Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / security / system / security / cryptography / Oid.cs / 1305376 / Oid.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // Oid.cs // namespace System.Security.Cryptography { using System.Collections; using System.Security.Cryptography.X509Certificates; internal enum OidGroup { AllGroups = 0, HashAlgorithm = 1, EncryptionAlgorithm = 2, PublicKeyAlgorithm = 3, SignatureAlgorithm = 4, Attribute = 5, ExtensionOrAttribute = 6, EnhancedKeyUsage = 7, Policy = 8, Template = 9 } public sealed class Oid { private string m_value = null; private string m_friendlyName = null; private OidGroup m_group = OidGroup.AllGroups; public Oid() {} public Oid(string oid) : this(oid, OidGroup.AllGroups, true) { } internal Oid(string oid, OidGroup group, bool lookupFriendlyName) { if (lookupFriendlyName) { // If we were passed the friendly name, retrieve the value string. string oidValue = X509Utils.FindOidInfo(CAPI.CRYPT_OID_INFO_NAME_KEY, oid, group); if (oidValue == null) oidValue = oid; this.Value = oidValue; } else { this.Value = oid; } m_group = group; } public Oid(string value, string friendlyName) { m_value = value; m_friendlyName = friendlyName; } public Oid(Oid oid) { if (oid == null) throw new ArgumentNullException("oid"); m_value = oid.m_value; m_friendlyName = oid.m_friendlyName; m_group = oid.m_group; } public string Value { get { return m_value; } set { m_value = value; } } public string FriendlyName { get { if(m_friendlyName == null && m_value != null) m_friendlyName = X509Utils.FindOidInfo(CAPI.CRYPT_OID_INFO_OID_KEY, m_value, m_group); return m_friendlyName; } set { m_friendlyName = value; // If we can find the matching OID value, then update it as well if (m_friendlyName != null) { // If FindOidInfo fails, we return a null string string oidValue = X509Utils.FindOidInfo(CAPI.CRYPT_OID_INFO_NAME_KEY, m_friendlyName, m_group); if (oidValue != null) m_value = oidValue; } } } } public sealed class OidCollection : ICollection { private ArrayList m_list; public OidCollection() { m_list = new ArrayList(); } public int Add(Oid oid) { return m_list.Add(oid); } public Oid this[int index] { get { return m_list[index] as Oid; } } // Indexer using an OID friendly name or value. public Oid this[string oid] { get { // If we were passed the friendly name, retrieve the value string. string oidValue = X509Utils.FindOidInfo(CAPI.CRYPT_OID_INFO_NAME_KEY, oid, OidGroup.AllGroups); if (oidValue == null) oidValue = oid; foreach (Oid entry in m_list) { if (entry.Value == oidValue) return entry; } return null; } } public int Count { get { return m_list.Count; } } public OidEnumerator GetEnumerator() { return new OidEnumerator(this); } ///IEnumerator IEnumerable.GetEnumerator() { return new OidEnumerator(this); } /// void ICollection.CopyTo(Array array, int index) { if (array == null) throw new ArgumentNullException("array"); if (array.Rank != 1) throw new ArgumentException(SR.GetString(SR.Arg_RankMultiDimNotSupported)); if (index < 0 || index >= array.Length) throw new ArgumentOutOfRangeException("index", SR.GetString(SR.ArgumentOutOfRange_Index)); if (index + this.Count > array.Length) throw new ArgumentException(SR.GetString(SR.Argument_InvalidOffLen)); for (int i=0; i < this.Count; i++) { array.SetValue(this[i], index); index++; } } public void CopyTo(Oid[] array, int index) { ((ICollection)this).CopyTo(array, index); } public bool IsSynchronized { get { return false; } } public Object SyncRoot { get { return this; } } } public sealed class OidEnumerator : IEnumerator { private OidCollection m_oids; private int m_current; private OidEnumerator() {} internal OidEnumerator(OidCollection oids) { m_oids = oids; m_current = -1; } public Oid Current { get { return m_oids[m_current]; } } /// Object IEnumerator.Current { get { return (Object) m_oids[m_current]; } } public bool MoveNext() { if (m_current == ((int) m_oids.Count - 1)) return false; m_current++; return true; } public void Reset() { m_current = -1; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // Oid.cs // namespace System.Security.Cryptography { using System.Collections; using System.Security.Cryptography.X509Certificates; internal enum OidGroup { AllGroups = 0, HashAlgorithm = 1, EncryptionAlgorithm = 2, PublicKeyAlgorithm = 3, SignatureAlgorithm = 4, Attribute = 5, ExtensionOrAttribute = 6, EnhancedKeyUsage = 7, Policy = 8, Template = 9 } public sealed class Oid { private string m_value = null; private string m_friendlyName = null; private OidGroup m_group = OidGroup.AllGroups; public Oid() {} public Oid(string oid) : this(oid, OidGroup.AllGroups, true) { } internal Oid(string oid, OidGroup group, bool lookupFriendlyName) { if (lookupFriendlyName) { // If we were passed the friendly name, retrieve the value string. string oidValue = X509Utils.FindOidInfo(CAPI.CRYPT_OID_INFO_NAME_KEY, oid, group); if (oidValue == null) oidValue = oid; this.Value = oidValue; } else { this.Value = oid; } m_group = group; } public Oid(string value, string friendlyName) { m_value = value; m_friendlyName = friendlyName; } public Oid(Oid oid) { if (oid == null) throw new ArgumentNullException("oid"); m_value = oid.m_value; m_friendlyName = oid.m_friendlyName; m_group = oid.m_group; } public string Value { get { return m_value; } set { m_value = value; } } public string FriendlyName { get { if(m_friendlyName == null && m_value != null) m_friendlyName = X509Utils.FindOidInfo(CAPI.CRYPT_OID_INFO_OID_KEY, m_value, m_group); return m_friendlyName; } set { m_friendlyName = value; // If we can find the matching OID value, then update it as well if (m_friendlyName != null) { // If FindOidInfo fails, we return a null string string oidValue = X509Utils.FindOidInfo(CAPI.CRYPT_OID_INFO_NAME_KEY, m_friendlyName, m_group); if (oidValue != null) m_value = oidValue; } } } } public sealed class OidCollection : ICollection { private ArrayList m_list; public OidCollection() { m_list = new ArrayList(); } public int Add(Oid oid) { return m_list.Add(oid); } public Oid this[int index] { get { return m_list[index] as Oid; } } // Indexer using an OID friendly name or value. public Oid this[string oid] { get { // If we were passed the friendly name, retrieve the value string. string oidValue = X509Utils.FindOidInfo(CAPI.CRYPT_OID_INFO_NAME_KEY, oid, OidGroup.AllGroups); if (oidValue == null) oidValue = oid; foreach (Oid entry in m_list) { if (entry.Value == oidValue) return entry; } return null; } } public int Count { get { return m_list.Count; } } public OidEnumerator GetEnumerator() { return new OidEnumerator(this); } /// IEnumerator IEnumerable.GetEnumerator() { return new OidEnumerator(this); } /// void ICollection.CopyTo(Array array, int index) { if (array == null) throw new ArgumentNullException("array"); if (array.Rank != 1) throw new ArgumentException(SR.GetString(SR.Arg_RankMultiDimNotSupported)); if (index < 0 || index >= array.Length) throw new ArgumentOutOfRangeException("index", SR.GetString(SR.ArgumentOutOfRange_Index)); if (index + this.Count > array.Length) throw new ArgumentException(SR.GetString(SR.Argument_InvalidOffLen)); for (int i=0; i < this.Count; i++) { array.SetValue(this[i], index); index++; } } public void CopyTo(Oid[] array, int index) { ((ICollection)this).CopyTo(array, index); } public bool IsSynchronized { get { return false; } } public Object SyncRoot { get { return this; } } } public sealed class OidEnumerator : IEnumerator { private OidCollection m_oids; private int m_current; private OidEnumerator() {} internal OidEnumerator(OidCollection oids) { m_oids = oids; m_current = -1; } public Oid Current { get { return m_oids[m_current]; } } /// Object IEnumerator.Current { get { return (Object) m_oids[m_current]; } } public bool MoveNext() { if (m_current == ((int) m_oids.Count - 1)) return false; m_current++; return true; } public void Reset() { m_current = -1; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
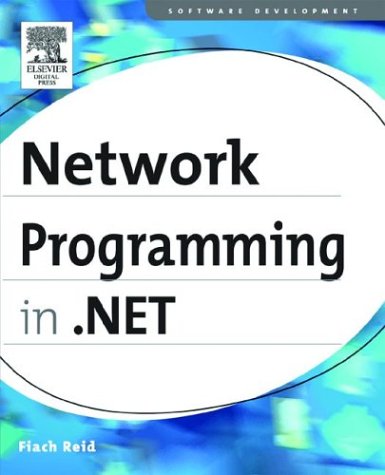
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Helpers.cs
- QilTypeChecker.cs
- MemoryFailPoint.cs
- XmlAttributeCollection.cs
- DataGridViewCellStyleConverter.cs
- MachineKey.cs
- DispatcherObject.cs
- ExtenderControl.cs
- LinqDataSource.cs
- TextElementCollectionHelper.cs
- RNGCryptoServiceProvider.cs
- UnwrappedTypesXmlSerializerManager.cs
- ServiceDescriptionReflector.cs
- SqlDependency.cs
- EnterpriseServicesHelper.cs
- TraceSection.cs
- ReadOnlyNameValueCollection.cs
- ObjectDataSourceChooseTypePanel.cs
- PageAction.cs
- KeyTimeConverter.cs
- EditorBrowsableAttribute.cs
- PriorityItem.cs
- ResourceReferenceExpression.cs
- TypedTableGenerator.cs
- ToolStripDropDownClosingEventArgs.cs
- InvalidFilterCriteriaException.cs
- Pool.cs
- Page.cs
- ObjectViewFactory.cs
- WindowInteractionStateTracker.cs
- ASCIIEncoding.cs
- CopyCodeAction.cs
- XmlWellformedWriter.cs
- propertytag.cs
- MetadataPropertyvalue.cs
- RuntimeConfig.cs
- PreservationFileReader.cs
- SHA1CryptoServiceProvider.cs
- XamlDesignerSerializationManager.cs
- CodeCompileUnit.cs
- DBConnection.cs
- ReadOnlyDataSource.cs
- SafeReadContext.cs
- ConfigXmlText.cs
- HttpHandlersSection.cs
- Utils.cs
- Number.cs
- CqlParser.cs
- ColorAnimation.cs
- ObjectFullSpanRewriter.cs
- ProgressBar.cs
- SystemEvents.cs
- MSG.cs
- AuthStoreRoleProvider.cs
- Expressions.cs
- SqlRecordBuffer.cs
- MethodAccessException.cs
- BuildManagerHost.cs
- KeyboardNavigation.cs
- TabItemWrapperAutomationPeer.cs
- DnsEndPoint.cs
- ToolBarPanel.cs
- WmlObjectListAdapter.cs
- TraceContext.cs
- XmlSchemaDatatype.cs
- Listbox.cs
- ToolBarButton.cs
- PropertyPathWorker.cs
- RelatedEnd.cs
- StringInfo.cs
- MemberMaps.cs
- Transform.cs
- PreservationFileWriter.cs
- DynamicPropertyHolder.cs
- WorkflowDesigner.cs
- WindowsButton.cs
- GeneralTransform3DCollection.cs
- ToolboxItemFilterAttribute.cs
- ObjectParameter.cs
- cookiecontainer.cs
- ZipIOCentralDirectoryBlock.cs
- FileClassifier.cs
- PropertyMappingExceptionEventArgs.cs
- AssemblyFilter.cs
- MetafileHeaderEmf.cs
- DeploymentSection.cs
- TabRenderer.cs
- MiniLockedBorderGlyph.cs
- HtmlEmptyTagControlBuilder.cs
- ResourceReferenceExpressionConverter.cs
- TimeoutException.cs
- HttpStreamMessageEncoderFactory.cs
- DecimalConstantAttribute.cs
- XmlSerializerSection.cs
- QueryOperationResponseOfT.cs
- DbDataRecord.cs
- ContactManager.cs
- RowUpdatingEventArgs.cs
- PointF.cs
- ErasingStroke.cs