Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Animation / Generated / DoubleKeyFrameCollection.cs / 1305600 / DoubleKeyFrameCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; namespace System.Windows.Media.Animation { ////// This collection is used in conjunction with a KeyFrameDoubleAnimation /// to animate a Double property value along a set of key frames. /// public class DoubleKeyFrameCollection : Freezable, IList { #region Data private List_keyFrames; private static DoubleKeyFrameCollection s_emptyCollection; #endregion #region Constructors /// /// Creates a new DoubleKeyFrameCollection. /// public DoubleKeyFrameCollection() : base() { _keyFrames = new List< DoubleKeyFrame>(2); } #endregion #region Static Methods ////// An empty DoubleKeyFrameCollection. /// public static DoubleKeyFrameCollection Empty { get { if (s_emptyCollection == null) { DoubleKeyFrameCollection emptyCollection = new DoubleKeyFrameCollection(); emptyCollection._keyFrames = new List< DoubleKeyFrame>(0); emptyCollection.Freeze(); s_emptyCollection = emptyCollection; } return s_emptyCollection; } } #endregion #region Freezable ////// Creates a freezable copy of this DoubleKeyFrameCollection. /// ///The copy public new DoubleKeyFrameCollection Clone() { return (DoubleKeyFrameCollection)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new DoubleKeyFrameCollection(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { DoubleKeyFrameCollection sourceCollection = (DoubleKeyFrameCollection) sourceFreezable; base.CloneCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< DoubleKeyFrame>(count); for (int i = 0; i < count; i++) { DoubleKeyFrame keyFrame = (DoubleKeyFrame)sourceCollection._keyFrames[i].Clone(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { DoubleKeyFrameCollection sourceCollection = (DoubleKeyFrameCollection) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< DoubleKeyFrame>(count); for (int i = 0; i < count; i++) { DoubleKeyFrame keyFrame = (DoubleKeyFrame)sourceCollection._keyFrames[i].CloneCurrentValue(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { DoubleKeyFrameCollection sourceCollection = (DoubleKeyFrameCollection) sourceFreezable; base.GetAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< DoubleKeyFrame>(count); for (int i = 0; i < count; i++) { DoubleKeyFrame keyFrame = (DoubleKeyFrame)sourceCollection._keyFrames[i].GetAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { DoubleKeyFrameCollection sourceCollection = (DoubleKeyFrameCollection) sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< DoubleKeyFrame>(count); for (int i = 0; i < count; i++) { DoubleKeyFrame keyFrame = (DoubleKeyFrame)sourceCollection._keyFrames[i].GetCurrentValueAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetCurrentValueAsFrozenCore . ////// /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); for (int i = 0; i < _keyFrames.Count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_keyFrames[i], isChecking); } return canFreeze; } #endregion #region IEnumerable ////// Returns an enumerator of the DoubleKeyFrames in the collection. /// public IEnumerator GetEnumerator() { ReadPreamble(); return _keyFrames.GetEnumerator(); } #endregion #region ICollection ////// Returns the number of DoubleKeyFrames in the collection. /// public int Count { get { ReadPreamble(); return _keyFrames.Count; } } ////// See public bool IsSynchronized { get { ReadPreamble(); return (IsFrozen || Dispatcher != null); } } ///ICollection.IsSynchronized . ////// See public object SyncRoot { get { ReadPreamble(); return ((ICollection)_keyFrames).SyncRoot; } } ///ICollection.SyncRoot . ////// Copies all of the DoubleKeyFrames in the collection to an /// array. /// void ICollection.CopyTo(Array array, int index) { ReadPreamble(); ((ICollection)_keyFrames).CopyTo(array, index); } ////// Copies all of the DoubleKeyFrames in the collection to an /// array of DoubleKeyFrames. /// public void CopyTo(DoubleKeyFrame[] array, int index) { ReadPreamble(); _keyFrames.CopyTo(array, index); } #endregion #region IList ////// Adds a DoubleKeyFrame to the collection. /// int IList.Add(object keyFrame) { return Add((DoubleKeyFrame)keyFrame); } ////// Adds a DoubleKeyFrame to the collection. /// public int Add(DoubleKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Add(keyFrame); WritePostscript(); return _keyFrames.Count - 1; } ////// Removes all DoubleKeyFrames from the collection. /// public void Clear() { WritePreamble(); if (_keyFrames.Count > 0) { for (int i = 0; i < _keyFrames.Count; i++) { OnFreezablePropertyChanged(_keyFrames[i], null); } _keyFrames.Clear(); WritePostscript(); } } ////// Returns true of the collection contains the given DoubleKeyFrame. /// bool IList.Contains(object keyFrame) { return Contains((DoubleKeyFrame)keyFrame); } ////// Returns true of the collection contains the given DoubleKeyFrame. /// public bool Contains(DoubleKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.Contains(keyFrame); } ////// Returns the index of a given DoubleKeyFrame in the collection. /// int IList.IndexOf(object keyFrame) { return IndexOf((DoubleKeyFrame)keyFrame); } ////// Returns the index of a given DoubleKeyFrame in the collection. /// public int IndexOf(DoubleKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.IndexOf(keyFrame); } ////// Inserts a DoubleKeyFrame into a specific location in the collection. /// void IList.Insert(int index, object keyFrame) { Insert(index, (DoubleKeyFrame)keyFrame); } ////// Inserts a DoubleKeyFrame into a specific location in the collection. /// public void Insert(int index, DoubleKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Insert(index, keyFrame); WritePostscript(); } ////// Returns true if the collection is frozen. /// public bool IsFixedSize { get { ReadPreamble(); return IsFrozen; } } ////// Returns true if the collection is frozen. /// public bool IsReadOnly { get { ReadPreamble(); return IsFrozen; } } ////// Removes a DoubleKeyFrame from the collection. /// void IList.Remove(object keyFrame) { Remove((DoubleKeyFrame)keyFrame); } ////// Removes a DoubleKeyFrame from the collection. /// public void Remove(DoubleKeyFrame keyFrame) { WritePreamble(); if (_keyFrames.Contains(keyFrame)) { OnFreezablePropertyChanged(keyFrame, null); _keyFrames.Remove(keyFrame); WritePostscript(); } } ////// Removes the DoubleKeyFrame at the specified index from the collection. /// public void RemoveAt(int index) { WritePreamble(); OnFreezablePropertyChanged(_keyFrames[index], null); _keyFrames.RemoveAt(index); WritePostscript(); } ////// Gets or sets the DoubleKeyFrame at a given index. /// object IList.this[int index] { get { return this[index]; } set { this[index] = (DoubleKeyFrame)value; } } ////// Gets or sets the DoubleKeyFrame at a given index. /// public DoubleKeyFrame this[int index] { get { ReadPreamble(); return _keyFrames[index]; } set { if (value == null) { throw new ArgumentNullException(String.Format(CultureInfo.InvariantCulture, "DoubleKeyFrameCollection[{0}]", index)); } WritePreamble(); if (value != _keyFrames[index]) { OnFreezablePropertyChanged(_keyFrames[index], value); _keyFrames[index] = value; Debug.Assert(_keyFrames[index] != null); WritePostscript(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
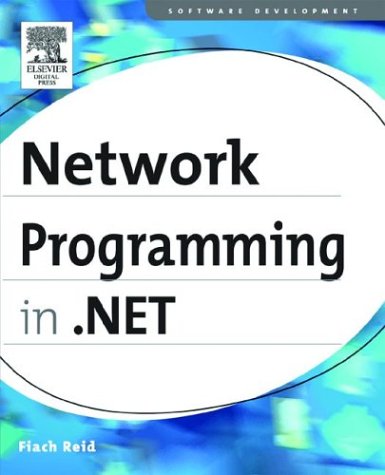
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesignerHelpers.cs
- AttributeCollection.cs
- DefaultWorkflowTransactionService.cs
- OpenFileDialog.cs
- FileIOPermission.cs
- StatusBarPanelClickEvent.cs
- ListViewInsertionMark.cs
- DataControlReferenceCollection.cs
- Wildcard.cs
- IItemProperties.cs
- TypographyProperties.cs
- BuildProvider.cs
- File.cs
- ContainerControl.cs
- ObjectQuery_EntitySqlExtensions.cs
- ResourceDictionaryCollection.cs
- QilTernary.cs
- ObjectAnimationUsingKeyFrames.cs
- WindowHelperService.cs
- TextFormatterContext.cs
- KeyBinding.cs
- MenuItemCollection.cs
- DbMetaDataColumnNames.cs
- HashSet.cs
- SingleAnimation.cs
- ComboBox.cs
- PtsPage.cs
- Int16.cs
- IdentitySection.cs
- CallSiteOps.cs
- Activity.cs
- QueryStringConverter.cs
- IntSecurity.cs
- ObservableDictionary.cs
- IteratorFilter.cs
- BufferAllocator.cs
- SafeLocalMemHandle.cs
- MetadataArtifactLoaderCompositeResource.cs
- Label.cs
- AppSettingsReader.cs
- ExceptionRoutedEventArgs.cs
- ProfileSection.cs
- ComponentResourceManager.cs
- NativeObjectSecurity.cs
- DbConnectionStringCommon.cs
- LayoutTableCell.cs
- CompositionTarget.cs
- XPathNode.cs
- AsyncCompletedEventArgs.cs
- _AutoWebProxyScriptHelper.cs
- SecurityTokenResolver.cs
- ServiceProviders.cs
- EntityDataSourceMemberPath.cs
- CorruptStoreException.cs
- Light.cs
- StylusOverProperty.cs
- StackOverflowException.cs
- IPEndPoint.cs
- InputProcessorProfiles.cs
- Size.cs
- RequestDescription.cs
- OdbcConnectionHandle.cs
- DbProviderConfigurationHandler.cs
- Native.cs
- Positioning.cs
- ContainerParagraph.cs
- WinInetCache.cs
- sqlinternaltransaction.cs
- SessionStateSection.cs
- Types.cs
- WebPartDisplayModeCancelEventArgs.cs
- RadioButtonRenderer.cs
- ObjectNavigationPropertyMapping.cs
- SortQueryOperator.cs
- ContentElementAutomationPeer.cs
- TemplatedAdorner.cs
- CompilationUnit.cs
- WebSysDisplayNameAttribute.cs
- TreeViewItemAutomationPeer.cs
- WmpBitmapEncoder.cs
- NamedPipeChannelListener.cs
- PassportAuthenticationEventArgs.cs
- XPathSelfQuery.cs
- Int32Rect.cs
- XamlStackWriter.cs
- RectIndependentAnimationStorage.cs
- RegexParser.cs
- ApplicationBuildProvider.cs
- ItemsControlAutomationPeer.cs
- InputManager.cs
- DataListItemEventArgs.cs
- diagnosticsswitches.cs
- InteropAutomationProvider.cs
- ThicknessKeyFrameCollection.cs
- XmlSchemaSequence.cs
- XmlChoiceIdentifierAttribute.cs
- SqlBuilder.cs
- WebChannelFactory.cs
- AccessDataSourceView.cs
- GenericUI.cs