Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / Windows / Interop / MSG.cs / 1305600 / MSG.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Diagnostics.CodeAnalysis; using System.Security; using MS.Internal.WindowsBase; namespace System.Windows.Interop { ////// This class is the managed version of the Win32 MSG datatype. /// ////// For Avalon/[....] interop to work, [....] needs to be able to modify MSG structs as they are /// processed, so they are passed by ref (it's also a perf gain) /// /// - but in the Partial Trust scenario, this would be a security vulnerability; allowing partially trusted code /// to intercept and arbitrarily change MSG contents could potentially create a spoofing opportunity. /// /// - so rather than try to secure all posible current and future extensibility points against untrusted code /// getting write access to a MSG struct during message processing, we decided the simpler, more performant, and /// more secure, both now and going forward, solution was to secure write access to the MSG struct directly /// at the source. /// /// - get access is unrestricted and should in-line nicely for zero perf cost /// /// - set access is restricted via a call to SecurityHelper.DemandUnrestrictedUIPermission, which is optimized /// to a no-op in the Full Trust scenario, and will throw a security exception in the Partial Trust scenario /// /// - NOTE: This breaks Avalon/[....] interop in the Partial Trust scenario, but that's not a supported /// scenario anyway. /// [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] [SuppressMessage("Microsoft.Naming", "CA1705:LongAcronymsShouldBePascalCased")] [SuppressMessage("Microsoft.Performance", "CA1815:OverrideEqualsAndOperatorEqualsOnValueTypes")] [StructLayout(LayoutKind.Sequential)] [Serializable] public struct MSG { ////// Critical: Setting critical data /// [SecurityCritical] [FriendAccessAllowed] // Built into Base, used by Core or Framework. internal MSG(IntPtr hwnd, int message, IntPtr wParam, IntPtr lParam, int time, int pt_x, int pt_y) { _hwnd = hwnd; _message = message; _wParam = wParam; _lParam = lParam; _time = time; _pt_x = pt_x; _pt_y = pt_y; } // // Public Properties: // ////// The handle of the window to which the message was sent. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// [SuppressMessage("Microsoft.Design", "CA1051:DoNotDeclareVisibleInstanceFields")] [SuppressMessage("Microsoft.Security", "CA2111:PointersShouldNotBeVisible")] [SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] public IntPtr hwnd { [SecurityCritical] get { return _hwnd; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _hwnd = value; } } ////// The Value of the window message. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// public int message { [SecurityCritical] get { return _message; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _message = value; } } ////// The wParam of the window message. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] [SuppressMessage("Microsoft.Security", "CA2111:PointersShouldNotBeVisible")] [SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] public IntPtr wParam { [SecurityCritical] get { return _wParam; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _wParam = value; } } ////// The lParam of the window message. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] [SuppressMessage("Microsoft.Security", "CA2111:PointersShouldNotBeVisible")] [SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] public IntPtr lParam { [SecurityCritical] get { return _lParam; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _lParam = value; } } ////// The time the window message was sent. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// public int time { [SecurityCritical] get { return _time; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _time = value; } } // In the original Win32, pt was a by-Value POINT structure ////// The X coordinate of the message POINT struct. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// [SuppressMessage("Microsoft.Naming", "CA1706:ShortAcronymsShouldBeUpperCased")] [SuppressMessage("Microsoft.Naming", "CA1707:IdentifiersShouldNotContainUnderscores")] public int pt_x { [SecurityCritical] get { return _pt_x; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _pt_x = value; } } ////// The Y coordinate of the message POINT struct. /// ////// Critical: This data can not be modified by Partial Trust code as that may be exploited for spoofing purposes /// PublicOK: This data is safe for Partial Trust code to read (getter), There is a demand on the setter to block Partial Trust code /// [SuppressMessage("Microsoft.Naming", "CA1706:ShortAcronymsShouldBeUpperCased")] [SuppressMessage("Microsoft.Naming", "CA1707:IdentifiersShouldNotContainUnderscores")] public int pt_y { [SecurityCritical] get { return _pt_y; } [SecurityCritical] set { SecurityHelper.DemandUnrestrictedUIPermission(); _pt_y = value; } } // // Internal data: // - do not alter the number, order or size of ANY of this members! // - they must agree EXACTLY with the native Win32 MSG structure ////// The handle of the window to which the message was sent. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private IntPtr _hwnd; ////// The Value of the window message. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private int _message; ////// The wParam of the window message. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private IntPtr _wParam; ////// The lParam of the window message. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private IntPtr _lParam; ////// The time the window message was sent. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private int _time; ////// The X coordinate of the message POINT struct. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private int _pt_x; ////// The Y coordinate of the message POINT struct. /// ////// Critical: This data can not be modified by Partial Trust code for spoofing purposes /// [SecurityCritical] private int _pt_y; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
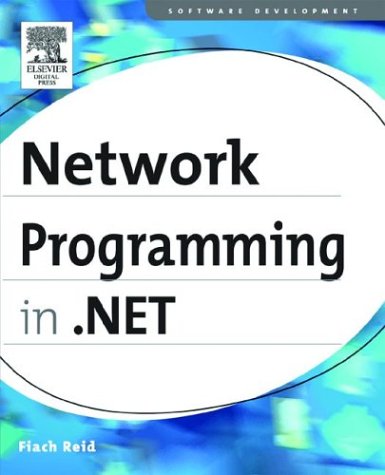
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PageHandlerFactory.cs
- BitmapImage.cs
- CreateUserWizardStep.cs
- NativeMethods.cs
- PrtTicket_Public_Simple.cs
- DataList.cs
- IntranetCredentialPolicy.cs
- NonDualMessageSecurityOverHttp.cs
- LocalizationComments.cs
- PeerApplicationLaunchInfo.cs
- WinEventTracker.cs
- XPathDocumentNavigator.cs
- TransportBindingElementImporter.cs
- Selection.cs
- ChangeBlockUndoRecord.cs
- QuadraticBezierSegment.cs
- RegexTree.cs
- ProcessModelSection.cs
- SqlStatistics.cs
- PropertyDescriptorCollection.cs
- RepeaterItemEventArgs.cs
- DBNull.cs
- XComponentModel.cs
- ModuleBuilderData.cs
- FontWeightConverter.cs
- ProgressBarRenderer.cs
- UrlRoutingHandler.cs
- MailBnfHelper.cs
- ListViewCommandEventArgs.cs
- SerializationSectionGroup.cs
- DataBoundControlAdapter.cs
- PointKeyFrameCollection.cs
- XamlDesignerSerializationManager.cs
- AttributeCollection.cs
- DocumentScope.cs
- XamlStyleSerializer.cs
- DesignerDataTableBase.cs
- CriticalFinalizerObject.cs
- DataGridViewUtilities.cs
- ValueQuery.cs
- XmlReflectionMember.cs
- SqlWebEventProvider.cs
- WindowsGraphicsWrapper.cs
- PointAnimationUsingKeyFrames.cs
- DocumentReference.cs
- EncryptedKey.cs
- HtmlTextArea.cs
- ProcessThreadCollection.cs
- InplaceBitmapMetadataWriter.cs
- SweepDirectionValidation.cs
- TypeConverter.cs
- XmlSerializerNamespaces.cs
- MenuItemBinding.cs
- GenericUriParser.cs
- AffineTransform3D.cs
- StdValidatorsAndConverters.cs
- GridProviderWrapper.cs
- DataShape.cs
- IPHostEntry.cs
- GroupItem.cs
- OletxCommittableTransaction.cs
- SingleObjectCollection.cs
- RewritingPass.cs
- ErrorStyle.cs
- SQLDateTimeStorage.cs
- IIS7WorkerRequest.cs
- TypeCodeDomSerializer.cs
- XmlValueConverter.cs
- XsltConvert.cs
- RowSpanVector.cs
- PrintDocument.cs
- MasterPageCodeDomTreeGenerator.cs
- ReaderWriterLockWrapper.cs
- LeftCellWrapper.cs
- ResourceSetExpression.cs
- QueryContinueDragEventArgs.cs
- ValueExpressions.cs
- PersonalizationAdministration.cs
- HashRepartitionStream.cs
- Rule.cs
- _ListenerResponseStream.cs
- DataGridViewColumnHeaderCell.cs
- AssemblyNameProxy.cs
- AssociationProvider.cs
- CorruptingExceptionCommon.cs
- CounterSample.cs
- ExtensionQuery.cs
- OperationAbortedException.cs
- Int32Rect.cs
- SafeRightsManagementHandle.cs
- DynamicEndpointElement.cs
- DispatcherHooks.cs
- AtomParser.cs
- PathTooLongException.cs
- HtmlInputControl.cs
- XmlEncoding.cs
- StatusBarPanel.cs
- XPathDocumentBuilder.cs
- Blend.cs
- Currency.cs