Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Base / System / Windows / Threading / PriorityRange.cs / 1 / PriorityRange.cs
using System; namespace System.Windows.Threading { ////// Represents a range of priorities. /// internal struct PriorityRange { ////// The range of all possible priorities. /// public static readonly PriorityRange All = new PriorityRange(DispatcherPriority.Inactive, DispatcherPriority.Send, true); // NOTE: should be Priority ////// A range that includes no priorities. /// public static readonly PriorityRange None = new PriorityRange(DispatcherPriority.Invalid, DispatcherPriority.Invalid, true); // NOTE: should be Priority ////// Constructs an instance of the PriorityRange class. /// public PriorityRange(DispatcherPriority min, DispatcherPriority max) : this() // NOTE: should be Priority { Initialize(min, true, max, true); } ////// Constructs an instance of the PriorityRange class. /// public PriorityRange(DispatcherPriority min, bool isMinInclusive, DispatcherPriority max, bool isMaxInclusive) : this() // NOTE: should be Priority { Initialize(min, isMinInclusive, max, isMaxInclusive); } ////// The minimum priority of this range. /// public DispatcherPriority Min // NOTE: should be Priority { get { return _min; } } ////// The maximum priority of this range. /// public DispatcherPriority Max // NOTE: should be Priority { get { return _max; } } ////// Whether or not the minimum priority in included in this range. /// public bool IsMinInclusive { get { return _isMinInclusive; } } ////// Whether or not the maximum priority in included in this range. /// public bool IsMaxInclusive { get { return _isMaxInclusive; } } ////// Whether or not this priority range is valid. /// public bool IsValid { get { // return _min != null && _min.IsValid && _max != null && _max.IsValid; return (_min > DispatcherPriority.Invalid && _min <= DispatcherPriority.Send && _max > DispatcherPriority.Invalid && _max <= DispatcherPriority.Send); } } ////// Whether or not this priority range contains the specified /// priority. /// public bool Contains(DispatcherPriority priority) // NOTE: should be Priority { /* if (priority == null || !priority.IsValid) { return false; } */ if(priority <= DispatcherPriority.Invalid || priority > DispatcherPriority.Send) { return false; } if (!IsValid) { return false; } bool contains = false; if (_isMinInclusive) { contains = (priority >= _min); } else { contains = (priority > _min); } if (contains) { if (_isMaxInclusive) { contains = (priority <= _max); } else { contains = (priority < _max); } } return contains; } ////// Whether or not this priority range contains the specified /// priority range. /// public bool Contains(PriorityRange priorityRange) { if (!priorityRange.IsValid) { return false; } if (!IsValid) { return false; } bool contains = false; if (priorityRange._isMinInclusive) { contains = Contains(priorityRange.Min); } else { if(priorityRange.Min >= _min && priorityRange.Min < _max) { contains = true; } } if (contains) { if (priorityRange._isMaxInclusive) { contains = Contains(priorityRange.Max); } else { if(priorityRange.Max > _min && priorityRange.Max <= _max) { contains = true; } } } return contains; } ////// Equality method for two PriorityRange /// public override bool Equals(object o) { if(o is PriorityRange) { return Equals((PriorityRange) o); } else { return false; } } ////// Equality method for two PriorityRange /// public bool Equals(PriorityRange priorityRange) { return priorityRange._min == this._min && priorityRange._isMinInclusive == this._isMinInclusive && priorityRange._max == this._max && priorityRange._isMaxInclusive == this._isMaxInclusive; } ////// Equality operator /// public static bool operator== (PriorityRange priorityRange1, PriorityRange priorityRange2) { return priorityRange1.Equals(priorityRange2); } ////// Inequality operator /// public static bool operator!= (PriorityRange priorityRange1, PriorityRange priorityRange2) { return !(priorityRange1 == priorityRange2); } ////// Returns a reasonable hash code for this PriorityRange instance. /// public override int GetHashCode() { return base.GetHashCode(); } private void Initialize(DispatcherPriority min, bool isMinInclusive, DispatcherPriority max, bool isMaxInclusive) // NOTE: should be Priority { /* if(min == null) { throw new ArgumentNullException("min"); } if (!min.IsValid) { throw new ArgumentException("Invalid priority.", "min"); } */ if(min < DispatcherPriority.Invalid || min > DispatcherPriority.Send) { // If we move to a Priority class, this exception will have to change too. throw new System.ComponentModel.InvalidEnumArgumentException("min", (int)min, typeof(DispatcherPriority)); } if(min == DispatcherPriority.Inactive) { throw new ArgumentException(SR.Get(SRID.InvalidPriority), "min"); } /* if(max == null) { throw new ArgumentNullException("max"); } if (!max.IsValid) { throw new ArgumentException("Invalid priority.", "max"); } */ if(max < DispatcherPriority.Invalid || max > DispatcherPriority.Send) { // If we move to a Priority class, this exception will have to change too. throw new System.ComponentModel.InvalidEnumArgumentException("max", (int)max, typeof(DispatcherPriority)); } if(max == DispatcherPriority.Inactive) { throw new ArgumentException(SR.Get(SRID.InvalidPriority), "max"); } if (max < min) { throw new ArgumentException(SR.Get(SRID.InvalidPriorityRangeOrder)); } _min = min; _isMinInclusive = isMinInclusive; _max = max; _isMaxInclusive = isMaxInclusive; } // This is a constructor for our special static members. private PriorityRange(DispatcherPriority min, DispatcherPriority max, bool ignored) // NOTE: should be Priority { _min = min; _isMinInclusive = true; _max = max; _isMaxInclusive = true; } private DispatcherPriority _min; // NOTE: should be Priority private bool _isMinInclusive; private DispatcherPriority _max; // NOTE: should be Priority private bool _isMaxInclusive; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; namespace System.Windows.Threading { ////// Represents a range of priorities. /// internal struct PriorityRange { ////// The range of all possible priorities. /// public static readonly PriorityRange All = new PriorityRange(DispatcherPriority.Inactive, DispatcherPriority.Send, true); // NOTE: should be Priority ////// A range that includes no priorities. /// public static readonly PriorityRange None = new PriorityRange(DispatcherPriority.Invalid, DispatcherPriority.Invalid, true); // NOTE: should be Priority ////// Constructs an instance of the PriorityRange class. /// public PriorityRange(DispatcherPriority min, DispatcherPriority max) : this() // NOTE: should be Priority { Initialize(min, true, max, true); } ////// Constructs an instance of the PriorityRange class. /// public PriorityRange(DispatcherPriority min, bool isMinInclusive, DispatcherPriority max, bool isMaxInclusive) : this() // NOTE: should be Priority { Initialize(min, isMinInclusive, max, isMaxInclusive); } ////// The minimum priority of this range. /// public DispatcherPriority Min // NOTE: should be Priority { get { return _min; } } ////// The maximum priority of this range. /// public DispatcherPriority Max // NOTE: should be Priority { get { return _max; } } ////// Whether or not the minimum priority in included in this range. /// public bool IsMinInclusive { get { return _isMinInclusive; } } ////// Whether or not the maximum priority in included in this range. /// public bool IsMaxInclusive { get { return _isMaxInclusive; } } ////// Whether or not this priority range is valid. /// public bool IsValid { get { // return _min != null && _min.IsValid && _max != null && _max.IsValid; return (_min > DispatcherPriority.Invalid && _min <= DispatcherPriority.Send && _max > DispatcherPriority.Invalid && _max <= DispatcherPriority.Send); } } ////// Whether or not this priority range contains the specified /// priority. /// public bool Contains(DispatcherPriority priority) // NOTE: should be Priority { /* if (priority == null || !priority.IsValid) { return false; } */ if(priority <= DispatcherPriority.Invalid || priority > DispatcherPriority.Send) { return false; } if (!IsValid) { return false; } bool contains = false; if (_isMinInclusive) { contains = (priority >= _min); } else { contains = (priority > _min); } if (contains) { if (_isMaxInclusive) { contains = (priority <= _max); } else { contains = (priority < _max); } } return contains; } ////// Whether or not this priority range contains the specified /// priority range. /// public bool Contains(PriorityRange priorityRange) { if (!priorityRange.IsValid) { return false; } if (!IsValid) { return false; } bool contains = false; if (priorityRange._isMinInclusive) { contains = Contains(priorityRange.Min); } else { if(priorityRange.Min >= _min && priorityRange.Min < _max) { contains = true; } } if (contains) { if (priorityRange._isMaxInclusive) { contains = Contains(priorityRange.Max); } else { if(priorityRange.Max > _min && priorityRange.Max <= _max) { contains = true; } } } return contains; } ////// Equality method for two PriorityRange /// public override bool Equals(object o) { if(o is PriorityRange) { return Equals((PriorityRange) o); } else { return false; } } ////// Equality method for two PriorityRange /// public bool Equals(PriorityRange priorityRange) { return priorityRange._min == this._min && priorityRange._isMinInclusive == this._isMinInclusive && priorityRange._max == this._max && priorityRange._isMaxInclusive == this._isMaxInclusive; } ////// Equality operator /// public static bool operator== (PriorityRange priorityRange1, PriorityRange priorityRange2) { return priorityRange1.Equals(priorityRange2); } ////// Inequality operator /// public static bool operator!= (PriorityRange priorityRange1, PriorityRange priorityRange2) { return !(priorityRange1 == priorityRange2); } ////// Returns a reasonable hash code for this PriorityRange instance. /// public override int GetHashCode() { return base.GetHashCode(); } private void Initialize(DispatcherPriority min, bool isMinInclusive, DispatcherPriority max, bool isMaxInclusive) // NOTE: should be Priority { /* if(min == null) { throw new ArgumentNullException("min"); } if (!min.IsValid) { throw new ArgumentException("Invalid priority.", "min"); } */ if(min < DispatcherPriority.Invalid || min > DispatcherPriority.Send) { // If we move to a Priority class, this exception will have to change too. throw new System.ComponentModel.InvalidEnumArgumentException("min", (int)min, typeof(DispatcherPriority)); } if(min == DispatcherPriority.Inactive) { throw new ArgumentException(SR.Get(SRID.InvalidPriority), "min"); } /* if(max == null) { throw new ArgumentNullException("max"); } if (!max.IsValid) { throw new ArgumentException("Invalid priority.", "max"); } */ if(max < DispatcherPriority.Invalid || max > DispatcherPriority.Send) { // If we move to a Priority class, this exception will have to change too. throw new System.ComponentModel.InvalidEnumArgumentException("max", (int)max, typeof(DispatcherPriority)); } if(max == DispatcherPriority.Inactive) { throw new ArgumentException(SR.Get(SRID.InvalidPriority), "max"); } if (max < min) { throw new ArgumentException(SR.Get(SRID.InvalidPriorityRangeOrder)); } _min = min; _isMinInclusive = isMinInclusive; _max = max; _isMaxInclusive = isMaxInclusive; } // This is a constructor for our special static members. private PriorityRange(DispatcherPriority min, DispatcherPriority max, bool ignored) // NOTE: should be Priority { _min = min; _isMinInclusive = true; _max = max; _isMaxInclusive = true; } private DispatcherPriority _min; // NOTE: should be Priority private bool _isMinInclusive; private DispatcherPriority _max; // NOTE: should be Priority private bool _isMaxInclusive; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
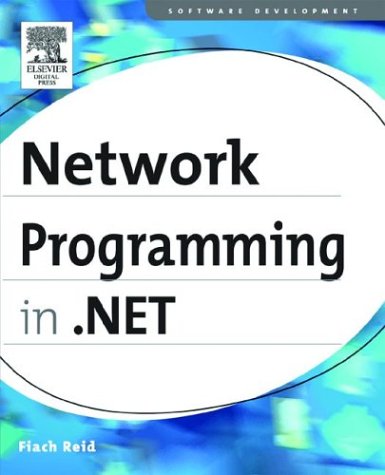
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TreeNodeClickEventArgs.cs
- SchemaMerger.cs
- DataGridTextBox.cs
- documentsequencetextview.cs
- NavigationHelper.cs
- TreeNode.cs
- ScriptControlDescriptor.cs
- LocalizabilityAttribute.cs
- DesigntimeLicenseContextSerializer.cs
- InvalidCastException.cs
- CodePropertyReferenceExpression.cs
- DiscriminatorMap.cs
- PresentationAppDomainManager.cs
- MiniMapControl.xaml.cs
- MemberBinding.cs
- InvalidEnumArgumentException.cs
- RuntimeEnvironment.cs
- InvokeBinder.cs
- ShapeTypeface.cs
- XmlArrayItemAttributes.cs
- XamlPoint3DCollectionSerializer.cs
- DayRenderEvent.cs
- ReflectionHelper.cs
- OperatingSystem.cs
- EntityCommandExecutionException.cs
- NamedObject.cs
- CodeDomDecompiler.cs
- ClientRolePrincipal.cs
- PageContentAsyncResult.cs
- MiniLockedBorderGlyph.cs
- XPathNodePointer.cs
- TextEditorTyping.cs
- ListBindingHelper.cs
- PerformanceCounterLib.cs
- Tool.cs
- SingleAnimation.cs
- ModelServiceImpl.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- DSASignatureDeformatter.cs
- ListViewDeletedEventArgs.cs
- DataStreamFromComStream.cs
- PackWebRequestFactory.cs
- FixedSOMImage.cs
- CatalogPart.cs
- ScriptingProfileServiceSection.cs
- CodeAttributeArgument.cs
- OdbcParameterCollection.cs
- WebPartConnectionsCloseVerb.cs
- ToolStripContentPanelRenderEventArgs.cs
- Rectangle.cs
- BoundsDrawingContextWalker.cs
- ArgumentValueSerializer.cs
- DataGridViewColumn.cs
- BindingContext.cs
- GraphicsContext.cs
- XPathSelectionIterator.cs
- TableParaClient.cs
- ProcessInfo.cs
- SourceFileBuildProvider.cs
- Deflater.cs
- JapaneseLunisolarCalendar.cs
- XmlNodeReader.cs
- XPathExpr.cs
- DataGridCommandEventArgs.cs
- DesignTimeParseData.cs
- EdmPropertyAttribute.cs
- ModuleElement.cs
- _ConnectStream.cs
- OutOfProcStateClientManager.cs
- ControlBindingsConverter.cs
- DynamicValueConverter.cs
- DesignerCalendarAdapter.cs
- XPathPatternParser.cs
- Component.cs
- ChangeProcessor.cs
- DecryptedHeader.cs
- SwitchAttribute.cs
- AdjustableArrowCap.cs
- WrapPanel.cs
- AutomationProperty.cs
- IList.cs
- Helper.cs
- DateTimeFormatInfoScanner.cs
- ToolTipAutomationPeer.cs
- TCEAdapterGenerator.cs
- ClientSideProviderDescription.cs
- CryptoConfig.cs
- InfoCardKeyedHashAlgorithm.cs
- MediaTimeline.cs
- Decoder.cs
- ChtmlMobileTextWriter.cs
- PropertyGridDesigner.cs
- InputLanguage.cs
- SynchronizedPool.cs
- SqlLiftIndependentRowExpressions.cs
- CheckBox.cs
- MsdtcClusterUtils.cs
- Mouse.cs
- OleStrCAMarshaler.cs
- httpstaticobjectscollection.cs