Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Base / MS / Internal / IO / Packaging / XmlStringTable.cs / 1 / XmlStringTable.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Static helper class that serves up Xml strings used to generate and parse // XmlDigitalSignatures. // // History: // 01/30/2005: [....]: Initial implementation. // //----------------------------------------------------------------------------- using System; using System.Xml; using System.Diagnostics; namespace MS.Internal.IO.Packaging { ////// Xml and string table /// ///See: http://www.w3.org/TR/2002/REC-xmldsig-core-20020212/ for details internal static class XTable { ////// Get string given the ID /// /// string id ///string internal static String Get(ID i) { // catch mismatch in table editing Debug.Assert((int)ID._IllegalValue == _table.Length - 1, "ID enumeration should match TableEntry array - verify edits are matching"); Debug.Assert(i >= ID.OpcSignatureNamespace && i < ID._IllegalValue, "string index out of bounds"); Debug.Assert(i == _table[(int)i].id, "table enum out of order - verify order"); return _table[(int)i].s; } ////// One-to-One mapping from enum to string - this must be kept in sync with _table /// internal enum ID : int { OpcSignatureNamespace, OpcSignatureNamespacePrefix, OpcSignatureNamespaceAttribute, W3CSignatureNamespaceRoot, RelationshipsTransformName, //SignatureNamespacePrefix, SignatureTagName, OpcSignatureAttrValue, SignedInfoTagName, ReferenceTagName, // CanonicalizationMethodTagName, SignatureMethodTagName, ObjectTagName, KeyInfoTagName, ManifestTagName, TransformTagName, TransformsTagName, AlgorithmAttrName, SourceIdAttrName, OpcAttrValue, OpcLinkAttrValue, TargetAttrName, SignatureValueTagName, UriAttrName, DigestMethodTagName, DigestValueTagName, SignaturePropertiesTagName, SignaturePropertyTagName, SignatureTimeTagName, SignatureTimeFormatTagName, SignatureTimeValueTagName, RelationshipsTagName, RelationshipReferenceTagName, RelationshipsGroupReferenceTagName, SourceTypeAttrName, SignaturePropertyIdAttrName, SignaturePropertyIdAttrValue, // PackageRelationshipPartName, _IllegalValue // sentinel - not a legal value - equivalent to "not set" or "null" } //----------------------------------------------------------------------------- // private members //----------------------------------------------------------------------------- internal struct TableEntry { internal XTable.ID id; internal string s; internal TableEntry(XTable.ID index, string str) { id = index; s = str; } }; private static readonly TableEntry[] _table = new TableEntry[] { new TableEntry( ID.OpcSignatureNamespace, "http://schemas.openxmlformats.org/package/2006/digital-signature" ), new TableEntry( ID.OpcSignatureNamespacePrefix, "opc" ), new TableEntry( ID.OpcSignatureNamespaceAttribute, "xmlns:opc" ), new TableEntry( ID.W3CSignatureNamespaceRoot, "http://www.w3.org/2000/09/xmldsig#" ), new TableEntry( ID.RelationshipsTransformName, "http://schemas.openxmlformats.org/package/2006/RelationshipTransform" ), // new TableEntry( ID.SignatureNamespacePrefix, "ds" ), new TableEntry( ID.SignatureTagName, "Signature" ), new TableEntry( ID.OpcSignatureAttrValue, "SignatureIdValue" ), new TableEntry( ID.SignedInfoTagName, "SignedInfo" ), new TableEntry( ID.ReferenceTagName, "Reference" ), // new TableEntry( ID.CanonicalizationMethodTagName, "CanonicalizationMethod" ), new TableEntry( ID.SignatureMethodTagName, "SignatureMethod" ), new TableEntry( ID.ObjectTagName, "Object" ), new TableEntry( ID.KeyInfoTagName, "KeyInfo" ), new TableEntry( ID.ManifestTagName, "Manifest" ), new TableEntry( ID.TransformTagName, "Transform" ), new TableEntry( ID.TransformsTagName, "Transforms" ), new TableEntry( ID.AlgorithmAttrName, "Algorithm" ), new TableEntry( ID.SourceIdAttrName, "SourceId" ), new TableEntry( ID.OpcAttrValue, "idPackageObject" ), new TableEntry( ID.OpcLinkAttrValue, "#idPackageObject" ), new TableEntry( ID.TargetAttrName, "Target" ), new TableEntry( ID.SignatureValueTagName, "SignatureValue" ), new TableEntry( ID.UriAttrName, "URI" ), new TableEntry( ID.DigestMethodTagName, "DigestMethod" ), new TableEntry( ID.DigestValueTagName, "DigestValue" ), new TableEntry( ID.SignaturePropertiesTagName, "SignatureProperties" ), new TableEntry( ID.SignaturePropertyTagName, "SignatureProperty" ), new TableEntry( ID.SignatureTimeTagName, "SignatureTime" ), new TableEntry( ID.SignatureTimeFormatTagName, "Format" ), new TableEntry( ID.SignatureTimeValueTagName, "Value" ), new TableEntry( ID.RelationshipsTagName, "Relationships" ), new TableEntry( ID.RelationshipReferenceTagName, "RelationshipReference" ), new TableEntry( ID.RelationshipsGroupReferenceTagName, "RelationshipsGroupReference" ), new TableEntry( ID.SourceTypeAttrName, "SourceType" ), new TableEntry( ID.SignaturePropertyIdAttrName, "Id" ), new TableEntry( ID.SignaturePropertyIdAttrValue, "idSignatureTime" ), new TableEntry( ID._IllegalValue, "" ), }; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
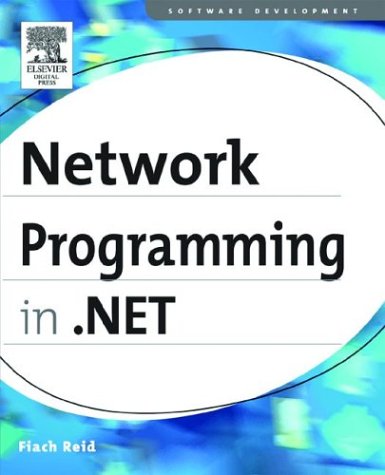
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Symbol.cs
- CharEnumerator.cs
- DrawingVisual.cs
- EncoderReplacementFallback.cs
- ToolStripItemClickedEventArgs.cs
- GenericTypeParameterBuilder.cs
- TextShapeableCharacters.cs
- CompressedStack.cs
- AnonymousIdentificationSection.cs
- MetadataArtifactLoaderCompositeResource.cs
- OpenFileDialog.cs
- ObfuscationAttribute.cs
- Part.cs
- FixedTextContainer.cs
- DataGridSortCommandEventArgs.cs
- __ConsoleStream.cs
- ReceiveActivityDesigner.cs
- PropertiesTab.cs
- dsa.cs
- CommonDialog.cs
- PropertyDescriptor.cs
- AmbientProperties.cs
- NameValuePermission.cs
- EventRoute.cs
- ConsumerConnectionPointCollection.cs
- AddInControllerImpl.cs
- ObfuscationAttribute.cs
- LoginUtil.cs
- AbstractExpressions.cs
- ContentIterators.cs
- EmptyStringExpandableObjectConverter.cs
- PingOptions.cs
- TraceContextEventArgs.cs
- HasCopySemanticsAttribute.cs
- XmlSchemaType.cs
- BlockUIContainer.cs
- MailWebEventProvider.cs
- ReliableOutputSessionChannel.cs
- OrderingExpression.cs
- ClipboardData.cs
- ProfessionalColors.cs
- DataFormats.cs
- UpdatePanelTriggerCollection.cs
- AppSecurityManager.cs
- CodeMemberProperty.cs
- indexingfiltermarshaler.cs
- SmiRecordBuffer.cs
- DecoderBestFitFallback.cs
- XslNumber.cs
- SimpleFieldTemplateFactory.cs
- ConfigsHelper.cs
- DbParameterHelper.cs
- DynamicUpdateCommand.cs
- MenuItemBindingCollection.cs
- ProfileSection.cs
- EdmError.cs
- ListViewGroupCollectionEditor.cs
- DetailsViewUpdatedEventArgs.cs
- ApplicationDirectory.cs
- Facet.cs
- ObjectSet.cs
- DoubleKeyFrameCollection.cs
- XmlDomTextWriter.cs
- HtmlHistory.cs
- TargetInvocationException.cs
- WindowsListView.cs
- TemplatePropertyEntry.cs
- CorruptingExceptionCommon.cs
- StorageBasedPackageProperties.cs
- FixedSOMGroup.cs
- DbModificationCommandTree.cs
- ScrollViewer.cs
- SessionIDManager.cs
- DuplicateWaitObjectException.cs
- MD5CryptoServiceProvider.cs
- ToolStripHighContrastRenderer.cs
- BoundField.cs
- Panel.cs
- CodeTypeOfExpression.cs
- WindowShowOrOpenTracker.cs
- PolyBezierSegmentFigureLogic.cs
- LoadWorkflowByInstanceKeyCommand.cs
- TextServicesDisplayAttribute.cs
- CheckoutException.cs
- RepeaterItemCollection.cs
- RemoteArgument.cs
- DBSqlParser.cs
- DataGridViewColumnEventArgs.cs
- XslVisitor.cs
- HashCryptoHandle.cs
- DbMetaDataFactory.cs
- SqlCacheDependencyDatabase.cs
- DesignerCategoryAttribute.cs
- CardSpacePolicyElement.cs
- xmlglyphRunInfo.cs
- PropertyGroupDescription.cs
- SafeNativeMethods.cs
- ContractUtils.cs
- PathFigure.cs
- CompositeDuplexElement.cs