Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Runtime / FuncCompletionCallbackWrapper.cs / 1305376 / FuncCompletionCallbackWrapper.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.Runtime { using System; using System.Collections.Generic; using System.Text; using System.Runtime.Serialization; using System.Runtime; using System.Security; [DataContract] class FuncCompletionCallbackWrapper: CompletionCallbackWrapper { static Type callbackType = typeof(CompletionCallback ); static Type[] callbackParameterTypes = new Type[] { typeof(NativeActivityContext), typeof(ActivityInstance),typeof(T) }; [DataMember(EmitDefaultValue = false)] T resultValue; public FuncCompletionCallbackWrapper(CompletionCallback callback, ActivityInstance owningInstance) : base(callback, owningInstance) { this.NeedsToGatherOutputs = true; } int GetResultId(ActivityWithResult activity) { if (activity.Result != null) { return activity.Result.Id; } else { for (int i = 0; i < activity.RuntimeArguments.Count; i++) { RuntimeArgument argument = activity.RuntimeArguments[i]; if (argument.IsResult) { return argument.Id; } } } return -1; } protected override void GatherOutputs(ActivityInstance completedInstance) { int resultId = -1; if (completedInstance.Activity.HandlerOf != null) { DelegateOutArgument resultArgument = completedInstance.Activity.HandlerOf.GetResultArgument(); if (resultArgument != null) { resultId = resultArgument.Id; } else { ActivityWithResult activity = completedInstance.Activity as ActivityWithResult; // for auto-generated results, we should bind the value from the Handler if available if (activity != null && TypeHelper.AreTypesCompatible(activity.ResultType, typeof(T))) { resultId = GetResultId(activity); } } } else { Fx.Assert(completedInstance.Activity is ActivityWithResult, "should only be using FuncCompletionCallbackWrapper with ActivityFunc and ActivityWithResult"); resultId = GetResultId((ActivityWithResult)completedInstance.Activity); } if (resultId >= 0) { Location location = completedInstance.Environment.GetSpecificLocation(resultId); Location typedLocation = location as Location ; if (typedLocation != null) { this.resultValue = typedLocation.Value; } else if (location != null) { this.resultValue = TypeHelper.Convert (location.Value); } } } protected internal override void Invoke(NativeActivityContext context, ActivityInstance completedInstance) { // Call the EnsureCallback overload that also looks for SomeMethod where T is the result type // and the signature matches. EnsureCallback(callbackType, callbackParameterTypes, callbackParameterTypes[2]); CompletionCallback completionCallback = (CompletionCallback )this.Callback; completionCallback(context, completedInstance, this.resultValue); } protected override void OnSerializingGenericCallback() { ValidateCallbackResolution(callbackType, callbackParameterTypes, callbackParameterTypes[2]); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
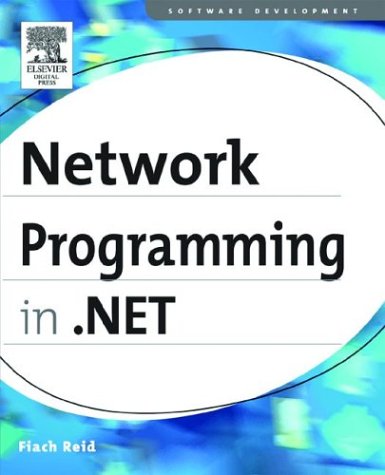
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XPathScanner.cs
- PackageProperties.cs
- RequestBringIntoViewEventArgs.cs
- MenuItemAutomationPeer.cs
- ThreadStartException.cs
- DataGridRowsPresenter.cs
- TransformCollection.cs
- ItemsPresenter.cs
- DrawingImage.cs
- ReferenceConverter.cs
- ListenerConstants.cs
- PointCollection.cs
- InplaceBitmapMetadataWriter.cs
- Operand.cs
- WebUtil.cs
- FunctionImportElement.cs
- SerializationStore.cs
- WizardSideBarListControlItem.cs
- localization.cs
- InkCanvasFeedbackAdorner.cs
- DataGridHeadersVisibilityToVisibilityConverter.cs
- ManualResetEvent.cs
- PeerTransportCredentialType.cs
- TemplateBaseAction.cs
- ColumnReorderedEventArgs.cs
- DateTimeValueSerializer.cs
- GlyphingCache.cs
- ArraySegment.cs
- XmlReader.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- XsdDuration.cs
- Helpers.cs
- LayoutTableCell.cs
- WebAdminConfigurationHelper.cs
- SafeRightsManagementPubHandle.cs
- ElementsClipboardData.cs
- DetailsViewRow.cs
- DataTableReaderListener.cs
- XmlCountingReader.cs
- sqlcontext.cs
- CrossContextChannel.cs
- DriveNotFoundException.cs
- InfoCardSymmetricCrypto.cs
- ViewBox.cs
- XmlSerializerSection.cs
- AutomationTextAttribute.cs
- File.cs
- Inflater.cs
- NameValueSectionHandler.cs
- SizeAnimationUsingKeyFrames.cs
- OleDbPermission.cs
- DataServiceStreamProviderWrapper.cs
- ProfileSettings.cs
- DbCommandTree.cs
- EntityException.cs
- DataSourceXmlElementAttribute.cs
- SetIterators.cs
- AbandonedMutexException.cs
- EndOfStreamException.cs
- TypeExtensionSerializer.cs
- VirtualPathUtility.cs
- xmlglyphRunInfo.cs
- validation.cs
- Boolean.cs
- ParserContext.cs
- WindowsListViewItemStartMenu.cs
- QilInvoke.cs
- HttpProtocolImporter.cs
- XmlDocumentType.cs
- DrawListViewSubItemEventArgs.cs
- DetailsViewRowCollection.cs
- EqualityComparer.cs
- HierarchicalDataTemplate.cs
- TypePropertyEditor.cs
- ErrorProvider.cs
- CustomErrorsSection.cs
- KeyboardDevice.cs
- StreamAsIStream.cs
- TraceHwndHost.cs
- TreeNodeCollection.cs
- Operators.cs
- Trace.cs
- Stopwatch.cs
- ResourcePermissionBase.cs
- TreeNodeStyle.cs
- ToolboxItemCollection.cs
- WindowsTooltip.cs
- LeaseManager.cs
- WindowsFont.cs
- Connector.cs
- SQLBoolean.cs
- Image.cs
- EncoderNLS.cs
- BlockUIContainer.cs
- ControlCollection.cs
- WmpBitmapDecoder.cs
- Attributes.cs
- XPathNodeList.cs
- NavigationExpr.cs
- EntityTypeBase.cs