Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Actions / InvokeBinder.cs / 1305376 / InvokeBinder.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Dynamic.Utils; namespace System.Dynamic { ////// Represents the invoke dynamic operation at the call site, providing the binding semantic and the details about the operation. /// public abstract class InvokeBinder : DynamicMetaObjectBinder { private readonly CallInfo _callInfo; ////// Initializes a new instance of the /// The signature of the arguments at the call site. protected InvokeBinder(CallInfo callInfo) { ContractUtils.RequiresNotNull(callInfo, "callInfo"); _callInfo = callInfo; } ///. /// /// The result type of the operation. /// public override sealed Type ReturnType { get { return typeof(object); } } ////// Gets the signature of the arguments at the call site. /// public CallInfo CallInfo { get { return _callInfo; } } ////// Performs the binding of the dynamic invoke operation if the target dynamic object cannot bind. /// /// The target of the dynamic invoke operation. /// The arguments of the dynamic invoke operation. ///The public DynamicMetaObject FallbackInvoke(DynamicMetaObject target, DynamicMetaObject[] args) { return FallbackInvoke(target, args, null); } ///representing the result of the binding. /// Performs the binding of the dynamic invoke operation if the target dynamic object cannot bind. /// /// The target of the dynamic invoke operation. /// The arguments of the dynamic invoke operation. /// The binding result to use if binding fails, or null. ///The public abstract DynamicMetaObject FallbackInvoke(DynamicMetaObject target, DynamicMetaObject[] args, DynamicMetaObject errorSuggestion); ///representing the result of the binding. /// Performs the binding of the dynamic invoke operation. /// /// The target of the dynamic invoke operation. /// An array of arguments of the dynamic invoke operation. ///The public sealed override DynamicMetaObject Bind(DynamicMetaObject target, DynamicMetaObject[] args) { ContractUtils.RequiresNotNull(target, "target"); ContractUtils.RequiresNotNullItems(args, "args"); return target.BindInvoke(this, args); } // this is a standard DynamicMetaObjectBinder internal override sealed bool IsStandardBinder { get { return true; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.representing the result of the binding.
Link Menu
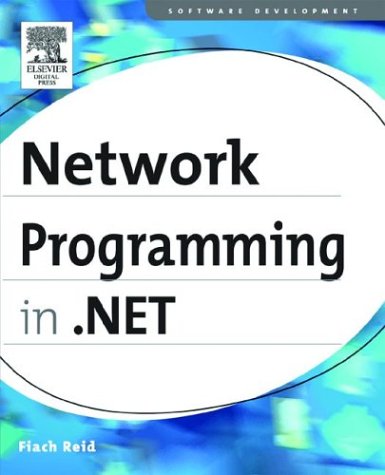
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SecurityRuntime.cs
- BoundField.cs
- Int16AnimationUsingKeyFrames.cs
- Cursors.cs
- localization.cs
- UnsafeNativeMethods.cs
- CorrelationKey.cs
- SqlGenerator.cs
- GeometryConverter.cs
- XXXOnTypeBuilderInstantiation.cs
- DataObjectSettingDataEventArgs.cs
- Attributes.cs
- WinOEToolBoxItem.cs
- ImportDesigner.xaml.cs
- InputScopeAttribute.cs
- ApplicationSecurityInfo.cs
- HttpStaticObjectsCollectionBase.cs
- NetPipeSection.cs
- Italic.cs
- DataViewListener.cs
- BrushConverter.cs
- Timeline.cs
- SQLBoolean.cs
- DataGridViewCellCancelEventArgs.cs
- DataRelation.cs
- ControlPropertyNameConverter.cs
- CommonXSendMessage.cs
- EntityAdapter.cs
- TextFormatter.cs
- ActivityTypeDesigner.xaml.cs
- odbcmetadatacolumnnames.cs
- AmbientLight.cs
- MethodCallConverter.cs
- OutputCacheModule.cs
- ItemDragEvent.cs
- TraceHelpers.cs
- DataShape.cs
- XPathArrayIterator.cs
- SafeFileMapViewHandle.cs
- CodeLinePragma.cs
- ProcessHostFactoryHelper.cs
- TrackingConditionCollection.cs
- SynchronizationContext.cs
- FontDifferentiator.cs
- HitTestWithGeometryDrawingContextWalker.cs
- CompressionTracing.cs
- TextCompositionManager.cs
- DesignTable.cs
- ReadOnlyDictionary.cs
- ValidationVisibilityAttribute.cs
- OutputCacheProfile.cs
- QilInvokeEarlyBound.cs
- EnumBuilder.cs
- Pick.cs
- PropertiesTab.cs
- ImageListUtils.cs
- DataSourceControl.cs
- QueryCacheManager.cs
- IncomingWebRequestContext.cs
- StateFinalizationActivity.cs
- HMACSHA512.cs
- InternalConfigEventArgs.cs
- Stacktrace.cs
- SafeHandles.cs
- ErrorCodes.cs
- ToolStripSplitStackLayout.cs
- GrammarBuilder.cs
- AssertHelper.cs
- DetailsViewDeletedEventArgs.cs
- ClassicBorderDecorator.cs
- TdsParserStateObject.cs
- SQLInt32.cs
- RC2.cs
- PropertyStore.cs
- VirtualDirectoryMapping.cs
- XmlTextReaderImplHelpers.cs
- DesignerDataView.cs
- StringUtil.cs
- InternalResources.cs
- RegexCode.cs
- WebPermission.cs
- StateDesigner.cs
- RightsManagementEncryptionTransform.cs
- ImageKeyConverter.cs
- GeometryCollection.cs
- GridItemPattern.cs
- VectorCollectionValueSerializer.cs
- TemplateBamlRecordReader.cs
- WrappedKeySecurityToken.cs
- XmlUTF8TextReader.cs
- PropertyDescriptorComparer.cs
- SqlConnection.cs
- XamlSerializerUtil.cs
- WebPartHeaderCloseVerb.cs
- WindowsTreeView.cs
- ISAPIApplicationHost.cs
- Table.cs
- FontNameConverter.cs
- TemplateAction.cs
- ConditionCollection.cs