Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / AccessibleTech / longhorn / Automation / UIAutomationClient / System / Windows / Automation / RangeValuePattern.cs / 1 / RangeValuePattern.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for RangeValue Pattern // // History: // 06/23/2003 : [....] Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Exposes a related set of properties that reflect a control's ability to manage a value /// within a finite range. It conveys a controls valid minimum and maximum values and its /// current value. /// /// Pattern requires MinValue less than MaxValue. /// MinimumValue and MaximumValue must be the same Object type as ValueAsObject. /// #if (INTERNAL_COMPILE) internal class RangeValuePattern : BasePattern #else public class RangeValuePattern : BasePattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private RangeValuePattern( AutomationElement el, SafePatternHandle hPattern, bool cached ) : base(el, hPattern) { _hPattern = hPattern; _cached = cached; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///Value pattern public static readonly AutomationPattern Pattern = RangeValuePatternIdentifiers.Pattern; ///Property ID: Value - Value of a value control, as a double public static readonly AutomationProperty ValueProperty = RangeValuePatternIdentifiers.ValueProperty; ///Property ID: IsReadOnly - Indicates that the value can only be read, not modified. public static readonly AutomationProperty IsReadOnlyProperty = RangeValuePatternIdentifiers.IsReadOnlyProperty; ///Property ID: Maximum value public static readonly AutomationProperty MinimumProperty = RangeValuePatternIdentifiers.MinimumProperty; ///Property ID: Maximum value public static readonly AutomationProperty MaximumProperty = RangeValuePatternIdentifiers.MaximumProperty; ///Property ID: LargeChange - Indicates a value to be added to or subtracted from the Value property when the element is moved a large distance. public static readonly AutomationProperty LargeChangeProperty = RangeValuePatternIdentifiers.LargeChangeProperty; ///Property ID: SmallChange - Indicates a value to be added to or subtracted from the Value property when the element is moved a small distance. public static readonly AutomationProperty SmallChangeProperty = RangeValuePatternIdentifiers.SmallChangeProperty; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Request to set the value that this UI element is representing /// /// Value to set the UI to, as a double /// ////// This API does not work inside the secure execution environment. /// public void SetValue(double value) { // Test the Enabled state prior to the more general Read-Only state. object enabled = _el.GetCurrentPropertyValue(AutomationElementIdentifiers.IsEnabledProperty); if (enabled is bool && !(bool)enabled) { throw new ElementNotEnabledException(); } // Test the Read-Only state after the more specific Enabled state. object readOnly = _el.GetCurrentPropertyValue(IsReadOnlyProperty); if (readOnly is bool && (bool)readOnly) { throw new InvalidOperationException(SR.Get(SRID.ValueReadonly)); } UiaCoreApi.RangeValuePattern_SetValue(_hPattern, value); } #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// /// This member allows access to previously requested /// cached properties for this element. The returned object /// has accessors for each property defined for this pattern. /// ////// Cached property values must have been previously requested /// using a CacheRequest. If you try to access a cached /// property that was not previously requested, an InvalidOperation /// Exception will be thrown. /// /// To get the value of a property at the current point in time, /// access the property via the Current accessor instead of /// Cached. /// public RangeValuePatternInformation Cached { get { Misc.ValidateCached(_cached); return new RangeValuePatternInformation(_el, true); } } ////// This member allows access to current property values /// for this element. The returned object has accessors for /// each property defined for this pattern. /// ////// This pattern must be from an AutomationElement with a /// Full reference in order to get current values. If the /// AutomationElement was obtained using AutomationElementMode.None, /// then it contains only cached data, and attempting to get /// the current value of any property will throw an InvalidOperationException. /// /// To get the cached value of a property that was previously /// specified using a CacheRequest, access the property via the /// Cached accessor instead of Current. /// public RangeValuePatternInformation Current { get { Misc.ValidateCurrent(_hPattern); return new RangeValuePatternInformation(_el, false); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods static internal object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new RangeValuePattern(el, hPattern, cached); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; private bool _cached; #endregion Private Fields //----------------------------------------------------- // // Nested Classes // //------------------------------------------------------ #region Nested Classes ////// This class provides access to either Cached or Current /// properties on a pattern via the pattern's .Cached or /// .Current accessors. /// public struct RangeValuePatternInformation { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors internal RangeValuePatternInformation(AutomationElement el, bool useCache) { _el = el; _useCache = useCache; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ///Value of a value control /// ////// This API does not work inside the secure execution environment. /// public double Value { get { object propValue = _el.GetPatternPropertyValue(ValueProperty, _useCache); if (propValue is int) { return (double)(int)propValue; } else if (propValue is Int32) { return (double)(Int32)propValue; } else if (propValue is byte) { return (double)(byte)propValue; } else if (propValue is DateTime) { return (double)((DateTime)propValue).Year; } else { return (double)propValue; } } } ////// Indicates that the value can only be read, not modified. ///returns True if the control is read-only /// ////// This API does not work inside the secure execution environment. /// public bool IsReadOnly { get { return (bool)_el.GetPatternPropertyValue(IsReadOnlyProperty, _useCache); } } ////// maximum value /// ////// This API does not work inside the secure execution environment. /// public double Maximum { get { object propValue = _el.GetPatternPropertyValue(MaximumProperty, _useCache); if (propValue is int) { return (double)(int)propValue; } else if (propValue is Int32) { return (double)(Int32)propValue; } else if (propValue is byte) { return (double)(byte)propValue; } else if (propValue is DateTime) { return (double)((DateTime)propValue).Year; } else { return (double)propValue; } } } ////// minimum value /// ////// This API does not work inside the secure execution environment. /// public double Minimum { get { object propValue = _el.GetPatternPropertyValue(MinimumProperty, _useCache); if (propValue is int) { return (double)(int)propValue; } else if (propValue is Int32) { return (double)(Int32)propValue; } else if (propValue is byte) { return (double)(byte)propValue; } else if (propValue is DateTime) { return (double)((DateTime)propValue).Year; } else { return (double)propValue; } } } ////// /// Gets a value to be added to or subtracted from the Value property /// when the element is moved a large distance. /// /// ////// This API does not work inside the secure execution environment. /// public double LargeChange { get { object propValue = _el.GetPatternPropertyValue(LargeChangeProperty, _useCache); if (propValue is int) { return (double)(int)propValue; } else if (propValue is Int32) { return (double)(Int32)propValue; } else if (propValue is byte) { return (double)(byte)propValue; } else if (propValue is DateTime) { return (double)((DateTime)propValue).Year; } else { return (double)propValue; } } } ////// /// Gets a value to be added to or subtracted from the Value property /// when the element is moved a small distance. /// /// ////// This API does not work inside the secure execution environment. /// public double SmallChange { get { object propValue = _el.GetPatternPropertyValue(SmallChangeProperty, _useCache); if (propValue is int) { return (double)(int)propValue; } else if (propValue is Int32) { return (double)(Int32)propValue; } else if (propValue is byte) { return (double)(byte)propValue; } else if (propValue is DateTime) { return (double)((DateTime)propValue).Year; } else { return (double)propValue; } } } #endregion Public Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationElement _el; // AutomationElement that contains the cache or live reference private bool _useCache; // true to use cache, false to use live reference to get current values #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
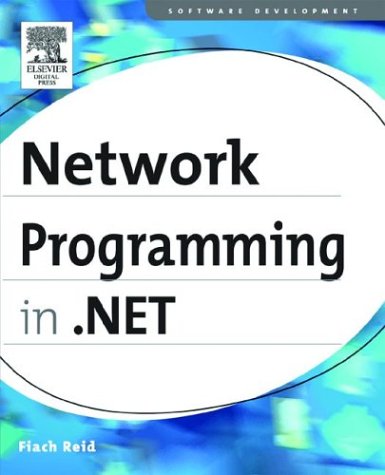
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RijndaelManaged.cs
- MasterPage.cs
- ApplicationDirectoryMembershipCondition.cs
- WizardForm.cs
- BitmapDecoder.cs
- HtmlHistory.cs
- RepeatInfo.cs
- METAHEADER.cs
- CacheMode.cs
- TextFindEngine.cs
- QueueAccessMode.cs
- MessageLogger.cs
- DbException.cs
- SettingsPropertyValueCollection.cs
- XomlCompilerError.cs
- RuleSettingsCollection.cs
- DataViewSetting.cs
- OrderedDictionary.cs
- InlineObject.cs
- NativeMethodsOther.cs
- WebServiceData.cs
- CalendarDateRange.cs
- SerializationFieldInfo.cs
- FileUtil.cs
- SspiSecurityTokenProvider.cs
- HtmlTableCell.cs
- LocatorGroup.cs
- ManagementOptions.cs
- MenuItemBindingCollection.cs
- OdbcFactory.cs
- ConfigurationStrings.cs
- OracleParameterBinding.cs
- ListView.cs
- ComponentFactoryHelpers.cs
- Tablet.cs
- WindowsListViewGroupHelper.cs
- DataGridPagingPage.cs
- UIElementParaClient.cs
- ToolStripMenuItemCodeDomSerializer.cs
- PerformanceCounterNameAttribute.cs
- ScriptComponentDescriptor.cs
- KernelTypeValidation.cs
- HiddenField.cs
- ColumnMapProcessor.cs
- ExpandCollapsePattern.cs
- CalendarDateRangeChangingEventArgs.cs
- DataGridViewButtonColumn.cs
- EdmType.cs
- HandledEventArgs.cs
- QilLoop.cs
- NodeLabelEditEvent.cs
- Compiler.cs
- CaseCqlBlock.cs
- DoubleCollection.cs
- DataRowComparer.cs
- WebSysDefaultValueAttribute.cs
- IndexOutOfRangeException.cs
- TargetException.cs
- ConfigurationSectionCollection.cs
- BuildProvider.cs
- SqlAggregateChecker.cs
- AppDomainFactory.cs
- DbConnectionPool.cs
- Type.cs
- Utils.cs
- OleDbInfoMessageEvent.cs
- DefaultAuthorizationContext.cs
- UnauthorizedAccessException.cs
- Bits.cs
- ContentControl.cs
- XmlSchemaType.cs
- DragDropManager.cs
- Filter.cs
- SqlCacheDependencyDatabaseCollection.cs
- VirtualDirectoryMapping.cs
- BrowserDefinition.cs
- PolicyStatement.cs
- ping.cs
- CacheManager.cs
- OuterGlowBitmapEffect.cs
- VBIdentifierTrimConverter.cs
- MatrixCamera.cs
- Literal.cs
- Deserializer.cs
- TakeQueryOptionExpression.cs
- SafeUserTokenHandle.cs
- ValueTypePropertyReference.cs
- Expression.cs
- InstallerTypeAttribute.cs
- ExtendedProtectionPolicyElement.cs
- RawKeyboardInputReport.cs
- BitmapSource.cs
- FontStyles.cs
- HttpListener.cs
- Roles.cs
- sqlinternaltransaction.cs
- Control.cs
- CryptoApi.cs
- WsatExtendedInformation.cs
- PropertyGridCommands.cs