Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Markup / RoutedEventConverter.cs / 1 / RoutedEventConverter.cs
// Copyright (C) Microsoft Corporation. All rights reserved. using System.ComponentModel; using System.Globalization; using System.Windows; namespace System.Windows.Markup { ////// Type converter for RoutedEvent type /// public sealed class RoutedEventConverter: TypeConverter { ////// Whether we can convert from a given type - this class only converts from string /// public override bool CanConvertFrom(ITypeDescriptorContext typeDescriptorContext, Type sourceType) { // We can only convert from a string and that too only if we have all the contextual information // Note: Sometimes even the serializer calls CanConvertFrom in order // to determine if it is a valid converter to use for serialization. if (sourceType == typeof(string) && (typeDescriptorContext is TypeConvertContext)) { return true; } return false; } ////// Whether we can convert to a given type - this class only converts to string /// public override bool CanConvertTo(ITypeDescriptorContext typeDescriptorContext, Type destinationType) { return false; } ////// Convert a string like "Button.Click" into the corresponding RoutedEvent /// public override object ConvertFrom(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object source) { string routedEventName = source as string; if( routedEventName != null ) { IServiceProvider serviceProvier = typeDescriptorContext as IServiceProvider; if (serviceProvier != null) { TypeConvertContext typeConvertContext = typeDescriptorContext as TypeConvertContext; ParserContext parserContext = typeConvertContext == null ? null : typeConvertContext.ParserContext; if( parserContext != null ) { XamlTypeMapper xamlTypeMapper = parserContext.XamlTypeMapper; if( xamlTypeMapper != null ) { RoutedEvent routedEvent; string nameSpace; nameSpace = ExtractNamespaceString( ref routedEventName, parserContext ); // Verify that there's at least one period. (A simple // but not foolproof check for "[class].[event]") if( routedEventName.IndexOf('.') != -1 ) { // When xamlTypeMapper is told to look for RoutedEventID, // we need "[class].[event]" because at this point we // have no idea what the owner type might be. // Fortunately, given "[class].[event]" xamlTypeMapper.GetRoutedEventID // can figure things out, so at this point we can pass // in 'null' for the ownerType and things will still work. routedEvent = xamlTypeMapper.GetRoutedEvent(null /* ownerType */, nameSpace, routedEventName); return routedEvent; } } } } } // Falling through here means we are unable to perform the conversion. throw GetConvertFromException(source); } ////// Convert a RoutedEventID into a XAML string like "Button.Click" /// public override object ConvertTo(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { throw GetConvertToException(value, destinationType); } // This routine is copied from TemplateBamlRecordReader. This functionality // is planned to be part of the utilities exposed by the parser, eliminating // the need to duplicate code. See task #18279 private string ExtractNamespaceString( ref string nameString, ParserContext parserContext ) { // The colon is what we look for to determine if there's a namespace prefix specifier. int nsIndex = nameString.IndexOf(':'); string nsPrefix = string.Empty; if( nsIndex != -1 ) { // Found a namespace prefix separator, so create replacement propertyName. // String processing - split "foons" from "BarClass.BazProp" nsPrefix = nameString.Substring (0, nsIndex); nameString = nameString.Substring (nsIndex + 1); } // Find the namespace, even if its the default one string namespaceURI = parserContext.XmlnsDictionary[nsPrefix]; if (namespaceURI == null) { throw new ArgumentException(SR.Get(SRID.ParserPrefixNSProperty, nsPrefix, nameString)); } return namespaceURI; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. // Copyright (C) Microsoft Corporation. All rights reserved. using System.ComponentModel; using System.Globalization; using System.Windows; namespace System.Windows.Markup { ////// Type converter for RoutedEvent type /// public sealed class RoutedEventConverter: TypeConverter { ////// Whether we can convert from a given type - this class only converts from string /// public override bool CanConvertFrom(ITypeDescriptorContext typeDescriptorContext, Type sourceType) { // We can only convert from a string and that too only if we have all the contextual information // Note: Sometimes even the serializer calls CanConvertFrom in order // to determine if it is a valid converter to use for serialization. if (sourceType == typeof(string) && (typeDescriptorContext is TypeConvertContext)) { return true; } return false; } ////// Whether we can convert to a given type - this class only converts to string /// public override bool CanConvertTo(ITypeDescriptorContext typeDescriptorContext, Type destinationType) { return false; } ////// Convert a string like "Button.Click" into the corresponding RoutedEvent /// public override object ConvertFrom(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object source) { string routedEventName = source as string; if( routedEventName != null ) { IServiceProvider serviceProvier = typeDescriptorContext as IServiceProvider; if (serviceProvier != null) { TypeConvertContext typeConvertContext = typeDescriptorContext as TypeConvertContext; ParserContext parserContext = typeConvertContext == null ? null : typeConvertContext.ParserContext; if( parserContext != null ) { XamlTypeMapper xamlTypeMapper = parserContext.XamlTypeMapper; if( xamlTypeMapper != null ) { RoutedEvent routedEvent; string nameSpace; nameSpace = ExtractNamespaceString( ref routedEventName, parserContext ); // Verify that there's at least one period. (A simple // but not foolproof check for "[class].[event]") if( routedEventName.IndexOf('.') != -1 ) { // When xamlTypeMapper is told to look for RoutedEventID, // we need "[class].[event]" because at this point we // have no idea what the owner type might be. // Fortunately, given "[class].[event]" xamlTypeMapper.GetRoutedEventID // can figure things out, so at this point we can pass // in 'null' for the ownerType and things will still work. routedEvent = xamlTypeMapper.GetRoutedEvent(null /* ownerType */, nameSpace, routedEventName); return routedEvent; } } } } } // Falling through here means we are unable to perform the conversion. throw GetConvertFromException(source); } ////// Convert a RoutedEventID into a XAML string like "Button.Click" /// public override object ConvertTo(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { throw GetConvertToException(value, destinationType); } // This routine is copied from TemplateBamlRecordReader. This functionality // is planned to be part of the utilities exposed by the parser, eliminating // the need to duplicate code. See task #18279 private string ExtractNamespaceString( ref string nameString, ParserContext parserContext ) { // The colon is what we look for to determine if there's a namespace prefix specifier. int nsIndex = nameString.IndexOf(':'); string nsPrefix = string.Empty; if( nsIndex != -1 ) { // Found a namespace prefix separator, so create replacement propertyName. // String processing - split "foons" from "BarClass.BazProp" nsPrefix = nameString.Substring (0, nsIndex); nameString = nameString.Substring (nsIndex + 1); } // Find the namespace, even if its the default one string namespaceURI = parserContext.XmlnsDictionary[nsPrefix]; if (namespaceURI == null) { throw new ArgumentException(SR.Get(SRID.ParserPrefixNSProperty, nsPrefix, nameString)); } return namespaceURI; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
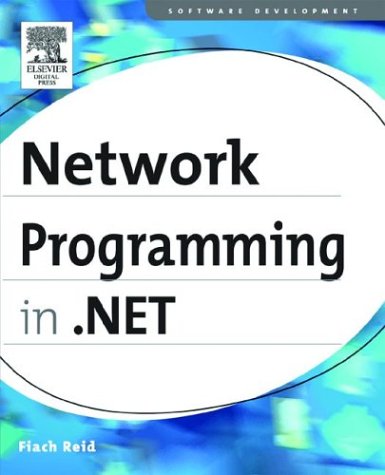
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NumericPagerField.cs
- HealthMonitoringSection.cs
- HashFinalRequest.cs
- BaseDataList.cs
- CollectionChangedEventManager.cs
- Accessible.cs
- IISMapPath.cs
- RegexCode.cs
- Attributes.cs
- ValuePattern.cs
- ThreadExceptionEvent.cs
- ImportCatalogPart.cs
- Crypto.cs
- X509InitiatorCertificateServiceElement.cs
- Marshal.cs
- IdnElement.cs
- Canvas.cs
- NotImplementedException.cs
- EventProvider.cs
- SoapSchemaMember.cs
- ListParagraph.cs
- StorageInfo.cs
- SymbolPair.cs
- EntityDataSourceContextCreatedEventArgs.cs
- CrossContextChannel.cs
- GeometryDrawing.cs
- AutoGeneratedFieldProperties.cs
- XmlTypeAttribute.cs
- FileDialogCustomPlace.cs
- TextChangedEventArgs.cs
- AssemblyCollection.cs
- SecureUICommand.cs
- DataServiceQueryProvider.cs
- UnsafeNativeMethods.cs
- SamlAdvice.cs
- PresentationSource.cs
- LassoSelectionBehavior.cs
- EpmSourcePathSegment.cs
- BitmapFrameEncode.cs
- ClearTypeHintValidation.cs
- Code.cs
- RowBinding.cs
- DragCompletedEventArgs.cs
- MimeMapping.cs
- DecimalAnimationBase.cs
- DependencyPropertyChangedEventArgs.cs
- _NtlmClient.cs
- TextPointer.cs
- tooltip.cs
- ControlCachePolicy.cs
- CommandManager.cs
- HttpListenerResponse.cs
- SemaphoreSecurity.cs
- BrowserCapabilitiesCompiler.cs
- XmlTextReaderImplHelpers.cs
- Hash.cs
- VisualStyleRenderer.cs
- SettingsPropertyCollection.cs
- StandardBindingElementCollection.cs
- TextRunProperties.cs
- ExpandableObjectConverter.cs
- ToolstripProfessionalRenderer.cs
- PtsHost.cs
- DefaultValueMapping.cs
- Avt.cs
- PartitionerStatic.cs
- RegistrySecurity.cs
- XmlImplementation.cs
- CompletedAsyncResult.cs
- LoadRetryStrategyFactory.cs
- SchemaImporterExtensionElement.cs
- ResourceDescriptionAttribute.cs
- AnnouncementService.cs
- Models.cs
- SingleObjectCollection.cs
- AssociationEndMember.cs
- EdmItemCollection.OcAssemblyCache.cs
- DataGridDetailsPresenter.cs
- HttpListenerPrefixCollection.cs
- BuildProvider.cs
- ImageCreator.cs
- FormatConvertedBitmap.cs
- SatelliteContractVersionAttribute.cs
- WindowHideOrCloseTracker.cs
- MessageDroppedTraceRecord.cs
- CustomCredentialPolicy.cs
- X509RawDataKeyIdentifierClause.cs
- MessageSecurityOverTcp.cs
- HtmlTitle.cs
- ParameterBuilder.cs
- EntityContainer.cs
- SortFieldComparer.cs
- Set.cs
- PerformanceCounterPermissionEntry.cs
- EventLogPermissionAttribute.cs
- SqlProviderManifest.cs
- HttpHandlerAction.cs
- ShadowGlyph.cs
- TableLayoutStyleCollection.cs
- AQNBuilder.cs