Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / DynamicData / DynamicData / ModelProviders / AssociationProvider.cs / 1305376 / AssociationProvider.cs
using System.Collections.ObjectModel; using System.Globalization; using System.Web.Resources; namespace System.Web.DynamicData.ModelProviders { ////// Specifies the association cardinality /// public enum AssociationDirection { ////// 1-1 /// OneToOne, ////// one to many /// OneToMany, ////// many to one /// ManyToOne, ////// many to many /// ManyToMany } ////// Base provider class for associations between columns /// Each provider type (e.g. Linq To Sql, Entity Framework, 3rd party) extends this class. /// public abstract class AssociationProvider { private TableProvider _toTable; ////// The type of association /// public virtual AssociationDirection Direction { get; protected set; } ////// The source column of the association /// public virtual ColumnProvider FromColumn { get; protected set; } ////// The destination table of the association /// public virtual TableProvider ToTable { get { if (_toTable != null) { return _toTable; } if (ToColumn != null) { return ToColumn.Table; } return null; } protected set { _toTable = value; } } ////// The destination column of the association /// public virtual ColumnProvider ToColumn { get; protected set; } ////// Returns true if the From Column part of the primary key of its table /// e.g. Order and Product are PKs in the Order_Details table /// public virtual bool IsPrimaryKeyInThisTable { get; protected set; } ////// The names of the underlying foreign keys that make up this association /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2227:CollectionPropertiesShouldBeReadOnly", Justification="It's a readonly collection, so the warning is incorrect")] public virtual ReadOnlyCollectionForeignKeyNames { get; protected set; } /// /// Returns a string representing the sort expression that would be used for /// sorting the column represented by this association. The parameter is the /// property of the strongly typed entity used as the sort key for that entity. /// For example, assume that this association represents the Category column /// in the Products table. The sortColumn paramater is "CategoryName", /// meaning that this method is being asked to return the sort expression for /// sorting the Category column by the CategoryName property of the Category entity. /// The result sort expression would be "Category.CategoryName". /// The result of this method should be affected by whether the underlying data /// model is capable of sorting the entity by the given sort column (see /// ColumnProvider.IsSortable). The method can return a null value to indicate /// that sorting is not supported. /// /// the column to sort the entity by ///the sort expression string, or null if sort is not supported for the /// given sort column public virtual string GetSortExpression(ColumnProvider sortColumn) { return null; } internal string GetSortExpression(ColumnProvider sortColumn, string format) { if (Direction == AssociationDirection.OneToMany || Direction == AssociationDirection.ManyToMany) { throw new InvalidOperationException(String.Format(CultureInfo.CurrentCulture, DynamicDataResources.AssociationProvider_DirectionDoesNotSupportSorting, Direction)); } if (sortColumn == null) { throw new ArgumentNullException("sortColumn"); } if (!ToTable.Columns.Contains(sortColumn)) { throw new ArgumentException(DynamicDataResources.AssociationProvider_SortColumnDoesNotBelongToEndTable, "sortColumn"); } if (sortColumn.IsSortable) { return String.Format(CultureInfo.InvariantCulture, format, FromColumn.Name, sortColumn.Name); } else { return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Collections.ObjectModel; using System.Globalization; using System.Web.Resources; namespace System.Web.DynamicData.ModelProviders { ////// Specifies the association cardinality /// public enum AssociationDirection { ////// 1-1 /// OneToOne, ////// one to many /// OneToMany, ////// many to one /// ManyToOne, ////// many to many /// ManyToMany } ////// Base provider class for associations between columns /// Each provider type (e.g. Linq To Sql, Entity Framework, 3rd party) extends this class. /// public abstract class AssociationProvider { private TableProvider _toTable; ////// The type of association /// public virtual AssociationDirection Direction { get; protected set; } ////// The source column of the association /// public virtual ColumnProvider FromColumn { get; protected set; } ////// The destination table of the association /// public virtual TableProvider ToTable { get { if (_toTable != null) { return _toTable; } if (ToColumn != null) { return ToColumn.Table; } return null; } protected set { _toTable = value; } } ////// The destination column of the association /// public virtual ColumnProvider ToColumn { get; protected set; } ////// Returns true if the From Column part of the primary key of its table /// e.g. Order and Product are PKs in the Order_Details table /// public virtual bool IsPrimaryKeyInThisTable { get; protected set; } ////// The names of the underlying foreign keys that make up this association /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2227:CollectionPropertiesShouldBeReadOnly", Justification="It's a readonly collection, so the warning is incorrect")] public virtual ReadOnlyCollectionForeignKeyNames { get; protected set; } /// /// Returns a string representing the sort expression that would be used for /// sorting the column represented by this association. The parameter is the /// property of the strongly typed entity used as the sort key for that entity. /// For example, assume that this association represents the Category column /// in the Products table. The sortColumn paramater is "CategoryName", /// meaning that this method is being asked to return the sort expression for /// sorting the Category column by the CategoryName property of the Category entity. /// The result sort expression would be "Category.CategoryName". /// The result of this method should be affected by whether the underlying data /// model is capable of sorting the entity by the given sort column (see /// ColumnProvider.IsSortable). The method can return a null value to indicate /// that sorting is not supported. /// /// the column to sort the entity by ///the sort expression string, or null if sort is not supported for the /// given sort column public virtual string GetSortExpression(ColumnProvider sortColumn) { return null; } internal string GetSortExpression(ColumnProvider sortColumn, string format) { if (Direction == AssociationDirection.OneToMany || Direction == AssociationDirection.ManyToMany) { throw new InvalidOperationException(String.Format(CultureInfo.CurrentCulture, DynamicDataResources.AssociationProvider_DirectionDoesNotSupportSorting, Direction)); } if (sortColumn == null) { throw new ArgumentNullException("sortColumn"); } if (!ToTable.Columns.Contains(sortColumn)) { throw new ArgumentException(DynamicDataResources.AssociationProvider_SortColumnDoesNotBelongToEndTable, "sortColumn"); } if (sortColumn.IsSortable) { return String.Format(CultureInfo.InvariantCulture, format, FromColumn.Name, sortColumn.Name); } else { return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
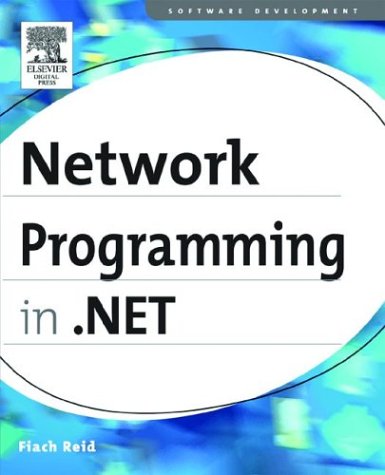
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlInputControl.cs
- Slider.cs
- InputManager.cs
- CFGGrammar.cs
- DrawListViewItemEventArgs.cs
- ToolStripItemClickedEventArgs.cs
- GenericWebPart.cs
- HostedTransportConfigurationBase.cs
- ReadWriteControlDesigner.cs
- _emptywebproxy.cs
- TypeUsage.cs
- CFStream.cs
- Brush.cs
- DeclarationUpdate.cs
- WebBrowser.cs
- CompositeFontInfo.cs
- CoTaskMemHandle.cs
- DataGridColumnHeadersPresenterAutomationPeer.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- httpserverutility.cs
- DataObject.cs
- XamlFilter.cs
- WindowShowOrOpenTracker.cs
- PenContext.cs
- AdapterSwitches.cs
- MultiTrigger.cs
- GenericPrincipal.cs
- CloudCollection.cs
- InitializationEventAttribute.cs
- StringValidator.cs
- ProgressBarBrushConverter.cs
- DataGridViewCellFormattingEventArgs.cs
- WmlLabelAdapter.cs
- ProgressBarAutomationPeer.cs
- StandardMenuStripVerb.cs
- WindowsRichEdit.cs
- SerializationSectionGroup.cs
- CngAlgorithm.cs
- MultiTrigger.cs
- PathGeometry.cs
- RangeContentEnumerator.cs
- UndoEngine.cs
- __Filters.cs
- RoutedEventConverter.cs
- DataServiceHostFactory.cs
- WebBodyFormatMessageProperty.cs
- XmlValidatingReader.cs
- ComboBoxItem.cs
- ResourceAttributes.cs
- Util.cs
- WebSysDescriptionAttribute.cs
- NetPipeSectionData.cs
- StreamedFramingRequestChannel.cs
- ToolboxSnapDragDropEventArgs.cs
- ChineseLunisolarCalendar.cs
- InvariantComparer.cs
- EmptyEnumerator.cs
- oledbmetadatacollectionnames.cs
- MetadataItem.cs
- MasterPageCodeDomTreeGenerator.cs
- FixedDocumentSequencePaginator.cs
- CompileXomlTask.cs
- DebugHandleTracker.cs
- InternalConfigSettingsFactory.cs
- WindowsIdentity.cs
- CommonProperties.cs
- TimelineGroup.cs
- EventPrivateKey.cs
- Vector3DKeyFrameCollection.cs
- SystemParameters.cs
- Utilities.cs
- ObjectTag.cs
- wmiprovider.cs
- ApplicationSecurityInfo.cs
- HitTestParameters.cs
- RowParagraph.cs
- XmlSerializerAssemblyAttribute.cs
- InfoCardKeyedHashAlgorithm.cs
- WindowsStreamSecurityUpgradeProvider.cs
- JavascriptCallbackMessageInspector.cs
- Expressions.cs
- NotifyParentPropertyAttribute.cs
- _AcceptOverlappedAsyncResult.cs
- Invariant.cs
- TimeSpanValidator.cs
- Cursor.cs
- StrongTypingException.cs
- TextElementEnumerator.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- FunctionCommandText.cs
- PermissionToken.cs
- PagerSettings.cs
- Cursors.cs
- DataPagerFieldCommandEventArgs.cs
- filewebresponse.cs
- DES.cs
- ControlBuilder.cs
- GlobalizationSection.cs
- MenuCommands.cs
- AssemblySettingAttributes.cs