Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Design / Glyphs / ConfigErrorGlyph.cs / 1305376 / ConfigErrorGlyph.cs
namespace System.Workflow.ComponentModel.Design { using System; using System.Drawing; using System.Drawing.Drawing2D; using System.Windows.Forms; #region Glyphs #region Class ConfigErrorGlyph //Class is internal but not sealed as we dont expect the ActivityDesigner writers to supply their own //Glyph instead based on designer actions the smart tag will be shown //Exception: StripItemConfigErrorGlyph public class ConfigErrorGlyph : DesignerGlyph { private static ConfigErrorGlyph defaultConfigErrorGlyph = null; internal static ConfigErrorGlyph Default { get { if (defaultConfigErrorGlyph == null) defaultConfigErrorGlyph = new ConfigErrorGlyph(); return defaultConfigErrorGlyph; } } public override bool CanBeActivated { get { return true; } } public override int Priority { get { return DesignerGlyph.ConfigErrorPriority; } } public override Rectangle GetBounds(ActivityDesigner designer, bool activated) { if (designer == null) throw new ArgumentNullException("designer"); Size configErrorSize = WorkflowTheme.CurrentTheme.AmbientTheme.GlyphSize; Size margin = WorkflowTheme.CurrentTheme.AmbientTheme.Margin; Point configErrorLocation = new Point(designer.Bounds.Right - configErrorSize.Width - margin.Width / 2, designer.Bounds.Top - configErrorSize.Height + margin.Height); Rectangle bounds = new Rectangle(configErrorLocation, configErrorSize); if (activated) { bounds.Width *= 2; AmbientTheme ambientTheme = WorkflowTheme.CurrentTheme.AmbientTheme; bounds.Inflate(ambientTheme.Margin.Width / 2, ambientTheme.Margin.Height / 2); } return bounds; } protected override void OnPaint(Graphics graphics, bool activated, AmbientTheme ambientTheme, ActivityDesigner designer) { Rectangle bounds = GetBounds(designer, false); Rectangle activatedBounds = GetBounds(designer, activated); Region clipRegion = null; Region oldClipRegion = graphics.Clip; try { if (oldClipRegion != null) { clipRegion = oldClipRegion.Clone(); if (activated) clipRegion.Union(activatedBounds); graphics.Clip = clipRegion; } if (activated) { graphics.FillRectangle(SystemBrushes.ButtonFace, activatedBounds); graphics.DrawRectangle(SystemPens.ControlDarkDark, activatedBounds.Left, activatedBounds.Top, activatedBounds.Width - 1, activatedBounds.Height - 1); activatedBounds.X += bounds.Width + ambientTheme.Margin.Width; activatedBounds.Width -= (bounds.Width + 2 * ambientTheme.Margin.Width); using (GraphicsPath dropDownIndicator = ActivityDesignerPaint.GetScrollIndicatorPath(activatedBounds, ScrollButton.Down)) { graphics.FillPath(SystemBrushes.ControlText, dropDownIndicator); graphics.DrawPath(SystemPens.ControlText, dropDownIndicator); } } ActivityDesignerPaint.DrawImage(graphics, AmbientTheme.ConfigErrorImage, bounds, DesignerContentAlignment.Fill); } finally { if (clipRegion != null) { graphics.Clip = oldClipRegion; clipRegion.Dispose(); } } } protected override void OnActivate(ActivityDesigner designer) { if (designer != null) { if (designer.DesignerActions.Count > 0) { Rectangle bounds = GetBounds(designer, false); Point location = designer.ParentView.LogicalPointToScreen(new Point(bounds.Left, bounds.Bottom)); DesignerHelpers.ShowDesignerVerbs(designer, location, DesignerHelpers.GetDesignerActionVerbs(designer, designer.DesignerActions)); } } } } #endregion #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
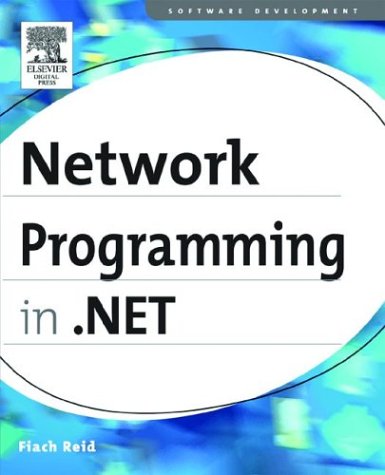
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DbMetaDataFactory.cs
- InternalResources.cs
- ExpandCollapseProviderWrapper.cs
- _CookieModule.cs
- DelegatingTypeDescriptionProvider.cs
- ProfileManager.cs
- DrawingImage.cs
- TextWriterEngine.cs
- FromReply.cs
- ApplicationServicesHostFactory.cs
- ToggleButton.cs
- UserNameSecurityTokenAuthenticator.cs
- CodeDOMProvider.cs
- EntityProxyTypeInfo.cs
- Debug.cs
- DataGridColumnFloatingHeader.cs
- Binding.cs
- ButtonField.cs
- FileSystemEventArgs.cs
- SoapEnumAttribute.cs
- PrintPreviewControl.cs
- __ConsoleStream.cs
- ProxyHwnd.cs
- DataGridViewTextBoxEditingControl.cs
- ColorBlend.cs
- DependencyObjectValidator.cs
- WebServiceClientProxyGenerator.cs
- XmlSchemaAttribute.cs
- SoapReflectionImporter.cs
- Panel.cs
- ProbeDuplexCD1AsyncResult.cs
- NotifyCollectionChangedEventArgs.cs
- AuthenticationSection.cs
- XAMLParseException.cs
- XmlMapping.cs
- SQLInt64Storage.cs
- BroadcastEventHelper.cs
- MiniConstructorInfo.cs
- input.cs
- GroupBoxAutomationPeer.cs
- cookieexception.cs
- NTAccount.cs
- Baml2006KeyRecord.cs
- SiteMapNode.cs
- LineVisual.cs
- RegexInterpreter.cs
- InputGestureCollection.cs
- WindowsFormsHelpers.cs
- PropertyRecord.cs
- SrgsRule.cs
- StrokeNodeData.cs
- PageFunction.cs
- OleDbCommand.cs
- TypefaceCollection.cs
- SafeWaitHandle.cs
- HttpWebRequest.cs
- UnsafeNativeMethods.cs
- ApplicationException.cs
- ListControlActionList.cs
- TextSelectionHelper.cs
- ContainerTracking.cs
- DoubleCollection.cs
- XdrBuilder.cs
- OperationCanceledException.cs
- Deflater.cs
- CryptoApi.cs
- UdpTransportBindingElement.cs
- DataGridViewCellMouseEventArgs.cs
- SecureUICommand.cs
- HandoffBehavior.cs
- HatchBrush.cs
- IdentityNotMappedException.cs
- UpWmlPageAdapter.cs
- SmtpMail.cs
- CodeIdentifiers.cs
- DataColumnPropertyDescriptor.cs
- GestureRecognizer.cs
- CommonDialog.cs
- StaticResourceExtension.cs
- UntypedNullExpression.cs
- MetadataArtifactLoaderCompositeFile.cs
- SelectionEditor.cs
- MatrixTransform3D.cs
- RealProxy.cs
- CellTreeNode.cs
- HtmlPageAdapter.cs
- DiscoveryDocumentReference.cs
- Transaction.cs
- TextTreeObjectNode.cs
- DataPagerField.cs
- ExpressionBuilderCollection.cs
- PropertyConverter.cs
- ErrorFormatterPage.cs
- ItemAutomationPeer.cs
- TextDocumentView.cs
- MimeAnyImporter.cs
- VisualProxy.cs
- CompiledAction.cs
- WebBrowserHelper.cs
- SemanticBasicElement.cs