Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / filewebresponse.cs / 1305376 / filewebresponse.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Runtime.Serialization; using System.IO; using System.Globalization; using System.Security.Permissions; using System.Diagnostics.CodeAnalysis; [Serializable] public class FileWebResponse : WebResponse, ISerializable, ICloseEx { const int DefaultFileStreamBufferSize = 8192; const string DefaultFileContentType = "application/octet-stream"; // fields bool m_closed; long m_contentLength; FileAccess m_fileAccess; WebHeaderCollection m_headers; Stream m_stream; Uri m_uri; // constructors internal FileWebResponse(FileWebRequest request, Uri uri, FileAccess access, bool asyncHint) { GlobalLog.Enter("FileWebResponse::FileWebResponse", "uri="+uri+", access="+access+", asyncHint="+asyncHint); try { m_fileAccess = access; if (access == FileAccess.Write) { m_stream = Stream.Null; } else { // // apparently, specifying async when the stream will be read // synchronously, or vice versa, can lead to a 10x perf hit. // While we don't know how the app will read the stream, we // use the hint from whether the app called BeginGetResponse // or GetResponse to supply the async flag to the stream ctor // m_stream = new FileWebStream(request, uri.LocalPath, FileMode.Open, FileAccess.Read, FileShare.Read, DefaultFileStreamBufferSize, asyncHint ); m_contentLength = m_stream.Length; } m_headers = new WebHeaderCollection(WebHeaderCollectionType.FileWebResponse); m_headers.AddInternal(HttpKnownHeaderNames.ContentLength, m_contentLength.ToString(NumberFormatInfo.InvariantInfo)); m_headers.AddInternal(HttpKnownHeaderNames.ContentType, DefaultFileContentType); m_uri = uri; } catch (Exception e) { Exception ex = new WebException(e.Message, e, WebExceptionStatus.ConnectFailure, null); GlobalLog.LeaveException("FileWebResponse::FileWebResponse", ex); throw ex; } GlobalLog.Leave("FileWebResponse::FileWebResponse"); } // // ISerializable constructor // [Obsolete("Serialization is obsoleted for this type. http://go.microsoft.com/fwlink/?linkid=14202")] protected FileWebResponse(SerializationInfo serializationInfo, StreamingContext streamingContext):base(serializationInfo, streamingContext) { m_headers = (WebHeaderCollection)serializationInfo.GetValue("headers", typeof(WebHeaderCollection)); m_uri = (Uri)serializationInfo.GetValue("uri", typeof(Uri)); m_contentLength = serializationInfo.GetInt64("contentLength"); m_fileAccess = (FileAccess )serializationInfo.GetInt32("fileAccess"); } // // ISerializable method // ///[SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Justification = "System.dll is still using pre-v4 security model and needs this demand")] [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter, SerializationFormatter=true)] void ISerializable.GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { GetObjectData(serializationInfo, streamingContext); } // // FxCop: provide some way for derived classes to access GetObjectData even if the derived class // explicitly re-inherits ISerializable. // [SecurityPermission(SecurityAction.Demand, SerializationFormatter=true)] protected override void GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { serializationInfo.AddValue("headers", m_headers, typeof(WebHeaderCollection)); serializationInfo.AddValue("uri", m_uri, typeof(Uri)); serializationInfo.AddValue("contentLength", m_contentLength); serializationInfo.AddValue("fileAccess", m_fileAccess); base.GetObjectData(serializationInfo, streamingContext); } // properties public override long ContentLength { get { CheckDisposed(); return m_contentLength; } } public override string ContentType { get { CheckDisposed(); return DefaultFileContentType; } } public override WebHeaderCollection Headers { get { CheckDisposed(); return m_headers; } } public override Uri ResponseUri { get { CheckDisposed(); return m_uri; } } // methods private void CheckDisposed() { if (m_closed) { throw new ObjectDisposedException(this.GetType().FullName); } } public override void Close() { ((ICloseEx)this).CloseEx(CloseExState.Normal); } void ICloseEx.CloseEx(CloseExState closeState) { GlobalLog.Enter("FileWebResponse::Close()"); try { if (!m_closed) { m_closed = true; Stream chkStream = m_stream; if (chkStream!=null) { if (chkStream is ICloseEx) ((ICloseEx)chkStream).CloseEx(closeState); else chkStream.Close(); m_stream = null; } } } finally { GlobalLog.Leave("FileWebResponse::Close()"); } } public override Stream GetResponseStream() { GlobalLog.Enter("FileWebResponse::GetResponseStream()"); try { CheckDisposed(); } finally { GlobalLog.Leave("FileWebResponse::GetResponseStream()"); } return m_stream; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System.Runtime.Serialization; using System.IO; using System.Globalization; using System.Security.Permissions; using System.Diagnostics.CodeAnalysis; [Serializable] public class FileWebResponse : WebResponse, ISerializable, ICloseEx { const int DefaultFileStreamBufferSize = 8192; const string DefaultFileContentType = "application/octet-stream"; // fields bool m_closed; long m_contentLength; FileAccess m_fileAccess; WebHeaderCollection m_headers; Stream m_stream; Uri m_uri; // constructors internal FileWebResponse(FileWebRequest request, Uri uri, FileAccess access, bool asyncHint) { GlobalLog.Enter("FileWebResponse::FileWebResponse", "uri="+uri+", access="+access+", asyncHint="+asyncHint); try { m_fileAccess = access; if (access == FileAccess.Write) { m_stream = Stream.Null; } else { // // apparently, specifying async when the stream will be read // synchronously, or vice versa, can lead to a 10x perf hit. // While we don't know how the app will read the stream, we // use the hint from whether the app called BeginGetResponse // or GetResponse to supply the async flag to the stream ctor // m_stream = new FileWebStream(request, uri.LocalPath, FileMode.Open, FileAccess.Read, FileShare.Read, DefaultFileStreamBufferSize, asyncHint ); m_contentLength = m_stream.Length; } m_headers = new WebHeaderCollection(WebHeaderCollectionType.FileWebResponse); m_headers.AddInternal(HttpKnownHeaderNames.ContentLength, m_contentLength.ToString(NumberFormatInfo.InvariantInfo)); m_headers.AddInternal(HttpKnownHeaderNames.ContentType, DefaultFileContentType); m_uri = uri; } catch (Exception e) { Exception ex = new WebException(e.Message, e, WebExceptionStatus.ConnectFailure, null); GlobalLog.LeaveException("FileWebResponse::FileWebResponse", ex); throw ex; } GlobalLog.Leave("FileWebResponse::FileWebResponse"); } // // ISerializable constructor // [Obsolete("Serialization is obsoleted for this type. http://go.microsoft.com/fwlink/?linkid=14202")] protected FileWebResponse(SerializationInfo serializationInfo, StreamingContext streamingContext):base(serializationInfo, streamingContext) { m_headers = (WebHeaderCollection)serializationInfo.GetValue("headers", typeof(WebHeaderCollection)); m_uri = (Uri)serializationInfo.GetValue("uri", typeof(Uri)); m_contentLength = serializationInfo.GetInt64("contentLength"); m_fileAccess = (FileAccess )serializationInfo.GetInt32("fileAccess"); } // // ISerializable method // ///[SuppressMessage("Microsoft.Security", "CA2123:OverrideLinkDemandsShouldBeIdenticalToBase", Justification = "System.dll is still using pre-v4 security model and needs this demand")] [SecurityPermission(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter, SerializationFormatter=true)] void ISerializable.GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { GetObjectData(serializationInfo, streamingContext); } // // FxCop: provide some way for derived classes to access GetObjectData even if the derived class // explicitly re-inherits ISerializable. // [SecurityPermission(SecurityAction.Demand, SerializationFormatter=true)] protected override void GetObjectData(SerializationInfo serializationInfo, StreamingContext streamingContext) { serializationInfo.AddValue("headers", m_headers, typeof(WebHeaderCollection)); serializationInfo.AddValue("uri", m_uri, typeof(Uri)); serializationInfo.AddValue("contentLength", m_contentLength); serializationInfo.AddValue("fileAccess", m_fileAccess); base.GetObjectData(serializationInfo, streamingContext); } // properties public override long ContentLength { get { CheckDisposed(); return m_contentLength; } } public override string ContentType { get { CheckDisposed(); return DefaultFileContentType; } } public override WebHeaderCollection Headers { get { CheckDisposed(); return m_headers; } } public override Uri ResponseUri { get { CheckDisposed(); return m_uri; } } // methods private void CheckDisposed() { if (m_closed) { throw new ObjectDisposedException(this.GetType().FullName); } } public override void Close() { ((ICloseEx)this).CloseEx(CloseExState.Normal); } void ICloseEx.CloseEx(CloseExState closeState) { GlobalLog.Enter("FileWebResponse::Close()"); try { if (!m_closed) { m_closed = true; Stream chkStream = m_stream; if (chkStream!=null) { if (chkStream is ICloseEx) ((ICloseEx)chkStream).CloseEx(closeState); else chkStream.Close(); m_stream = null; } } } finally { GlobalLog.Leave("FileWebResponse::Close()"); } } public override Stream GetResponseStream() { GlobalLog.Enter("FileWebResponse::GetResponseStream()"); try { CheckDisposed(); } finally { GlobalLog.Leave("FileWebResponse::GetResponseStream()"); } return m_stream; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
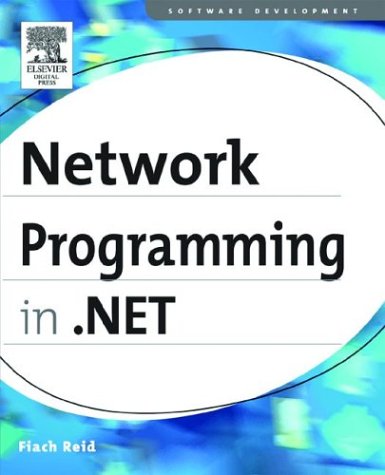
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StickyNoteAnnotations.cs
- XmlCharType.cs
- JobPageOrder.cs
- WindowsListViewItem.cs
- PropertyKey.cs
- InputLangChangeRequestEvent.cs
- _UriSyntax.cs
- Model3DCollection.cs
- FieldToken.cs
- XmlSchemaSet.cs
- DictionaryEntry.cs
- CqlGenerator.cs
- OutgoingWebResponseContext.cs
- IssuedSecurityTokenProvider.cs
- WebEventTraceProvider.cs
- ClientEventManager.cs
- ClientReliableChannelBinder.cs
- SecurityKeyIdentifier.cs
- UntypedNullExpression.cs
- RemoveStoryboard.cs
- MulticastDelegate.cs
- Formatter.cs
- FlowDocumentPaginator.cs
- VisualBasicReference.cs
- XmlProcessingInstruction.cs
- ClientSettingsStore.cs
- GregorianCalendarHelper.cs
- SmiContextFactory.cs
- StateMachineSubscriptionManager.cs
- HelpInfo.cs
- SoapSchemaExporter.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- ValidationRuleCollection.cs
- SoapInteropTypes.cs
- UnsafeNativeMethods.cs
- DataSourceListEditor.cs
- KeyInstance.cs
- MaterialCollection.cs
- NeutralResourcesLanguageAttribute.cs
- SqlDataReaderSmi.cs
- ReadOnlyPropertyMetadata.cs
- GridSplitter.cs
- XLinq.cs
- ErrorEventArgs.cs
- DataGridViewRowsAddedEventArgs.cs
- FileEnumerator.cs
- BrowsableAttribute.cs
- TheQuery.cs
- WebPartCancelEventArgs.cs
- SiteMapProvider.cs
- XmlSchemaSimpleContentExtension.cs
- DeferredSelectedIndexReference.cs
- GridView.cs
- Reference.cs
- Delegate.cs
- HttpGetClientProtocol.cs
- ConfigurationManagerInternal.cs
- DefaultProxySection.cs
- TextWriterTraceListener.cs
- UpdateTranslator.cs
- SurrogateChar.cs
- ResolveCriteria.cs
- FixedFlowMap.cs
- XmlILModule.cs
- DetailsViewAutoFormat.cs
- DoubleUtil.cs
- XPathSelfQuery.cs
- SmiXetterAccessMap.cs
- WSTrust.cs
- xmlformatgeneratorstatics.cs
- AssertFilter.cs
- BitmapEffectDrawingContextWalker.cs
- HttpConfigurationSystem.cs
- TrailingSpaceComparer.cs
- BlockCollection.cs
- Action.cs
- DiscreteKeyFrames.cs
- EntityDataSourceDesignerHelper.cs
- EventRouteFactory.cs
- SchemaSetCompiler.cs
- GeneralTransform3DGroup.cs
- SourceElementsCollection.cs
- SQLDateTimeStorage.cs
- TypeToken.cs
- ListViewAutomationPeer.cs
- SafeEventHandle.cs
- GroupQuery.cs
- ObjectItemCachedAssemblyLoader.cs
- ObjectItemNoOpAssemblyLoader.cs
- CodeAttributeDeclarationCollection.cs
- CursorInteropHelper.cs
- GlyphingCache.cs
- DataColumnCollection.cs
- WebReferenceOptions.cs
- ParameterCollection.cs
- SerialStream.cs
- RadioButtonBaseAdapter.cs
- SapiRecoInterop.cs
- EdmSchemaError.cs
- XmlSchemaComplexContentExtension.cs