Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / Security / ADMembershipUser.cs / 1 / ADMembershipUser.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Security { using System.Web; using System.Web.Configuration; using System.Security.Principal; using System.Security.Permissions; using System.Globalization; using System.Runtime.Serialization; using System.Diagnostics; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Serializable] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class ActiveDirectoryMembershipUser : MembershipUser { internal bool emailModified = true; internal bool commentModified = true; internal bool isApprovedModified = true; // // private variables needed for the providerUserKey // (We need to store the provider user key here rather than the base class // to be able to do custom serialization) // private byte[] sidBinaryForm = null; [NonSerialized] private SecurityIdentifier sid = null; public override DateTime LastLoginDate { get { throw new NotSupportedException(SR.GetString(SR.ADMembership_UserProperty_not_supported, "LastLoginDate")); } set { throw new NotSupportedException(SR.GetString(SR.ADMembership_UserProperty_not_supported, "LastLoginDate")); } } public override DateTime LastActivityDate { get { throw new NotSupportedException(SR.GetString(SR.ADMembership_UserProperty_not_supported, "LastActivityDate")); } set { throw new NotSupportedException(SR.GetString(SR.ADMembership_UserProperty_not_supported, "LastActivityDate")); } } public override string Email { get { return base.Email; } set { base.Email = value; emailModified = true; } } public override string Comment { get { return base.Comment; } set { base.Comment = value; commentModified = true; } } public override bool IsApproved { get { return base.IsApproved; } set { base.IsApproved = value; isApprovedModified = true; } } public override object ProviderUserKey { get { if (sid == null && sidBinaryForm != null) sid = new SecurityIdentifier(sidBinaryForm, 0); return sid; } } public ActiveDirectoryMembershipUser(string providerName, string name, object providerUserKey, string email, string passwordQuestion, string comment, bool isApproved, bool isLockedOut, DateTime creationDate, DateTime lastLoginDate, DateTime lastActivityDate, DateTime lastPasswordChangedDate, DateTime lastLockoutDate) :base(providerName, name, null, email, passwordQuestion, comment, isApproved, isLockedOut, creationDate, lastLoginDate, lastActivityDate, lastPasswordChangedDate, lastLockoutDate) { if ((providerUserKey != null) && !(providerUserKey is SecurityIdentifier)) throw new ArgumentException( SR.GetString( SR.ADMembership_InvalidProviderUserKey ), "providerUserKey" ); sid = (SecurityIdentifier) providerUserKey; if (sid != null) { // // store the sid in binary form for serialization // sidBinaryForm = new byte[sid.BinaryLength]; sid.GetBinaryForm(sidBinaryForm, 0); } } internal ActiveDirectoryMembershipUser(string providerName, string name, byte[] sidBinaryForm, object providerUserKey, string email, string passwordQuestion, string comment, bool isApproved, bool isLockedOut, DateTime creationDate, DateTime lastLoginDate, DateTime lastActivityDate, DateTime lastPasswordChangedDate, DateTime lastLockoutDate, bool valuesAreUpdated) :base(providerName, name, null, email, passwordQuestion, comment, isApproved, isLockedOut, creationDate, lastLoginDate, lastActivityDate, lastPasswordChangedDate, lastLockoutDate) { if (valuesAreUpdated) { emailModified = false; commentModified = false; isApprovedModified = false; } Debug.Assert(sidBinaryForm != null); this.sidBinaryForm = sidBinaryForm; Debug.Assert((providerUserKey != null) && (providerUserKey is SecurityIdentifier)); sid = (SecurityIdentifier) providerUserKey; } protected ActiveDirectoryMembershipUser() { } // Default CTor: Callable by derived class only. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Security { using System.Web; using System.Web.Configuration; using System.Security.Principal; using System.Security.Permissions; using System.Globalization; using System.Runtime.Serialization; using System.Diagnostics; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Serializable] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class ActiveDirectoryMembershipUser : MembershipUser { internal bool emailModified = true; internal bool commentModified = true; internal bool isApprovedModified = true; // // private variables needed for the providerUserKey // (We need to store the provider user key here rather than the base class // to be able to do custom serialization) // private byte[] sidBinaryForm = null; [NonSerialized] private SecurityIdentifier sid = null; public override DateTime LastLoginDate { get { throw new NotSupportedException(SR.GetString(SR.ADMembership_UserProperty_not_supported, "LastLoginDate")); } set { throw new NotSupportedException(SR.GetString(SR.ADMembership_UserProperty_not_supported, "LastLoginDate")); } } public override DateTime LastActivityDate { get { throw new NotSupportedException(SR.GetString(SR.ADMembership_UserProperty_not_supported, "LastActivityDate")); } set { throw new NotSupportedException(SR.GetString(SR.ADMembership_UserProperty_not_supported, "LastActivityDate")); } } public override string Email { get { return base.Email; } set { base.Email = value; emailModified = true; } } public override string Comment { get { return base.Comment; } set { base.Comment = value; commentModified = true; } } public override bool IsApproved { get { return base.IsApproved; } set { base.IsApproved = value; isApprovedModified = true; } } public override object ProviderUserKey { get { if (sid == null && sidBinaryForm != null) sid = new SecurityIdentifier(sidBinaryForm, 0); return sid; } } public ActiveDirectoryMembershipUser(string providerName, string name, object providerUserKey, string email, string passwordQuestion, string comment, bool isApproved, bool isLockedOut, DateTime creationDate, DateTime lastLoginDate, DateTime lastActivityDate, DateTime lastPasswordChangedDate, DateTime lastLockoutDate) :base(providerName, name, null, email, passwordQuestion, comment, isApproved, isLockedOut, creationDate, lastLoginDate, lastActivityDate, lastPasswordChangedDate, lastLockoutDate) { if ((providerUserKey != null) && !(providerUserKey is SecurityIdentifier)) throw new ArgumentException( SR.GetString( SR.ADMembership_InvalidProviderUserKey ), "providerUserKey" ); sid = (SecurityIdentifier) providerUserKey; if (sid != null) { // // store the sid in binary form for serialization // sidBinaryForm = new byte[sid.BinaryLength]; sid.GetBinaryForm(sidBinaryForm, 0); } } internal ActiveDirectoryMembershipUser(string providerName, string name, byte[] sidBinaryForm, object providerUserKey, string email, string passwordQuestion, string comment, bool isApproved, bool isLockedOut, DateTime creationDate, DateTime lastLoginDate, DateTime lastActivityDate, DateTime lastPasswordChangedDate, DateTime lastLockoutDate, bool valuesAreUpdated) :base(providerName, name, null, email, passwordQuestion, comment, isApproved, isLockedOut, creationDate, lastLoginDate, lastActivityDate, lastPasswordChangedDate, lastLockoutDate) { if (valuesAreUpdated) { emailModified = false; commentModified = false; isApprovedModified = false; } Debug.Assert(sidBinaryForm != null); this.sidBinaryForm = sidBinaryForm; Debug.Assert((providerUserKey != null) && (providerUserKey is SecurityIdentifier)); sid = (SecurityIdentifier) providerUserKey; } protected ActiveDirectoryMembershipUser() { } // Default CTor: Callable by derived class only. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
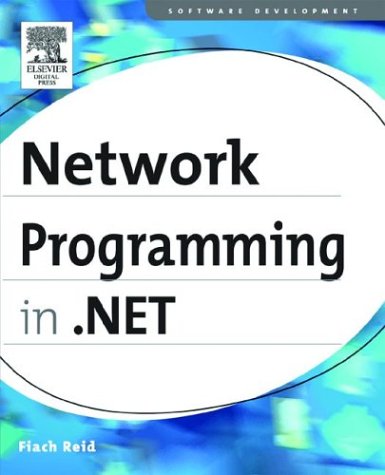
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlElementEventArgs.cs
- GridViewPageEventArgs.cs
- Polygon.cs
- MDIControlStrip.cs
- TextDecorationCollectionConverter.cs
- Pair.cs
- TrackingStringDictionary.cs
- ConnectionStringsSection.cs
- ExtensionDataObject.cs
- XmlSchemaAppInfo.cs
- ChangesetResponse.cs
- PositiveTimeSpanValidator.cs
- HtmlInputControl.cs
- SoapIncludeAttribute.cs
- TextElementAutomationPeer.cs
- NavigationProperty.cs
- SimpleTableProvider.cs
- ReadOnlyDataSourceView.cs
- ConditionalAttribute.cs
- CreateInstanceBinder.cs
- FileRegion.cs
- ContentHostHelper.cs
- GridViewCommandEventArgs.cs
- CancelAsyncOperationRequest.cs
- CodeSnippetExpression.cs
- PreservationFileReader.cs
- ClipboardData.cs
- ObjectPersistData.cs
- SocketException.cs
- XmlSchemaCollection.cs
- XmlHierarchicalDataSourceView.cs
- ObjectConverter.cs
- ObjectTokenCategory.cs
- UInt64Storage.cs
- EncoderParameters.cs
- _NtlmClient.cs
- SystemIPGlobalProperties.cs
- AesManaged.cs
- SoapAttributeOverrides.cs
- CaretElement.cs
- TextDecorations.cs
- Mouse.cs
- ToolStripItemEventArgs.cs
- GradientBrush.cs
- WhitespaceSignificantCollectionAttribute.cs
- OrCondition.cs
- ViewEventArgs.cs
- HttpCachePolicyElement.cs
- PolyLineSegment.cs
- ObfuscationAttribute.cs
- PaintEvent.cs
- PageFunction.cs
- PropertyInformation.cs
- AnnouncementInnerClientCD1.cs
- XsltException.cs
- VerificationException.cs
- TabControlAutomationPeer.cs
- FlowDocument.cs
- ColorBlend.cs
- PenLineCapValidation.cs
- RuntimeConfig.cs
- GridItemPattern.cs
- FreezableCollection.cs
- ComponentResourceKeyConverter.cs
- DummyDataSource.cs
- ReferencedAssembly.cs
- StateItem.cs
- SecurityResources.cs
- DnsEndPoint.cs
- Baml2006KeyRecord.cs
- Message.cs
- RankException.cs
- DateTimeOffset.cs
- Wizard.cs
- SharedUtils.cs
- Point3DCollectionConverter.cs
- ReadOnlyCollection.cs
- SocketPermission.cs
- ActiveXSite.cs
- TokenBasedSetEnumerator.cs
- sqlpipe.cs
- VectorConverter.cs
- RangeBaseAutomationPeer.cs
- ErrorRuntimeConfig.cs
- ThreadExceptionEvent.cs
- ProtocolsConfigurationEntry.cs
- TextBoxAutoCompleteSourceConverter.cs
- HostDesigntimeLicenseContext.cs
- BooleanConverter.cs
- BCLDebug.cs
- JournalEntryListConverter.cs
- DragDeltaEventArgs.cs
- NominalTypeEliminator.cs
- CompositeActivityTypeDescriptor.cs
- TaskFormBase.cs
- BookmarkInfo.cs
- IRCollection.cs
- RuntimeCompatibilityAttribute.cs
- RelationshipEnd.cs
- ContextDataSourceView.cs