Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / Xml / System / Xml / Dom / XmlProcessingInstruction.cs / 1 / XmlProcessingInstruction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System; using System.IO; using System.Diagnostics; using System.Text; using System.Xml.XPath; // Represents a processing instruction, which XML defines to keep // processor-specific information in the text of the document. public class XmlProcessingInstruction : XmlLinkedNode { string target; string data; protected internal XmlProcessingInstruction( string target, string data, XmlDocument doc ) : base( doc ) { this.target = target; this.data = data; } // Gets the name of the node. public override String Name { get { if (target != null) return target; return String.Empty; } } // Gets the name of the current node without the namespace prefix. public override string LocalName { get { return Name;} } // Gets or sets the value of the node. public override String Value { get { return data;} set { Data = value; } //use Data instead of data so that event will be fired } // Gets the target of the processing instruction. public String Target { get { return target;} } // Gets or sets the content of processing instruction, // excluding the target. public String Data { get { return data;} set { XmlNode parent = ParentNode; XmlNodeChangedEventArgs args = GetEventArgs( this, parent, parent, data, value, XmlNodeChangedAction.Change ); if (args != null) BeforeEvent( args ); data = value; if (args != null) AfterEvent( args ); } } // Gets or sets the concatenated values of the node and // all its children. public override string InnerText { get { return data;} set { Data = value; } //use Data instead of data so that change event will be fired } // Gets the type of the current node. public override XmlNodeType NodeType { get { return XmlNodeType.ProcessingInstruction;} } // Creates a duplicate of this node. public override XmlNode CloneNode(bool deep) { Debug.Assert( OwnerDocument != null ); return OwnerDocument.CreateProcessingInstruction( target, data ); } // Saves the node to the specified XmlWriter. public override void WriteTo(XmlWriter w) { w.WriteProcessingInstruction(target, data); } // Saves all the children of the node to the specified XmlWriter. public override void WriteContentTo(XmlWriter w) { // Intentionally do nothing } internal override string XPLocalName { get { return Name; } } internal override XPathNodeType XPNodeType { get { return XPathNodeType.ProcessingInstruction; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
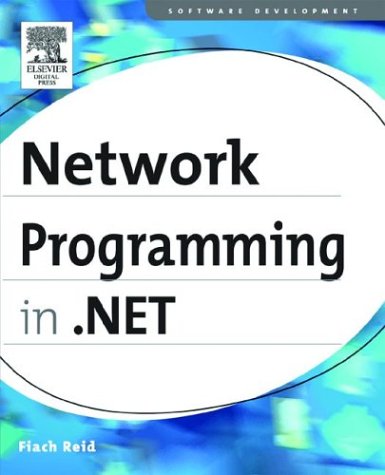
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplatePartAttribute.cs
- ContextMenuStrip.cs
- TraceSection.cs
- XmlDocumentFieldSchema.cs
- MarshalByRefObject.cs
- CompositeFontFamily.cs
- Transform.cs
- SqlTransaction.cs
- BufferedGraphics.cs
- EventLogEntry.cs
- FixedSOMImage.cs
- DataKey.cs
- ProcessRequestArgs.cs
- DataBoundControlDesigner.cs
- StrokeNodeOperations2.cs
- SessionPageStateSection.cs
- ContextMenu.cs
- MarkupCompilePass2.cs
- HandlerMappingMemo.cs
- PasswordTextContainer.cs
- TypeResolvingOptions.cs
- ExpressionContext.cs
- _CommandStream.cs
- SHA256Managed.cs
- InputScopeNameConverter.cs
- BufferedResponseStream.cs
- RequestContext.cs
- HMACSHA512.cs
- Formatter.cs
- MailMessageEventArgs.cs
- DoubleAnimationUsingPath.cs
- ImageListStreamer.cs
- TextSelection.cs
- HtmlInputImage.cs
- TextServicesCompartmentEventSink.cs
- BaseParagraph.cs
- ChangesetResponse.cs
- WorkflowWebHostingModule.cs
- ByteStreamGeometryContext.cs
- RoleGroupCollection.cs
- PropertyMap.cs
- DaylightTime.cs
- Size3DValueSerializer.cs
- CallSiteOps.cs
- WorkflowTraceTransfer.cs
- DataGridViewRowPrePaintEventArgs.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- ProfileSettings.cs
- WindowsFont.cs
- ResXResourceReader.cs
- WebSysDescriptionAttribute.cs
- ZipIOCentralDirectoryFileHeader.cs
- FixedDSBuilder.cs
- XmlStreamStore.cs
- TableRow.cs
- Binding.cs
- TypeConstant.cs
- Grid.cs
- DataSourceCacheDurationConverter.cs
- TableItemPattern.cs
- HtmlInputButton.cs
- ServerValidateEventArgs.cs
- StrokeCollection.cs
- ExpandableObjectConverter.cs
- assertwrapper.cs
- GetPageNumberCompletedEventArgs.cs
- DBDataPermissionAttribute.cs
- TypefaceCollection.cs
- TokenBasedSet.cs
- DragEvent.cs
- EntitySetDataBindingList.cs
- DateTimeOffsetStorage.cs
- CompiledIdentityConstraint.cs
- BinaryCommonClasses.cs
- EntitySqlQueryCacheEntry.cs
- Int64AnimationUsingKeyFrames.cs
- WorkflowMarkupSerializationProvider.cs
- TextOptionsInternal.cs
- ToolBarTray.cs
- NotificationContext.cs
- DbMetaDataCollectionNames.cs
- SecuritySessionSecurityTokenAuthenticator.cs
- ImageMap.cs
- StringWriter.cs
- TransactionTraceIdentifier.cs
- RegisteredScript.cs
- TimelineClockCollection.cs
- LoginView.cs
- PrivateFontCollection.cs
- SvcMapFileLoader.cs
- WorkflowElementDialog.cs
- SchemaElementDecl.cs
- ChtmlTextWriter.cs
- WeakReferenceEnumerator.cs
- TransactionOptions.cs
- ConfigErrorGlyph.cs
- CorrelationActionMessageFilter.cs
- IndependentAnimationStorage.cs
- SecurityTokenRequirement.cs
- SqlFacetAttribute.cs