Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / RelationshipEndMember.cs / 1305376 / RelationshipEndMember.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Data.Common; namespace System.Data.Metadata.Edm { ////// Initializes a new instance of the RelationshipEndMember class /// public abstract class RelationshipEndMember : EdmMember { #region Constructors ////// Initializes a new instance of RelationshipEndMember /// /// name of the relationship end member /// Ref type that this end refers to /// The multiplicity of this relationship end ///Thrown if name or endRefType arguments is null ///Thrown if name argument is empty string internal RelationshipEndMember(string name, RefType endRefType, RelationshipMultiplicity multiplicity) : base(name, TypeUsage.Create(endRefType, new FacetValues{ Nullable = false })) { _relationshipMultiplicity = multiplicity; _deleteBehavior = OperationAction.None; } #endregion #region Fields private OperationAction _deleteBehavior; private RelationshipMultiplicity _relationshipMultiplicity; #endregion #region Properties ////// Returns the operational behaviour for this end /// [MetadataProperty(BuiltInTypeKind.OperationAction, true)] public OperationAction DeleteBehavior { get { return _deleteBehavior; } internal set { Util.ThrowIfReadOnly(this); _deleteBehavior = value; } } ////// Returns the multiplicity for this relationship end /// [MetadataProperty(BuiltInTypeKind.RelationshipMultiplicity, false)] public RelationshipMultiplicity RelationshipMultiplicity { get { return _relationshipMultiplicity; } } #endregion public EntityType GetEntityType() { if (TypeUsage == null) return null; return (EntityType)((RefType)TypeUsage.EdmType).ElementType; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
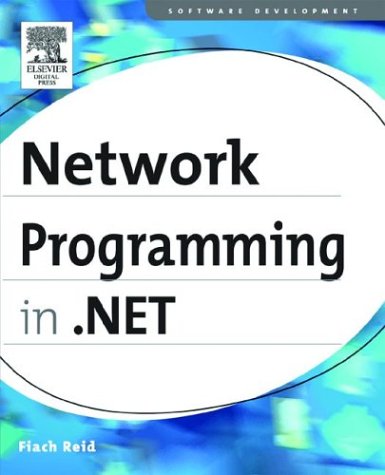
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DnsPermission.cs
- AutomationAttributeInfo.cs
- TextEditorDragDrop.cs
- SHA256Managed.cs
- VirtualPath.cs
- AttributeSetAction.cs
- XpsS0ValidatingLoader.cs
- SymbolTable.cs
- SuppressIldasmAttribute.cs
- MiniParameterInfo.cs
- ChameleonKey.cs
- PickBranchDesigner.xaml.cs
- ParallelDesigner.cs
- RNGCryptoServiceProvider.cs
- InputMethod.cs
- SecurityChannelFaultConverter.cs
- CodeDesigner.cs
- BuildManager.cs
- WindowsScrollBar.cs
- CompositionAdorner.cs
- TextServicesDisplayAttributePropertyRanges.cs
- DrawingDrawingContext.cs
- LinearQuaternionKeyFrame.cs
- Config.cs
- _SslSessionsCache.cs
- HtmlString.cs
- _NTAuthentication.cs
- AssociationProvider.cs
- DesigntimeLicenseContext.cs
- InvalidateEvent.cs
- XmlSchemaProviderAttribute.cs
- TraceSource.cs
- PriorityRange.cs
- CacheMemory.cs
- ICspAsymmetricAlgorithm.cs
- OleDbDataAdapter.cs
- ObjectIDGenerator.cs
- Convert.cs
- ServiceReference.cs
- PackagePart.cs
- CodeCatchClauseCollection.cs
- RsaSecurityKey.cs
- BamlRecords.cs
- XmlSchemaInferenceException.cs
- TextTreePropertyUndoUnit.cs
- DispatcherEventArgs.cs
- mansign.cs
- FormClosingEvent.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- ScheduleChanges.cs
- Pkcs7Recipient.cs
- _FixedSizeReader.cs
- IApplicationTrustManager.cs
- SpoolingTask.cs
- BaseTemplateBuildProvider.cs
- RequestStatusBarUpdateEventArgs.cs
- DesignerForm.cs
- CacheVirtualItemsEvent.cs
- IdentityManager.cs
- TabControlEvent.cs
- DataGridRowHeader.cs
- EventArgs.cs
- CurrentTimeZone.cs
- RuntimeEnvironment.cs
- Oid.cs
- TreeNodeSelectionProcessor.cs
- FormatterConverter.cs
- Button.cs
- UdpChannelListener.cs
- MemberHolder.cs
- DeobfuscatingStream.cs
- MobileCategoryAttribute.cs
- FormView.cs
- CanonicalFormWriter.cs
- AttributeEmitter.cs
- CommandField.cs
- XmlSchemaInclude.cs
- NativeMethods.cs
- assemblycache.cs
- TabletCollection.cs
- Freezable.cs
- XamlWriter.cs
- WebControlAdapter.cs
- _NTAuthentication.cs
- SystemResourceHost.cs
- WebPartConnectionsConfigureVerb.cs
- ProfileEventArgs.cs
- WebResourceUtil.cs
- StateInitialization.cs
- SearchForVirtualItemEventArgs.cs
- BitStack.cs
- EditorServiceContext.cs
- TypeLibConverter.cs
- PeerFlooder.cs
- GridErrorDlg.cs
- ScriptReferenceBase.cs
- WmlPageAdapter.cs
- EntityContainerRelationshipSet.cs
- DataPager.cs
- XmlQueryRuntime.cs