Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / xsp / System / Web / Extensions / Compilation / WCFModel / AbstractSvcMapFileLoader.cs / 1 / AbstractSvcMapFileLoader.cs
//------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All Rights Reserved. // //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Globalization; using System.IO; using System.Text; using System.Xml; using System.Xml.Schema; #if WEB_EXTENSIONS_CODE using System.Web.Resources; #else using Microsoft.VSDesigner.Resources.Microsoft.VSDesigner; #endif #if WEB_EXTENSIONS_CODE namespace System.Web.Compilation.WCFModel #else namespace Microsoft.VSDesigner.WCFModel #endif { ////// Helper class that wraps the creation of the appropriate XML serializer /// for the svcmap class. /// /// The SvcMapFileLoader is responsible for serializing/deserializing all the referenced /// metadata and extension files. /// ///#if WEB_EXTENSIONS_CODE internal abstract class AbstractSvcMapFileLoader #else [CLSCompliant(true)] public abstract class AbstractSvcMapFileLoader #endif { /// /// Cached XML serializer for SvcMapFile objects /// private System.Xml.Serialization.XmlSerializer serializer; ////// Schema for the svcmap file /// private static XmlSchemaSet m_ServiceMapSchemaSet; ////// Load map file /// /// /// The name of the file that we are currently loading. This value is only used for /// error reporting. /// ///Deserialized SvcMapFile ///public virtual SvcMapFile LoadMapFile(string fileName) { if (fileName == null) throw new ArgumentNullException("fileName"); using (System.IO.TextReader mapFileReader = this.GetMapFileReader()) { List proxyGenerationErrors = new List (); XmlReaderSettings readerSettings = new XmlReaderSettings(); readerSettings.Schemas = ServiceMapSchemaSet; readerSettings.ValidationType = ValidationType.Schema; readerSettings.ValidationFlags = XmlSchemaValidationFlags.ReportValidationWarnings; ValidationEventHandler handler = delegate(object sender, System.Xml.Schema.ValidationEventArgs e) { bool isError = (e.Severity == XmlSeverityType.Error); proxyGenerationErrors.Add(new ProxyGenerationError(ProxyGenerationError.GeneratorState.LoadMetadata, fileName, e.Exception, !isError)); if (isError) { throw e.Exception; } }; readerSettings.ValidationEventHandler += handler; SvcMapFile svcMapFile = null; try { XmlReader xReader = XmlReader.Create(mapFileReader, readerSettings, (string)null); try { svcMapFile = (SvcMapFile)Serializer.Deserialize(xReader); } catch (System.InvalidOperationException ex) { System.Xml.XmlException xmlException = ex.InnerException as System.Xml.XmlException; if (xmlException != null) { // the innerException contains detail error message throw xmlException; } System.Xml.Schema.XmlSchemaException schemaException = ex.InnerException as System.Xml.Schema.XmlSchemaException; if (schemaException != null) { if (schemaException.LineNumber > 0) { // append line/position to the message throw new System.Xml.Schema.XmlSchemaException( String.Format(CultureInfo.CurrentCulture, WCFModelStrings.ReferenceGroup_AppendLinePosition, schemaException.Message, schemaException.LineNumber, schemaException.LinePosition), schemaException, schemaException.LineNumber, schemaException.LinePosition); } throw schemaException; } throw; } // basic check to prevent loading an invalid svcmap file ValidateSvcMapFile(svcMapFile); foreach (MetadataFile metadataFile in svcMapFile.MetadataList) { metadataFile.IsExistingFile = true; LoadMetadataFile(metadataFile); } foreach (ExtensionFile extensionFile in svcMapFile.Extensions) { extensionFile.IsExistingFile = true; LoadExtensionFile(extensionFile); } } finally { readerSettings.ValidationEventHandler -= handler; } if (svcMapFile != null) { svcMapFile.SetLoadErrors(proxyGenerationErrors); } return svcMapFile; } } /// /// Load metadata file from file system /// /// ///public virtual void LoadMetadataFile(MetadataFile metadataFile) { try { // clean up metadataFile.CleanUpContent(); metadataFile.LoadContent(this.ReadMetadataFile(metadataFile.FileName)); } catch (Exception ex) { metadataFile.ErrorInLoading = ex; } } /// /// Load extension file /// /// ///public virtual void LoadExtensionFile(ExtensionFile extensionFile) { try { // clean up extensionFile.CleanUpContent(); // load extensionFile.ContentBuffer = ReadExtensionFile(extensionFile.FileName); } catch (System.Exception ex) { extensionFile.ErrorInLoading = ex; } } /// /// Get access to a text reader that gets access to the svcmap byte stream /// ///protected abstract System.IO.TextReader GetMapFileReader(); /// /// Get access to a byte array that contain the contents of the given extension /// file /// /// /// Name of the extension file. Could be a path relative to the svcmap file location /// or the name of an item in a metadata storage. /// ///protected abstract byte[] ReadExtensionFile(string name); /// /// Get access to a byte array that contain the contents of the given metadata /// file /// /// /// Name of the metadata file. Could be a path relative to the svcmap file location /// or the name of an item in a metadata storage. /// ///protected abstract byte[] ReadMetadataFile(string name); /// /// Do basic check after the svcmap file is loaded /// /// ////// private void ValidateSvcMapFile(SvcMapFile svcMapFile) { // check duplicated file names Dictionary externalFileList = new Dictionary (StringComparer.OrdinalIgnoreCase); foreach (MetadataFile metadataFile in svcMapFile.MetadataList) { if (metadataFile.FileName != null) { if (externalFileList.ContainsKey(metadataFile.FileName)) { throw new FormatException(String.Format(CultureInfo.CurrentCulture, WCFModelStrings.ReferenceGroup_TwoExternalFilesWithSameName, metadataFile.FileName)); } else { externalFileList.Add(metadataFile.FileName, metadataFile); } } } foreach (ExtensionFile extensionFile in svcMapFile.Extensions) { if (extensionFile.FileName != null) { if (externalFileList.ContainsKey(extensionFile.FileName)) { throw new FormatException(String.Format(CultureInfo.CurrentCulture, WCFModelStrings.ReferenceGroup_TwoExternalFilesWithSameName, extensionFile.FileName)); } else { externalFileList.Add(extensionFile.FileName, extensionFile); } } } } /// /// Demand-create an XmlSerializer for the SvcMap file... /// protected virtual System.Xml.Serialization.XmlSerializer Serializer { get { if (serializer == null) { serializer = new System.Xml.Serialization.XmlSerializer(typeof(SvcMapFile), SvcMapFile.NamespaceUri); } return serializer; } } ////////// event handler to collect validation error messages ///// ///// ///// ///////private void OnValidationError(object sender, System.Xml.Schema.ValidationEventArgs e) //{ // bool isError = (e.Severity == XmlSeverityType.Error); // if (m_ProxyGenerationErrors != null) // { // m_ProxyGenerationErrors.Add(new ProxyGenerationError(ProxyGenerationError.GeneratorState.LoadMetadata, fileName, e.Exception, !isError)); // } // if (isError) // { // throw e.Exception; // } //} /// /// The schema of the service map file /// ////// private static XmlSchemaSet ServiceMapSchemaSet { get { if (m_ServiceMapSchemaSet == null) { XmlSchema serviceMapSchema; System.IO.Stream fileStream = typeof(AbstractSvcMapFileLoader).Assembly.GetManifestResourceStream(typeof(AbstractSvcMapFileLoader), @"Schema.ServiceMapSchema.xsd"); System.Diagnostics.Debug.Assert(fileStream != null, "Couldn't find ServiceMapSchema.xsd resource"); serviceMapSchema = XmlSchema.Read(fileStream, null); m_ServiceMapSchemaSet = new XmlSchemaSet(); m_ServiceMapSchemaSet.Add(serviceMapSchema); } return m_ServiceMapSchemaSet; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // Copyright (C) Microsoft Corporation. All Rights Reserved. // //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Globalization; using System.IO; using System.Text; using System.Xml; using System.Xml.Schema; #if WEB_EXTENSIONS_CODE using System.Web.Resources; #else using Microsoft.VSDesigner.Resources.Microsoft.VSDesigner; #endif #if WEB_EXTENSIONS_CODE namespace System.Web.Compilation.WCFModel #else namespace Microsoft.VSDesigner.WCFModel #endif { ////// Helper class that wraps the creation of the appropriate XML serializer /// for the svcmap class. /// /// The SvcMapFileLoader is responsible for serializing/deserializing all the referenced /// metadata and extension files. /// ///#if WEB_EXTENSIONS_CODE internal abstract class AbstractSvcMapFileLoader #else [CLSCompliant(true)] public abstract class AbstractSvcMapFileLoader #endif { /// /// Cached XML serializer for SvcMapFile objects /// private System.Xml.Serialization.XmlSerializer serializer; ////// Schema for the svcmap file /// private static XmlSchemaSet m_ServiceMapSchemaSet; ////// Load map file /// /// /// The name of the file that we are currently loading. This value is only used for /// error reporting. /// ///Deserialized SvcMapFile ///public virtual SvcMapFile LoadMapFile(string fileName) { if (fileName == null) throw new ArgumentNullException("fileName"); using (System.IO.TextReader mapFileReader = this.GetMapFileReader()) { List proxyGenerationErrors = new List (); XmlReaderSettings readerSettings = new XmlReaderSettings(); readerSettings.Schemas = ServiceMapSchemaSet; readerSettings.ValidationType = ValidationType.Schema; readerSettings.ValidationFlags = XmlSchemaValidationFlags.ReportValidationWarnings; ValidationEventHandler handler = delegate(object sender, System.Xml.Schema.ValidationEventArgs e) { bool isError = (e.Severity == XmlSeverityType.Error); proxyGenerationErrors.Add(new ProxyGenerationError(ProxyGenerationError.GeneratorState.LoadMetadata, fileName, e.Exception, !isError)); if (isError) { throw e.Exception; } }; readerSettings.ValidationEventHandler += handler; SvcMapFile svcMapFile = null; try { XmlReader xReader = XmlReader.Create(mapFileReader, readerSettings, (string)null); try { svcMapFile = (SvcMapFile)Serializer.Deserialize(xReader); } catch (System.InvalidOperationException ex) { System.Xml.XmlException xmlException = ex.InnerException as System.Xml.XmlException; if (xmlException != null) { // the innerException contains detail error message throw xmlException; } System.Xml.Schema.XmlSchemaException schemaException = ex.InnerException as System.Xml.Schema.XmlSchemaException; if (schemaException != null) { if (schemaException.LineNumber > 0) { // append line/position to the message throw new System.Xml.Schema.XmlSchemaException( String.Format(CultureInfo.CurrentCulture, WCFModelStrings.ReferenceGroup_AppendLinePosition, schemaException.Message, schemaException.LineNumber, schemaException.LinePosition), schemaException, schemaException.LineNumber, schemaException.LinePosition); } throw schemaException; } throw; } // basic check to prevent loading an invalid svcmap file ValidateSvcMapFile(svcMapFile); foreach (MetadataFile metadataFile in svcMapFile.MetadataList) { metadataFile.IsExistingFile = true; LoadMetadataFile(metadataFile); } foreach (ExtensionFile extensionFile in svcMapFile.Extensions) { extensionFile.IsExistingFile = true; LoadExtensionFile(extensionFile); } } finally { readerSettings.ValidationEventHandler -= handler; } if (svcMapFile != null) { svcMapFile.SetLoadErrors(proxyGenerationErrors); } return svcMapFile; } } /// /// Load metadata file from file system /// /// ///public virtual void LoadMetadataFile(MetadataFile metadataFile) { try { // clean up metadataFile.CleanUpContent(); metadataFile.LoadContent(this.ReadMetadataFile(metadataFile.FileName)); } catch (Exception ex) { metadataFile.ErrorInLoading = ex; } } /// /// Load extension file /// /// ///public virtual void LoadExtensionFile(ExtensionFile extensionFile) { try { // clean up extensionFile.CleanUpContent(); // load extensionFile.ContentBuffer = ReadExtensionFile(extensionFile.FileName); } catch (System.Exception ex) { extensionFile.ErrorInLoading = ex; } } /// /// Get access to a text reader that gets access to the svcmap byte stream /// ///protected abstract System.IO.TextReader GetMapFileReader(); /// /// Get access to a byte array that contain the contents of the given extension /// file /// /// /// Name of the extension file. Could be a path relative to the svcmap file location /// or the name of an item in a metadata storage. /// ///protected abstract byte[] ReadExtensionFile(string name); /// /// Get access to a byte array that contain the contents of the given metadata /// file /// /// /// Name of the metadata file. Could be a path relative to the svcmap file location /// or the name of an item in a metadata storage. /// ///protected abstract byte[] ReadMetadataFile(string name); /// /// Do basic check after the svcmap file is loaded /// /// ////// private void ValidateSvcMapFile(SvcMapFile svcMapFile) { // check duplicated file names Dictionary externalFileList = new Dictionary (StringComparer.OrdinalIgnoreCase); foreach (MetadataFile metadataFile in svcMapFile.MetadataList) { if (metadataFile.FileName != null) { if (externalFileList.ContainsKey(metadataFile.FileName)) { throw new FormatException(String.Format(CultureInfo.CurrentCulture, WCFModelStrings.ReferenceGroup_TwoExternalFilesWithSameName, metadataFile.FileName)); } else { externalFileList.Add(metadataFile.FileName, metadataFile); } } } foreach (ExtensionFile extensionFile in svcMapFile.Extensions) { if (extensionFile.FileName != null) { if (externalFileList.ContainsKey(extensionFile.FileName)) { throw new FormatException(String.Format(CultureInfo.CurrentCulture, WCFModelStrings.ReferenceGroup_TwoExternalFilesWithSameName, extensionFile.FileName)); } else { externalFileList.Add(extensionFile.FileName, extensionFile); } } } } /// /// Demand-create an XmlSerializer for the SvcMap file... /// protected virtual System.Xml.Serialization.XmlSerializer Serializer { get { if (serializer == null) { serializer = new System.Xml.Serialization.XmlSerializer(typeof(SvcMapFile), SvcMapFile.NamespaceUri); } return serializer; } } ////////// event handler to collect validation error messages ///// ///// ///// ///////private void OnValidationError(object sender, System.Xml.Schema.ValidationEventArgs e) //{ // bool isError = (e.Severity == XmlSeverityType.Error); // if (m_ProxyGenerationErrors != null) // { // m_ProxyGenerationErrors.Add(new ProxyGenerationError(ProxyGenerationError.GeneratorState.LoadMetadata, fileName, e.Exception, !isError)); // } // if (isError) // { // throw e.Exception; // } //} /// /// The schema of the service map file /// ////// private static XmlSchemaSet ServiceMapSchemaSet { get { if (m_ServiceMapSchemaSet == null) { XmlSchema serviceMapSchema; System.IO.Stream fileStream = typeof(AbstractSvcMapFileLoader).Assembly.GetManifestResourceStream(typeof(AbstractSvcMapFileLoader), @"Schema.ServiceMapSchema.xsd"); System.Diagnostics.Debug.Assert(fileStream != null, "Couldn't find ServiceMapSchema.xsd resource"); serviceMapSchema = XmlSchema.Read(fileStream, null); m_ServiceMapSchemaSet = new XmlSchemaSet(); m_ServiceMapSchemaSet.Add(serviceMapSchema); } return m_ServiceMapSchemaSet; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
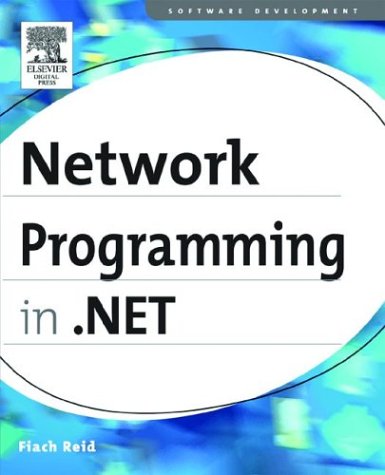
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplatedWizardStep.cs
- CorrelationTokenInvalidatedHandler.cs
- FontFamilyConverter.cs
- entityreference_tresulttype.cs
- MessageBox.cs
- PersonalizableAttribute.cs
- Calendar.cs
- XmlILOptimizerVisitor.cs
- IpcClientChannel.cs
- ComplexType.cs
- XmlSchemaProviderAttribute.cs
- Color.cs
- ComponentResourceManager.cs
- DiscriminatorMap.cs
- BStrWrapper.cs
- EntityType.cs
- PersistChildrenAttribute.cs
- Win32PrintDialog.cs
- AudioLevelUpdatedEventArgs.cs
- HelpEvent.cs
- SoapFormatterSinks.cs
- ProcessStartInfo.cs
- CustomServiceCredentials.cs
- WebPartPersonalization.cs
- UrlAuthorizationModule.cs
- OracleConnection.cs
- XmlComment.cs
- HtmlInputHidden.cs
- DataGridViewCellCollection.cs
- StoreContentChangedEventArgs.cs
- ToolStripMenuItem.cs
- HostingMessageProperty.cs
- __Filters.cs
- XmlnsCache.cs
- CompilerErrorCollection.cs
- ConnectionPoolRegistry.cs
- StatusBarItemAutomationPeer.cs
- AddingNewEventArgs.cs
- SolidBrush.cs
- ValueOfAction.cs
- DependentList.cs
- StringAttributeCollection.cs
- CustomValidator.cs
- complextypematerializer.cs
- HMACSHA384.cs
- MetadataArtifactLoaderResource.cs
- Misc.cs
- TreeNodeConverter.cs
- ScriptHandlerFactory.cs
- IdentityNotMappedException.cs
- ComponentChangedEvent.cs
- Image.cs
- OracleConnection.cs
- ByteStack.cs
- TypeUtil.cs
- SctClaimDictionary.cs
- MsmqTransportReceiveParameters.cs
- SimpleWebHandlerParser.cs
- Parser.cs
- ServiceOperation.cs
- sqlnorm.cs
- IdlingCommunicationPool.cs
- ClientBuildManager.cs
- RefType.cs
- PageStatePersister.cs
- ClientFactory.cs
- OdbcCommand.cs
- RankException.cs
- TriState.cs
- Interfaces.cs
- ImageSource.cs
- SEHException.cs
- MarshalByRefObject.cs
- HttpRequestWrapper.cs
- TextModifier.cs
- ItemsControlAutomationPeer.cs
- DesignerHelpers.cs
- ParameterBuilder.cs
- XmlBoundElement.cs
- TableCellCollection.cs
- DeviceContext.cs
- ContextMenuStripActionList.cs
- DataServiceQueryException.cs
- ExtentJoinTreeNode.cs
- WindowsScrollBar.cs
- Vector3dCollection.cs
- DataRelation.cs
- ListParagraph.cs
- XmlnsCompatibleWithAttribute.cs
- Slider.cs
- WebPartDescription.cs
- OperationAbortedException.cs
- TextSearch.cs
- Formatter.cs
- AssemblyHelper.cs
- AncillaryOps.cs
- DataGridViewCellValidatingEventArgs.cs
- HtmlAnchor.cs
- CipherData.cs
- DispatchWrapper.cs