the operation has completed. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationCompleted /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationCompleted { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationCompleted += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationCompleted -= value; } } } ////// An event indicating that the priority of an operation was changed. /// ////// Note that any thread can change the priority of operations, /// so this event can be raised by any thread. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationPriorityChanged /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationPriorityChanged { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationPriorityChanged += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationPriorityChanged -= value; } } } ////// An event indicating that an operation was aborted. /// ////// Note that any thread can abort an operation, so this event /// can be raised by any thread. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationAborted /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationAborted { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationAborted += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationAborted -= value; } } } // Only we can create these things. internal DispatcherHooks() { } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseDispatcherInactive(Dispatcher dispatcher) { EventHandler dispatcherInactive = _dispatcherInactive; if(dispatcherInactive != null) { dispatcherInactive(dispatcher, EventArgs.Empty); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationPosted(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationPosted = _operationPosted; if(operationPosted != null) { operationPosted(dispatcher, new DispatcherHookEventArgs(operation)); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationCompleted(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationCompleted = _operationCompleted; if(operationCompleted != null) { operationCompleted(dispatcher, new DispatcherHookEventArgs(operation)); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationPriorityChanged(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationPriorityChanged = _operationPriorityChanged; if(operationPriorityChanged != null) { operationPriorityChanged(dispatcher, new DispatcherHookEventArgs(operation)); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationAborted(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationAborted = _operationAborted; if(operationAborted != null) { operationAborted(dispatcher, new DispatcherHookEventArgs(operation)); } } private object _instanceLock = new object(); ////// Do not expose to partially trusted code. /// [SecurityCritical] private EventHandler _dispatcherInactive; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationPosted; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationCompleted; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationPriorityChanged; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationAborted; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Security; using System.Security.Permissions; namespace System.Windows.Threading { ////// Additional information provided about a dispatcher. /// public sealed class DispatcherHooks { ////// An event indicating the the dispatcher has no more operations to process. /// ////// Note that this event will be raised by the dispatcher thread when /// there is no more pending work to do. /// /// Note also that this event could come before the last operation is /// invoked, because that is when we determine that the queue is empty. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _dispatcherInactive /// TreatAsSafe: link-demands /// public event EventHandler DispatcherInactive { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _dispatcherInactive += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _dispatcherInactive -= value; } } } ////// An event indicating that an operation was posted to the dispatcher. /// ////// Typically this is due to the BeginInvoke API, but the Invoke API can /// also cause this if any priority other than DispatcherPriority.Send is /// specified, or if the destination dispatcher is owned by a different /// thread. /// /// Note that any thread can post operations, so this event can be /// raised by any thread. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationPosted /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationPosted { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationPosted += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationPosted -= value; } } } ////// An event indicating that an operation was completed. /// ////// Note that this event will be raised by the dispatcher thread after /// the operation has completed. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationCompleted /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationCompleted { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationCompleted += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationCompleted -= value; } } } ////// An event indicating that the priority of an operation was changed. /// ////// Note that any thread can change the priority of operations, /// so this event can be raised by any thread. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationPriorityChanged /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationPriorityChanged { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationPriorityChanged += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationPriorityChanged -= value; } } } ////// An event indicating that an operation was aborted. /// ////// Note that any thread can abort an operation, so this event /// can be raised by any thread. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationAborted /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationAborted { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationAborted += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationAborted -= value; } } } // Only we can create these things. internal DispatcherHooks() { } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseDispatcherInactive(Dispatcher dispatcher) { EventHandler dispatcherInactive = _dispatcherInactive; if(dispatcherInactive != null) { dispatcherInactive(dispatcher, EventArgs.Empty); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationPosted(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationPosted = _operationPosted; if(operationPosted != null) { operationPosted(dispatcher, new DispatcherHookEventArgs(operation)); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationCompleted(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationCompleted = _operationCompleted; if(operationCompleted != null) { operationCompleted(dispatcher, new DispatcherHookEventArgs(operation)); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationPriorityChanged(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationPriorityChanged = _operationPriorityChanged; if(operationPriorityChanged != null) { operationPriorityChanged(dispatcher, new DispatcherHookEventArgs(operation)); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationAborted(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationAborted = _operationAborted; if(operationAborted != null) { operationAborted(dispatcher, new DispatcherHookEventArgs(operation)); } } private object _instanceLock = new object(); ////// Do not expose to partially trusted code. /// [SecurityCritical] private EventHandler _dispatcherInactive; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationPosted; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationCompleted; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationPriorityChanged; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationAborted; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
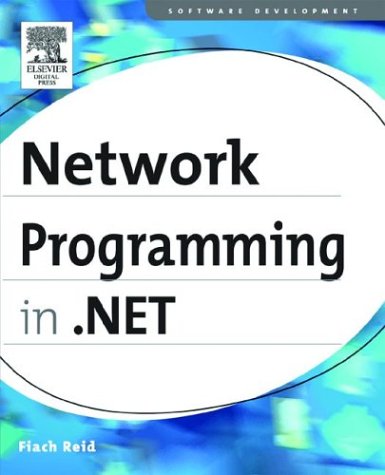
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityDataSourceWrapperCollection.cs
- KeyTimeConverter.cs
- CorrelationManager.cs
- HandlerFactoryCache.cs
- ExtenderProviderService.cs
- SchemaNames.cs
- GenericWebPart.cs
- RtfToXamlLexer.cs
- XmlEncodedRawTextWriter.cs
- DataBindingCollection.cs
- Point3DCollection.cs
- SdlChannelSink.cs
- EventMappingSettings.cs
- SerializerProvider.cs
- RelationshipFixer.cs
- ManipulationLogic.cs
- AmbientLight.cs
- PageSettings.cs
- SerialPinChanges.cs
- DataColumnMapping.cs
- AutomationEventArgs.cs
- ImageDrawing.cs
- AppearanceEditorPart.cs
- EntitySqlQueryBuilder.cs
- shaperfactoryquerycachekey.cs
- HttpTransportElement.cs
- FontFamily.cs
- StringExpressionSet.cs
- SecuritySessionServerSettings.cs
- SqlConnectionStringBuilder.cs
- Axis.cs
- ImageField.cs
- DetectEofStream.cs
- IdleTimeoutMonitor.cs
- AstTree.cs
- UnsafeNativeMethodsPenimc.cs
- RecordBuilder.cs
- Int32.cs
- EntityTransaction.cs
- UseManagedPresentationBindingElement.cs
- PrintDialog.cs
- XsdCachingReader.cs
- ExpressionBinding.cs
- DataGridViewCellStyleEditor.cs
- AliasGenerator.cs
- FormsIdentity.cs
- MessageQueueException.cs
- HashUtility.cs
- DragStartedEventArgs.cs
- WebPartDescription.cs
- ISessionStateStore.cs
- PageSetupDialog.cs
- AssemblyFilter.cs
- SoapExtensionTypeElement.cs
- StaticSiteMapProvider.cs
- CopyOnWriteList.cs
- PolyBezierSegment.cs
- MonthCalendar.cs
- activationcontext.cs
- AsymmetricSignatureDeformatter.cs
- BezierSegment.cs
- RootDesignerSerializerAttribute.cs
- DeferredElementTreeState.cs
- CommandDevice.cs
- AnimationException.cs
- ReversePositionQuery.cs
- IEnumerable.cs
- GenericPrincipal.cs
- ComponentSerializationService.cs
- ApplyTemplatesAction.cs
- PKCS1MaskGenerationMethod.cs
- XmlChildEnumerator.cs
- InternalsVisibleToAttribute.cs
- CodeDomComponentSerializationService.cs
- PropertyPathConverter.cs
- BamlRecords.cs
- QueryUtil.cs
- SQLGuidStorage.cs
- IndicFontClient.cs
- Vector3DIndependentAnimationStorage.cs
- LayoutManager.cs
- GiveFeedbackEventArgs.cs
- CommandLibraryHelper.cs
- FilteredDataSetHelper.cs
- KeyConverter.cs
- SecondaryIndexList.cs
- DefaultValueMapping.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- SqlCommand.cs
- FixedSOMPageConstructor.cs
- Opcode.cs
- ClientReliableChannelBinder.cs
- KeyInterop.cs
- HttpListenerPrefixCollection.cs
- InteropBitmapSource.cs
- AncestorChangedEventArgs.cs
- MailDefinitionBodyFileNameEditor.cs
- NativeWindow.cs
- TextBoxBase.cs
- ExtenderProvidedPropertyAttribute.cs