Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / System / Windows / Media / Generated / Matrix.cs / 1 / Matrix.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.WindowsBase; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Windows; using System.Windows.Media; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { [Serializable] [TypeConverter(typeof(MatrixConverter))] [ValueSerializer(typeof(MatrixValueSerializer))] // Used by MarkupWriter partial struct Matrix : IFormattable { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Compares two Matrix instances for exact equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Matrix instances are exactly equal, false otherwise /// /// The first Matrix to compare /// The second Matrix to compare public static bool operator == (Matrix matrix1, Matrix matrix2) { if (matrix1.IsDistinguishedIdentity || matrix2.IsDistinguishedIdentity) { return matrix1.IsIdentity == matrix2.IsIdentity; } else { return matrix1.M11 == matrix2.M11 && matrix1.M12 == matrix2.M12 && matrix1.M21 == matrix2.M21 && matrix1.M22 == matrix2.M22 && matrix1.OffsetX == matrix2.OffsetX && matrix1.OffsetY == matrix2.OffsetY; } } ////// Compares two Matrix instances for exact inequality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Matrix instances are exactly unequal, false otherwise /// /// The first Matrix to compare /// The second Matrix to compare public static bool operator != (Matrix matrix1, Matrix matrix2) { return !(matrix1 == matrix2); } ////// Compares two Matrix instances for object equality. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the two Matrix instances are exactly equal, false otherwise /// /// The first Matrix to compare /// The second Matrix to compare public static bool Equals (Matrix matrix1, Matrix matrix2) { if (matrix1.IsDistinguishedIdentity || matrix2.IsDistinguishedIdentity) { return matrix1.IsIdentity == matrix2.IsIdentity; } else { return matrix1.M11.Equals(matrix2.M11) && matrix1.M12.Equals(matrix2.M12) && matrix1.M21.Equals(matrix2.M21) && matrix1.M22.Equals(matrix2.M22) && matrix1.OffsetX.Equals(matrix2.OffsetX) && matrix1.OffsetY.Equals(matrix2.OffsetY); } } ////// Equals - compares this Matrix with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the object is an instance of Matrix and if it's equal to "this". /// /// The object to compare to "this" public override bool Equals(object o) { if ((null == o) || !(o is Matrix)) { return false; } Matrix value = (Matrix)o; return Matrix.Equals(this,value); } ////// Equals - compares this Matrix with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if "value" is equal to "this". /// /// The Matrix to compare to "this" public bool Equals(Matrix value) { return Matrix.Equals(this, value); } ////// Returns the HashCode for this Matrix /// ////// int - the HashCode for this Matrix /// public override int GetHashCode() { if (IsDistinguishedIdentity) { return c_identityHashCode; } else { // Perform field-by-field XOR of HashCodes return M11.GetHashCode() ^ M12.GetHashCode() ^ M21.GetHashCode() ^ M22.GetHashCode() ^ OffsetX.GetHashCode() ^ OffsetY.GetHashCode(); } } ////// Parse - returns an instance converted from the provided string using /// the culture "en-US" /// string with Matrix data /// public static Matrix Parse(string source) { IFormatProvider formatProvider = CultureInfo.GetCultureInfo("en-us"); TokenizerHelper th = new TokenizerHelper(source, formatProvider); Matrix value; String firstToken = th.NextTokenRequired(); // The token will already have had whitespace trimmed so we can do a // simple string compare. if (firstToken == "Identity") { value = Identity; } else { value = new Matrix( Convert.ToDouble(firstToken, formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider)); } // There should be no more tokens in this string. th.LastTokenRequired(); return value; } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Creates a string representation of this object based on the current culture. /// ////// A string representation of this object. /// public override string ToString() { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, null /* format provider */); } ////// Creates a string representation of this object based on the IFormatProvider /// passed in. If the provider is null, the CurrentCulture is used. /// ////// A string representation of this object. /// public string ToString(IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal string ConvertToString(string format, IFormatProvider provider) { if (IsIdentity) { return "Identity"; } // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "{1:" + format + "}{0}{2:" + format + "}{0}{3:" + format + "}{0}{4:" + format + "}{0}{5:" + format + "}{0}{6:" + format + "}", separator, _m11, _m12, _m21, _m22, _offsetX, _offsetY); } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.WindowsBase; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Windows; using System.Windows.Media; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { [Serializable] [TypeConverter(typeof(MatrixConverter))] [ValueSerializer(typeof(MatrixValueSerializer))] // Used by MarkupWriter partial struct Matrix : IFormattable { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Compares two Matrix instances for exact equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Matrix instances are exactly equal, false otherwise /// /// The first Matrix to compare /// The second Matrix to compare public static bool operator == (Matrix matrix1, Matrix matrix2) { if (matrix1.IsDistinguishedIdentity || matrix2.IsDistinguishedIdentity) { return matrix1.IsIdentity == matrix2.IsIdentity; } else { return matrix1.M11 == matrix2.M11 && matrix1.M12 == matrix2.M12 && matrix1.M21 == matrix2.M21 && matrix1.M22 == matrix2.M22 && matrix1.OffsetX == matrix2.OffsetX && matrix1.OffsetY == matrix2.OffsetY; } } ////// Compares two Matrix instances for exact inequality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which are logically equal may fail. /// Furthermore, using this equality operator, Double.NaN is not equal to itself. /// ////// bool - true if the two Matrix instances are exactly unequal, false otherwise /// /// The first Matrix to compare /// The second Matrix to compare public static bool operator != (Matrix matrix1, Matrix matrix2) { return !(matrix1 == matrix2); } ////// Compares two Matrix instances for object equality. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the two Matrix instances are exactly equal, false otherwise /// /// The first Matrix to compare /// The second Matrix to compare public static bool Equals (Matrix matrix1, Matrix matrix2) { if (matrix1.IsDistinguishedIdentity || matrix2.IsDistinguishedIdentity) { return matrix1.IsIdentity == matrix2.IsIdentity; } else { return matrix1.M11.Equals(matrix2.M11) && matrix1.M12.Equals(matrix2.M12) && matrix1.M21.Equals(matrix2.M21) && matrix1.M22.Equals(matrix2.M22) && matrix1.OffsetX.Equals(matrix2.OffsetX) && matrix1.OffsetY.Equals(matrix2.OffsetY); } } ////// Equals - compares this Matrix with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if the object is an instance of Matrix and if it's equal to "this". /// /// The object to compare to "this" public override bool Equals(object o) { if ((null == o) || !(o is Matrix)) { return false; } Matrix value = (Matrix)o; return Matrix.Equals(this,value); } ////// Equals - compares this Matrix with the passed in object. In this equality /// Double.NaN is equal to itself, unlike in numeric equality. /// Note that double values can acquire error when operated upon, such that /// an exact comparison between two values which /// are logically equal may fail. /// ////// bool - true if "value" is equal to "this". /// /// The Matrix to compare to "this" public bool Equals(Matrix value) { return Matrix.Equals(this, value); } ////// Returns the HashCode for this Matrix /// ////// int - the HashCode for this Matrix /// public override int GetHashCode() { if (IsDistinguishedIdentity) { return c_identityHashCode; } else { // Perform field-by-field XOR of HashCodes return M11.GetHashCode() ^ M12.GetHashCode() ^ M21.GetHashCode() ^ M22.GetHashCode() ^ OffsetX.GetHashCode() ^ OffsetY.GetHashCode(); } } ////// Parse - returns an instance converted from the provided string using /// the culture "en-US" /// string with Matrix data /// public static Matrix Parse(string source) { IFormatProvider formatProvider = CultureInfo.GetCultureInfo("en-us"); TokenizerHelper th = new TokenizerHelper(source, formatProvider); Matrix value; String firstToken = th.NextTokenRequired(); // The token will already have had whitespace trimmed so we can do a // simple string compare. if (firstToken == "Identity") { value = Identity; } else { value = new Matrix( Convert.ToDouble(firstToken, formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider), Convert.ToDouble(th.NextTokenRequired(), formatProvider)); } // There should be no more tokens in this string. th.LastTokenRequired(); return value; } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties #endregion Public Properties //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ #region Protected Methods #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Creates a string representation of this object based on the current culture. /// ////// A string representation of this object. /// public override string ToString() { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, null /* format provider */); } ////// Creates a string representation of this object based on the IFormatProvider /// passed in. If the provider is null, the CurrentCulture is used. /// ////// A string representation of this object. /// public string ToString(IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null /* format string */, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal string ConvertToString(string format, IFormatProvider provider) { if (IsIdentity) { return "Identity"; } // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "{1:" + format + "}{0}{2:" + format + "}{0}{3:" + format + "}{0}{4:" + format + "}{0}{5:" + format + "}{0}{6:" + format + "}", separator, _m11, _m12, _m21, _m22, _offsetX, _offsetY); } #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties #endregion Dependency Properties //----------------------------------------------------- // // Internal Fields // //------------------------------------------------------ #region Internal Fields #endregion Internal Fields #region Constructors //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #endregion Constructors } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
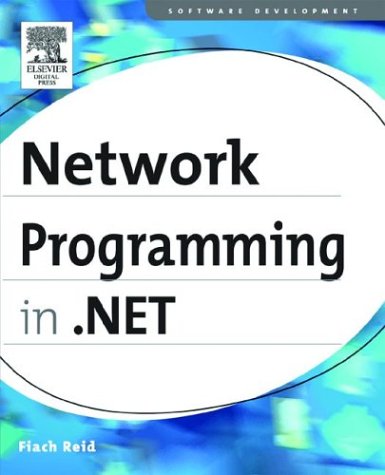
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventLogTraceListener.cs
- AlternateViewCollection.cs
- SubclassTypeValidator.cs
- CustomBindingCollectionElement.cs
- XmlSchemaImporter.cs
- CatalogZoneBase.cs
- SspiNegotiationTokenProviderState.cs
- DesignBindingConverter.cs
- EventPrivateKey.cs
- SiteMapSection.cs
- AnnotationObservableCollection.cs
- PageCache.cs
- SelfIssuedAuthRSAPKCS1SignatureFormatter.cs
- ObservableDictionary.cs
- Translator.cs
- ParserStreamGeometryContext.cs
- Helpers.cs
- SafeNativeMethodsMilCoreApi.cs
- InnerItemCollectionView.cs
- DoubleLinkList.cs
- Process.cs
- DisplayInformation.cs
- DoWhile.cs
- Constants.cs
- BaseTemplatedMobileComponentEditor.cs
- PerfCounters.cs
- SqlFlattener.cs
- FormCollection.cs
- EmbossBitmapEffect.cs
- SqlGatherProducedAliases.cs
- ApplyTemplatesAction.cs
- BorderGapMaskConverter.cs
- FuncCompletionCallbackWrapper.cs
- RedistVersionInfo.cs
- SchemaObjectWriter.cs
- XmlBaseReader.cs
- ClientSettingsStore.cs
- NavigateEvent.cs
- AnnotationHighlightLayer.cs
- CaseInsensitiveComparer.cs
- NameValuePermission.cs
- ProfessionalColorTable.cs
- ValuePattern.cs
- WeakEventTable.cs
- CLSCompliantAttribute.cs
- SystemTcpStatistics.cs
- BulletedList.cs
- BasicHttpMessageSecurity.cs
- PathSegment.cs
- PropertyMapper.cs
- FormsAuthenticationModule.cs
- DirectionalAction.cs
- XPathScanner.cs
- DirectionalLight.cs
- PEFileReader.cs
- Menu.cs
- TTSVoice.cs
- DrawingImage.cs
- DocumentSchemaValidator.cs
- BaseDataList.cs
- SwitchLevelAttribute.cs
- DataGridViewColumnEventArgs.cs
- NamespaceList.cs
- TimeSpanSecondsConverter.cs
- TimersDescriptionAttribute.cs
- Context.cs
- KeyConstraint.cs
- MemberAccessException.cs
- PrintDialog.cs
- QuaternionAnimationUsingKeyFrames.cs
- DataGridHeaderBorder.cs
- BuildManager.cs
- SqlDataSourceView.cs
- ToolZone.cs
- cookie.cs
- WebControlAdapter.cs
- NotifyIcon.cs
- ImportContext.cs
- ConfigXmlElement.cs
- GridViewSelectEventArgs.cs
- KeyEventArgs.cs
- EntityContainerEntitySetDefiningQuery.cs
- DbProviderFactoriesConfigurationHandler.cs
- AsmxEndpointPickerExtension.cs
- DateTimeUtil.cs
- ComponentEditorForm.cs
- ReliabilityContractAttribute.cs
- TraceHwndHost.cs
- SqlDataSource.cs
- DbgCompiler.cs
- LiteralLink.cs
- FrameworkElement.cs
- RadioButton.cs
- PasswordBox.cs
- DataPagerField.cs
- CapabilitiesAssignment.cs
- HostDesigntimeLicenseContext.cs
- QueueProcessor.cs
- OuterGlowBitmapEffect.cs
- FormsAuthenticationUserCollection.cs