Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / ColorContextHelper.cs / 1 / ColorContextHelper.cs
//------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2001, 2002, 2003 // // File: ColorContextHelper.cs //----------------------------------------------------------------------------- using System; using System.IO; using System.Runtime.InteropServices; using System.Windows.Media; using System.Windows.Media.Imaging; using MS.Internal; using MS.Win32; using System.Security; using System.Security.Permissions; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Diagnostics; using System.Globalization; using Microsoft.Win32.SafeHandles; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { #region SafeProfileHandle internal class SafeProfileHandle : SafeHandleZeroOrMinusOneIsInvalid { ////// Use this constructor if the handle exists at construction time. /// ////// Critical: The ctor of the base class requires SecurityPermission /// [SecurityCritical] internal SafeProfileHandle() : base(true) { } ////// Use this constructor if the handle exists at construction time. /// ////// Critical: The ctor of the base class requires SecurityPermission /// This code calls SetHandle /// [SecurityCritical] internal SafeProfileHandle(IntPtr profile) : base(true) { SetHandle(profile); } ////// Critical - calls unmanaged code, not treat as safe because you must /// validate that handle is a valid color context handle. /// [SecurityCritical] protected override bool ReleaseHandle() { if (!UnsafeNativeMethods.Mscms.CloseColorProfile(handle)) { HRESULT.Check(Marshal.GetHRForLastWin32Error()); } return true; } } #endregion #region ColorContextHelper ////// Class to call into MSCMS color context APIs /// internal class ColorContextHelper { /// Constructor internal ColorContextHelper() { } /// Opens a color profile ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void OpenColorProfile(IntPtr pProfile) { // No need to get rid of the old handle as it will get GC'ed _profileHandle = UnsafeNativeMethods.Mscms.OpenColorProfile( pProfile, NativeMethods.PROFILE_READ, // DesiredAccess NativeMethods.FILE_SHARE_READ, // ShareMode NativeMethods.OPEN_EXISTING // CreationMode ); if (_profileHandle == null || _profileHandle.IsInvalid) { HRESULT.Check(Marshal.GetHRForLastWin32Error()); } } /// Retrieves the profile header ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void GetColorProfileHeader(IntPtr pHeader) { if (_profileHandle == null || _profileHandle.IsInvalid) { throw new InvalidOperationException(SR.Get(SRID.Image_ColorContextInvalid)); } HRESULT.Check(UnsafeNativeMethods.Mscms.GetColorProfileHeader(_profileHandle, pHeader)); } /// Retrieves the color profile from handle ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void GetColorProfileFromHandle(IntPtr pBuffer, IntPtr pdwSize) { if (_profileHandle == null || _profileHandle.IsInvalid) { throw new InvalidOperationException(SR.Get(SRID.Image_ColorContextInvalid)); } HRESULT.Check(UnsafeNativeMethods.Mscms.GetColorProfileFromHandle(_profileHandle, pBuffer, pdwSize)); } ////// Critical - Accesses critical resource _profileHandle /// TreatAsSafe - No inputs and just checks if SafeHandle is valid or not. /// internal bool IsInvalid { [SecurityCritical, SecurityTreatAsSafe] get { return (_profileHandle == null || _profileHandle.IsInvalid); } } ////// ProfileHandle /// ////// SecurityCritical: This comes out of an elevation needs to be critical and tracked. /// internal SafeProfileHandle ProfileHandle { [SecurityCritical] get { return _profileHandle; } } #region Data members ////// SecurityCritical: This comes out of an elevation needs to be critical and tracked. /// [SecurityCritical] SafeProfileHandle _profileHandle; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2001, 2002, 2003 // // File: ColorContextHelper.cs //----------------------------------------------------------------------------- using System; using System.IO; using System.Runtime.InteropServices; using System.Windows.Media; using System.Windows.Media.Imaging; using MS.Internal; using MS.Win32; using System.Security; using System.Security.Permissions; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Diagnostics; using System.Globalization; using Microsoft.Win32.SafeHandles; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using UnsafeNativeMethods=MS.Win32.PresentationCore.UnsafeNativeMethods; namespace System.Windows.Media { #region SafeProfileHandle internal class SafeProfileHandle : SafeHandleZeroOrMinusOneIsInvalid { ////// Use this constructor if the handle exists at construction time. /// ////// Critical: The ctor of the base class requires SecurityPermission /// [SecurityCritical] internal SafeProfileHandle() : base(true) { } ////// Use this constructor if the handle exists at construction time. /// ////// Critical: The ctor of the base class requires SecurityPermission /// This code calls SetHandle /// [SecurityCritical] internal SafeProfileHandle(IntPtr profile) : base(true) { SetHandle(profile); } ////// Critical - calls unmanaged code, not treat as safe because you must /// validate that handle is a valid color context handle. /// [SecurityCritical] protected override bool ReleaseHandle() { if (!UnsafeNativeMethods.Mscms.CloseColorProfile(handle)) { HRESULT.Check(Marshal.GetHRForLastWin32Error()); } return true; } } #endregion #region ColorContextHelper ////// Class to call into MSCMS color context APIs /// internal class ColorContextHelper { /// Constructor internal ColorContextHelper() { } /// Opens a color profile ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void OpenColorProfile(IntPtr pProfile) { // No need to get rid of the old handle as it will get GC'ed _profileHandle = UnsafeNativeMethods.Mscms.OpenColorProfile( pProfile, NativeMethods.PROFILE_READ, // DesiredAccess NativeMethods.FILE_SHARE_READ, // ShareMode NativeMethods.OPEN_EXISTING // CreationMode ); if (_profileHandle == null || _profileHandle.IsInvalid) { HRESULT.Check(Marshal.GetHRForLastWin32Error()); } } /// Retrieves the profile header ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void GetColorProfileHeader(IntPtr pHeader) { if (_profileHandle == null || _profileHandle.IsInvalid) { throw new InvalidOperationException(SR.Get(SRID.Image_ColorContextInvalid)); } HRESULT.Check(UnsafeNativeMethods.Mscms.GetColorProfileHeader(_profileHandle, pHeader)); } /// Retrieves the color profile from handle ////// SecurityCritical: Calls unmanaged code, accepts InPtr/unverified data. /// [SecurityCritical] internal void GetColorProfileFromHandle(IntPtr pBuffer, IntPtr pdwSize) { if (_profileHandle == null || _profileHandle.IsInvalid) { throw new InvalidOperationException(SR.Get(SRID.Image_ColorContextInvalid)); } HRESULT.Check(UnsafeNativeMethods.Mscms.GetColorProfileFromHandle(_profileHandle, pBuffer, pdwSize)); } ////// Critical - Accesses critical resource _profileHandle /// TreatAsSafe - No inputs and just checks if SafeHandle is valid or not. /// internal bool IsInvalid { [SecurityCritical, SecurityTreatAsSafe] get { return (_profileHandle == null || _profileHandle.IsInvalid); } } ////// ProfileHandle /// ////// SecurityCritical: This comes out of an elevation needs to be critical and tracked. /// internal SafeProfileHandle ProfileHandle { [SecurityCritical] get { return _profileHandle; } } #region Data members ////// SecurityCritical: This comes out of an elevation needs to be critical and tracked. /// [SecurityCritical] SafeProfileHandle _profileHandle; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
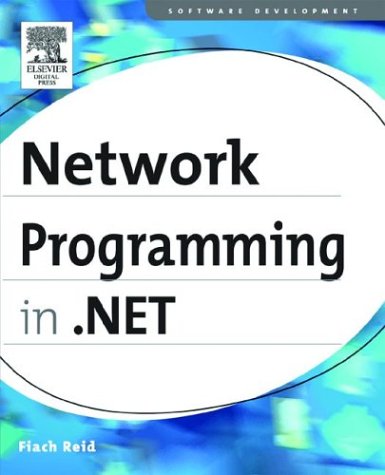
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OleDbErrorCollection.cs
- MonitorWrapper.cs
- SmtpLoginAuthenticationModule.cs
- ChtmlFormAdapter.cs
- ProgressBarAutomationPeer.cs
- FixedPageStructure.cs
- FileDetails.cs
- HandleExceptionArgs.cs
- RequestNavigateEventArgs.cs
- ResolveNameEventArgs.cs
- AssociationSet.cs
- TargetParameterCountException.cs
- TargetException.cs
- HtmlInputImage.cs
- _SafeNetHandles.cs
- WebPermission.cs
- XmlSchemaSimpleType.cs
- SystemIPInterfaceProperties.cs
- _Events.cs
- UrlPropertyAttribute.cs
- HttpHandlersSection.cs
- KerberosReceiverSecurityToken.cs
- SolidBrush.cs
- DesignerResources.cs
- FusionWrap.cs
- ConstNode.cs
- AxisAngleRotation3D.cs
- DataReaderContainer.cs
- DeferrableContentConverter.cs
- DbExpressionRules.cs
- XmlNodeComparer.cs
- StorageBasedPackageProperties.cs
- TemplateControlBuildProvider.cs
- PopOutPanel.cs
- ErrorLog.cs
- AvTraceDetails.cs
- SafeNativeMethods.cs
- ObjectDataSourceChooseMethodsPanel.cs
- UserPersonalizationStateInfo.cs
- TrackingExtract.cs
- TcpChannelFactory.cs
- ItemAutomationPeer.cs
- sqlstateclientmanager.cs
- WindowsListViewGroup.cs
- RadioButtonList.cs
- ToolStripPanel.cs
- SpecialFolderEnumConverter.cs
- basecomparevalidator.cs
- InternalCache.cs
- SecurityToken.cs
- ItemAutomationPeer.cs
- MsmqInputChannel.cs
- Menu.cs
- WebServiceHandler.cs
- ItemsControlAutomationPeer.cs
- Code.cs
- ResourcesBuildProvider.cs
- nulltextcontainer.cs
- ColorTransform.cs
- DelegatedStream.cs
- DataService.cs
- DictionaryEditChange.cs
- ConstNode.cs
- AutomationPropertyInfo.cs
- IOThreadScheduler.cs
- SelectionList.cs
- ConnectionString.cs
- SplitContainer.cs
- InternalMappingException.cs
- ResXResourceReader.cs
- RoleGroupCollection.cs
- Quad.cs
- GridViewEditEventArgs.cs
- LinkedList.cs
- ToolBarButton.cs
- SubqueryRules.cs
- ExtenderHelpers.cs
- TemplateNameScope.cs
- StateElement.cs
- SingleConverter.cs
- XmlArrayAttribute.cs
- GridItemProviderWrapper.cs
- Misc.cs
- CalendarModeChangedEventArgs.cs
- COM2ColorConverter.cs
- ManipulationDevice.cs
- ObjectToModelValueConverter.cs
- AutomationPeer.cs
- FullTextBreakpoint.cs
- ClientScriptManager.cs
- TextEffectResolver.cs
- ValueUtilsSmi.cs
- FilterQueryOptionExpression.cs
- FrameworkElementFactoryMarkupObject.cs
- ColumnHeaderConverter.cs
- ValidateNames.cs
- Thumb.cs
- TransformDescriptor.cs
- QilCloneVisitor.cs
- DocumentViewerBase.cs