Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Common / EntitySql / CqlQuery.cs / 1 / CqlQuery.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backup [....] //--------------------------------------------------------------------- namespace System.Data.Common.EntitySql { using System; using System.Collections.Generic; using System.Globalization; using System.Text; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; ////// Provides eSql text Parsing and Compilation services. /// ////// This class exposes services that performs syntactic and semantic analysis of eSql queries. /// The syntactic validation ensures the given query conforms to eSql formal grammar. The semantic analysis will /// perform (list not exhaustive): type resolution and validation, ensure semantic and scoping rules, etc. /// The services exposed by this class are: /// //////
/// Queries can be formulated in O-Space, C-Space and S-Space and the services exposed by this class are agnostic of the especific typespace or /// metadata instance passed as required parameter in the semantic analysis by the perspective parameter. It is assumed that the perspective and /// metadata was properly initialized. /// Provided that the query syntacticaly correct and meaningful within the given typespace, the result will be a valid DbCommandTree otherwise /// EntityException will be thrown indicating the reason(s) why the given query cannot be accepted. It is also possible that MetadataException and /// MappingException be thrown if mapping or metadata related problems are encountered during compilation. ///- Translation from eSql text queries to valid CommandTrees
///- Translation from eSql text queries to an AST. The AST can then be consumed by the Semantic analysis and produce a DbCommandTree
///- Given an AST, perform semantic analysis producing a valid DbCommandTree (if validateTree is true)
//////
internal static class CqlQuery { ///- ///
- ///
/// Compiles an eSql query string producing an expression constructed using the specified command tree /// /// The command tree with which to construct the expression /// eSql query text /// perspective /// parser options/// parameters /// a validated DbCommandTree ///Thrown when Syntatic or Semantic rules are violated and the query cannot be accepted ///Thrown when metadata related service requests fail ///Thrown when mapping related service requests fail ////// This method is not thread safe. /// ////// internal static DbExpression Compile(DbCommandTree builderTree, string queryText, Perspective perspective, ParserOptions parserOptions, Dictionary parameters, Dictionary variables) { #region BID TRACING // // enter bid scope // IntPtr bidCookie = IntPtr.Zero; EntityBid.ScopeEnter(out bidCookie, " perspective.TargetDataspace: %d{DataSpace}", (int)perspective.TargetDataspace); #endregion DbExpression expression = null; try { // // Validate arguments // EntityUtil.CheckArgumentNull(builderTree, "builderTree"); EntityUtil.CheckArgumentNull(queryText, "queryText"); EntityUtil.CheckArgumentNull(perspective, "perspective"); // // define parser options - if null, give default options // ParserOptions options = parserOptions ?? new ParserOptions(); // // Invoke Parser // Expr astExpr = Parse(queryText, options); // // Perform Semantic Analysis/Conversion // expression = AnalyzeSemantics(builderTree, astExpr, perspective, options, parameters, variables); TypeHelpers.AssertEdmType(expression.ResultType); } finally { #region BID TRACING // // leave bid scope // EntityBid.ScopeLeave(ref bidCookie); #endregion } Debug.Assert(null != expression, "null != expression post-condition FAILED"); return expression; } /// /// Compiles an eSql query string producing a validated command tree /// /// eSql query text /// perspective /// parser options/// parameters /// a validated DbCommandTree ///Thrown when Syntatic or Semantic rules are violated and the query cannot be accepted ///Thrown when metadata related service requests fail ///Thrown when mapping related service requests fail ////// This method is not thread safe. /// ////// internal static DbCommandTree Compile( string queryText, Perspective perspective, ParserOptions parserOptions, Dictionary parameters ) { return CqlQuery.Compile( queryText, perspective, parserOptions, parameters, null /* variables */, true /* validate cqt */); } /// /// Compiles an eSql query string producing a possibly validated command tree /// /// eSql query string /// perspective /// parser options/// ordinary parameters /// variable parameters /// Controls whether or the Command Tree produced by parsing the given string is validated before it is returned to the caller /// The Command Tree produced by parsing the given string. If validateTree is true the Command Tree has been validated ///Thrown when Syntatic or Semantic rules are violated and the query cannot be accepted ///Thrown when metadata related service requests fail ///Thrown when mapping related service requests fail ////// This method is not thread safe. /// ////// internal static DbCommandTree Compile( string query, Perspective perspective, ParserOptions parserOptions, Dictionary parameters, Dictionary variables, bool validateTree ) { #region BID TRACING // // enter bid scope // IntPtr bidCookie = IntPtr.Zero; EntityBid.ScopeEnter(out bidCookie, " perspective.TargetDataspace: %d{DataSpace}, validateTree: %d{bool}", (int)perspective.TargetDataspace, validateTree); #endregion DbCommandTree commandTree = null; try { // // Validate arguments // EntityUtil.CheckArgumentNull(query, "query"); EntityUtil.CheckArgumentNull(perspective, "perspective"); // // define parser options - if null, give default options // ParserOptions options = parserOptions ?? new ParserOptions(); // // Invoke Parser // Expr astExpr = Parse(query, options); // // Perform Semantic Analysis/Conversion // commandTree = AnalyzeSemantics(astExpr, perspective, options, parameters, variables); // // Invoke Cqt validation // if (validateTree) { commandTree.Validate(); } TypeHelpers.AssertEdmType(commandTree); } finally { #region BID TRACING // // leave bid scope // EntityBid.ScopeLeave(ref bidCookie); #endregion } Debug.Assert(null != commandTree, "null != commandTree post-condition FAILED"); return commandTree; } /// /// Parse eSql query string into and AST /// /// eSql query /// parser options/// Ast ///Thrown when Syntatic or Semantic rules are violated and the query cannot be accepted ////// This method is not thread safe. /// ///internal static Expr Parse( string query, ParserOptions parserOptions ) { #region BID TRACING // // enter bid scope // IntPtr bidCookie = IntPtr.Zero; EntityBid.ScopeEnter(out bidCookie, " "); #endregion Expr astExpr = null; try { // // Validate arguments // EntityUtil.CheckArgumentNull(query, "query"); if (query.Trim().Length == 0) { throw EntityUtil.Argument(System.Data.Entity.Strings.InvalidEmptyQueryTextArgument); } #region BID TRACING // // trace arguments // if (EntityBid.TraceOn) { EntityBid.Trace(" parserOptions.AllowQuotedIdentifiers=%d{bool}\n", parserOptions.AllowQuotedIdentifiers); EntityBid.Trace(" parserOptions.DefaultOrderByCollation='%ls'\n", parserOptions.DefaultOrderByCollation); EntityBid.Trace(" parserOptions.IdentifierCaseSensitiveness=%d{System.Data.Common.ParserOptions.CaseSensitiveness}\n", (int)parserOptions.IdentifierCaseSensitiveness); EntityBid.Trace(" query BEGIN\n"); EntityBid.PutStr(query); EntityBid.Trace(" query END\n"); } #endregion // // Create Parser // CqlParser cqlParser = new CqlParser(parserOptions, true); // // Invoke parser // astExpr = cqlParser.Parse(query); #region BID TRACING // // trace result // if (EntityBid.TraceOn) { EntityBid.Trace(" astExpr=%d#\n", (null != astExpr) ? astExpr.GetHashCode() : 0); } #endregion } finally { #region BID TRACING // // leave bid scope // EntityBid.ScopeLeave(ref bidCookie); #endregion } if (null == astExpr) { throw EntityUtil.EntitySqlError(query, System.Data.Entity.Strings.InvalidEmptyQuery, 0); } return astExpr; } /// /// Performs semantic conversion, validation and creates an expression using the specified command tree /// /// The command tree to use when creating the expression /// Abstract Syntax Tree /// perspective /// parser options/// ordinary parameters /// variable parameters /// a valid expression ///Parameters name/types must be bound before invoking this method ///Thrown when Syntatic or Semantic rules are violated and the query cannot be accepted. ///Thrown as inner exception of a EntityException when metadata related service requests fail. ///Thrown as inner exception of a EntityException when mapping related service requests fail. ////// This method is not thread safe. /// ////// internal static DbExpression AnalyzeSemantics(DbCommandTree builderTree, Expr astExpr, Perspective perspective, ParserOptions parserOptions, Dictionary parameters, Dictionary variables) { #region BID TRACING // // enter bid scope // IntPtr bidCookie = IntPtr.Zero; EntityBid.ScopeEnter(out bidCookie, " "); #endregion DbExpression expression = null; try { // // Validate Parameters // EntityUtil.CheckArgumentNull(builderTree, "builderTree"); EntityUtil.CheckArgumentNull(astExpr, "astExpr"); EntityUtil.CheckArgumentNull(perspective, "perspective"); #region BID TRACING // // trace arguments // if (EntityBid.TraceOn) { EntityBid.Trace(" astExpr=%d#\n", astExpr.GetHashCode()); EntityBid.Trace(" perspective=%d, perspective Type='%ls'\n", perspective.GetHashCode(), perspective.GetType().Name); if (null != parserOptions) { EntityBid.Trace(" parserOptions.AllowQuotedIdentifiers=%d{bool}\n", parserOptions.AllowQuotedIdentifiers); EntityBid.Trace(" parserOptions.DefaultOrderByCollation='%ls'\n", parserOptions.DefaultOrderByCollation); EntityBid.Trace(" parserOptions.IdentifierCaseSensitiveness=%d{System.Data.Common.ParserOptions.CaseSensitiveness}\n", (int)parserOptions.IdentifierCaseSensitiveness); } else EntityBid.Trace(" parserOptions is NULL\n"); if (null != parameters) foreach (KeyValuePair kvp in parameters) EntityBid.Trace(" parameter='%ls', Type='%ls'\n", kvp.Key, kvp.Value.EdmType.Name); else EntityBid.Trace(" parameters=null\n"); if (null != variables) foreach (KeyValuePair kvp in variables) EntityBid.Trace(" variable='%ls', Type='%ls'\n", kvp.Key, kvp.Value.EdmType.Name); else EntityBid.Trace(" variables=null\n"); } #endregion // // invoke semantic analysis // expression = (new SemanticAnalyzer(new SemanticResolver(perspective, parserOptions, parameters, variables))).Analyze(astExpr, builderTree); #region BID TRACING // // trace result // if (EntityBid.TraceOn && null != expression) { EntityBid.Trace(" DbExpression DUMP BEGIN\n"); DbExpression.TraceInfo(expression); EntityBid.Trace(" DbExpression DUMP END\n"); } #endregion } // // Wrap MetadataException as EntityException inner exception // catch (System.Data.MetadataException metadataException) { throw EntityUtil.EntitySqlError(System.Data.Entity.Strings.GeneralExceptionAsQueryInnerException("Metadata"), metadataException); } // // Wrap MappingException as EntityException inner exception // catch (System.Data.MappingException mappingException) { throw EntityUtil.EntitySqlError(System.Data.Entity.Strings.GeneralExceptionAsQueryInnerException("Mapping"), mappingException); } finally { #region BID TRACING // // leave Bid scope // EntityBid.ScopeLeave(ref bidCookie); #endregion } Debug.Assert(null != expression, "null != expression post-condition FAILED"); return expression; } /// /// Performs semantic conversion, validation and creates a command tree /// /// Abstract Syntax Tree /// perspective /// parser options/// ordinary parameters /// variable parameters /// a valid command tree ///Parameters name/types must be bound before invoking this method ///Thrown when Syntatic or Semantic rules are violated and the query cannot be accepted. ///Thrown as inner exception of a EntityException when metadata related service requests fail. ///Thrown as inner exception of a EntityException when mapping related service requests fail. ////// This method is not thread safe. /// ////// internal static DbCommandTree AnalyzeSemantics( Expr astExpr, Perspective perspective, ParserOptions parserOptions, Dictionary parameters, Dictionary variables) { #region BID TRACING // // enter bid scope // IntPtr bidCookie = IntPtr.Zero; EntityBid.ScopeEnter(out bidCookie, " "); #endregion DbCommandTree commandTree = null; try { // // Validate Parameters // EntityUtil.CheckArgumentNull(astExpr, "astExpr"); EntityUtil.CheckArgumentNull(perspective, "perspective"); #region BID TRACING // // trace arguments // if (EntityBid.TraceOn) { EntityBid.Trace(" astExpr=%d#\n", astExpr.GetHashCode()); EntityBid.Trace(" perspective=%d, perspective Type='%ls'\n", perspective.GetHashCode(), perspective.GetType().Name); if (null != parserOptions) { EntityBid.Trace(" parserOptions.AllowQuotedIdentifiers=%d{bool}\n", parserOptions.AllowQuotedIdentifiers); EntityBid.Trace(" parserOptions.DefaultOrderByCollation='%ls'\n", parserOptions.DefaultOrderByCollation); EntityBid.Trace(" parserOptions.IdentifierCaseSensitiveness=%d{System.Data.Common.ParserOptions.CaseSensitiveness}\n", (int)parserOptions.IdentifierCaseSensitiveness); } else EntityBid.Trace(" parserOptions is NULL\n"); if (null != parameters) foreach (KeyValuePair kvp in parameters) EntityBid.Trace(" parameter='%ls', Type='%ls'\n", kvp.Key, kvp.Value.EdmType.Name); else EntityBid.Trace(" parameters=null\n"); if (null != variables) foreach (KeyValuePair kvp in variables) EntityBid.Trace(" variable='%ls', Type='%ls'\n", kvp.Key, kvp.Value.EdmType.Name); else EntityBid.Trace(" variables=null\n"); } #endregion // // invoke semantic analysis // commandTree = (new SemanticAnalyzer(new SemanticResolver(perspective, parserOptions, parameters, variables))).Analyze(astExpr); #region BID TRACING // // trace result // if (EntityBid.TraceOn && null != commandTree) { EntityBid.Trace(" DbCommandTree DUMP BEGIN\n"); commandTree.Trace(); EntityBid.Trace(" DbCommandTree DUMP END\n"); } #endregion } // // Wrap MetadataException as EntityException inner exception // catch (System.Data.MetadataException metadataException) { throw EntityUtil.EntitySqlError(System.Data.Entity.Strings.GeneralExceptionAsQueryInnerException("Metadata"), metadataException); } // // Wrap MappingException as EntityException inner exception // catch (System.Data.MappingException mappingException) { throw EntityUtil.EntitySqlError(System.Data.Entity.Strings.GeneralExceptionAsQueryInnerException("Mapping"), mappingException); } finally { #region BID TRACING // // leave Bid scope // EntityBid.ScopeLeave(ref bidCookie); #endregion } Debug.Assert(null != commandTree, "null != commandTree post-condition FAILED"); return commandTree; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backup [....] //--------------------------------------------------------------------- namespace System.Data.Common.EntitySql { using System; using System.Collections.Generic; using System.Globalization; using System.Text; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; ////// Provides eSql text Parsing and Compilation services. /// ////// This class exposes services that performs syntactic and semantic analysis of eSql queries. /// The syntactic validation ensures the given query conforms to eSql formal grammar. The semantic analysis will /// perform (list not exhaustive): type resolution and validation, ensure semantic and scoping rules, etc. /// The services exposed by this class are: /// //////
/// Queries can be formulated in O-Space, C-Space and S-Space and the services exposed by this class are agnostic of the especific typespace or /// metadata instance passed as required parameter in the semantic analysis by the perspective parameter. It is assumed that the perspective and /// metadata was properly initialized. /// Provided that the query syntacticaly correct and meaningful within the given typespace, the result will be a valid DbCommandTree otherwise /// EntityException will be thrown indicating the reason(s) why the given query cannot be accepted. It is also possible that MetadataException and /// MappingException be thrown if mapping or metadata related problems are encountered during compilation. ///- Translation from eSql text queries to valid CommandTrees
///- Translation from eSql text queries to an AST. The AST can then be consumed by the Semantic analysis and produce a DbCommandTree
///- Given an AST, perform semantic analysis producing a valid DbCommandTree (if validateTree is true)
//////
internal static class CqlQuery { ///- ///
- ///
/// Compiles an eSql query string producing an expression constructed using the specified command tree /// /// The command tree with which to construct the expression /// eSql query text /// perspective /// parser options/// parameters /// a validated DbCommandTree ///Thrown when Syntatic or Semantic rules are violated and the query cannot be accepted ///Thrown when metadata related service requests fail ///Thrown when mapping related service requests fail ////// This method is not thread safe. /// ////// internal static DbExpression Compile(DbCommandTree builderTree, string queryText, Perspective perspective, ParserOptions parserOptions, Dictionary parameters, Dictionary variables) { #region BID TRACING // // enter bid scope // IntPtr bidCookie = IntPtr.Zero; EntityBid.ScopeEnter(out bidCookie, " perspective.TargetDataspace: %d{DataSpace}", (int)perspective.TargetDataspace); #endregion DbExpression expression = null; try { // // Validate arguments // EntityUtil.CheckArgumentNull(builderTree, "builderTree"); EntityUtil.CheckArgumentNull(queryText, "queryText"); EntityUtil.CheckArgumentNull(perspective, "perspective"); // // define parser options - if null, give default options // ParserOptions options = parserOptions ?? new ParserOptions(); // // Invoke Parser // Expr astExpr = Parse(queryText, options); // // Perform Semantic Analysis/Conversion // expression = AnalyzeSemantics(builderTree, astExpr, perspective, options, parameters, variables); TypeHelpers.AssertEdmType(expression.ResultType); } finally { #region BID TRACING // // leave bid scope // EntityBid.ScopeLeave(ref bidCookie); #endregion } Debug.Assert(null != expression, "null != expression post-condition FAILED"); return expression; } /// /// Compiles an eSql query string producing a validated command tree /// /// eSql query text /// perspective /// parser options/// parameters /// a validated DbCommandTree ///Thrown when Syntatic or Semantic rules are violated and the query cannot be accepted ///Thrown when metadata related service requests fail ///Thrown when mapping related service requests fail ////// This method is not thread safe. /// ////// internal static DbCommandTree Compile( string queryText, Perspective perspective, ParserOptions parserOptions, Dictionary parameters ) { return CqlQuery.Compile( queryText, perspective, parserOptions, parameters, null /* variables */, true /* validate cqt */); } /// /// Compiles an eSql query string producing a possibly validated command tree /// /// eSql query string /// perspective /// parser options/// ordinary parameters /// variable parameters /// Controls whether or the Command Tree produced by parsing the given string is validated before it is returned to the caller /// The Command Tree produced by parsing the given string. If validateTree is true the Command Tree has been validated ///Thrown when Syntatic or Semantic rules are violated and the query cannot be accepted ///Thrown when metadata related service requests fail ///Thrown when mapping related service requests fail ////// This method is not thread safe. /// ////// internal static DbCommandTree Compile( string query, Perspective perspective, ParserOptions parserOptions, Dictionary parameters, Dictionary variables, bool validateTree ) { #region BID TRACING // // enter bid scope // IntPtr bidCookie = IntPtr.Zero; EntityBid.ScopeEnter(out bidCookie, " perspective.TargetDataspace: %d{DataSpace}, validateTree: %d{bool}", (int)perspective.TargetDataspace, validateTree); #endregion DbCommandTree commandTree = null; try { // // Validate arguments // EntityUtil.CheckArgumentNull(query, "query"); EntityUtil.CheckArgumentNull(perspective, "perspective"); // // define parser options - if null, give default options // ParserOptions options = parserOptions ?? new ParserOptions(); // // Invoke Parser // Expr astExpr = Parse(query, options); // // Perform Semantic Analysis/Conversion // commandTree = AnalyzeSemantics(astExpr, perspective, options, parameters, variables); // // Invoke Cqt validation // if (validateTree) { commandTree.Validate(); } TypeHelpers.AssertEdmType(commandTree); } finally { #region BID TRACING // // leave bid scope // EntityBid.ScopeLeave(ref bidCookie); #endregion } Debug.Assert(null != commandTree, "null != commandTree post-condition FAILED"); return commandTree; } /// /// Parse eSql query string into and AST /// /// eSql query /// parser options/// Ast ///Thrown when Syntatic or Semantic rules are violated and the query cannot be accepted ////// This method is not thread safe. /// ///internal static Expr Parse( string query, ParserOptions parserOptions ) { #region BID TRACING // // enter bid scope // IntPtr bidCookie = IntPtr.Zero; EntityBid.ScopeEnter(out bidCookie, " "); #endregion Expr astExpr = null; try { // // Validate arguments // EntityUtil.CheckArgumentNull(query, "query"); if (query.Trim().Length == 0) { throw EntityUtil.Argument(System.Data.Entity.Strings.InvalidEmptyQueryTextArgument); } #region BID TRACING // // trace arguments // if (EntityBid.TraceOn) { EntityBid.Trace(" parserOptions.AllowQuotedIdentifiers=%d{bool}\n", parserOptions.AllowQuotedIdentifiers); EntityBid.Trace(" parserOptions.DefaultOrderByCollation='%ls'\n", parserOptions.DefaultOrderByCollation); EntityBid.Trace(" parserOptions.IdentifierCaseSensitiveness=%d{System.Data.Common.ParserOptions.CaseSensitiveness}\n", (int)parserOptions.IdentifierCaseSensitiveness); EntityBid.Trace(" query BEGIN\n"); EntityBid.PutStr(query); EntityBid.Trace(" query END\n"); } #endregion // // Create Parser // CqlParser cqlParser = new CqlParser(parserOptions, true); // // Invoke parser // astExpr = cqlParser.Parse(query); #region BID TRACING // // trace result // if (EntityBid.TraceOn) { EntityBid.Trace(" astExpr=%d#\n", (null != astExpr) ? astExpr.GetHashCode() : 0); } #endregion } finally { #region BID TRACING // // leave bid scope // EntityBid.ScopeLeave(ref bidCookie); #endregion } if (null == astExpr) { throw EntityUtil.EntitySqlError(query, System.Data.Entity.Strings.InvalidEmptyQuery, 0); } return astExpr; } /// /// Performs semantic conversion, validation and creates an expression using the specified command tree /// /// The command tree to use when creating the expression /// Abstract Syntax Tree /// perspective /// parser options/// ordinary parameters /// variable parameters /// a valid expression ///Parameters name/types must be bound before invoking this method ///Thrown when Syntatic or Semantic rules are violated and the query cannot be accepted. ///Thrown as inner exception of a EntityException when metadata related service requests fail. ///Thrown as inner exception of a EntityException when mapping related service requests fail. ////// This method is not thread safe. /// ////// internal static DbExpression AnalyzeSemantics(DbCommandTree builderTree, Expr astExpr, Perspective perspective, ParserOptions parserOptions, Dictionary parameters, Dictionary variables) { #region BID TRACING // // enter bid scope // IntPtr bidCookie = IntPtr.Zero; EntityBid.ScopeEnter(out bidCookie, " "); #endregion DbExpression expression = null; try { // // Validate Parameters // EntityUtil.CheckArgumentNull(builderTree, "builderTree"); EntityUtil.CheckArgumentNull(astExpr, "astExpr"); EntityUtil.CheckArgumentNull(perspective, "perspective"); #region BID TRACING // // trace arguments // if (EntityBid.TraceOn) { EntityBid.Trace(" astExpr=%d#\n", astExpr.GetHashCode()); EntityBid.Trace(" perspective=%d, perspective Type='%ls'\n", perspective.GetHashCode(), perspective.GetType().Name); if (null != parserOptions) { EntityBid.Trace(" parserOptions.AllowQuotedIdentifiers=%d{bool}\n", parserOptions.AllowQuotedIdentifiers); EntityBid.Trace(" parserOptions.DefaultOrderByCollation='%ls'\n", parserOptions.DefaultOrderByCollation); EntityBid.Trace(" parserOptions.IdentifierCaseSensitiveness=%d{System.Data.Common.ParserOptions.CaseSensitiveness}\n", (int)parserOptions.IdentifierCaseSensitiveness); } else EntityBid.Trace(" parserOptions is NULL\n"); if (null != parameters) foreach (KeyValuePair kvp in parameters) EntityBid.Trace(" parameter='%ls', Type='%ls'\n", kvp.Key, kvp.Value.EdmType.Name); else EntityBid.Trace(" parameters=null\n"); if (null != variables) foreach (KeyValuePair kvp in variables) EntityBid.Trace(" variable='%ls', Type='%ls'\n", kvp.Key, kvp.Value.EdmType.Name); else EntityBid.Trace(" variables=null\n"); } #endregion // // invoke semantic analysis // expression = (new SemanticAnalyzer(new SemanticResolver(perspective, parserOptions, parameters, variables))).Analyze(astExpr, builderTree); #region BID TRACING // // trace result // if (EntityBid.TraceOn && null != expression) { EntityBid.Trace(" DbExpression DUMP BEGIN\n"); DbExpression.TraceInfo(expression); EntityBid.Trace(" DbExpression DUMP END\n"); } #endregion } // // Wrap MetadataException as EntityException inner exception // catch (System.Data.MetadataException metadataException) { throw EntityUtil.EntitySqlError(System.Data.Entity.Strings.GeneralExceptionAsQueryInnerException("Metadata"), metadataException); } // // Wrap MappingException as EntityException inner exception // catch (System.Data.MappingException mappingException) { throw EntityUtil.EntitySqlError(System.Data.Entity.Strings.GeneralExceptionAsQueryInnerException("Mapping"), mappingException); } finally { #region BID TRACING // // leave Bid scope // EntityBid.ScopeLeave(ref bidCookie); #endregion } Debug.Assert(null != expression, "null != expression post-condition FAILED"); return expression; } /// /// Performs semantic conversion, validation and creates a command tree /// /// Abstract Syntax Tree /// perspective /// parser options/// ordinary parameters /// variable parameters /// a valid command tree ///Parameters name/types must be bound before invoking this method ///Thrown when Syntatic or Semantic rules are violated and the query cannot be accepted. ///Thrown as inner exception of a EntityException when metadata related service requests fail. ///Thrown as inner exception of a EntityException when mapping related service requests fail. ////// This method is not thread safe. /// ////// internal static DbCommandTree AnalyzeSemantics( Expr astExpr, Perspective perspective, ParserOptions parserOptions, Dictionary parameters, Dictionary variables) { #region BID TRACING // // enter bid scope // IntPtr bidCookie = IntPtr.Zero; EntityBid.ScopeEnter(out bidCookie, " "); #endregion DbCommandTree commandTree = null; try { // // Validate Parameters // EntityUtil.CheckArgumentNull(astExpr, "astExpr"); EntityUtil.CheckArgumentNull(perspective, "perspective"); #region BID TRACING // // trace arguments // if (EntityBid.TraceOn) { EntityBid.Trace(" astExpr=%d#\n", astExpr.GetHashCode()); EntityBid.Trace(" perspective=%d, perspective Type='%ls'\n", perspective.GetHashCode(), perspective.GetType().Name); if (null != parserOptions) { EntityBid.Trace(" parserOptions.AllowQuotedIdentifiers=%d{bool}\n", parserOptions.AllowQuotedIdentifiers); EntityBid.Trace(" parserOptions.DefaultOrderByCollation='%ls'\n", parserOptions.DefaultOrderByCollation); EntityBid.Trace(" parserOptions.IdentifierCaseSensitiveness=%d{System.Data.Common.ParserOptions.CaseSensitiveness}\n", (int)parserOptions.IdentifierCaseSensitiveness); } else EntityBid.Trace(" parserOptions is NULL\n"); if (null != parameters) foreach (KeyValuePair kvp in parameters) EntityBid.Trace(" parameter='%ls', Type='%ls'\n", kvp.Key, kvp.Value.EdmType.Name); else EntityBid.Trace(" parameters=null\n"); if (null != variables) foreach (KeyValuePair kvp in variables) EntityBid.Trace(" variable='%ls', Type='%ls'\n", kvp.Key, kvp.Value.EdmType.Name); else EntityBid.Trace(" variables=null\n"); } #endregion // // invoke semantic analysis // commandTree = (new SemanticAnalyzer(new SemanticResolver(perspective, parserOptions, parameters, variables))).Analyze(astExpr); #region BID TRACING // // trace result // if (EntityBid.TraceOn && null != commandTree) { EntityBid.Trace(" DbCommandTree DUMP BEGIN\n"); commandTree.Trace(); EntityBid.Trace(" DbCommandTree DUMP END\n"); } #endregion } // // Wrap MetadataException as EntityException inner exception // catch (System.Data.MetadataException metadataException) { throw EntityUtil.EntitySqlError(System.Data.Entity.Strings.GeneralExceptionAsQueryInnerException("Metadata"), metadataException); } // // Wrap MappingException as EntityException inner exception // catch (System.Data.MappingException mappingException) { throw EntityUtil.EntitySqlError(System.Data.Entity.Strings.GeneralExceptionAsQueryInnerException("Mapping"), mappingException); } finally { #region BID TRACING // // leave Bid scope // EntityBid.ScopeLeave(ref bidCookie); #endregion } Debug.Assert(null != commandTree, "null != commandTree post-condition FAILED"); return commandTree; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
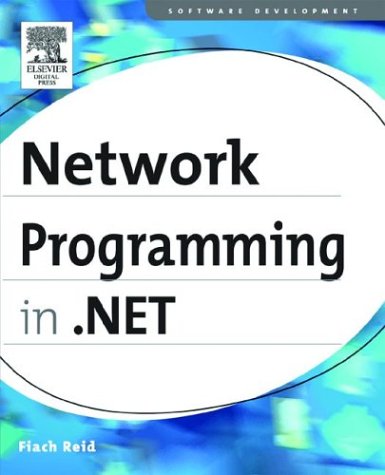
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataServices.cs
- ScriptResourceInfo.cs
- SafeProcessHandle.cs
- CreatingCookieEventArgs.cs
- DataGridViewCellPaintingEventArgs.cs
- NameValueConfigurationCollection.cs
- SignatureGenerator.cs
- ConfigurationStrings.cs
- ParenthesizePropertyNameAttribute.cs
- ValidatorUtils.cs
- SafeProcessHandle.cs
- ImmutableAssemblyCacheEntry.cs
- CommonGetThemePartSize.cs
- WebDescriptionAttribute.cs
- ControlPersister.cs
- IsolatedStoragePermission.cs
- XPathDocument.cs
- DataView.cs
- SoapFault.cs
- SqlRetyper.cs
- UIHelper.cs
- XmlSchemaValidator.cs
- HttpListenerResponse.cs
- WhitespaceRuleReader.cs
- RadioButtonStandardAdapter.cs
- RectAnimationUsingKeyFrames.cs
- AddValidationError.cs
- Attributes.cs
- EntityDataSourceChangingEventArgs.cs
- MetaChildrenColumn.cs
- LazyInitializer.cs
- GPPOINTF.cs
- DataAdapter.cs
- SafeBitVector32.cs
- MonthCalendarDesigner.cs
- TextBoxAutoCompleteSourceConverter.cs
- OverrideMode.cs
- Composition.cs
- Exceptions.cs
- WebContext.cs
- ClosableStream.cs
- XmlLanguageConverter.cs
- MsmqInputChannel.cs
- TextEffectResolver.cs
- DataTableReaderListener.cs
- DoubleAnimationUsingPath.cs
- DrawingContextDrawingContextWalker.cs
- ContainerFilterService.cs
- Win32.cs
- PageContentCollection.cs
- CodeActivity.cs
- PropertyPanel.cs
- TableCell.cs
- UserInitiatedNavigationPermission.cs
- DataObjectFieldAttribute.cs
- mediaeventshelper.cs
- rsa.cs
- IndentTextWriter.cs
- VirtualizingPanel.cs
- EffectiveValueEntry.cs
- ActivityExecutionContext.cs
- MarshalByRefObject.cs
- StrokeCollection2.cs
- ExecutionScope.cs
- TextPointerBase.cs
- SplitterPanel.cs
- WebServiceData.cs
- XmlDigitalSignatureProcessor.cs
- ModelServiceImpl.cs
- MediaCommands.cs
- PathFigure.cs
- IISMapPath.cs
- DecoderBestFitFallback.cs
- Base64Stream.cs
- DynamicDiscoveryDocument.cs
- Input.cs
- RegexCompilationInfo.cs
- Attributes.cs
- ResolveResponseInfo.cs
- ThreadInterruptedException.cs
- Expander.cs
- XmlSortKey.cs
- HttpWriter.cs
- SqlDataSourceSelectingEventArgs.cs
- Hashtable.cs
- SerializationEventsCache.cs
- BooleanProjectedSlot.cs
- AmbientValueAttribute.cs
- __Filters.cs
- CodeParameterDeclarationExpression.cs
- ObjectQuery_EntitySqlExtensions.cs
- PathTooLongException.cs
- StateMachine.cs
- ActivityScheduledQuery.cs
- OrderedEnumerableRowCollection.cs
- DynamicRenderer.cs
- SpellerStatusTable.cs
- NonParentingControl.cs
- RowCache.cs
- UnionCqlBlock.cs