Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / PtsHost / BaseParagraph.cs / 1 / BaseParagraph.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: BaseParagraph represents a paragraph corresponding to part // of a text based content. // // History: // 05/05/2003 : grzegorz - moving from Avalon branch. // 10/30/2004 : grzegorz - ElementReference cleanup. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using MS.Internal.Documents; using MS.Internal.Text; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { ////// BaseParagraph represents a paragraph corresponding to part of a text /// based content. /// internal abstract class BaseParagraph : UnmanagedHandle { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Constructor. /// /// /// Element associated with paragraph. /// /// /// Content's structural cache /// protected BaseParagraph(DependencyObject element, StructuralCache structuralCache) : base(structuralCache.PtsContext) { _element = element; _structuralCache = structuralCache; _changeType = PTS.FSKCHANGE.fskchNone; _stopAsking = false; UpdateLastFormatPositions(); } #endregion Constructors // ------------------------------------------------------------------ // // PTS callbacks // // ----------------------------------------------------------------- #region PTS callbacks ////// UpdGetParaChange /// /// /// OUT: kind of change /// /// /// OUT: no changes after? /// internal virtual void UpdGetParaChange( out PTS.FSKCHANGE fskch, out int fNoFurtherChanges) { fskch = _changeType; fNoFurtherChanges = PTS.FromBoolean(_stopAsking); #if TEXTPANELLAYOUTDEBUG if (StructuralCache.CurrentFormatContext.IncrementalUpdate) { TextPanelDebug.Log("Para.UpdGetParaChange, Para=" + this.GetType().Name + " Change=" + _changeType.ToString(), TextPanelDebug.Category.ContentChange); } #endif } ////// Collapse margins /// /// /// IN: Paragraph's para client /// /// /// IN: input margin collapsing state /// /// /// IN: current direction (of the track, in which margin collapsing is happening) /// /// /// IN: suppress empty space at the top of page /// /// /// OUT: dvr, calculated based on margin collapsing state /// internal virtual void CollapseMargin( BaseParaClient paraClient, MarginCollapsingState mcs, uint fswdir, bool suppressTopSpace, out int dvr) { // Suppress top space only in paginated scenarios. dvr = (mcs == null || (suppressTopSpace)) ? 0 : mcs.Margin; } ////// Get Para Properties /// /// /// OUT: paragraph properties /// internal abstract void GetParaProperties( ref PTS.FSPAP fspap); ////// CreateParaclient /// /// /// OUT: opaque to PTS paragraph client /// internal abstract void CreateParaclient( out IntPtr pfsparaclient); #endregion PTS callbacks // ------------------------------------------------------------------ // // Internal Methods // // ------------------------------------------------------------------ #region Internal Methods ////// Set update info. Those flags are used later by PTS to decide /// if paragraph needs to be updated and when to stop asking for /// update information. /// /// /// Type of change within the paragraph. /// /// /// Synchronization point is reached? /// internal virtual void SetUpdateInfo(PTS.FSKCHANGE fskch, bool stopAsking) { _changeType = fskch; _stopAsking = stopAsking; } ////// Clear previously accumulated update info. /// internal virtual void ClearUpdateInfo() { _changeType = PTS.FSKCHANGE.fskchNone; _stopAsking = true; } ////// Invalidate content's structural cache. Returns: 'true' if entire paragraph /// is invalid. /// /// /// Position to start invalidation from. /// internal virtual bool InvalidateStructure(int startPosition) { Debug.Assert(ParagraphEndCharacterPosition >= startPosition); int openEdgeCp = TextContainerHelper.GetCPFromElement(StructuralCache.TextContainer, Element, ElementEdge.BeforeStart); return (openEdgeCp == startPosition); } ////// Invalidate accumulated format caches. /// internal virtual void InvalidateFormatCache() { } ////// Update number of characters consumed by the paragraph. /// internal void UpdateLastFormatPositions() { _lastFormatCch = Cch; } #endregion Internal Methods //------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods ////// Retrieve PTS paragraph properties. /// /// /// Paragraph properties to initialize. /// /// /// Ignore element properties? /// protected void GetParaProperties(ref PTS.FSPAP fspap, bool ignoreElementProps) { if (!ignoreElementProps) { fspap.fKeepWithNext = PTS.FromBoolean(DynamicPropertyReader.GetKeepWithNext(_element)); // Can be broken only if Block.BreakPageBefore is set fspap.fBreakPageBefore = _element is Block ? PTS.FromBoolean(StructuralCache.CurrentFormatContext.FinitePage && ((Block)_element).BreakPageBefore) : PTS.FromBoolean(false); // Can be broken only if Block.BreakColumnBefore is set fspap.fBreakColumnBefore = _element is Block ? PTS.FromBoolean(((Block)_element).BreakColumnBefore) : PTS.FromBoolean(false); } } #endregion Protected Methods //------------------------------------------------------------------- // // Properties / Fields // //------------------------------------------------------------------- #region Properties / Fields ////// CharacterPosition at the start of paragraph. /// internal int ParagraphStartCharacterPosition { get { // This is done here, rather than deriving for two reasons - This is special cased for text paragraph - no other // paras should use their cps in this way, and this is also not a virtual method, so can be used from C'Tor. if(this is TextParagraph) { return TextContainerHelper.GetCPFromElement(StructuralCache.TextContainer, Element, ElementEdge.AfterStart); } else { return TextContainerHelper.GetCPFromElement(StructuralCache.TextContainer, Element, ElementEdge.BeforeStart); } } } ////// CharacterPosition at the end of paragraph. The first character /// that does not belong to the paragraph. /// internal int ParagraphEndCharacterPosition { get { // This is done here, rather than deriving for two reasons - This is special cased for text paragraph - no other // paras should use their cps in this way, and this is also not a virtual method, so can be used from C'Tor. if(this is TextParagraph) { return TextContainerHelper.GetCPFromElement(StructuralCache.TextContainer, Element, ElementEdge.BeforeEnd); } else { return TextContainerHelper.GetCPFromElement(StructuralCache.TextContainer, Element, ElementEdge.AfterEnd); } } } ////// Incremental update information for the paragraph. /// Those fields are used only during incremental update process. /// protected PTS.FSKCHANGE _changeType; protected bool _stopAsking; ////// Number of characters consumed by the paragraph. /// internal int Cch { get { int cch = TextContainerHelper.GetCchFromElement(StructuralCache.TextContainer, Element); // This is done here, rather than deriving for two reasons - This is special cased for text paragraph - no other // paras should use their cps in this way, and this is also not a virtual method, so can be used from C'Tor. if (this is TextParagraph && Element is TextElement) { Invariant.Assert(cch >= 2); cch -= 2; } return cch; } } ////// Cch paragraph had during last format /// internal int LastFormatCch { get { return _lastFormatCch; } } protected int _lastFormatCch; ////// The next and previous sibling in paragraph list. /// internal BaseParagraph Next; internal BaseParagraph Previous; ////// Content's structural cache. /// internal StructuralCache StructuralCache { get { return _structuralCache; } } protected readonly StructuralCache _structuralCache; ////// Object associated with the paragraph. /// internal DependencyObject Element { get { return _element; } } protected readonly DependencyObject _element; #endregion Properties / Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // Description: BaseParagraph represents a paragraph corresponding to part // of a text based content. // // History: // 05/05/2003 : grzegorz - moving from Avalon branch. // 10/30/2004 : grzegorz - ElementReference cleanup. // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using MS.Internal.Documents; using MS.Internal.Text; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace MS.Internal.PtsHost { ////// BaseParagraph represents a paragraph corresponding to part of a text /// based content. /// internal abstract class BaseParagraph : UnmanagedHandle { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Constructor. /// /// /// Element associated with paragraph. /// /// /// Content's structural cache /// protected BaseParagraph(DependencyObject element, StructuralCache structuralCache) : base(structuralCache.PtsContext) { _element = element; _structuralCache = structuralCache; _changeType = PTS.FSKCHANGE.fskchNone; _stopAsking = false; UpdateLastFormatPositions(); } #endregion Constructors // ------------------------------------------------------------------ // // PTS callbacks // // ----------------------------------------------------------------- #region PTS callbacks ////// UpdGetParaChange /// /// /// OUT: kind of change /// /// /// OUT: no changes after? /// internal virtual void UpdGetParaChange( out PTS.FSKCHANGE fskch, out int fNoFurtherChanges) { fskch = _changeType; fNoFurtherChanges = PTS.FromBoolean(_stopAsking); #if TEXTPANELLAYOUTDEBUG if (StructuralCache.CurrentFormatContext.IncrementalUpdate) { TextPanelDebug.Log("Para.UpdGetParaChange, Para=" + this.GetType().Name + " Change=" + _changeType.ToString(), TextPanelDebug.Category.ContentChange); } #endif } ////// Collapse margins /// /// /// IN: Paragraph's para client /// /// /// IN: input margin collapsing state /// /// /// IN: current direction (of the track, in which margin collapsing is happening) /// /// /// IN: suppress empty space at the top of page /// /// /// OUT: dvr, calculated based on margin collapsing state /// internal virtual void CollapseMargin( BaseParaClient paraClient, MarginCollapsingState mcs, uint fswdir, bool suppressTopSpace, out int dvr) { // Suppress top space only in paginated scenarios. dvr = (mcs == null || (suppressTopSpace)) ? 0 : mcs.Margin; } ////// Get Para Properties /// /// /// OUT: paragraph properties /// internal abstract void GetParaProperties( ref PTS.FSPAP fspap); ////// CreateParaclient /// /// /// OUT: opaque to PTS paragraph client /// internal abstract void CreateParaclient( out IntPtr pfsparaclient); #endregion PTS callbacks // ------------------------------------------------------------------ // // Internal Methods // // ------------------------------------------------------------------ #region Internal Methods ////// Set update info. Those flags are used later by PTS to decide /// if paragraph needs to be updated and when to stop asking for /// update information. /// /// /// Type of change within the paragraph. /// /// /// Synchronization point is reached? /// internal virtual void SetUpdateInfo(PTS.FSKCHANGE fskch, bool stopAsking) { _changeType = fskch; _stopAsking = stopAsking; } ////// Clear previously accumulated update info. /// internal virtual void ClearUpdateInfo() { _changeType = PTS.FSKCHANGE.fskchNone; _stopAsking = true; } ////// Invalidate content's structural cache. Returns: 'true' if entire paragraph /// is invalid. /// /// /// Position to start invalidation from. /// internal virtual bool InvalidateStructure(int startPosition) { Debug.Assert(ParagraphEndCharacterPosition >= startPosition); int openEdgeCp = TextContainerHelper.GetCPFromElement(StructuralCache.TextContainer, Element, ElementEdge.BeforeStart); return (openEdgeCp == startPosition); } ////// Invalidate accumulated format caches. /// internal virtual void InvalidateFormatCache() { } ////// Update number of characters consumed by the paragraph. /// internal void UpdateLastFormatPositions() { _lastFormatCch = Cch; } #endregion Internal Methods //------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods ////// Retrieve PTS paragraph properties. /// /// /// Paragraph properties to initialize. /// /// /// Ignore element properties? /// protected void GetParaProperties(ref PTS.FSPAP fspap, bool ignoreElementProps) { if (!ignoreElementProps) { fspap.fKeepWithNext = PTS.FromBoolean(DynamicPropertyReader.GetKeepWithNext(_element)); // Can be broken only if Block.BreakPageBefore is set fspap.fBreakPageBefore = _element is Block ? PTS.FromBoolean(StructuralCache.CurrentFormatContext.FinitePage && ((Block)_element).BreakPageBefore) : PTS.FromBoolean(false); // Can be broken only if Block.BreakColumnBefore is set fspap.fBreakColumnBefore = _element is Block ? PTS.FromBoolean(((Block)_element).BreakColumnBefore) : PTS.FromBoolean(false); } } #endregion Protected Methods //------------------------------------------------------------------- // // Properties / Fields // //------------------------------------------------------------------- #region Properties / Fields ////// CharacterPosition at the start of paragraph. /// internal int ParagraphStartCharacterPosition { get { // This is done here, rather than deriving for two reasons - This is special cased for text paragraph - no other // paras should use their cps in this way, and this is also not a virtual method, so can be used from C'Tor. if(this is TextParagraph) { return TextContainerHelper.GetCPFromElement(StructuralCache.TextContainer, Element, ElementEdge.AfterStart); } else { return TextContainerHelper.GetCPFromElement(StructuralCache.TextContainer, Element, ElementEdge.BeforeStart); } } } ////// CharacterPosition at the end of paragraph. The first character /// that does not belong to the paragraph. /// internal int ParagraphEndCharacterPosition { get { // This is done here, rather than deriving for two reasons - This is special cased for text paragraph - no other // paras should use their cps in this way, and this is also not a virtual method, so can be used from C'Tor. if(this is TextParagraph) { return TextContainerHelper.GetCPFromElement(StructuralCache.TextContainer, Element, ElementEdge.BeforeEnd); } else { return TextContainerHelper.GetCPFromElement(StructuralCache.TextContainer, Element, ElementEdge.AfterEnd); } } } ////// Incremental update information for the paragraph. /// Those fields are used only during incremental update process. /// protected PTS.FSKCHANGE _changeType; protected bool _stopAsking; ////// Number of characters consumed by the paragraph. /// internal int Cch { get { int cch = TextContainerHelper.GetCchFromElement(StructuralCache.TextContainer, Element); // This is done here, rather than deriving for two reasons - This is special cased for text paragraph - no other // paras should use their cps in this way, and this is also not a virtual method, so can be used from C'Tor. if (this is TextParagraph && Element is TextElement) { Invariant.Assert(cch >= 2); cch -= 2; } return cch; } } ////// Cch paragraph had during last format /// internal int LastFormatCch { get { return _lastFormatCch; } } protected int _lastFormatCch; ////// The next and previous sibling in paragraph list. /// internal BaseParagraph Next; internal BaseParagraph Previous; ////// Content's structural cache. /// internal StructuralCache StructuralCache { get { return _structuralCache; } } protected readonly StructuralCache _structuralCache; ////// Object associated with the paragraph. /// internal DependencyObject Element { get { return _element; } } protected readonly DependencyObject _element; #endregion Properties / Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
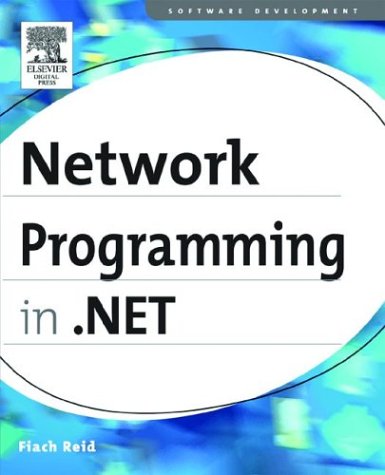
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridColumnCollection.cs
- GradientBrush.cs
- LongCountAggregationOperator.cs
- SqlDataSourceQuery.cs
- CompilationUtil.cs
- DescendantBaseQuery.cs
- ServiceModelEnumValidator.cs
- ServiceDesigner.xaml.cs
- PlatformNotSupportedException.cs
- XslAst.cs
- QueryOutputWriter.cs
- CodeGeneratorAttribute.cs
- OperandQuery.cs
- ResourceBinder.cs
- AliasedExpr.cs
- activationcontext.cs
- Rotation3D.cs
- IisTraceWebEventProvider.cs
- Mouse.cs
- ArgumentNullException.cs
- DecimalAnimation.cs
- BitmapEffectrendercontext.cs
- DataView.cs
- SimpleType.cs
- BoundField.cs
- DetailsViewRow.cs
- ExpressionBuilder.cs
- UnsafeMethods.cs
- BuilderInfo.cs
- ListItemConverter.cs
- MouseBinding.cs
- ConfigurationManagerHelperFactory.cs
- MulticastIPAddressInformationCollection.cs
- NonSerializedAttribute.cs
- SQLMoney.cs
- ChtmlFormAdapter.cs
- DoubleStorage.cs
- MULTI_QI.cs
- _UriSyntax.cs
- ExpandableObjectConverter.cs
- CustomAttribute.cs
- StructuredTypeEmitter.cs
- OperationCanceledException.cs
- _FtpDataStream.cs
- Solver.cs
- GestureRecognitionResult.cs
- MailHeaderInfo.cs
- PixelFormats.cs
- Cloud.cs
- List.cs
- HtmlInputControl.cs
- CheckBoxStandardAdapter.cs
- GroupBoxRenderer.cs
- ProcessInfo.cs
- GeneralTransformGroup.cs
- ToolStripStatusLabel.cs
- EntityClientCacheEntry.cs
- DataGridRow.cs
- ExpanderAutomationPeer.cs
- MediaTimeline.cs
- DayRenderEvent.cs
- DbFunctionCommandTree.cs
- IArgumentProvider.cs
- _ChunkParse.cs
- ConstraintConverter.cs
- OperationSelectorBehavior.cs
- OdbcPermission.cs
- PriorityChain.cs
- CommandConverter.cs
- InvokeProviderWrapper.cs
- TransformerTypeCollection.cs
- ReceiveReply.cs
- _ConnectOverlappedAsyncResult.cs
- Bitmap.cs
- PanelContainerDesigner.cs
- BrowsableAttribute.cs
- CommandBinding.cs
- IdnElement.cs
- ObjectDataSourceDisposingEventArgs.cs
- KnownColorTable.cs
- RoutedEventArgs.cs
- MetadataArtifactLoaderResource.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- CreateUserWizard.cs
- FollowerQueueCreator.cs
- ScaleTransform3D.cs
- OutputCacheSettingsSection.cs
- StrongNameUtility.cs
- XmlSchemaSimpleContentExtension.cs
- XmlnsPrefixAttribute.cs
- TcpConnectionPoolSettings.cs
- StrokeNodeOperations.cs
- SQLInt16.cs
- ExpandedWrapper.cs
- SqlDataSourceEnumerator.cs
- SharedDp.cs
- WebHttpBindingCollectionElement.cs
- UIElementAutomationPeer.cs
- DataExpression.cs
- FixedTextSelectionProcessor.cs