Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / UI / PostBackOptions.cs / 1 / PostBackOptions.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * PostBackOptions class definition * * Copyright (c) 2003 Microsoft Corporation */ namespace System.Web.UI { using System; using System.ComponentModel; using System.Text; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class PostBackOptions { private string _actionUrl; private string _argument; private string _validationGroup; private bool _autoPostBack; private bool _requiresJavaScriptProtocol; private bool _performValidation; private bool _trackFocus; private bool _clientSubmit = true; private Control _targetControl; public PostBackOptions(Control targetControl) : this(targetControl, null, null, false, false, false, true, false, null) { } public PostBackOptions(Control targetControl, string argument) : this(targetControl, argument, null, false, false, false, true, false, null) { } public PostBackOptions(Control targetControl, string argument, string actionUrl, bool autoPostBack, bool requiresJavaScriptProtocol, bool trackFocus, bool clientSubmit, bool performValidation, string validationGroup) { if (targetControl == null) throw new ArgumentNullException("targetControl"); _actionUrl = actionUrl; _argument = argument; _autoPostBack = autoPostBack; _clientSubmit = clientSubmit; _requiresJavaScriptProtocol = requiresJavaScriptProtocol; _performValidation = performValidation; _trackFocus = trackFocus; _targetControl = targetControl; _validationGroup = validationGroup; } [DefaultValue("")] public string ActionUrl { get { return _actionUrl; } set { _actionUrl = value; } } [DefaultValue("")] public string Argument { get { return _argument; } set { _argument = value; } } [DefaultValue(false)] public bool AutoPostBack { get { return _autoPostBack; } set { _autoPostBack = value; } } [DefaultValue(true)] public bool ClientSubmit { get { return _clientSubmit; } set { _clientSubmit = value; } } [DefaultValue(true)] public bool RequiresJavaScriptProtocol { get { return _requiresJavaScriptProtocol; } set { _requiresJavaScriptProtocol = value; } } [DefaultValue(false)] public bool PerformValidation { get { return _performValidation; } set { _performValidation = value; } } [DefaultValue("")] public string ValidationGroup { get { return _validationGroup; } set { _validationGroup = value; } } [DefaultValue(null)] public Control TargetControl { get { return _targetControl; } } [DefaultValue(false)] public bool TrackFocus { get { return _trackFocus; } set { _trackFocus = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * PostBackOptions class definition * * Copyright (c) 2003 Microsoft Corporation */ namespace System.Web.UI { using System; using System.ComponentModel; using System.Text; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class PostBackOptions { private string _actionUrl; private string _argument; private string _validationGroup; private bool _autoPostBack; private bool _requiresJavaScriptProtocol; private bool _performValidation; private bool _trackFocus; private bool _clientSubmit = true; private Control _targetControl; public PostBackOptions(Control targetControl) : this(targetControl, null, null, false, false, false, true, false, null) { } public PostBackOptions(Control targetControl, string argument) : this(targetControl, argument, null, false, false, false, true, false, null) { } public PostBackOptions(Control targetControl, string argument, string actionUrl, bool autoPostBack, bool requiresJavaScriptProtocol, bool trackFocus, bool clientSubmit, bool performValidation, string validationGroup) { if (targetControl == null) throw new ArgumentNullException("targetControl"); _actionUrl = actionUrl; _argument = argument; _autoPostBack = autoPostBack; _clientSubmit = clientSubmit; _requiresJavaScriptProtocol = requiresJavaScriptProtocol; _performValidation = performValidation; _trackFocus = trackFocus; _targetControl = targetControl; _validationGroup = validationGroup; } [DefaultValue("")] public string ActionUrl { get { return _actionUrl; } set { _actionUrl = value; } } [DefaultValue("")] public string Argument { get { return _argument; } set { _argument = value; } } [DefaultValue(false)] public bool AutoPostBack { get { return _autoPostBack; } set { _autoPostBack = value; } } [DefaultValue(true)] public bool ClientSubmit { get { return _clientSubmit; } set { _clientSubmit = value; } } [DefaultValue(true)] public bool RequiresJavaScriptProtocol { get { return _requiresJavaScriptProtocol; } set { _requiresJavaScriptProtocol = value; } } [DefaultValue(false)] public bool PerformValidation { get { return _performValidation; } set { _performValidation = value; } } [DefaultValue("")] public string ValidationGroup { get { return _validationGroup; } set { _validationGroup = value; } } [DefaultValue(null)] public Control TargetControl { get { return _targetControl; } } [DefaultValue(false)] public bool TrackFocus { get { return _trackFocus; } set { _trackFocus = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
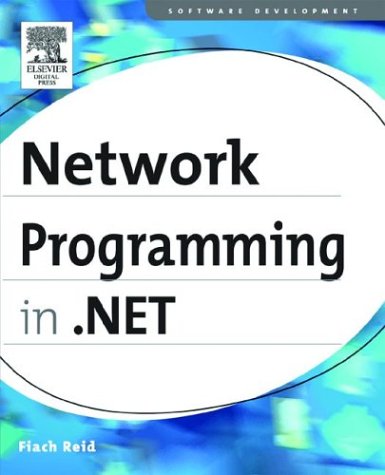
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SerialPort.cs
- NameValueFileSectionHandler.cs
- Context.cs
- AnimatedTypeHelpers.cs
- QueueProcessor.cs
- XamlGridLengthSerializer.cs
- ImageListUtils.cs
- diagnosticsswitches.cs
- CommandField.cs
- SafeRegistryKey.cs
- TransformerConfigurationWizardBase.cs
- AffineTransform3D.cs
- MemberDomainMap.cs
- TabletDevice.cs
- ObjectListShowCommandsEventArgs.cs
- ProjectionPath.cs
- CodeNamespace.cs
- KeyGesture.cs
- DetailsViewDeletedEventArgs.cs
- PriorityRange.cs
- DropShadowEffect.cs
- TreeViewBindingsEditorForm.cs
- ReflectionPermission.cs
- DrawingVisual.cs
- ClosableStream.cs
- ToolStrip.cs
- ObjectKeyFrameCollection.cs
- TextParaLineResult.cs
- IResourceProvider.cs
- MemoryStream.cs
- AliasGenerator.cs
- WorkflowDesigner.cs
- SrgsDocument.cs
- PtsHelper.cs
- WebUtil.cs
- DiscoveryExceptionDictionary.cs
- MimeReflector.cs
- WebEventCodes.cs
- ListControl.cs
- SoapAttributeAttribute.cs
- ToggleProviderWrapper.cs
- DataObjectCopyingEventArgs.cs
- DesignOnlyAttribute.cs
- AssemblyBuilder.cs
- SqlEnums.cs
- KeyboardEventArgs.cs
- WCFBuildProvider.cs
- HttpFormatExtensions.cs
- RelationshipConstraintValidator.cs
- ActivityTypeResolver.xaml.cs
- RotateTransform3D.cs
- _BaseOverlappedAsyncResult.cs
- XmlSerializerVersionAttribute.cs
- ApplicationInfo.cs
- MailAddress.cs
- UserControl.cs
- SelectionEditingBehavior.cs
- SQLGuid.cs
- ComplusTypeValidator.cs
- WindowsFormsHost.cs
- FunctionNode.cs
- RawMouseInputReport.cs
- IgnoreDataMemberAttribute.cs
- RijndaelManagedTransform.cs
- QilXmlReader.cs
- MenuItemBinding.cs
- ChangePasswordDesigner.cs
- SHA1.cs
- DataGridRowHeaderAutomationPeer.cs
- HtmlTable.cs
- DbgCompiler.cs
- ChameleonKey.cs
- GestureRecognitionResult.cs
- PersonalizationProviderCollection.cs
- SqlConnectionHelper.cs
- ParallelLoopState.cs
- DataGridCommandEventArgs.cs
- DropDownList.cs
- OperationParameterInfoCollection.cs
- CodeDelegateInvokeExpression.cs
- _LoggingObject.cs
- XmlAttributeAttribute.cs
- DataControlCommands.cs
- ConfigurationManagerHelper.cs
- ApplicationTrust.cs
- AstTree.cs
- LowerCaseStringConverter.cs
- UInt16.cs
- ReferenceEqualityComparer.cs
- EventHandlerList.cs
- SemanticKeyElement.cs
- XmlDataLoader.cs
- HttpListenerResponse.cs
- Solver.cs
- RayHitTestParameters.cs
- OleServicesContext.cs
- _ChunkParse.cs
- SqlCaseSimplifier.cs
- WinFormsComponentEditor.cs
- StorageRoot.cs