Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Common / EntitySql / MethodExpr.cs / 2 / MethodExpr.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backup [....] //--------------------------------------------------------------------- namespace System.Data.Common.EntitySql { using System; using System.Globalization; using System.Collections; using System.Collections.Generic; using System.Data.Common.CommandTrees; using System.Diagnostics; ////// represents a method/function/aggregate function/type constructor expression /// internal sealed class MethodExpr : Expr { private Expr _leftExpr; private Identifier _methodIdentifier; private DistinctKind _distinctKind; private ExprList_args; private string _internalAggregateName; private DbExpression _dummyExpression = null; private ExprList _relationships; /// /// initializes method ast node /// /// /// /// /// internal MethodExpr( Expr left, Identifier methodId, DistinctKind distinctKind, ExprListargs ) { _leftExpr = left; _methodIdentifier = methodId; _distinctKind = distinctKind; _args = args; } /// /// intializes a method ast node /// /// /// /// /// /// internal MethodExpr(Expr left, Identifier methodId, DistinctKind distinctKind, ExprListargs, ExprList relationships) { _leftExpr = left; _methodIdentifier = methodId; _distinctKind = distinctKind; _args = args; _relationships = relationships; } /// /// left expression /// internal Expr LeftExpr { get { return _leftExpr; } } ////// method identifier /// internal Identifier MethodIdentifier { get { return _methodIdentifier; } } ////// method name /// internal string MethodName { get { return MethodIdentifier.Name; } } ////// returns a dotexpr with the entire prefix /// internal DotExpr MethodPrefixExpr { get { return new DotExpr(LeftExpr, MethodIdentifier); } } ////// defines if funtion has distict annotation /// internal DistinctKind DistinctKind { get { return _distinctKind; } } ////// argument list /// internal ExprListArgs { get { return _args; } } /// /// optional relationship list /// internal ExprListRelationships { get { return _relationships; } } /// /// Returns true if there are associated relationship expressions /// internal bool HasRelationships { get { return null != _relationships && _relationships.Count > 0; } } // // Aggregate helpers // ////// defines an internal name to be used as aggregate function /// used by semantic conversion /// internal string InternalAggregateName { get { return _internalAggregateName; } } ////// defines if a given function is aggregate /// used by semantic conversion /// internal bool WasResolved { get { return (null != _internalAggregateName); } } internal DbExpression DummyExpression { get { return _dummyExpression; } } internal void SetAggregateInfo( string internalAggregateName, DbExpression dummyExpr ) { Debug.Assert(internalAggregateName != null); Debug.Assert(dummyExpr != null); _internalAggregateName = internalAggregateName; _dummyExpression = dummyExpr; } internal void ResetDummyExpression() { _dummyExpression = null; } internal void ResetAggregateInfo() { _internalAggregateName = null; _dummyExpression = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backup [....] //--------------------------------------------------------------------- namespace System.Data.Common.EntitySql { using System; using System.Globalization; using System.Collections; using System.Collections.Generic; using System.Data.Common.CommandTrees; using System.Diagnostics; ////// represents a method/function/aggregate function/type constructor expression /// internal sealed class MethodExpr : Expr { private Expr _leftExpr; private Identifier _methodIdentifier; private DistinctKind _distinctKind; private ExprList_args; private string _internalAggregateName; private DbExpression _dummyExpression = null; private ExprList _relationships; /// /// initializes method ast node /// /// /// /// /// internal MethodExpr( Expr left, Identifier methodId, DistinctKind distinctKind, ExprListargs ) { _leftExpr = left; _methodIdentifier = methodId; _distinctKind = distinctKind; _args = args; } /// /// intializes a method ast node /// /// /// /// /// /// internal MethodExpr(Expr left, Identifier methodId, DistinctKind distinctKind, ExprListargs, ExprList relationships) { _leftExpr = left; _methodIdentifier = methodId; _distinctKind = distinctKind; _args = args; _relationships = relationships; } /// /// left expression /// internal Expr LeftExpr { get { return _leftExpr; } } ////// method identifier /// internal Identifier MethodIdentifier { get { return _methodIdentifier; } } ////// method name /// internal string MethodName { get { return MethodIdentifier.Name; } } ////// returns a dotexpr with the entire prefix /// internal DotExpr MethodPrefixExpr { get { return new DotExpr(LeftExpr, MethodIdentifier); } } ////// defines if funtion has distict annotation /// internal DistinctKind DistinctKind { get { return _distinctKind; } } ////// argument list /// internal ExprListArgs { get { return _args; } } /// /// optional relationship list /// internal ExprListRelationships { get { return _relationships; } } /// /// Returns true if there are associated relationship expressions /// internal bool HasRelationships { get { return null != _relationships && _relationships.Count > 0; } } // // Aggregate helpers // ////// defines an internal name to be used as aggregate function /// used by semantic conversion /// internal string InternalAggregateName { get { return _internalAggregateName; } } ////// defines if a given function is aggregate /// used by semantic conversion /// internal bool WasResolved { get { return (null != _internalAggregateName); } } internal DbExpression DummyExpression { get { return _dummyExpression; } } internal void SetAggregateInfo( string internalAggregateName, DbExpression dummyExpr ) { Debug.Assert(internalAggregateName != null); Debug.Assert(dummyExpr != null); _internalAggregateName = internalAggregateName; _dummyExpression = dummyExpr; } internal void ResetDummyExpression() { _dummyExpression = null; } internal void ResetAggregateInfo() { _internalAggregateName = null; _dummyExpression = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
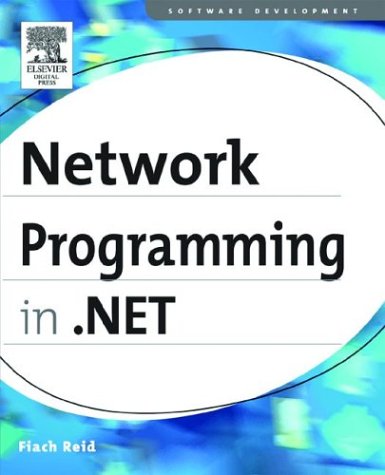
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BoundPropertyEntry.cs
- DocobjHost.cs
- ClientProxyGenerator.cs
- TextDpi.cs
- DataSourceCacheDurationConverter.cs
- TableLayoutRowStyleCollection.cs
- BamlVersionHeader.cs
- DynamicQueryableWrapper.cs
- ArrayMergeHelper.cs
- CatalogZoneBase.cs
- CommandConverter.cs
- UriWriter.cs
- TemplateParser.cs
- HybridDictionary.cs
- DirectoryNotFoundException.cs
- SerialPort.cs
- XhtmlBasicFormAdapter.cs
- InstalledFontCollection.cs
- CodeAttachEventStatement.cs
- TemplateColumn.cs
- SessionParameter.cs
- AdvancedBindingPropertyDescriptor.cs
- ExceptionRoutedEventArgs.cs
- MediaContextNotificationWindow.cs
- ProcessHostMapPath.cs
- RelationalExpressions.cs
- ExceptionTrace.cs
- SimpleTextLine.cs
- ActivityCodeDomSerializationManager.cs
- HtmlTitle.cs
- OleDbEnumerator.cs
- ExternalException.cs
- UserControlDesigner.cs
- EmptyStringExpandableObjectConverter.cs
- FileLoadException.cs
- MulticastOption.cs
- InvalidAsynchronousStateException.cs
- UnsafeNativeMethods.cs
- RequestSecurityTokenSerializer.cs
- ConfigurationStrings.cs
- KeyboardEventArgs.cs
- TransactionManager.cs
- COM2Properties.cs
- ToolStripContainer.cs
- ObjectHandle.cs
- PageVisual.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- ActivityBuilderHelper.cs
- CallbackValidatorAttribute.cs
- FactoryGenerator.cs
- RenderCapability.cs
- HierarchicalDataSourceIDConverter.cs
- WebPartTracker.cs
- BitmapData.cs
- TypedDataSetSchemaImporterExtension.cs
- WSHttpSecurity.cs
- KerberosTokenFactoryCredential.cs
- InkSerializer.cs
- EmulateRecognizeCompletedEventArgs.cs
- NumberSubstitution.cs
- DoWhileDesigner.xaml.cs
- FactorySettingsElement.cs
- ReturnType.cs
- PenLineCapValidation.cs
- TypeValidationEventArgs.cs
- CodeSnippetStatement.cs
- DCSafeHandle.cs
- SByteStorage.cs
- TcpConnectionPool.cs
- RelationshipEndMember.cs
- TransformDescriptor.cs
- UnsafeNativeMethods.cs
- RuntimeEnvironment.cs
- PageWrapper.cs
- _SslState.cs
- SpeechUI.cs
- DetailsViewModeEventArgs.cs
- SerializationAttributes.cs
- MenuBindingsEditorForm.cs
- UInt64.cs
- LoadItemsEventArgs.cs
- FeedUtils.cs
- SqlMethodCallConverter.cs
- WorkflowInstanceSuspendedRecord.cs
- ValueConversionAttribute.cs
- RoutedEventValueSerializer.cs
- ContextMenuAutomationPeer.cs
- StylusPoint.cs
- RegexCharClass.cs
- PeerResolverSettings.cs
- WmlTextViewAdapter.cs
- RtType.cs
- PageCache.cs
- NamespaceCollection.cs
- ALinqExpressionVisitor.cs
- XmlAggregates.cs
- ProxyAttribute.cs
- ImageButton.cs
- CollectionContainer.cs
- StructuralType.cs