Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Recovery / LogEntryUtils.cs / 1 / LogEntryUtils.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // This file contains useful routines for serialization and deserialization using System; using System.IO; using System.ServiceModel; using System.ServiceModel.Channels; using System.Text; using System.Xml; using Microsoft.Transactions.Bridge; using Microsoft.Transactions.Wsat.Messaging; using Microsoft.Transactions.Wsat.Protocol; using DiagnosticUtility = Microsoft.Transactions.Bridge.DiagnosticUtility; namespace Microsoft.Transactions.Wsat.Recovery { static class SerializationUtils { public static byte ReadByte(MemoryStream mem) { int value = mem.ReadByte(); if (value == -1) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.DeserializationDataCorrupt))); } return (byte) value; } public static void WriteEndpointAddress(MemoryStream mem, EndpointAddress address, ProtocolVersion protocolVersion) { try { XmlDictionaryWriter writer = XmlDictionaryWriter.CreateBinaryWriter(mem, BinaryMessageEncoderFactory.XmlDictionary); address.WriteTo(MessagingVersionHelper.AddressingVersion(protocolVersion), writer); writer.Flush(); } catch (XmlException e) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.EndpointReferenceSerializationFailed), e)); } } public static EndpointAddress ReadEndpointAddress(MemoryStream mem, ProtocolVersion protocolVersion) { try { XmlDictionaryReader reader = XmlDictionaryReader.CreateBinaryReader(mem, BinaryMessageEncoderFactory.XmlDictionary, XmlDictionaryReaderQuotas.Max); return EndpointAddress.ReadFrom(MessagingVersionHelper.AddressingVersion(protocolVersion), reader); } catch (XmlException e) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.DeserializationDataCorrupt), e)); } } public static void WriteString(MemoryStream mem, string value) { byte[] buffer = Encoding.UTF8.GetBytes(value); if (buffer.Length > ushort.MaxValue) { // MSDTC assumes that all strings will be under 64K in length. Violation of this // assumption creates an invalid log entry. The safest thing to do is crash // and write nothing at all. DiagnosticUtility.FailFast("Serializing a string that is too long"); } WriteUShort(mem, (ushort) buffer.Length); mem.Write(buffer, 0, buffer.Length); } public static string ReadString(MemoryStream mem) { long length = ReadUShort(mem); if (mem.Length - length < mem.Position) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.DeserializationDataCorrupt))); } string value; try { value = Encoding.UTF8.GetString(mem.GetBuffer(), (int)mem.Position, (int)length); } catch (ArgumentOutOfRangeException e) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.DeserializationDataCorrupt), e)); } IncrementPosition(mem, length); return value; } public static void WriteUShort(MemoryStream mem, ushort value) { byte[] buffer = BitConverter.GetBytes(value); mem.Write(buffer, 0, buffer.Length); } public static ushort ReadUShort(MemoryStream mem) { if (mem.Length - 2 < mem.Position) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.DeserializationDataCorrupt))); } ushort value = BitConverter.ToUInt16(mem.GetBuffer(), (int) mem.Position); IncrementPosition(mem, 2); return value; } public static void WriteInt(MemoryStream mem, int value) { byte[] buffer = BitConverter.GetBytes(value); mem.Write(buffer, 0, buffer.Length); } public static int ReadInt(MemoryStream mem) { if (mem.Length - 4 < mem.Position) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.DeserializationDataCorrupt))); } int value = BitConverter.ToInt32(mem.GetBuffer(), (int) mem.Position); IncrementPosition(mem, 4); return value; } public static void WriteGuid(MemoryStream mem, ref Guid value) { byte[] buffer = value.ToByteArray(); mem.Write(buffer, 0, buffer.Length); } public static Guid ReadGuid(MemoryStream mem) { if (mem.Length - 16 < mem.Position) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.DeserializationDataCorrupt))); } byte[] buffer = new byte[16]; Array.Copy(mem.GetBuffer(), mem.Position, buffer, 0, 16); IncrementPosition(mem, 16); return new Guid(buffer); } public static void WriteUInt(MemoryStream mem, uint value) { byte[] buffer = BitConverter.GetBytes(value); mem.Write(buffer, 0, buffer.Length); } public static uint ReadUInt(MemoryStream mem) { if (mem.Length - 4 < mem.Position) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.DeserializationDataCorrupt))); } uint value = BitConverter.ToUInt32(mem.GetBuffer(), (int)mem.Position); IncrementPosition(mem, 4); return value; } public static void WriteTimeout(MemoryStream mem, TimeSpan span) { WriteUInt(mem, (uint)span.TotalSeconds); } public static TimeSpan ReadTimeout(MemoryStream mem) { return TimeSpan.FromSeconds(ReadUInt(mem)); } public static byte[] ReadBytes(MemoryStream mem, int bytes) { if (mem.Length < bytes || mem.Length - bytes < mem.Position) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.DeserializationDataCorrupt))); } byte[] buffer = new byte[bytes]; mem.Read(buffer, 0, buffer.Length); return buffer; } public static void AlignPosition(MemoryStream mem, int alignment) { int lowerBits = alignment - 1; long newPosition = (mem.Position + lowerBits) & ~lowerBits; if (mem.Position > newPosition || mem.Length < newPosition) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.DeserializationDataCorrupt))); } mem.Position = newPosition; } public static void IncrementPosition(MemoryStream mem, long increment) { if (mem.Length < increment || mem.Length - increment < mem.Position) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new SerializationException(SR.GetString(SR.DeserializationDataCorrupt))); } mem.Position += increment; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
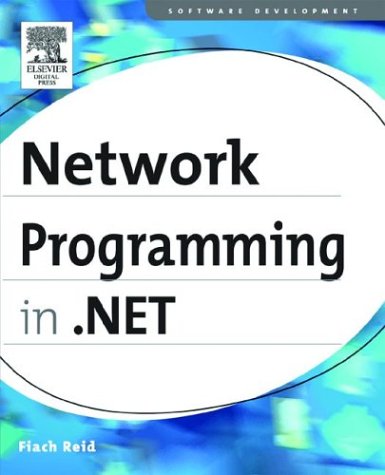
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- codemethodreferenceexpression.cs
- RegisteredExpandoAttribute.cs
- XmlDataDocument.cs
- HandlerMappingMemo.cs
- DataObjectMethodAttribute.cs
- Maps.cs
- MediaContext.cs
- UiaCoreTypesApi.cs
- AutoResetEvent.cs
- DataGridHeaderBorder.cs
- InProcStateClientManager.cs
- DecimalAnimation.cs
- BindingExpressionBase.cs
- VectorKeyFrameCollection.cs
- EllipseGeometry.cs
- SourceSwitch.cs
- DataGridViewColumnEventArgs.cs
- PageAsyncTaskManager.cs
- Configuration.cs
- DependencyObjectCodeDomSerializer.cs
- InkCanvasFeedbackAdorner.cs
- Calendar.cs
- MSAANativeProvider.cs
- DataFormats.cs
- RegexRunner.cs
- NodeInfo.cs
- TextModifier.cs
- XmlDataSource.cs
- VisualTreeUtils.cs
- BooleanAnimationBase.cs
- MethodCallConverter.cs
- EditorBrowsableAttribute.cs
- ReferenceConverter.cs
- ClosableStream.cs
- OdbcConnectionStringbuilder.cs
- SqlDataSourceCommandEventArgs.cs
- PackWebRequestFactory.cs
- SpoolingTask.cs
- Debugger.cs
- XMLUtil.cs
- DataGridRowsPresenter.cs
- ThrowOnMultipleAssignment.cs
- InfoCardKeyedHashAlgorithm.cs
- InfiniteIntConverter.cs
- PageContentCollection.cs
- SecureStringHasher.cs
- Control.cs
- ItemCheckedEvent.cs
- LogWriteRestartAreaAsyncResult.cs
- Zone.cs
- PointAnimationBase.cs
- XmlSchemaGroupRef.cs
- SqlCommandSet.cs
- TextBoxLine.cs
- SplitterEvent.cs
- EntityModelSchemaGenerator.cs
- OptimizerPatterns.cs
- UnmanagedHandle.cs
- EncodingInfo.cs
- RecipientInfo.cs
- NullableConverter.cs
- TcpConnectionPoolSettingsElement.cs
- PostBackOptions.cs
- NamedObject.cs
- XmlRawWriter.cs
- Matrix.cs
- ClientBuildManagerCallback.cs
- TextUtf8RawTextWriter.cs
- ToolStripDropTargetManager.cs
- WpfMemberInvoker.cs
- DatagridviewDisplayedBandsData.cs
- EntityDataSource.cs
- x509utils.cs
- ToolStripContentPanelRenderEventArgs.cs
- DataMember.cs
- ScriptingScriptResourceHandlerSection.cs
- ImpersonateTokenRef.cs
- Parameter.cs
- TraceContextEventArgs.cs
- SparseMemoryStream.cs
- SystemIPInterfaceStatistics.cs
- TypeLibConverter.cs
- IisTraceWebEventProvider.cs
- EnumConverter.cs
- ArglessEventHandlerProxy.cs
- Table.cs
- Nullable.cs
- DSASignatureFormatter.cs
- ArrayElementGridEntry.cs
- NativeCompoundFileAPIs.cs
- DataContractSerializerOperationGenerator.cs
- NavigationPropertyEmitter.cs
- While.cs
- DataListItemEventArgs.cs
- NativeWrapper.cs
- StagingAreaInputItem.cs
- DependencyObjectValidator.cs
- StorageAssociationSetMapping.cs
- OleDbDataAdapter.cs
- Trustee.cs